Selenium Webdriver API(6)
33、右键 context_click 在某个对象上点击右键
#encoding=utf-8
from selenium import webdriver
import unittest
import time
from selenium.webdriver import ActionChains
import win32con
import win32clipboard as w
#设置剪切板
def setText(aString):
w.OpenClipboard()
w.EmptyClipboard()
w.SetClipboardData(win32con.CF_UNICODETEXT,aString)
w.CloseClipboard()
class visitSogouByIE(unittest.TestCase):
def setUp(self):
#启动浏览器
self.driver = webdriver.Ie(executable_path = "D:\\IEDriverServer")
def test_rightClick(self):
url = "http://www.sogou.com"
self.driver.get(url)
#找到搜索输入框
searchBox = self.driver.find_element_by_id("query")
#将焦点切换到搜索框
searchBox.click()
time.sleep(2)
#在搜索输入框中执行一个鼠标右键点击操作
ActionChains(self.driver).context_click(searchBox).perform()
#将某个数据设置到剪切板 “魔兽世界”,相当于执行了复制操作
setText(u"魔兽世界")
#发送一个粘贴命令,字符p代替粘贴操作
ActionChains(self.driver).send_keys("p").perform()
#点击搜索按钮
self.driver.find_element_by_id("stb").click()
def tearDown(self):
self.driver.quit()
if __name__ == "__main__":
unittest.main()
34、左键长按、释放 click_and_hold release
#encoding=utf-8
import time
import unittest
from selenium import webdriver
from selenium.webdriver import ActionChains
class visitLocalWebByIE(unittest.TestCase):
def setUp(self):
#启动浏览器
self.driver = webdriver.Ie(executable_path = "D:\\IEDriverServer")
def test_leftClickHoldAndRelease(self):
url = "http://127.0.0.1:8080/test_mouse.html"
#访问自定义网页
self.driver.get(url)
div = self.driver.find_element_by_id("div1")
#在id属性值为div1的元素上执行按下鼠标左键并保持
ActionChains(self.driver).click_and_hold(div).perform()
time.sleep(2)
#在id属性值为div1的元素上释放被一直按下的鼠标左键
ActionChains(self.driver).release(div).perform()
time.sleep(2)
ActionChains(self.driver).click_and_hold(div).perform()
time.sleep(2)
ActionChains(self.driver).release(div).perform()
def tearDown(self):
self.driver.quit()
if __name__ == "__main__":
unittest.main()
35、鼠标悬停 move_to_element
#encoding=utf-8
import time
import unittest
from selenium import webdriver
from selenium.webdriver import ActionChains
class visitLocalWebByIe(unittest.TestCase):
def setUp(self):
#启动浏览器
self.driver = webdriver.Ie(executable_path = "D:\\IEDriverServer")
def test_mouseHover(self):
url = "http://127.0.0.1:8080/test_mouse_hover.html"
#访问自定义网页
self.driver.get(url)
#找到页面上的第一个链接元素
link1 = self.driver.find_element_by_link_text(u"鼠标指过来1")
#找到页面上的第二个链接元素
link2 = self.driver.find_element_by_link_text(u"鼠标指过来2")
#找到页面上的p元素
p = self.driver.find_element_by_xpath("//p")
print link1.text,link2.text
#将鼠标悬浮在第一个链接元素上
ActionChains(self.driver).move_to_element(link1).perform()
time.sleep(2)
#将鼠标从第一个链接元素上移动到p元素
ActionChains(self.driver).move_to_element(p).perform()
time.sleep(2)
#将鼠标悬浮到第二个链接元素上
ActionChains(self.driver).move_to_element(link2).perform()
time.sleep(2)
#将鼠标从第二个链接元素上移到p元素
ActionChains(self.driver).move_to_element(p).perform()
time.sleep(2)
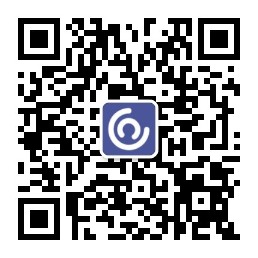
def tearDown(self):
self.driver.quit()
if __name__ == "__main__":
unittest.main()
36、判断元素是否在页面中 find_element
#encoding=utf-8
import time
import unittest
from selenium import webdriver
class visitSoGouByIe(unittest.TestCase):
def setUp(self):
#启动浏览器
self.driver = webdriver.Ie(executable_path = "D:\\IEDriverServer")
def isElementPresent(self,by,value):
#从selemium.common.exceptions模块导入NoSuchElementExcetion异常类
from selenium.common.exceptions import NoSuchElementException
try:
element = self.driver.find_element(by = by,value = value) #使用某种定位方式及值来定位元素
except NoSuchElementException,e:
#打印异常信息
print e
#发生NoSuchElementEception异常,说明页面中未找到该元素,则返回False
return False
else:
# 没有发生异常,表示在页面中找到了元素,返回True
return True
def test_isElementPresent(self):
url = "http://www.sogou.com"
#访问搜狗
self.driver.get(url)
#判断页面元素id属性值为"query"的页面元素是否存在
res = self.isElementPresent("id","query")
if res is True:
print u"所查找的元素存在于页面上!"
else:
print u"页面中未找到所需要的元素!"
def tearDown(self):
self.driver.quit()
if __name__ == "__main__":
unittest.main()