版权声明:转载或者引用请标明来源! https://blog.csdn.net/qq575792372/article/details/87461119
目录
React组件之间通信
一、父子组件之间的通信
这种主要是父组件直接传参子组件,子组件用props接收
// 父组件
class Animal extends React.Component{
constructor(props){
super(props)
}
render(){
return(
<div>
<Cat name="小猫"></Cat>
</div>
)
}
}
// 子组件
class Cat extends React.Component{
constructor(props){
super(props)
}
render(){
return(
<div>
// 这里用props接收到父组件的参数
{this.props.name}
</div>
)
}
}
二、子组件向父组件通信
// 父组件
class Animal extends React.Component{
constructor(props){
super(props)
this.state={
msg:'',
}
}
getMessage(value){
this.setState({msg:value})
}
render(){
return(
<div>
来自子组件传递的值:{this.state.msg}
<Cat name="小猫" setMessage={this.getMessage.bind(this)}></Cat>
</div>
)
}
}
// 子组件
class Cat extends React.Component{
constructor(props){
super(props)
}
componentDidMount(){
// 主要是这里,在子组件里调用父组件传递的setMessage方法就可以实现通信到父组件了
this.props.setMessage("我是子组件哦")
}
render(){
return(
<div>
</div>
)
}
}
三、子组件向另外一个子组件通信
第一种是通过相同的父组件来传参,可能麻烦一点,也就是子组件cat调用父组件animal,值传递到animal过去之后,animal再调用子组件dog,以此来达到兄弟组件之间的通信。
第二种是使用context来传递,官网上也有对应的例子,
// 父组件
class Animal extends React.Component{
constructor(props){
super(props)
}
getChildContext(){
return{
msg:''
}
}
render(){
return(
<div>
<Cat/>
<Dog/>
</div>
)
}
}
// 子组件1
class Cat extends React.Component{
constructor(props){
super(props)
}
componentDidMount(){
}
render(){
return(
<div>
{this.context.msg}
</div>
)
}
}
// 子组件2
class Dog extends React.Component{
constructor(props){
super(props)
}
componentDidMount(){
// 这里可以修改
this.context.msg="小狗呼叫小猫"
}
render(){
return(
<div>
{this.context.msg}
</div>
)
}
}
第三种,使用React的状态管理框架,例如redux以及mobx,同样可以达到数据多处可以访问的到的情况
扫描二维码关注公众号,回复:
5411700 查看本文章
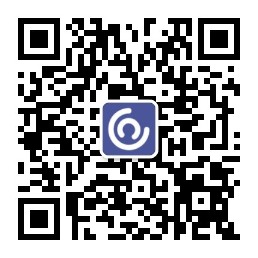