题面:
C. Rest Stops
Input file: standard input
Output file: standard output
Time limit: 1 second
Memory limit: 256 megabytes
Farmer John and his personal trainer Bessie are hiking up Mount Vancowver. For their purposes (and yours), the mountain can be represented as a long straight trail of length L meters (1 ≤ L ≤ 10^6). Farmer John will hike the trail at a constant travel rate of rF seconds per meter (1 ≤ rF ≤ 10^6). Since he is working on his stamina, he will not take any rest stops along the way.
Bessie, however, is allowed to take rest stops, where she might find some tasty grass. Of course, she cannot stop just anywhere! There are N rest stops along the trail (1 ≤ N ≤ 10^6); the i-th stop is xi meters from the start of the trail (0 < xi < L) and has a tastiness value ci (1 ≤ ci ≤ 10^6). If Bessie rests at stop i for t seconds, she receives ci ·t tastiness units.
When not at a rest stop,Bessie will be hiking at a fixed travel rate of rB seconds per meter(1 ≤ rB ≤ 10^6). Since Bessie is young and fit, rB is strictly less than rF.
Bessie would like to maximize her consumption of tasty grass. But she is worried about Farmer John; she thinks that if at any point along the hike she is behind Farmer John on the trail, he might lose all motivation to continue!
Help Bessie find the maximum total tastiness units she can obtain while making sure that Farmer John completes the hike.
Input
The first line of input contains four integers: L, N, rF, and rB. The next N lines describe the rest stops. For each i between 1 and N, the i + 1-st line contains two integers xi and ci, describing the position of the i-th rest stop and the tastiness of the grass there.
It is guaranteed that rF > rB, and 0 < x1 < ... < xN < L. Note that rF and rB are given in seconds per meter!
Output
A single integer: the maximum total tastiness units Bessie can obtain.
Example
Input
10 2 4 3
7 2
8 1
Output
15
Note
In this example, it is optimal for Bessie to stop for 7 seconds at the x = 7 rest stop (acquiring 14 tastiness units) and then stop for an additional 1 second at the x = 8 rest stop (acquiring 1 more tastiness unit, for a total of 15 tastiness units).
题目描述:
农夫和他的母牛Bessie一起去爬山,母牛跑地比农夫快。母牛可以在休息站停下来吃草,直到农夫赶上来了,奶牛就要继续前进。每个休息站的草都有对应的美味值,在休息站获得的美味度等于停留的时间乘于美味值,求怎样吃草才能才能使美味度总和最大。
题目分析:
这道题关键是黑体字:农夫和母牛的快慢是用每米多少秒来衡量的,也就是单位为s/m,而不是m/s!剩下就一直贪心就可以了。
我们可能会有这种想法(自己太渣了,只有自己才会有这种想法):直接把每个站的草吃一遍就能使美味度是最大的,但是这样的贪心策略是不对的。我们举个例子:

当奶牛的“速度为”a s/m,农夫的“速度”为b s/m时,如果先到距离为5的站,再到距离为6的站,总的停留时间为5*(b-a)+(6-5)*(b-a),化简一下就是6*(b-a);如果我们直接到距离为6的话,那么总的停留时间直接就是6*(b-a)了。我们会发现,先到距离为5的点吃草,再到距离为6的点吃草的停留时间和直接到距离为6吃草的停留时间是一样的。也就是说,在相同的停留时间内,要吃美味值最大的休息站的草,才能使总的美味度最大。如果我们先吃前面的草,再吃后面的草,就会使停留时间内有一些时间点的美味值不是最大的,就不符合题意。示意图:

总的美味度最大

总的美味度变小了
所以,我们每次都选取美味值最大的休息站的停留吃草,就可以使总的美味度最大了。
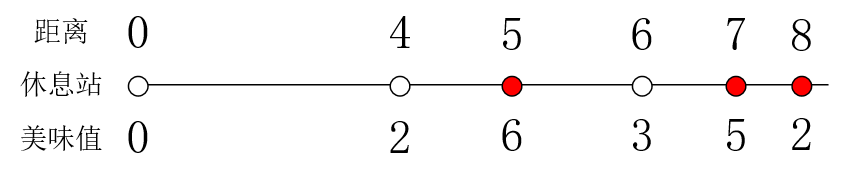
注意:当吃完最大的草后,要吃后面最大的草。在美味值2,6,3,5,2中,最大的美味值是6,所以先去美味值为6的休息站吃草。被农夫赶上来后,就去吃后面最大的草(后面最大美味值的休息站有:3,5,2),也就是美味值为5的休息站,同理最后去美味值为2的休息站。
AC代码:
1 #include <cstdio> 2 #include <cstring> 3 #include <iostream> 4 #include <cmath> 5 #include <set> 6 #include <algorithm> 7 using namespace std; 8 const int maxn = 1e5+5; 9 int l, n, rf, rb; 10 11 struct stop{ 12 int x; 13 long long c; 14 }; 15 16 stop s[maxn]; //记录休息站的信息 17 18 int max_c[maxn]; //用来记录后面休息站美味值最大的下标 19 20 void test(){ 21 cout << endl; 22 for(int i = 0; i < n; i++){ 23 cout << s[i].x << " " << s[i].c; 24 cout << endl; 25 } 26 27 cout << endl; 28 29 for(int i = 0; i < n; i++){ 30 cout << max_c[i] << endl; 31 } 32 cout << endl; 33 } 34 35 int main(){ 36 scanf("%d%d%d%d", &l, &n, &rf, &rb); 37 38 for(int i = 0; i < n; i++){ 39 scanf("%d%lld", &s[i].x, &s[i].c); 40 } 41 42 int maxx = 0; 43 int p; //记录上一个美味值最大的休息站的下标 44 45 for(int i = n-1; i >= 0; i--){ 46 if(maxx < s[i].c){ 47 maxx = s[i].c; 48 p = i; //记录下标 49 } 50 max_c[i] = p; //不断更新从最后面到当前休息站美味值最大的下标 51 } 52 max_c[n] = -1; //结束标记 53 54 //test(); 测试用 55 56 int temp = 0; //上一个停留的休息站的位置 57 long long u, dis, d; 58 long long res = 0; 59 for(int i = 0; i != -1; i = max_c[i+1]){ 60 u = max_c[i]; //获得美味值最大的休息站的下标 61 dis = s[u].x - temp; 62 63 d = dis*(rf-rb); //停留时间 64 res += d*s[u].c; 65 66 temp = s[u].x; //记录停留站位置 67 } 68 69 cout << res << endl; 70 return 0; 71 }