第一步:分析
把贪吃蛇这个游戏当做一个对象,他又由一下部分组成:
1.小蛇;
2.食物;
3.游戏背景(地图)
同样我们将这三部分也当做三个对象,逐个分析,对它们添加自己的属性和方法,因为地图比较简单只需要设置样式即可,就不做分析,分析顺序按照难度从易到难来分析;(形参皆为游戏对象后期传进去的属性)
一,食物
(1)属性
function Food(width, height, color) {
this.width = width || 20
this.height = height || 20
this.color = color || “green”
this.x = 0;
this.y = 0;
}
(2)运用对象的原型对对象设置方法—>生成食物初始化每个食物(即每次只有一个食物产生),remove()是为了初始化每个食物(即每次只有一个食物产生);
//原型对象添加方法—>把食物显示在地图上的
Food.prototype.init = function (map) {
remove();
//1.创建小盒子
var div = document.createElement(“div”);
//2.div添加到map中
map.appendChild(div);
//3.设置样式
div.style.width = this.width + “px”;
div.style.height = this.height + “px”;
div.style.backgroundColor = this.color;
div.style.position = “absolute”;
//生成随机数
console.log();
this.x = parseInt(Math.random() * (map.offsetWidth / this.width)) * this.width;
this.y = parseInt(Math.random() * (map.offsetHeight / this.height)) * this.height
div.style.left = this.x + “px”;
div.style.top = this.y + “px”;
elements.push(div);
}
function remove() {
for (var i = 0; i < elements.length; i++) {
elements[i].parentElement.removeChild(elements[i]);
elements.splice(i, 1);
}
}
3.用函数自调用的方法包裹食物对象,并且将Food暴露在window下
二,小蛇
(1)添加小蛇的属性
function Snack(width, height, direction) {
this.width = width || 20;
this.height = height || 20;
this.direction = direction || “right”
this.beforeDirection = this.direction;
this.body = [
{x: 3, y: 2, color: “red”,},//头部
{x: 2, y: 2, color: “orange”},
{x: 1, y: 2, color: “orange”},
]
}
(2)生成小蛇,并用remove初始化小蛇
Snack.prototype.init = function (map) {
remove();
function remove() {
for (var i = 0; i < elements.length; i++) {
elements[i].parentElement.removeChild(elements[i]);
}
elements.splice(0, elements.length);
}
for (var i = 0; i < this.body.length; i++) {
var div = document.createElement("div")
map.appendChild(div);
div.style.width = this.width + "px";
div.style.height = this.height + "px";
div.style.backgroundColor = this.body[i].color;
div.style.borderRadius = "50%";
div.style.position = "absolute";
div.style.left = this.body[i].x * this.width + "px";
div.style.top = this.body[i].y * this.width + "px";
elements.push(div);
}
}
(3)用原型给小蛇添加移动函数
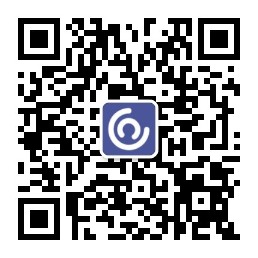
Snack.prototype.move = function (food, map) {
var index = this.body.length - 1;
for (var i = index; i > 0; i–) {
this.body[i].x = this.body[i - 1].x;
this.body[i].y = this.body[i - 1].y;
}
//判断小蛇头部坐标的位置
switch (this.direction) {
case"right":
this.body[0].x += 1;
break;
case"left":
this.body[0].x -= 1;
break;
case"top":
this.body[0].y -= 1;
break;
case"buttom":
this.body[0].y += 1;
break;
}
//判断蛇头的坐标
//获取蛇头的坐标
var headX=this.body[0].xthis.width;
var headY=this.body[0].ythis.height;
var foodX=food.x;
var foodY=food.y;
if (headXfoodX&&headYfoodY) {
food.init(map);
var last=this.body[this.body.length-1];
this.body.push({
x:last.x,
y:last.y,
color:last.color
})
}
// console.log(this.body.length);
my$(“num1”).innerHTML=this.body.length-3;
}
(4)同样用函数的自调用包裹小蛇整体,暴露给window
第二步:将小蛇,食物,背景三个对象添加到游戏对象中
function Game() {
this.food = new Food();
this.snack = new Snack();
this.map = my$(“map”);
}
第三步:设置游戏规则,换言之就是讲规则当做游戏的方法添加到游戏对象中
/1.不能碰壁
Game.prototype.runSnack=function(){
var that=this;
//移动
var timeId=setInterval(function () {
this.snack.move(this.food,this.map);
this.snack.init(this.map);
//判断横纵坐标最大值
var maxX=map.offsetWidth/this.snack.width;
var maxY=map.offsetHeight/this.snack.height;
//蛇头的坐标
var headX=this.snack.body[0].x;
var headY=this.snack.body[0].y;
if (headX<0||headX>=maxX){
clearInterval(timeId);
alert(“游戏结束”);
}
if (headY<0||headY>=maxY){
clearInterval(timeId);
alert(“游戏结束”);
}
//把这个函数绑定在that指向上
}.bind(that),100)
}
/2.改变方向
Game.prototype.keyDown=function(){
var that=this;
document.addEventListener(“keydown”,function (e) {
this.snack.beforeDirection = this.snack.direction;
switch (e.keyCode){
case 37:
this.snack.beforeDirection !== “right” ? this.snack.direction = “left”: this.snack.direction = “right”;
break;
case 38:
this.snack.beforeDirection !== “buttom” ? this.snack.direction = “top”: this.snack.direction = “buttom”;
break;
case 39:
this.snack.beforeDirection !== “left” ? this.snack.direction = “right”: this.snack.direction = “left”;
break;
case 40:
this.snack.beforeDirection !== “top” ? this.snack.direction = “buttom”: this.snack.direction = “top”;
break;
}
}.bind(that))
};
/3.调用小蛇,食物,两个规则
Game.prototype.init = function () {
//显示食物和小蛇
this.food.init(this.map);
this.snack.init(this.map);
//调用移动函数
this.runSnack();
this.keyDown(this.map)
}
/4.包裹函数,暴露在window中
第四步:调用游戏对象,实现效果
var game=new Game();
game.init();
第五步大功告成!
总结:难度不大,思路很重要,尤其是将对象灵活使用十分关键,还有通过原型添加对象的方法也很关键,只要熟练掌握这两点即可轻松解决
ps:纯属原创,抄袭必究!