备忘录模式:在不破坏封装性的前提下,捕获一个对象的内部状态,并在该对象之外保存这个状态。这样以后就可以将对象恢复到原先保存的状态。【组合】
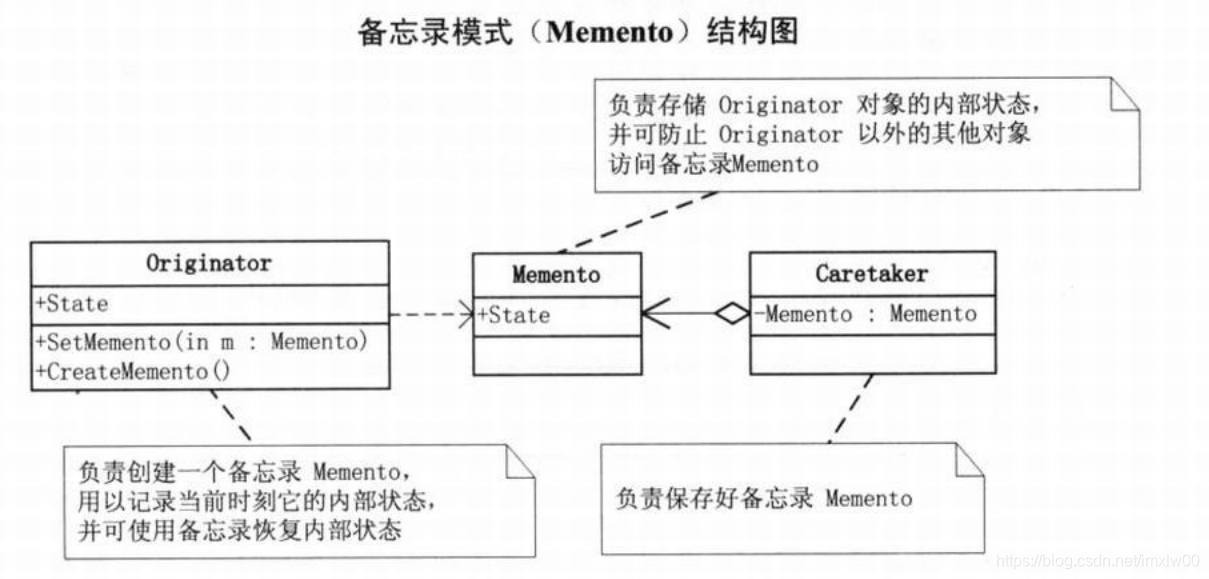
public class Memento {
private String state;
public Memento(String state) {
this.state = state;
}
public String getState() {
return state;
}
public void setState(String state) {
this.state = state;
}
}
public class Originator {
private String state;
public Memento createMemento() {
return new Memento(this.state);
}
public void recoverMemento(Memento memento) {
this.setState(memento.getState());
}
public void show() {
System.out.println("state = " + this.state);
}
public String getState() {
return state;
}
public void setState(String state) {
this.state = state;
}
}
public class CareTaker {
private Memento memento;
public Memento getMemento() {
return memento;
}
public void setMemento(Memento memento) {
this.memento = memento;
}
}
public class MementoClient {
public static void main(String[] args) {
Originator originator = new Originator();
originator.setState("On");
originator.show();
CareTaker careTaker = new CareTaker();
careTaker.setMemento(originator.createMemento());
originator.setState("Off");
originator.show();
originator.recoverMemento(careTaker.getMemento());
originator.show();
}
}
案例 游戏
public class GameRole {
private int vit;
private int atk;
private int def;
public int getVit() {
return vit;
}
public void setVit(int vit) {
this.vit = vit;
}
public int getAtk() {
return atk;
}
public void setAtk(int atk) {
this.atk = atk;
}
public int getDef() {
return def;
}
public void setDef(int def) {
this.def = def;
}
public void stateDisplay() {
System.out.println("角色当前状态:");
System.out.println("体力:" + this.vit);
System.out.println("体力:" + this.atk);
System.out.println("体力:" + this.def);
System.out.println(" ");
}
public void getInitState() {
this.vit = 100;
this.atk = 100;
this.def = 100;
}
public void fight() {
this.vit = 0;
this.atk = 0;
this.def = 0;
}
public RoleStateMemento saveState() {
return new RoleStateMemento(vit, atk, def);
}
public void recoveryState(RoleStateMemento memento) {
this.vit = memento.getVit();
this.atk = memento.getAtk();
this.def = memento.getDef();
}
}
public class RoleStateMemento {
private int vit;
private int atk;
private int def;
public RoleStateMemento(int vit, int atk, int def) {
this.vit = vit;
this.atk = atk;
this.def = def;
}
public int getVit() {
return vit;
}
public void setVit(int vit) {
this.vit = vit;
}
public int getAtk() {
return atk;
}
public void setAtk(int atk) {
this.atk = atk;
}
public int getDef() {
return def;
}
public void setDef(int def) {
this.def = def;
}
}
public class RoleStateCaretaker {
private RoleStateMemento memento;
public RoleStateMemento getMemento() {
return memento;
}
public void setMemento(RoleStateMemento memento) {
this.memento = memento;
}
}
public class Client {
public static void main(String[] args) {
GameRole lixiaoyao = new GameRole();
lixiaoyao.getInitState();
lixiaoyao.stateDisplay();
RoleStateCaretaker stateAdmin = new RoleStateCaretaker();
stateAdmin.setMemento(lixiaoyao.saveState());
lixiaoyao.fight();
lixiaoyao.stateDisplay();
lixiaoyao.recoveryState(stateAdmin.getMemento());
lixiaoyao.stateDisplay();
}
}