版权声明:转载的话 请标明出处 https://blog.csdn.net/qq_28198181/article/details/83794964
在使用java的时候 希望从excel中读取到一些单元格的数据
1.Java读取的excel的api
这里用到了一个叫jxl的api如下:
<dependency>
<groupId>net.sourceforge.jexcelapi</groupId>
<artifactId>jxl</artifactId>
<version>2.6.12</version>
</dependency>
在java中需要去导入一些类去读取excel
import jxl.Workbook; //java读取excel表使用的类
import jxl.Cell; //java读取表格里的单元格的类
import jxl.Sheet; //java读取的工作铺的类
首先:
创建一个File 去读取文件(我以D盘redisInput文件下的GPSINFO.xls文件为例)
注意:不能够读取xlsx后缀的excel文件,否则会报错: Unable to recognize OLE stream
File Inputfile = new File("D:\\redisInput\\GPSINFO.xls");
使用字符流去接File的数据
FileInputStream fileInputStream = new FileInputStream(Inputfile);
workbook去接fileInputStream
Workbook workbook = Workbook.getWorkbook(fileInputStream);
这样读取到了excel文件,但是需要去判断是哪一个工作簿,要用到Sheet类
Sheet readfirst = workbook.getSheet(0);
如果getsheet(0)那么就是去访问第一个工作簿里的数据,然后可以在sheet类中看有多少有效行和有效列
扫描二维码关注公众号,回复:
4650561 查看本文章
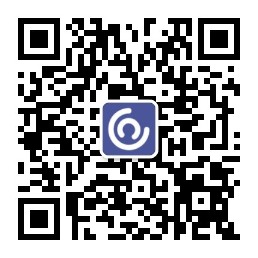
int rows = readfirst.getRows();
int clomns = readfirst.getColumns();
System.out.println("row:" + rows);
System.out.println("clomns:" + clomns);
如果想看每个单元格的数据可以使用一个双重循环去读取每一个有效单元格里数据
for(int i =1;i<rows;i++) {
for(int j =1;i<rows;i++) {
Cell cell = readfirst.getCell(j,i); //j在前 i 在后是根据excel下标来判断的
String s = cell.getContents();
System.out.println("cell"+s);
}
这样就把所有的有效单元格输出了。
2.读到的单元格进行打印
但是我想让它按照我想要的格式进行输出,比如 excel表中有sim卡 ,车牌号,终端号,我希望让它能够生成一个特有的格式让我使用比如:
转成-》
这种格式的数据
所以一个完整过程如下:
public class AnalysisExcel {
Workbook workbook = null;
File Inputfile = new File("D:\\redisInput\\GPSINFO.xls");
File outputfile =new File("D:\\redisInput\\GPSINFO.txt");
public void ToAnalysisExcel() {
// Unable to recognize OLE stream 不支持xlsx格式 支持xls格式
try {
FileInputStream fileInputStream = new FileInputStream(Inputfile);
workbook = Workbook.getWorkbook(fileInputStream);
FileOutputStream fileOutputStream = new FileOutputStream(outputfile);
BufferedOutputStream bw = new BufferedOutputStream(fileOutputStream); //输出语句
Sheet readfirst = workbook.getSheet(0);
int rows = readfirst.getRows();
int clomns = readfirst.getColumns();
System.out.println("row:" + rows);
System.out.println("clomns:" + clomns);
for(int i =1;i<rows;i++) {
Cell[] cells = readfirst.getRow(i); //循环得到每一行的单元格对象
//根据每一个单元格对象的到里面的值
String brandNum= cells[0].getContents();
String deviceCode = cells[1].getContents();
String sim =cells[2].getContents();
System.out.println("rand:"+brandNum+",vehicleNum:"+deviceCode+",sim:"+sim);
//将得到的值放在一个我需要的格式的string对象中
String output = "\n"+sim+"\n" +
"{\n" +
" \"brandColor\": 500000,\n" +
" \"brandNumber\": \""+brandNum+"\",\n" +
" \"deviceCode\": \""+deviceCode+"\",\n" +
" \"simCard\": \""+sim+"\"\n" +
"}"+
"\n";
//write and flush到 我需要的文件中去,flush后才能成功
byte[] outputbyte = new String(output).getBytes();
bw.write(outputbyte);
bw.flush();
}
} catch (FileNotFoundException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
} catch (BiffException e) {
e.printStackTrace();
}
}
}