前台:
$.ajax({ type: "get", url: consts.dataServerHost+'/后台名称/data/getRainData', dataType: "jsonp", jsonp: 'callbackName', data: { path: "excel文件路径", dataFormat:"jsonp" }, success: function (data) { let htmlStr = ""; for (let i in data) { htmlStr += `<li class='datetime-list' id='pe-${data[i]}'><span>${data[i]}</span></li>`; } $("#rainfall-date").html(htmlStr); for (let i in data) { $("#pe-" + data[i]).click(function () { let checked = false; if ($(this).hasClass("actived")) { $(this).removeClass("actived"); $(".rainfall-datetime ul li").removeClass("actived"); } else { $(".rainfall-datetime ul li").removeClass("actived"); $(this).addClass("actived"); checked = true; // evt.fire("syscontrol_baseinfo", { firer: this, data: { type: "rainfall", display: checked} }); } }); } }, error: function (e) { } });后台:
两个文件位置如下所示:
dataManager:
@RequestMapping("/getRainData") public String getRainData(HttpServletRequest req, HttpServletResponse res) throws Exception { String localpath=req.getParameter("path"); File file = new File(localpath); String dateStr = ""; JSONArray jsonArray = new JSONArray(); if(file.exists()){ ArrayList<ArrayList<Object>> result = ExcelUtil.readExcel(file); for(int i = 0 ;i < result.size() ;i++){ if(!result.get(i).get(0).toString().isEmpty()&&!result.get(i).get(0).toString().equals("时间")){ // System.out.println(result.get(i).get(0).toString().substring(0,10)); String temp = result.get(i).get(0).toString().substring(0,10); if(!dateStr.contains(temp)) dateStr += temp+","; } } dateStr = dateStr.substring(0,dateStr.length()-1); String [] list = dateStr.split(","); for(int i=0;i<list.length;i++){ jsonArray.add(list[i]); } } if (req.getParameter("dataFormat") != null) { String dataFormat = req.getParameter("dataFormat").trim().toLowerCase(); if (dataFormat.equals("jsonp")) { String jsonp = req.getParameter("callbackName"); return jsonp + "(" + jsonArray.toString() + ")"; } } return jsonArray.toString(); }
excel:
//默认单元格内容为数字时格式 private static DecimalFormat df = new DecimalFormat("0"); // 默认单元格格式化日期字符串 private static SimpleDateFormat sdf = new SimpleDateFormat( "yyyy-MM-dd HH:mm:ss"); // 格式化数字 private static DecimalFormat nf = new DecimalFormat("0.00"); public static ArrayList<ArrayList<Object>> readExcel(File file){ if(file == null){ return null; } if(file.getName().endsWith("xlsx")){ //处理ecxel2007 return readExcel2007(file); }else{ //处理ecxel2003 return readExcel2003(file); } } /* * @return 将返回结果存储在ArrayList内,存储结构与二位数组类似 * lists.get(0).get(0)表示过去Excel中0行0列单元格 */ public static ArrayList<ArrayList<Object>> readExcel2003(File file){ try{ ArrayList<ArrayList<Object>> rowList = new ArrayList<ArrayList<Object>>(); ArrayList<Object> colList; HSSFWorkbook wb = new HSSFWorkbook(new FileInputStream(file)); HSSFSheet sheet = wb.getSheetAt(0); HSSFRow row; HSSFCell cell; Object value; for(int i = sheet.getFirstRowNum() , rowCount = 0; rowCount < sheet.getPhysicalNumberOfRows() ; i++ ){ row = sheet.getRow(i); colList = new ArrayList<Object>(); if(row == null){ //当读取行为空时 if(i != sheet.getPhysicalNumberOfRows()){//判断是否是最后一行 rowList.add(colList); } continue; }else{ rowCount++; } for( int j = row.getFirstCellNum() ; j <= row.getLastCellNum() ;j++){ cell = row.getCell(j); if(cell == null || cell.getCellType() == HSSFCell.CELL_TYPE_BLANK){ //当该单元格为空 if(j != row.getLastCellNum()){//判断是否是该行中最后一个单元格 colList.add(""); } continue; } switch(cell.getCellType()){ case XSSFCell.CELL_TYPE_STRING: //System.out.println(i + "行" + j + " 列 is String type"); value = cell.getStringCellValue(); break; case XSSFCell.CELL_TYPE_NUMERIC: if ("@".equals(cell.getCellStyle().getDataFormatString())) { value = df.format(cell.getNumericCellValue()); } else if ("General".equals(cell.getCellStyle() .getDataFormatString())) { value = nf.format(cell.getNumericCellValue()); } else { value = sdf.format(HSSFDateUtil.getJavaDate(cell .getNumericCellValue())); } //System.out.println(i + "行" + j // + " 列 is Number type ; DateFormt:" // + value.toString()); break; case XSSFCell.CELL_TYPE_BOOLEAN: //System.out.println(i + "行" + j + " 列 is Boolean type"); value = Boolean.valueOf(cell.getBooleanCellValue()); break; case XSSFCell.CELL_TYPE_BLANK: //System.out.println(i + "行" + j + " 列 is Blank type"); value = ""; break; default: //System.out.println(i + "行" + j + " 列 is default type"); value = cell.toString(); }// end switch colList.add(value); }//end for j rowList.add(colList); }//end for i return rowList; }catch(Exception e){ return null; } } public static ArrayList<ArrayList<Object>> readExcel2007(File file){ try{ ArrayList<ArrayList<Object>> rowList = new ArrayList<ArrayList<Object>>(); ArrayList<Object> colList; XSSFWorkbook wb = new XSSFWorkbook(new FileInputStream(file)); XSSFSheet sheet = wb.getSheetAt(0); XSSFRow row; XSSFCell cell; Object value; for(int i = sheet.getFirstRowNum() , rowCount = 0; rowCount < sheet.getPhysicalNumberOfRows() ; i++ ){ row = sheet.getRow(i); colList = new ArrayList<Object>(); if(row == null){ //当读取行为空时 if(i != sheet.getPhysicalNumberOfRows()){//判断是否是最后一行 rowList.add(colList); } continue; }else{ rowCount++; } for( int j = row.getFirstCellNum() ; j <= row.getLastCellNum() ;j++){ cell = row.getCell(j); if(cell == null || cell.getCellType() == HSSFCell.CELL_TYPE_BLANK){ //当该单元格为空 if(j != row.getLastCellNum()){//判断是否是该行中最后一个单元格 colList.add(""); } continue; } switch(cell.getCellType()){ case XSSFCell.CELL_TYPE_STRING: //System.out.println(i + "行" + j + " 列 is String type"); value = cell.getStringCellValue(); break; case XSSFCell.CELL_TYPE_NUMERIC: if ("@".equals(cell.getCellStyle().getDataFormatString())) { value = df.format(cell.getNumericCellValue()); } else if ("General".equals(cell.getCellStyle() .getDataFormatString())) { value = nf.format(cell.getNumericCellValue()); } else { value = sdf.format(HSSFDateUtil.getJavaDate(cell .getNumericCellValue())); } //System.out.println(i + "行" + j // + " 列 is Number type ; DateFormt:" // + value.toString()); break; case XSSFCell.CELL_TYPE_BOOLEAN: //System.out.println(i + "行" + j + " 列 is Boolean type"); value = Boolean.valueOf(cell.getBooleanCellValue()); break; case XSSFCell.CELL_TYPE_BLANK: //System.out.println(i + "行" + j + " 列 is Blank type"); value = ""; break; default: //System.out.println(i + "行" + j + " 列 is default type"); value = cell.toString(); }// end switch colList.add(value); }//end for j rowList.add(colList); }//end for i return rowList; }catch(Exception e){ //System.out.println("exception"); return null; } } public static void writeExcel(ArrayList<ArrayList<Object>> result,String path){ if(result == null){ return; } HSSFWorkbook wb = new HSSFWorkbook(); HSSFSheet sheet = wb.createSheet("sheet1"); for(int i = 0 ;i < result.size() ; i++){ HSSFRow row = sheet.createRow(i); if(result.get(i) != null){ for(int j = 0; j < result.get(i).size() ; j ++){ HSSFCell cell = row.createCell(j); cell.setCellValue(result.get(i).get(j).toString()); } } } ByteArrayOutputStream os = new ByteArrayOutputStream(); try { wb.write(os); } catch (IOException e){ e.printStackTrace(); } byte[] content = os.toByteArray(); File file = new File(path);//Excel文件生成后存储的位置。 OutputStream fos = null; try { fos = new FileOutputStream(file); fos.write(content); os.close(); fos.close(); }catch (Exception e){ e.printStackTrace(); } } public static DecimalFormat getDf() { return df; } public static void setDf(DecimalFormat df) { ExcelUtil.df = df; } public static SimpleDateFormat getSdf() { return sdf; } public static void setSdf(SimpleDateFormat sdf) { ExcelUtil.sdf = sdf; } public static DecimalFormat getNf() { return nf; } public static void setNf(DecimalFormat nf) { ExcelUtil.nf = nf; }
扫描二维码关注公众号,回复:
124883 查看本文章
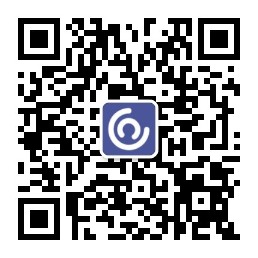