https://github.com/DamonOehlman/detect-browser
detect-browser
This is a package that attempts to detect a browser vendor and version (in a semver compatible format) using a navigator useragent in a browser or process.version
in node.
这个包使用浏览器中的navigator useragent或node中的process.version
检测浏览器厂商和版本(与semver兼容的格式)
Example Usage
const { detect } = require('detect-browser'); const browser = detect(); // handle the case where we don't detect the browser if (browser) { console.log(browser.name); console.log(browser.version); console.log(browser.os); }
Or you can use a switch statement:
const { detect } = require('detect-browser'); const browser = detect(); // handle the case where we don't detect the browser switch (browser && browser.name) { case 'chrome': case 'firefox': console.log('supported'); break; case 'edge': console.log('kinda ok'); break; default: console.log('not supported'); }
看下图控制器第一行的输出我们就能够看见得到的是{name : "chrome",version : "70.0.3538", os : "Mac OS"}
Adding additional browser support
The current list of browsers that can be detected by detect-browser
is not exhaustive. If you have a browser that you would like to add support for then please submit a pull request with the implementation.
但是目前能够被detect-browser
这个库检测到的浏览器列表还不够详尽。如果你有想要加入的浏览器,那么就请提交一个实现的请求
Creating an acceptable implementation requires two things需要两样东西:
- A test demonstrating that the regular expression you have defined identifies your new browser correctly. Examples of this can be found in the
test/logic.js
file.test测试中阐述了你用来正确识别你的浏览器的自定义的正则表达式,例子如test/logic.js,看下面
- Write the actual regex to the
index.js
file. In most cases adding the regex to the list of existing regexes will be suitable (if usage ofdetect-brower
returnsundefined
for instance), but in some cases you might have to add it before an existing regex. This would be true for a case where you have a browser that is a specialised variant of an existing browser but is identified as the non-specialised case.在index.js中写下真是的正则表达式。在大多数情况下列表中已存在的正则表达式与添加进的是相配的,但是在一些情况下你就必须要将其添加在已存在的正则表达式之前。如果您的浏览器是现有浏览器的特殊版本,但被识别为非特殊的,那么这种情况就会发生
When writing the regular expression remember that you would write it containing a single capturing group which captures the version number of the browser.
在编写正则表达式时,请记住,您将编写包含捕获浏览器版本号的单个捕获组的表达式
detect-browser/index.js
function detect() { if (typeof navigator !== 'undefined') {//下面图中有显示navigator return parseUserAgent(navigator.userAgent);//navigator.userAgent结果为:Mozilla/5.0 (Macintosh; Intel Mac OS X 10_13_6) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/70.0.3538.77 Safari/537.36 } return getNodeVersion(); } function detectOS(userAgentString) { var rules = getOperatingSystemRules();//匹配操作系统的正则表达式 var detected = rules.filter(function (os) { return os.rule && os.rule.test(userAgentString); })[0]; return detected ? detected.name : null; } function getNodeVersion() { var isNode = typeof process !== 'undefined' && process.version; return isNode && { name: 'node', version: process.version.slice(1), os: process.platform }; } function parseUserAgent(userAgentString) { var browsers = getBrowserRules();//得到浏览器的正则表达式 if (!userAgentString) { return null; } var detected = browsers.map(function(browser) { var match = browser.rule.exec(userAgentString); var version = match && match[1].split(/[._]/).slice(0,3); if (version && version.length < 3) { version = version.concat(version.length == 1 ? [0, 0] : [0]); } return match && { name: browser.name, version: version.join('.') }; }).filter(Boolean)[0] || null; if (detected) { detected.os = detectOS(userAgentString); } if (/alexa|bot|crawl(er|ing)|facebookexternalhit|feedburner|google web preview|nagios|postrank|pingdom|slurp|spider|yahoo!|yandex/i.test(userAgentString)) { detected = detected || {}; detected.bot = true; } return detected; } function getBrowserRules() { //返回相应的浏览器的正则表达式 return buildRules([ [ 'aol', /AOLShield\/([0-9\._]+)/ ], [ 'edge', /Edge\/([0-9\._]+)/ ], [ 'yandexbrowser', /YaBrowser\/([0-9\._]+)/ ], [ 'vivaldi', /Vivaldi\/([0-9\.]+)/ ], [ 'kakaotalk', /KAKAOTALK\s([0-9\.]+)/ ], [ 'samsung', /SamsungBrowser\/([0-9\.]+)/ ], [ 'chrome', /(?!Chrom.*OPR)Chrom(?:e|ium)\/([0-9\.]+)(:?\s|$)/ ], [ 'phantomjs', /PhantomJS\/([0-9\.]+)(:?\s|$)/ ], [ 'crios', /CriOS\/([0-9\.]+)(:?\s|$)/ ], [ 'firefox', /Firefox\/([0-9\.]+)(?:\s|$)/ ], [ 'fxios', /FxiOS\/([0-9\.]+)/ ], [ 'opera', /Opera\/([0-9\.]+)(?:\s|$)/ ], [ 'opera', /OPR\/([0-9\.]+)(:?\s|$)$/ ], [ 'ie', /Trident\/7\.0.*rv\:([0-9\.]+).*\).*Gecko$/ ], [ 'ie', /MSIE\s([0-9\.]+);.*Trident\/[4-7].0/ ], [ 'ie', /MSIE\s(7\.0)/ ], [ 'bb10', /BB10;\sTouch.*Version\/([0-9\.]+)/ ], [ 'android', /Android\s([0-9\.]+)/ ], [ 'ios', /Version\/([0-9\._]+).*Mobile.*Safari.*/ ], [ 'safari', /Version\/([0-9\._]+).*Safari/ ], [ 'facebook', /FBAV\/([0-9\.]+)/], [ 'instagram', /Instagram\s([0-9\.]+)/], [ 'ios-webview', /AppleWebKit\/([0-9\.]+).*Mobile/] ]); } function getOperatingSystemRules() {//匹配操作系统的正则表达式 return buildRules([ [ 'iOS', /iP(hone|od|ad)/ ], [ 'Android OS', /Android/ ], [ 'BlackBerry OS', /BlackBerry|BB10/ ], [ 'Windows Mobile', /IEMobile/ ], [ 'Amazon OS', /Kindle/ ], [ 'Windows 3.11', /Win16/ ], [ 'Windows 95', /(Windows 95)|(Win95)|(Windows_95)/ ], [ 'Windows 98', /(Windows 98)|(Win98)/ ], [ 'Windows 2000', /(Windows NT 5.0)|(Windows 2000)/ ], [ 'Windows XP', /(Windows NT 5.1)|(Windows XP)/ ], [ 'Windows Server 2003', /(Windows NT 5.2)/ ], [ 'Windows Vista', /(Windows NT 6.0)/ ], [ 'Windows 7', /(Windows NT 6.1)/ ], [ 'Windows 8', /(Windows NT 6.2)/ ], [ 'Windows 8.1', /(Windows NT 6.3)/ ], [ 'Windows 10', /(Windows NT 10.0)/ ], [ 'Windows ME', /Windows ME/ ], [ 'Open BSD', /OpenBSD/ ], [ 'Sun OS', /SunOS/ ], [ 'Linux', /(Linux)|(X11)/ ], [ 'Mac OS', /(Mac_PowerPC)|(Macintosh)/ ], [ 'QNX', /QNX/ ], [ 'BeOS', /BeOS/ ], [ 'OS/2', /OS\/2/ ], [ 'Search Bot', /(nuhk)|(Googlebot)|(Yammybot)|(Openbot)|(Slurp)|(MSNBot)|(Ask Jeeves\/Teoma)|(ia_archiver)/ ] ]); } function buildRules(ruleTuples) { return ruleTuples.map(function(tuple) { return { name: tuple[0], rule: tuple[1] }; }); } module.exports = { detect: detect, detectOS: detectOS, getNodeVersion: getNodeVersion, parseUserAgent: parseUserAgent };
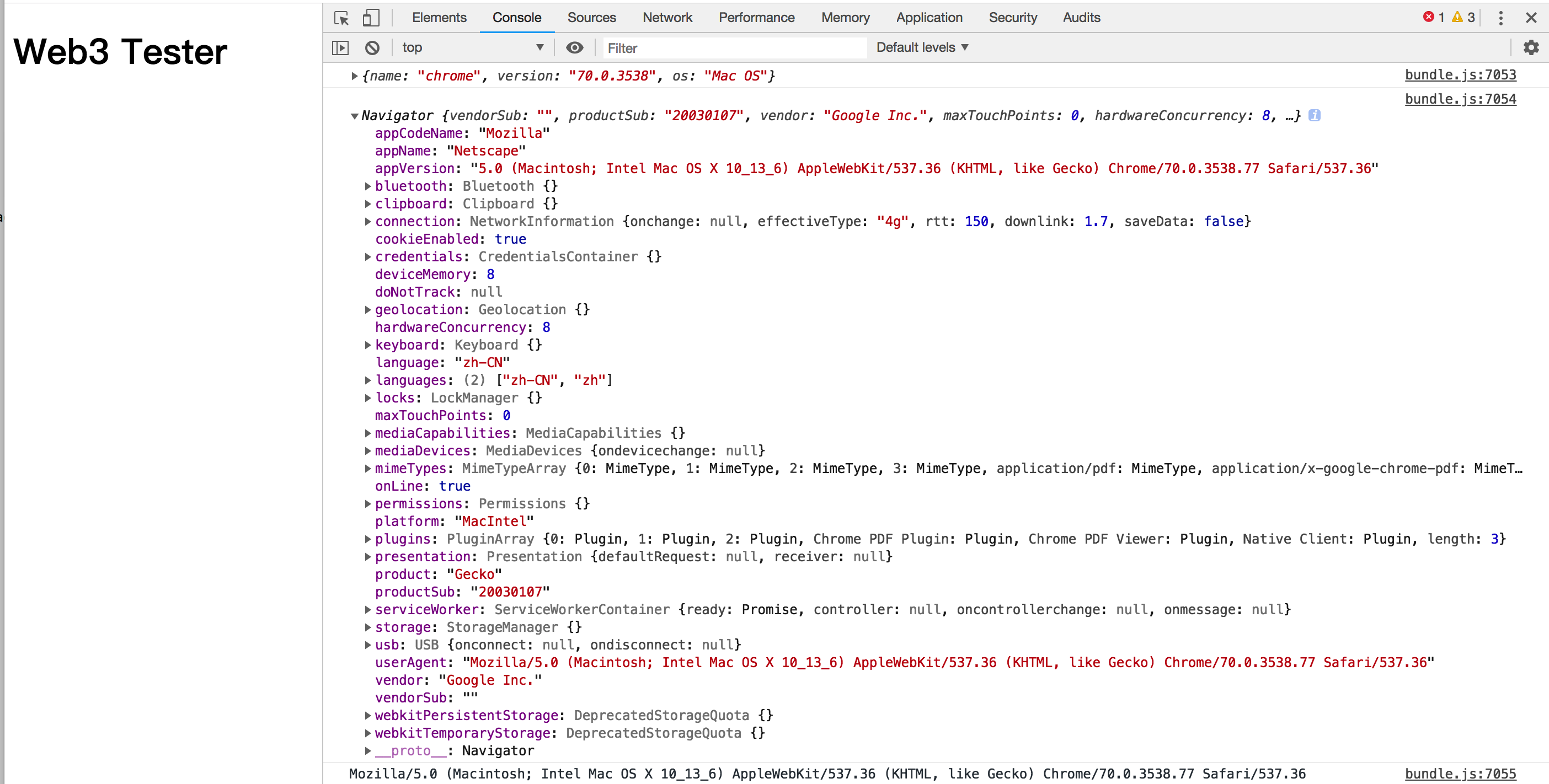
detect-browser/test/logic.js
使用例子来进行说明某类userAgent会检测出什么样的结果:
var test = require('tape'); var { parseUserAgent } = require('../'); function assertAgentString(t, agentString, expectedResult) { t.deepEqual(parseUserAgent(agentString), expectedResult); } test('detects Chrome', function(t) { assertAgentString(t, 'Mozilla/5.0 (X11; Linux x86_64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/50.0.2661.102 Safari/537.36', { name: 'chrome', version: '50.0.2661', os: 'Linux' } ); assertAgentString(t, 'Mozilla/5.0 (Windows NT 6.1) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/41.0.2228.0 Safari/537.36', { name: 'chrome', version: '41.0.2228', os: 'Windows 7' } ); t.end(); }); test('handles no browser', function(t) { assertAgentString(t, null, null ); t.end(); });