Description
对Samuel星球的探险已经取得了非常巨大的成就,于是科学家们将目光投向了Samuel星球所在的星系——一个巨大的由千百万星球构成的Samuel星系。 星际空间站的Samuel II巨型计算机经过长期探测,已经锁定了Samuel星系中许多星球的空间坐标,并对这些星球从1开始编号1、2、3……。 一些先遣飞船已经出发,在星球之间开辟探险航线。 探险航线是双向的,例如从1号星球到3号星球开辟探险航线,那么从3号星球到1号星球也可以使用这条航线。 例如下图所示:
在5个星球之间,有5条探险航线。 A、B两星球之间,如果某条航线不存在,就无法从A星球抵达B星球,我们则称这条航线为关键航线。 显然上图中,1号与5号星球之间的关键航线有1条:即为4-5航线。 然而,在宇宙中一些未知的磁暴和行星的冲撞,使得已有的某些航线被破坏,随着越来越多的航线被破坏,探险飞船又不能及时回复这些航线,可见两个星球之间的关键航线会越来越多。 假设在上图中,航线4-2(从4号星球到2号星球)被破坏。此时,1号与5号星球之间的关键航线就有3条:1-3,3-4,4-5。 小联的任务是,不断关注航线被破坏的情况,并随时给出两个星球之间的关键航线数目。现在请你帮助完成。

Input
第一行有两个整数N,M。表示有N个星球(1< N < 30000),初始时已经有M条航线(1 < M < 100000)。随后有M行,每行有两个不相同的整数A、B表示在星球A与B之间存在一条航线。接下来每行有三个整数C、A、B。C为1表示询问当前星球A和星球B之间有多少条关键航线;C为0表示在星球A和星球B之间的航线被破坏,当后面再遇到C为1的情况时,表示询问航线被破坏后,关键路径的情况,且航线破坏后不可恢复; C为-1表示输入文件结束,这时该行没有A,B的值。被破坏的航线数目与询问的次数总和不超过40000。
Output
对每个C为1的询问,输出一行一个整数表示关键航线数目。 注意:我们保证无论航线如何被破坏,任意时刻任意两个星球都能够相互到达。在整个数据中,任意两个星球之间最多只可能存在一条直接的航线。
Sample Input
5 5
1 2
1 3
3 4
4 5
4 2
1 1 5
0 4 2
1 5 1
-1
1 2
1 3
3 4
4 5
4 2
1 1 5
0 4 2
1 5 1
-1
Sample Output
1
3
只有撤销操作没有加入操作,这很容易想到要离线逆序处理,我们发现题目中的一句话十分要命:“注意:我们保证无论航线如何被破坏,任意时刻任意两个星球都能够相互到达。在整个数据中,任意两个星球之间最多只可能存在一条直接的航线。”。这个图任意时刻都联通,它至少是一棵树,所以我们先对删完边的图求一个生成树,树上的边权都是1,因为每条边都是关键路径,然后按照询问逆序处理,如果是破坏边,就把(x, y)的区间的边赋为0,表示这些边不可能成为关键边,如果是询问直接查询(x, y)的树上的路径的1的个数。
注意在建成生成树之后要把非树边先计算上。
以上操作用树链剖分做就特别简单了。
并查集记得路径压缩...忘了写路径压缩T到怀疑人生。
代码不长。
扫描二维码关注公众号,回复:
3910918 查看本文章
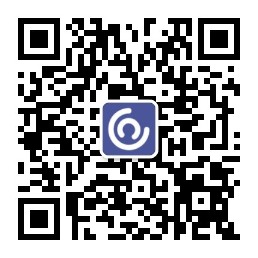
#include <iostream> #include <cstdio> #include <algorithm> #include <cstring> #include <vector> #include <queue> #include <map> using namespace std; #define reg register inline int read() { int res = 0;char ch=getchar();bool fu=0; while(!isdigit(ch))fu|=(ch=='-'),ch=getchar(); while(isdigit(ch))res=(res<<3)+(res<<1)+(ch^48), ch=getchar(); return fu?-res:res; } #define N 30005 #define M 100005 #define pii pair<int, int> #define mkp make_pair int n, m, q; struct que { int opt, x, y; }qu[N*2]; struct EDGE { int x, y; }EDG[M]; struct edge { int nxt, to; }ed[N<<1]; int head[N], cnt; inline void add(int x, int y) { ed[++cnt] = (edge){head[x], y}; head[x] = cnt; } int ufs[N]; int getF(int x) {return x==ufs[x]?x:ufs[x]=getF(ufs[x]);} map <pii, int> ex, use; int siz[N], father[N], son[N], dep[N], id[N], rnk[N], top[N], tot; void dfs1(int x, int fa) { siz[x] = 1; dep[x] = dep[fa] + 1; father[x] = fa; for (reg int i = head[x] ; i ; i = ed[i].nxt) { int to = ed[i].to; if (to == fa) continue; dfs1(to, x); siz[x] += siz[to]; if (siz[to] > siz[son[x]]) son[x] = to; } } void dfs2(int x, int tep) { id[x] = ++tot, rnk[tot] = x, top[x] = tep; if (son[x]) dfs2(son[x], tep); for (reg int i = head[x] ; i ; i = ed[i].nxt) { int to = ed[i].to; if (to == son[x] or to == father[x]) continue; dfs2(to, to); } } int tr[N<<2], tag[N<<2]; #define ls o << 1 #define rs o << 1 | 1 void build(int l, int r, int o) { if (l == r) {tr[o]=1;return;} int mid=(l+r)>>1; build(l, mid, ls), build(mid+1,r, rs); tr[o]=tr[ls]+tr[rs]; } inline void spread(int o) { if (!tag[o]) return ; tag[ls] = tag[rs] = 1; tag[o] = 0; tr[ls] = tr[rs] = 0; } void change(int l, int r, int o, int ql, int qr) { if (l >= ql and r <= qr) { tr[o] = 0, tag[o] = 1; return ; } int mid=(l+r)>>1; spread(o); if (ql <= mid) change(l, mid, ls, ql, qr); if (qr > mid) change(mid + 1, r, rs, ql, qr); tr[o] = tr[ls] + tr[rs]; } int query(int l, int r, int o, int ql, int qr) { if (l >= ql and r <= qr) return tr[o]; spread(o); int mid=(l+r)>>1, res=0; if (ql<=mid) res+=query(l, mid, ls, ql, qr); if (qr>mid) res+=query(mid+1, r, rs, ql, qr); return res; } inline void changes(int x, int y) { while(top[x] != top[y]) { if (dep[top[x]] < dep[top[y]]) swap(x, y); change(1, n, 1, id[top[x]], id[x]); x = father[top[x]]; } if (id[x] > id[y]) swap(x, y); change(1, n, 1, id[x] + 1, id[y]); } inline int querys(int x, int y) { int res = 0; while(top[x]!=top[y]) { if (dep[top[x]] < dep[top[y]]) swap(x, y); res += query(1, n, 1, id[top[x]], id[x]); x = father[top[x]]; } if (id[x] > id[y]) swap(x, y); res += query(1, n, 1, id[x] + 1, id[y]); return res; } int ans[N*2], ttans; int main() { n = read(), m = read(); for (reg int i = 1 ; i <= m ; i ++) { EDG[i] = (EDGE){read(), read()}; if (EDG[i].x > EDG[i].y) swap(EDG[i].x, EDG[i].y); ex[mkp(EDG[i].x, EDG[i].y)] = 1; } while(1) { int opt = read(); if (opt == -1) break; qu[++q] = (que){opt, read(), read()}; if (qu[q].x > qu[q].y) swap(qu[q].x, qu[q].y); if (opt == 0) ex[mkp(qu[q].x, qu[q].y)] = 0; } for (reg int i = 1 ; i <= n ; i ++) ufs[i] = i; for (reg int i = 1 ; i <= m ; i ++) { int x = EDG[i].x, y = EDG[i].y; if (!ex[mkp(x, y)]) continue; int fx = getF(x), fy = getF(y); if (fx == fy) continue; ufs[fx] = fy; add(x, y), add(y, x); use[mkp(x, y)] = 1; } dfs1(1, 0), dfs2(1, 1); build(1, n, 1); for (reg int i = 1 ; i <= m ; i ++) { int x = EDG[i].x, y = EDG[i].y; if (!ex[mkp(x, y)]) continue; if (!use[mkp(x, y)]) changes(x, y); } for (reg int i = q ; i >= 1 ; i --) { int op = qu[i].opt, x = qu[i].x, y = qu[i].y; if (!op) changes(x, y); else ans[++ttans] = querys(x, y); } for (reg int i = ttans ; i >= 1 ; i --) printf("%d\n", ans[i]); return 0; }