1、对于输入矩阵、数组进行洗牌的两个函数:numpy.random.shuffle(x) 和 numpy.random.permutation(x)是有区别的:
区别1:.permutation(x)可以将洗牌后的副本输出,而.shuffle(x)输出是None。对于.shuffle(x)重新洗牌后的副本还是x来表示。见例子:
import numpy as np
a = np.array([[1,2,3,4], [5,6,7,8], [3,4,5,6], [1,4,7,8]])
print(a)
print('/*/*/*/*/*')
b = np.random.permutation(a)
print(b)
print('/*/*/*/*/*')
c = np.random.shuffle(a)
print(c)
print(a)
输出:
[[1 2 3 4]
[5 6 7 8]
[3 4 5 6]
[1 4 7 8]]
/*/*/*/*/*
[[1 2 3 4]
[1 4 7 8]
[5 6 7 8]
[3 4 5 6]]
/*/*/*/*/*
None
[[1 4 7 8]
[1 2 3 4]
[5 6 7 8]
[3 4 5 6]]
区别2:如果传入的是一个整数的时候,.permutation(x)可以返回一个洗牌后的arange,而.shuffle(x)中要求x不能是整数。
import numpy as np
a = 10
b = np.random.permutation(a)
print(b)
print('/*/*/*/*/*')
c = np.random.shuffle(a)
print(a)
输出:
[7 8 2 0 4 5 1 9 6 3]
File "F:/learning/test-package/train.py", line 7, in <module>
c = np.random.shuffle(a)
/*/*/*/*/*
File "mtrand.pyx", line 4796, in mtrand.RandomState.shuffle (numpy\random\mtrand\mtrand.c:38843)
TypeError: object of type 'int' has no len()
2、numpy.random.uniform(-x, x)———随机生成(-x到x范围内的一个数)
print(np.random.uniform(-1, 1))
输出:
第一次:-0.33214855896802553
第二次:-0.5749959388706738
3、numpy.random.seed(x)—————方法改变随机数生成器的种子,可以在调用其他随机模块函数之前调用此函数。x -- 改变随机数生成器的种子seed。本函数没有返回值。
注意:seed(()是不能直接访问的,需要导入 random 模块,然后通过 random 静态对象调用该方法。
import numpy as np
np.random.seed( 5 )
print("Random number with seed 5 : ", np.random.random())
# 生成同一个随机数
np.random.seed( 5 )
print("Random number with seed 5 : ", np.random.random())
# 生成同一个随机数
np.random.seed( 1 )
print("Random number with seed 1 : ", np.random.random())
输出:
Random number with seed 5 : 0.22199317108973948
Random number with seed 5 : 0.22199317108973948
Random number with seed 1 : 0.417022004702574
4、区分np.nonzero()与np.count_nonzero()的区别:
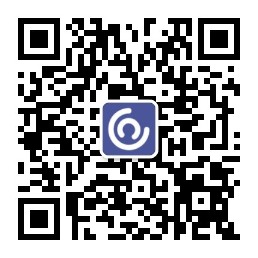
①、返回值不一样:np.nonzero()是返回非零元素对应的索引值(类型是一个;count_nonzero返回满足(条件)的数量的统计和,
②、应用范围不同:np.nonzero()可以对矩阵、数组或者元组(只存在数字)进行操作;np.count_nonzero()只能应用于矩阵中。
接下来看实例:
Example 1:矩阵
import numpy as np
array = np.array([[1, 0, 3], [1, 6, 7], [1, 9, 11]])
non_ones = np.nonzero(array)
count_zeros = np.count_nonzero(array != 0) #矩阵array中非零元素个数的统计
count_ones = np.count_nonzero(array != 1) #矩阵array中非壹元素个数的统计
print(array)
print(non_ones)
print(count_zeros)
print(count_ones)
输出:
[[ 1 0 3]
[ 1 6 7]
[ 1 9 11]]
(array([0, 0, 1, 1, 1, 2, 2, 2], dtype=int64), array([0, 2, 0, 1, 2, 0, 1, 2], dtype=int64))
8
6
Example 2:列表
import numpy as np
list = [1, 2, 0, 4, 0]
non_ones = np.nonzero(list)
print(list)
print(non_ones)
输出:
[1, 2, 0, 4, 0]
(array([0, 1, 3], dtype=int64),)
Example 3:元组(非全数字)
import numpy as np
tuple = ('apple', 0, 1, 4, 1, 0)
non_ones = np.nonzero(tuple )
print(tuple)
print(non_ones)
输出:
('apple', 0, 1, 4, 1, 0)
(array([0, 1, 2, 3, 4, 5], dtype=int64),)
Example 4:元组(全数字)
import numpy as np
tuple = (0, 1, 4, 1, 0)
non_ones = np.nonzero(tuple )
print(tuple)
print(non_ones)
输出:
(0, 1, 4, 1, 0)
(array([1, 2, 3], dtype=int64),)
4、随机生成矩阵:np.empty()与np.random.random()
注意:np.empty()可以指定随机生成数据的类型(numpy.
empty
(shape, dtype=float, order='C'))
np.random.random()
import numpy as np
b = np.empty([3, 4], dtype=int)
d = np.random.random([3, 4])
print(b)
print(d)
输出:
[[ -89792704 32762 -89814704 32762]
[1915740112 0 1952805488 1884251237]
[1852795252 0 0 0]]
[[0.23151918 0.02996494 0.41285224 0.68917454]
[0.67876318 0.05227411 0.02135239 0.02125393]
[0.84004973 0.62453174 0.88129274 0.5860845 ]]