题目描述(Medium)
Sort a linked list in O(n log n) time using constant space complexity.
题目链接
https://leetcode.com/problems/sort-list/description/
Example 1:
Input: 4->2->1->3
Output: 1->2->3->4
Example 2:
Input: -1->5->3->4->0
Output: -1->0->3->4->5
算法分析
归并排序,使用快慢指针找到中间节点,并将前后半段断开,再合并两个链表。
提交代码:
扫描二维码关注公众号,回复:
3741740 查看本文章
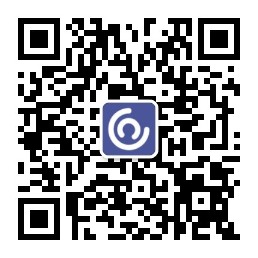
/**
* Definition for singly-linked list.
* struct ListNode {
* int val;
* ListNode *next;
* ListNode(int x) : val(x), next(NULL) {}
* };
*/
class Solution {
public:
ListNode* sortList(ListNode* head) {
if (head == NULL || head->next == NULL) return head;
ListNode* fast = head, *slow = head;
while (fast->next && fast->next->next)
{
fast = fast->next->next;
slow = slow->next;
}
fast = slow;
slow = slow->next;
fast->next = NULL;
ListNode* l1 = sortList(head);
ListNode* l2 = sortList(slow);
return mergeTwoLists(l1, l2);
}
ListNode* mergeTwoLists(ListNode* l1, ListNode* l2) {
ListNode* dummy = new ListNode(-1);
for (ListNode* p = dummy; l1 != NULL || l2 != NULL; p = p->next)
{
int val1 = l1 != NULL ? l1->val : INT_MAX;
int val2 = l2 != NULL ? l2->val : INT_MAX;
if (val1 <= val2)
{
p->next = l1;
l1 = l1->next;
}
else
{
p->next = l2;
l2 = l2->next;
}
}
return dummy->next;
}
};