再使用 Feign
和 Ribbon
之前,我们先来看看基础的 LoadBalancerClient
负载均衡客户端。
LoadBalancerClient
1、添加依赖:
<!--继承 1.5.4.RELEASE版本spring boot-->
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>1.5.4.RELEASE</version>
<relativePath/> <!-- lookup parent from repository -->
</parent>
<dependencies>
<!--服务提供者,亦是消费者-->
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-eureka</artifactId>
</dependency>
<!--mvc模块 -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
</dependencies>
<!--继承 Dalston.SR1版本spring cloud-->
<dependencyManagement>
<dependencies>
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-dependencies</artifactId>
<version>Dalston.SR1</version>
<type>pom</type>
<scope>import</scope>
</dependency>
</dependencies>
</dependencyManagement>
2、application.properties
配置一把,:
spring.application.name=eureka-consumer
server.port=8003
# 注册到服务注册中心
eureka.client.serviceUrl.defaultZone=http://localhost:8001/eureka/
3、写一个HelloController
来消费另一个微服务的接口:
@RestController
public class HelloController {
@Autowired
LoadBalancerClient loadBalancerClient;
@Autowired
RestTemplate restTemplate;
@GetMapping("/hello")
public String hello() {
//eureka-client为实例名称,指定要消费的服务在注册中心中的实例名称
ServiceInstance serviceInstance = loadBalancerClient.choose("eureka-client");
//手动拼接参数
String url = "http://" + serviceInstance.getHost() + ":" + serviceInstance.getPort() + "/hello";
System.out.println(url);
//restTemplate 消费服务
return restTemplate.getForObject(url, String.class);
}
}
4、启动:
@SpringBootApplication
@EnableDiscoveryClient
public class EurekaConsumerApplication {
@Bean
public RestTemplate restTemplate() {
return new RestTemplate(); //初始化RestTemplate,交给Spring容器管理
}
public static void main(String[] args) {
SpringApplication.run(EurekaConsumerApplication.class, args);
}
}
初始化RestTemplate,用来真正发起REST请求。@EnableDiscoveryClient注解用来将当前应用加入到服务治理体系中。
启动后,可以看到注册中心如下图,在注册中心中,EUREKA-CLIENT
是服务提供者,EUREKA-CONSUMER
是消费者。关于EUREKA-CLIENT
服务提供者和服务注册中心的创建,可以参考 服务注册与发现eureka
浏览器输入 http://localhost:8003/hello ,可看到以下结果:
再看看服务提供者EUREKA-CLIENT
,再浏览器中输入 http://localhost:8002/hello ,结果如下,和消费者的结果当然是一样的~
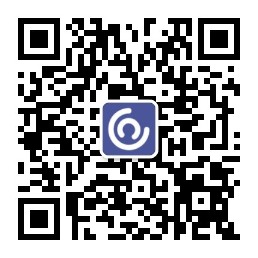
Spring Cloud Ribbon
Spring Cloud Ribbon是基于Netflix Ribbon实现的一套客户端负载均衡的工具。它是一个基于HTTP和TCP的客户端负载均衡器。它可以通过在客户端中配置 ribbonServerList 来设置服务端列表去轮询访问以达到均衡负载的作用。
下面,我们在上面eureka-consumer
项目的基础上来实现。
1、导入依赖,如下图,因为刚才的eureka-consumer
消费者实例中,已经引用了eureka
依赖,而eureka
依赖中存在ribbon
,所以,就不需要单独应用ribbon
模块了。:
如果项目中没有应用eureka
,则要引入如下依赖:
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-ribbon</artifactId>
</dependency>
2、添加一个接口:
@GetMapping("/hello2")
public String hello2() {
return restTemplate.getForObject("http://eureka-client/hello", String.class);
}
3、启动:
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.cloud.client.discovery.EnableDiscoveryClient;
import org.springframework.cloud.client.loadbalancer.LoadBalanced;
import org.springframework.context.annotation.Bean;
import org.springframework.web.client.RestTemplate;
@SpringBootApplication
@EnableDiscoveryClient
public class EurekaConsumerApplication {
@Bean
@LoadBalanced //实现客户端负载均衡
public RestTemplate restTemplate() {
return new RestTemplate();
}
public static void main(String[] args) {
SpringApplication.run(EurekaConsumerApplication.class, args);
}
}
启动eureka-consumer
,接下来可以将eureka-client
服务提供者多启动几份,访问 http://localhost:8003/hello2 来观察其负载均衡的效果。
Spring Cloud Feign
Spring Cloud Feign是一套基于Netflix Feign实现的声明式服务调用客户端。它使得编写Web服务客户端变得更加简单。我们只需要通过创建接口并用注解来配置它既可完成对Web服务接口的绑定。
仍然是在上面eureka-consumer
项目的基础上来实现。
1、添加依赖:
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-feign</artifactId>
</dependency>
2、定义消费方法:
import org.springframework.cloud.netflix.feign.FeignClient;
import org.springframework.web.bind.annotation.GetMapping;
@FeignClient("eureka-client")
public interface Hello2Controller {
@GetMapping("/hello")
String hello3(); //消费eureka-client实例下的/hello接口
}
使用 @FeignClient
注解来指定这个接口所要调用的服务名称,接口中定义的各个函数使用Spring MVC的注解就可以来绑定服务提供方的REST接口,比如上面就是绑定 eureka-client
服务的 /hello
。
3、消费,再添加一个接口方法:
@Autowired
Hello2Controller hello2Controller;
@GetMapping("/hello3")
public String hello3() {
return hello2Controller.hello3();
}
4、启动:
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.cloud.client.discovery.EnableDiscoveryClient;
import org.springframework.cloud.client.loadbalancer.LoadBalanced;
import org.springframework.cloud.netflix.feign.EnableFeignClients;
import org.springframework.context.annotation.Bean;
import org.springframework.web.client.RestTemplate;
@SpringBootApplication
@EnableDiscoveryClient
@EnableFeignClients
public class EurekaConsumerApplication {
@Bean
@LoadBalanced
public RestTemplate restTemplate() {
return new RestTemplate();
}
public static void main(String[] args) {
SpringApplication.run(EurekaConsumerApplication.class, args);
}
}
在原来的基础上,添加 @EnableFeignClients
注解,通过@EnableFeignClients
注解开启扫描Spring Cloud Feign客户端的功能。
到这就完了。由于Feign是基于Ribbon实现的,所以它自带了客户端负载均衡功能,也可以通过Ribbon的IRule进行策略扩展。
启动eureka-consumer
,同样,接下来可以将eureka-client
服务提供者多启动几份,访问 http://localhost:8003/hello3 来观察其负载均衡的效果。