传送门
题面:
The New Year holidays are over, but Resha doesn't want to throw away the New Year tree. He invited his best friends Kerim and Gural to help him to redecorate the New Year tree.
The New Year tree is an undirected tree with n vertices and root in the vertex 1.
You should process the queries of the two types:
- Change the colours of all vertices in the subtree of the vertex v to the colour c.
- Find the number of different colours in the subtree of the vertex v.
Input
The first line contains two integers n, m (1 ≤ n, m ≤ 4·105) — the number of vertices in the tree and the number of the queries.
The second line contains n integers ci (1 ≤ ci ≤ 60) — the colour of the i-th vertex.
Each of the next n - 1 lines contains two integers xj, yj (1 ≤ xj, yj ≤ n) — the vertices of the j-th edge. It is guaranteed that you are given correct undirected tree.
The last m lines contains the description of the queries. Each description starts with the integer tk (1 ≤ tk ≤ 2) — the type of the k-th query. For the queries of the first type then follows two integers vk, ck (1 ≤ vk ≤ n, 1 ≤ ck ≤ 60) — the number of the vertex whose subtree will be recoloured with the colour ck. For the queries of the second type then follows integer vk (1 ≤ vk ≤ n) — the number of the vertex for which subtree you should find the number of different colours.
Output
For each query of the second type print the integer a — the number of different colours in the subtree of the vertex given in the query.
Each of the numbers should be printed on a separate line in order of query appearing in the input.
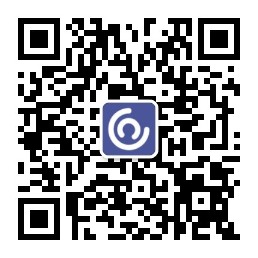
Examples
Input
7 10
1 1 1 1 1 1 1
1 2
1 3
1 4
3 5
3 6
3 7
1 3 2
2 1
1 4 3
2 1
1 2 5
2 1
1 6 4
2 1
2 2
2 3
Output
2
3
4
5
1
2
Input
23 30
1 2 2 6 5 3 2 1 1 1 2 4 5 3 4 4 3 3 3 3 3 4 6
1 2
1 3
1 4
2 5
2 6
3 7
3 8
4 9
4 10
4 11
6 12
6 13
7 14
7 15
7 16
8 17
8 18
10 19
10 20
10 21
11 22
11 23
2 1
2 5
2 6
2 7
2 8
2 9
2 10
2 11
2 4
1 12 1
1 13 1
1 14 1
1 15 1
1 16 1
1 17 1
1 18 1
1 19 1
1 20 1
1 21 1
1 22 1
1 23 1
2 1
2 5
2 6
2 7
2 8
2 9
2 10
2 11
2 4
Output
6
1
3
3
2
1
2
3
5
5
1
2
2
1
1
1
2
3
题意:
给你一颗n个节点的树,每个节点被染上了颜色,然后就是m次查询。 查询的方式有两种 1,将以z为根的子树的结点全部更新为颜色X 2,问以z为根的子树的结点的不同颜色数量。
题目分析:
这个问题类似poj2777,只不过poj2777中是维护的是一段线段上的染色问题,而这个问题是维护子树上的染色问题。
而因为题目中问的是以某个结点的子树上的不同颜色的问题,因此我们就可以用dfs序获得每一个结点的子树区间。之后我们就将这个树形的问题转化成了一个线性区间的问题,如此一来的做法就跟poj2777一样了。
因为最多出现的颜色种类为60,因此我们可以用一个long long的二进制数对每一种颜色进行维护,之后我们就可以用一颗线段树对dfs序进行维护了。
对于操作1,我们可以用线段树对结点z的子树进行区间更新。
对于操作2,我们可以用线段树对结点z的子树进行区间查询,之后只需要统计得到的答案中二进制1的个数即为答案。
代码:
#include <bits/stdc++.h>
#define maxn 400005
using namespace std;
typedef long long ll;
struct ST{
ll num,add;
}tr[maxn<<2];
struct edge{
int to,next,type;
}q[maxn<<1];
ll num[maxn];
int head[maxn],cnt;
int in[maxn],out[maxn],tot;
void add_edge(int from,int to){
q[cnt].next=head[from];
q[cnt].to=to;
head[from]=cnt++;
}
void dfs(int x,int fa){//获得dfs序
in[x]=++tot;
for(int i=head[x];i!=-1;i=q[i].next){
int to=q[i].to;
if(to==fa) continue;
dfs(to,x);
}
out[x]=tot;
}
void init(){
memset(head,-1,sizeof(head));
cnt=tot=0;
}
void push_up(int rt){//区间合并,每一次合并时将不同的颜色合并
tr[rt].num=tr[rt<<1].num|tr[rt<<1|1].num;
}
void push_down(int rt){//lazy操作
if(tr[rt].add){
tr[rt<<1].add=tr[rt].add;
tr[rt<<1|1].add=tr[rt].add;
tr[rt<<1].num=tr[rt].add;
tr[rt<<1|1].num=tr[rt].add;
tr[rt].add=0;
}
}
void update(int L,int R,int l,int r,int rt,ll val){//区间更新
if(L<=l&&R>=r){
tr[rt].num=(1ll<<(val-1));
tr[rt].add=(1ll<<(val-1));
return ;
}
push_down(rt);
int mid=(l+r)>>1;
if(L<=mid) update(L,R,l,mid,rt<<1,val);
if(R>mid) update(L,R,mid+1,r,rt<<1|1,val);
push_up(rt);
}
ll query(int L,int R,int l,int r,int rt){//区间查询
if(L<=l&&R>=r){
return tr[rt].num;
}
int mid=(l+r)>>1;
push_down(rt);
ll res=0;
if(L<=mid) res|=query(L,R,l,mid,rt<<1);
if(R>mid) res|=query(L,R,mid+1,r,rt<<1|1);
return res;
}
int main()
{
int n,Q;
//freopen("in.txt","r",stdin);
cin>>n>>Q;
init();
for(int i=1;i<=n;i++){
scanf("%d",&num[i]);
num[i];
}
for(int i=1;i<n;i++){
int from,to;
scanf("%d%d",&from,&to);
add_edge(from,to);
add_edge(to,from);
}
dfs(1,-1);
int N;
for(N=1;N<n;N<<=1);
for(int i=1;i<=n;i++){
int lll=in[i];
int rrr=in[i];
ll tmp;
tmp=num[i];
update(lll,rrr,1,N,1,tmp);
}
while(Q--){
int id,l;
scanf("%d%d",&id,&l);
if(id==1){
int val;
scanf("%d",&val);//对于l结点的子树区间[in[l],out[l]]进行区间更新
update(in[l],out[l],1,N,1,1ll*val);
}
else{
ll tmp=query(in[l],out[l],1,N,1);
tmp=__builtin_popcountll(tmp);//获取二进制位1的函数
printf("%d\n",tmp);
}
}
return 0;
}