Recently, TeaTree acquire new knoledge gcd (Greatest Common Divisor), now she want to test you.
As we know, TeaTree is a tree and her root is node 1, she have n nodes and n-1 edge, for each node i, it has it’s value v[i].
For every two nodes i and j (i is not equal to j), they will tell their Lowest Common Ancestors (LCA) a number : gcd(v[i],v[j]).
For each node, you have to calculate the max number that it heard. some definition:
In graph theory and computer science, the lowest common ancestor (LCA) of two nodes u and v in a tree is the lowest (deepest) node that has both u and v as descendants, where we define each node to be a descendant of itself.
Input
On the first line, there is a positive integer n, which describe the number of nodes.
Next line there are n-1 positive integers f[2] ,f[3], …, f[n], f[i] describe the father of node i on tree.
Next line there are n positive integers v[2] ,v[3], …, v[n], v[i] describe the value of node i.
n<=100000, f[i]<i, v[i]<=100000
Output
Your output should include n lines, for i-th line, output the max number that node i heard.
For the nodes who heard nothing, output -1.
Sample Input
4 1 1 3 4 1 6 9
Sample Output
2 -1 3 -1
思路:求lca的最大gcd,只需要考虑任意两个有倍数关系的节点,所以在一开始,将有相同值的节点记录在一起。
然后从最大的值开始枚举gcd值。
求lca可以rmq或者树链剖分来求。
代码:
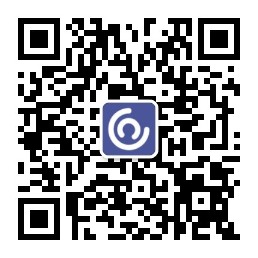
#include <iostream>
#include <stdio.h>
#include <string.h>
#include <algorithm>
#include <queue>
#include <map>
#include <vector>
#include <set>
#include <string>
#include <math.h>
const int inf=0x3f3f3f3f;
using namespace std;
const int maxn=1e5+9;
int f[maxn],id[maxn],top[maxn];
int son[maxn],size[maxn],depth[maxn];
int dfn[maxn],D;
int head[maxn],num;
int cnt;
int n;
vector<int> vec[maxn];
int h[maxn];
int ans[maxn];
struct Edge
{
int u,v,next,w,id;
}edge[maxn<<1];
void addEdge(int u,int v,int w)
{
edge[num].u=u;
edge[num].v=v;
edge[num].w=w;
edge[num].next=head[u];
head[u]=num++;
}
void init()
{
num=0;
cnt=0;
memset(head,-1,sizeof(head));
memset(son,-1,sizeof(son));
}
void dfs(int u,int pre,int dep)
{
f[u]=pre;
size[u]=1;
depth[u]=dep;
dfn[u]=++D;
for(int i=head[u];i!=-1;i=edge[i].next)
{
int v=edge[i].v;
if(v==pre) continue;
dfs(v,u,dep+1);
size[u]+=size[v];
if(son[u]==-1||size[son[u]]<size[v])
{
son[u]=v;
}
dfn[u]=++D;
}
}
void dfs2(int u,int tp)
{
top[u]=tp;
id[u]=++cnt;
if(son[u]==-1) return;
dfs2(son[u],tp);
for(int i=head[u];i!=-1;i=edge[i].next)
{
int v=edge[i].v;
if(v!=f[u]&&son[u]!=v)
{
dfs2(v,v);
}
}
}
int lca(int x,int y)
{
int fx=top[x],fy=top[y];
while(fx!=fy)
{
if(depth[fx]>=depth[fy])
{
x=f[fx];
}
else
{
y=f[fy];
}
fx=top[x],fy=top[y];
}
if(depth[x]>depth[y]) swap(x,y);
return x;
}
int mx;
inline void solve(int g)
{
vector<int> Node;
for(int i=g;i<=mx;i+=g)
{
for(auto &t:vec[i])
{
Node.push_back(t);
}
}
sort(Node.begin(),Node.end(),[&](int x,int y){return dfn[x]<dfn[y];});
int up=Node.size();
for(int i=1;i<up;i++)
{
int u=lca(Node[i],Node[i-1]);
ans[u]=max(ans[u],g);
}
}
int main()
{
#ifndef ONLINE_JUDGE
freopen("in.txt","r",stdin);
freopen("out.txt","w",stdout);
#endif
scanf("%d",&n);
init();
for(int i=2;i<=n;i++)
{
int x;
scanf("%d",&x);
addEdge(i,x,0);
addEdge(x,i,0);
}
for(int i=1;i<=n;i++)
{
scanf("%d",&h[i]);
vec[h[i]].push_back(i);
}
dfs(1,0,1);
dfs2(1,1);
::mx=*max_element(h+1,h+1+n);
for(int i=mx;i;i--)
{
solve(i);
}
for(int i=1;i<=n;i++)
{
printf("%d\n",ans[i]?ans[i]:-1);
}
return 0;
}