Karma
https://baike.baidu.com/item/%E7%BE%AF%E7%A3%A8/7618552?fromtitle=Karma&fromid=878453
1,意译作“业”。业;行动;命运。一个人生命中的自然与必然事件,由前世的作为所决定。含有善恶、苦乐果报之意味,亦即与因果关系相结合的一种持续作用力。,2,在 佛教中,既是“作持”性质的戒条(属于各部“广律”的犍度篇部分),更是僧团中的议事规则。举凡授戒、说戒、忏悔,乃至各种僧团公共事条的处理所应遵行的一定程序,统称为“羯磨”,故此词意译为“办事”。
https://astrisk.github.io/javascript/2017/04/20/js-karma/
Karma 是Google 开源的一个基于Node.js 的 JavaScript 测试执行过程管理工具(Test Runner)。 Karma 可以在不同的桌面或移动设备浏览器上,或在持续集成的服务器上测试 JavaScript 代码。Karma 支持 Chrome、ChromeCanary、 Safari、Firefox、 IE、Opera、 PhantomJS,知道如何捕获浏览器正使用的默认路径,这些路径可能在启动器配置文件被忽视(overridden)。Karma 就是一个可以和多重测试框架协作的测试执行过程管理工具,它有针对Jasmine、 Mocha 和AngularJS 的适配器,它也可以与 Jenkins 或 Travis 整合,用于执行持续集成测试。
安装:
http://blog.fens.me/nodejs-karma-jasmine/
npm install -g karma
// configkarma init
// runkarma start
详细见:
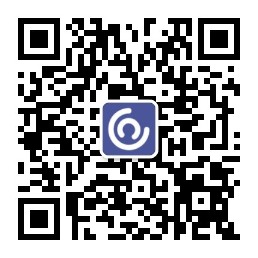
http://www.cnblogs.com/wushangjue/p/4539189.html
配置:
karma.config.js
module.exports = function (config) { config.set({ basePath: '', frameworks: ['jasmine'], files: ['*.js'], exclude: ['karma.conf.js'], reporters: ['progress'], port: 9876, colors: true, logLevel: config.LOG_INFO, autoWatch: true, browsers: ['Chrome'], captureTimeout: 60000, singleRun: false }); };
覆盖率报告:
http://blog.fens.me/nodejs-karma-jasmine/
npm install karma-coverage
reporters: ['progress','coverage'], preprocessors : {'src.js': 'coverage'}, coverageReporter: { type : 'html', dir : 'coverage/' }
Jasmine 断言API
https://jasmine.github.io/2.0/custom_matcher.html
Expectations
Expectations are built with the function
expect
which takes a value, called the actual. It is chained with a Matcher function, which takes the expected value.describe("The 'toBe' matcher compares with ===", function() {
Matchers
Each matcher implements a boolean comparison between the actual value and the expected value. It is responsible for reporting to Jasmine if the expectation is true or false. Jasmine will then pass or fail the spec.
it("and has a positive case", function() { expect(true).toBe(true); });
Any matcher can evaluate to a negative assertion by chaining the call to
expect
with anot
before calling the matcher.it("and can have a negative case", function() { expect(false).not.toBe(true); }); });
Included Matchers
Jasmine has a rich set of matchers included. Each is used here - all expectations and specs pass. There is also the ability to write custom matchers for when a project's domain calls for specific assertions that are not included below.
将ES6转换为 UMD
UMD:
http://web.jobbole.com/82238/
既然CommonJs和AMD风格一样流行,似乎缺少一个统一的规范。所以人们产生了这样的需求,希望有支持两种风格的“通用”模式,于是通用模块规范(UMD)诞生了。
不得不承认,这个模式略难看,但是它兼容了AMD和CommonJS,同时还支持老式的“全局”变量规范:
(function (root, factory) { if (typeof define === 'function' && define.amd) { // AMD define(['jquery'], factory); } else if (typeof exports === 'object') { // Node, CommonJS之类的 module.exports = factory(require('jquery')); } else { // 浏览器全局变量(root 即 window) root.returnExports = factory(root.jQuery); } }(this, function ($) { // 方法 function myFunc(){}; // 暴露公共方法 return myFunc; }));
http://www.cnblogs.com/snandy/archive/2012/03/19/2406596.html
AMD以浏览器为第一(browser-first)的原则发展,选择异步加载模块。它的模块支持对象(objects)、函数(functions)、构造器(constructors)、字符串(strings)、JSON等各种类型的模块。因此在浏览器中它非常灵活。
CommonJS module以服务器端为第一(server-first)的原则发展,选择同步加载模块。它的模块是无需包装的(unwrapped modules)且贴近于ES.next/Harmony的模块格式。但它仅支持对象类型(objects)模块。
这迫使一些人又想出另一个更通用格式 UMD(Universal Module Definition)。希望提供一个前后端跨平台的解决方案。
UMD的实现很简单,先判断是否支持Node.js模块格式(exports是否存在),存在则使用Node.js模块格式。
再判断是否支持AMD(define是否存在),存在则使用AMD方式加载模块。前两个都不存在,则将模块公开到全局(window或global)。
https://github.com/umdjs/umd
This repository formalizes the design and implementation of the Universal Module Definition (UMD) API for JavaScript modules. These are modules which are capable of working everywhere, be it in the client, on the server or elsewhere.
The UMD pattern typically attempts to offer compatibility with the most popular script loaders of the day (e.g RequireJS amongst others). In many cases it uses AMD as a base, with special-casing added to handle CommonJS compatibility.
将ES6模块转换为UMD代码
https://babel.bootcss.com/docs/plugins/transform-es2015-modules-umd/
输入
export default 42;
输出
(function (global, factory) { if (typeof define === "function" && define.amd) { define(["exports"], factory); } else if (typeof exports !== "undefined") { factory(exports); } else { var mod = { exports: {} }; factory(mod.exports); global.actual = mod.exports; } })(this, function (exports) { "use strict"; Object.defineProperty(exports, "__esModule", { value: true }); exports.default = 42; });
安装
npm install --save-dev babel-plugin-transform-es2015-modules-umd
用法
通过 .babelrc(推荐)
.babelrc
{ "plugins": ["transform-es2015-modules-umd"] }
当模块在浏览器中运行时,你可以覆盖特定的库的名称。例如
es-promise
库将自己暴露为global.Promise
而不是global.es6Promise
。这可以通过如下代码实现:{ "plugins": [ ["transform-es2015-modules-umd", { "globals": { "es6-promise": "Promise" } }] ] }
自己动手
https://github.com/fanqingsong/code-snippet/tree/master/javascript/karma_demo
karma.config.js
// Karma configuration // Generated on Thu Aug 16 2018 01:07:59 GMT+0800 (中国标准时间) module.exports = function(config) { config.set({ // base path that will be used to resolve all patterns (eg. files, exclude) basePath: '', // frameworks to use // available frameworks: https://npmjs.org/browse/keyword/karma-adapter frameworks: ['jasmine', 'browserify'], /** * 需要加载到浏览器的文件列表 */ // list of files / patterns to load in the browser files: [ 'test/*[s|S]pec.js', ], // list of files / patterns to exclude exclude: [ 'karma.conf.js' ], // preprocess matching files before serving them to the browser // available preprocessors: https://npmjs.org/browse/keyword/karma-preprocessor preprocessors: { 'test/*[s|S]pec.js' : [ 'browserify' ] }, //karma-browserify 配置,配置为使用 babelify browserify: { debug: true, transform: [['babelify', {presets: ['es2015'], plugins: ['transform-class-properties']}]] }, // test results reporter to use // possible values: 'dots', 'progress' // available reporters: https://npmjs.org/browse/keyword/karma-reporter reporters: ['progress','kjhtml'], // web server port port: 9876, // enable / disable colors in the output (reporters and logs) colors: true, // level of logging // possible values: config.LOG_DISABLE || config.LOG_ERROR || config.LOG_WARN || config.LOG_INFO || config.LOG_DEBUG logLevel: config.LOG_INFO, // enable / disable watching file and executing tests whenever any file changes autoWatch: true, // start these browsers // available browser launchers: https://npmjs.org/browse/keyword/karma-launcher browsers: ['Chrome'], // Continuous Integration mode // if true, Karma captures browsers, runs the tests and exits singleRun: false, // Concurrency level // how many browser should be started simultaneous concurrency: Infinity, }) }
Player.js
class Player { constructor() { } play(song) { this.currentlyPlayingSong = song; this.isPlaying = true; } pause() { this.isPlaying = false; } resume() { if (this.isPlaying) { throw new Error("song is already playing"); } this.isPlaying = true; } makeFavorite() { this.currentlyPlayingSong.persistFavoriteStatus(true); } }; export default Player;
Song.js
class Song { constructor() { } persistFavoriteStatus(value) { // something complicated throw new Error("not yet implemented"); } } export default Song;
testPlayerSpec.js
import Player from "../js/Player"; import Song from '../js/Song'; console.log("====================================") describe("Player", function() { var player; var song; beforeEach(function() { player = new Player(); song = new Song(); jasmine.addMatchers({ toBePlaying: function () { return { compare: function (actual, expected) { var player = actual; return { pass: player.currentlyPlayingSong === expected && player.isPlaying } } }; } }); }); console.log("====================================!!! out") it("should be able to play a Song", function() { console.log("====================================!!!") player.play(song); expect(player.currentlyPlayingSong).toEqual(song); //demonstrates use of custom matcher expect(player).toBePlaying(song); }); describe("when song has been paused", function() { beforeEach(function() { player.play(song); player.pause(); }); it("should indicate that the song is currently paused", function() { expect(player.isPlaying).toBeFalsy(); // demonstrates use of 'not' with a custom matcher expect(player).not.toBePlaying(song); }); it("should be possible to resume", function() { player.resume(); expect(player.isPlaying).toBeTruthy(); expect(player.currentlyPlayingSong).toEqual(song); }); }); // demonstrates use of spies to intercept and test method calls it("tells the current song if the user has made it a favorite", function() { spyOn(song, 'persistFavoriteStatus'); player.play(song); player.makeFavorite(); expect(song.persistFavoriteStatus).toHaveBeenCalledWith(true); }); // //demonstrates use of expected exceptions // describe("#resume", function() { // it("should throw an exception if song is already playing", function() { // player.play(song); // expect(function() { // player.resume(); // }).toThrowError("song is already playing"); // }); // }); });
参考:
http://blog.leanote.com/post/[email protected]/%E5%89%8D%E7%AB%AF-es6
https://wenjs.me/p/es6-testing-workflow-with-karma