You are given a graph with n nodes and m directed edges. One lowercase letter is assigned to each node. We define a path's value as the number of the most frequently occurring letter. For example, if letters on a path are "abaca", then the value of that path is 3. Your task is find a path whose value is the largest.
Input
The first line contains two positive integers n, m (1 ≤ n, m ≤ 300 000), denoting that the graph has n nodes and m directed edges.
The second line contains a string s with only lowercase English letters. The i-th character is the letter assigned to the i-th node.
Then m lines follow. Each line contains two integers x, y (1 ≤ x, y ≤ n), describing a directed edge from x to y. Note that x can be equal to y and there can be multiple edges between x and y. Also the graph can be not connected.
Output
Output a single line with a single integer denoting the largest value. If the value can be arbitrarily large, output -1 instead.
Examples
Input
5 4 abaca 1 2 1 3 3 4 4 5
Output
3
Input
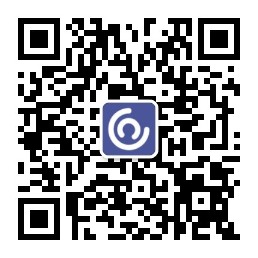
6 6 xzyabc 1 2 3 1 2 3 5 4 4 3 6 4
Output
-1
Input
10 14 xzyzyzyzqx 1 2 2 4 3 5 4 5 2 6 6 8 6 5 2 10 3 9 10 9 4 6 1 10 2 8 3 7
Output
4
Note
In the first sample, the path with largest value is 1 → 3 → 4 → 5. The value is 3because the letter 'a' appears 3 times.
题意:求含有相同字母最多的路径上这种字母的数量。
思路:如果成环,那么肯定不能计算。
需要计算路径上最多的字母个数,可以考虑分别计算每个字母的最大数量。
想要在图上dp,需要按树上dp的思路,由子节点推父亲节点?那么可以看成是有出度为0点一步步往前推。
所以拓扑排序以后,dp就解决了。
代码:
#include <iostream>
#include <stdio.h>
#include <string.h>
#include <algorithm>
#include <queue>
#include <map>
#include <vector>
#include <set>
#include <string>
#include <math.h>
#include <stack>
typedef long long ll;
#define INF 0x3f3f3f3f
const int maxn=3e5+10;
const int MAXN=1e3+10;
using namespace std;
int n,m;
char s[maxn];
int deg[maxn];
vector<int> vec[maxn];
vector<int> mp;
int dp[maxn];
bool vis[maxn];
bool topSort()
{
stack<int> sta;
for(int i=1;i<=n;i++)
{
if(deg[i]==0)
{
sta.push(i);
}
}
while(sta.empty()==0)
{
int tmp=sta.top();
mp.push_back(tmp);
sta.pop();
for(int j=0;j<vec[tmp].size();j++)
{
if(--deg[vec[tmp][j]]==0)
{
sta.push(vec[tmp][j]);
}
}
}
return mp.size()==n;
}
int dfs(int u)
{
memset(dp,0,sizeof(dp));
char ch=u+'a';
int ans=0;
for(int i=mp.size()-1;i>=0;i--)
{
int tmp=mp[i];
for(int j=0;j<vec[tmp].size();j++)
{
dp[tmp]=max(dp[tmp],dp[vec[tmp][j]]);
}
if(s[tmp-1]==ch)
{
dp[tmp]++;
}
ans=max(ans,dp[tmp]);
}
return ans;
}
int main(int argc, char const *argv[])
{
#ifndef ONLINE_JUDGE
freopen("in.txt","r",stdin);
freopen("out.txt","w",stdout);
#endif
cin>>n>>m;
memset(deg,0,sizeof(deg));
scanf("%s",s);
for(int i=1;i<=m;i++)
{
int x,y;
scanf("%d%d",&x,&y);
vec[x].push_back(y);
deg[y]++;
}
if(topSort()==0)
{
printf("-1\n");
}
else
{
int ans=0;
for(int i=0;i<26;i++)
{
ans=max(ans,dfs(i));
}
printf("%d\n",ans);
}
return 0;
}