深搜dfs
找多个解或步数已知
看一个例题:判断有几个最大水娃
10 12
W........WW.
.WWW.....WWW
....WW...WW.
.........WW.
.........W..
..W......W..
.W.W.....WW.
W.W.W.....W.
.W.W......W.
..W.......W.
输出 3
附上已ac代码
#include <stdio.h>
#include <iostream>
#include <algorithm>
#include <string.h>
using namespace std;
char mp[101][101];
int n,m,sum=0;
void DFS(int x,int y)
{
if(x>=0&&x<n&&y>=0&&y<m&&mp[x][y]=='W')
{
mp[x][y]='.';
DFS(x-1,y);
DFS(x-1,y-1);
DFS(x-1,y+1);
DFS(x,y-1);
DFS(x,y+1);
DFS(x+1,y);
DFS(x+1,y-1);
DFS(x+1,y+1);
}
}
int main()
{
int i,j;
sum=0;
scanf("%d%d",&n,&m);
memset(mp,'0',sizeof(mp));
getchar();
for(i=0;i<n;i++)
for(j=0;j<m;j++)
scanf(" %c",&mp[i][j]);
for(i=0;i<n;i++)
for(j=0;j<m;j++)
{
if(mp[i][j]=='W')
{
DFS(i,j);
sum++;
}
}
printf("%d\n",sum);
return 0;
}
广搜bfs
单一的最短路 规模最小的路径搜索 搜到的就是最优解
看一个例题:求s到e的最短距离 #为墙不可走
输入:
4 4
. . . . (中间有空格)
. s . #
. # . .
. . . e
输出 4
附上代码 若有错误欢迎大佬指正
#include <algorithm>
#include <iostream>
#include <cstdio>
#include <cstring>
#include <cstdlib>
#include <cmath>
#include <queue>
int vis[350][350];
int dir[4][2]={-1,0,1,0,0,1,0,-1};
using namespace std;
struct node
{
int x;
int y;
int time;
}start,now;
int map1[350][350];
int m,n;
queue <node> q;
int bfs(int x,int y)
{
int i;
memset(vis,0,sizeof(vis));
start.x=x;
start.y=y;
start.time=0;
vis[x][y]=1;
q.push(start);
while(!q.empty())
{
now=q.front();
q.pop();
if(map1[now.x][now.y]=='e')
return now.time;
for(i=0;i<4;i++)
{
start.x=now.x+dir[i][0];
start.y=now.y+dir[i][1];
start.time=now.time+1;
if(start.x>=0&&start.y>=0&&start.x<n&&start.y<m&&vis[start.x][start.y]==0&&map1[start.x][start.y]!='#')
{
vis[start.x][start.y]=1;
q.push(start);
}
}
}
return -1;
}
int main()
{
int i,j,c=0,d=0;
scanf("%d%d",&n,&m);
for(i=0;i<n;i++)
{
getchar();
for(j=0;j<m;j++)
{
scanf(" %c",&map1[i][j]);
if(map1[i][j]=='s')
{
c=i;
d=j;
}
}
}
int ans=bfs(c,d);
printf("%d\n",ans);
return 0;
}
附一个模板题
扫描二维码关注公众号,回复:
2605110 查看本文章
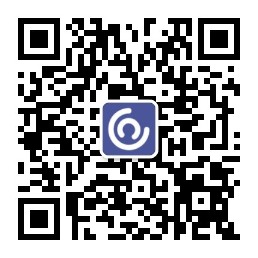
蓝色空间号和万有引力号进入了四维水洼,发现了四维物体--魔戒。
这里我们把飞船和魔戒都抽象为四维空间中的一个点,分别标为 "S" 和 "E"。空间中可能存在障碍物,标为 "#",其他为可以通过的位置。
现在他们想要尽快到达魔戒进行探索,你能帮他们算出最小时间是最少吗?我们认为飞船每秒只能沿某个坐标轴方向移动一个单位,且不能越出四维空间。
Input
输入数据有多组(数据组数不超过 30),到 EOF 结束。
每组输入 4 个数 x, y, z, w 代表四维空间的尺寸(1 <= x, y, z, w <= 30)。
接下来的空间地图输入按照 x, y, z, w 轴的顺序依次给出,你只要按照下面的坐标关系循环读入即可。
for 0, x-1
for 0, y-1
for 0, z-1
for 0, w-1
保证 "S" 和 "E" 唯一。
Output
对于每组数据,输出一行,到达魔戒所需的最短时间。
如果无法到达,输出 "WTF"(不包括引号)。
Sample Input
2 2 2 2 .. .S .. #. #. .E .# .. 2 2 2 2 .. .S #. ## E. .# #. ..
Sample Output
1 3
#include <algorithm>
#include <iostream>
#include <cstdio>
#include <cstring>
#include <cstdlib>
#include <cmath>
#include <queue>
using namespace std;
int vis[33][33][33][33];
int dir[8][4]= {{0,0,0,1},{0,0,0,-1},{0,0,1,0},{0,0,-1,0},{0,1,0,0},{0,-1,0,0},{1,0,0,0},{-1,0,0,0}};
struct node
{
int x;
int y;
int z;
int w;
int time;
} start,now;
char map1[33][33][33][33];
int n,m,nn,mm;
queue <node> q;
int bfs(int x,int y,int z,int w)
{
int i;
memset(vis,0,sizeof(vis));
while(!q.empty())
q.pop();
start.x=x;
start.y=y;
start.z=z;
start.w=w;
start.time=0;
vis[x][y][z][w]=1;
q.push(start);
while(!q.empty())
{
now=q.front();
q.pop();
if(map1[now.x][now.y][now.z][now.w]=='E')
return now.time;
for(i=0; i<8; i++)
{
start.x=now.x+dir[i][0];
start.y=now.y+dir[i][1];
start.z=now.z+dir[i][2];
start.w=now.w+dir[i][3];
start.time=now.time+1;
if(start.x>=0&&start.y>=0&&start.z>=0&&start.w>=0&&start.x<n&&start.y<m&&start.z<nn&&start.w<mm&&vis[start.x][start.y][start.z][start.w]==0&&map1[start.x][start.y][start.z][start.w]!='#')
{
vis[start.x][start.y][start.z][start.w]=1;
q.push(start);
}
}
}
return -1;
}
int main()
{
int i,j,k,l,c=0,d=0,e=0,f=0;
while(~scanf("%d%d%d%d",&n,&m,&nn,&mm))
{
memset(vis,0,sizeof(vis));
memset(map1,0,sizeof(map1));
c=0,d=0,e=0,f=0;
for(i=0; i<n; i++)
{
for(j=0; j<m; j++)
{
for(k=0; k<nn; k++)
{
getchar();
for(l=0; l<mm; l++)
{
scanf("%c",&map1[i][j][k][l]);
if(map1[i][j][k][l]=='S')
{
c=i;
d=j;
e=k;
f=l;
}
}
}
}
}
int ans=bfs(c,d,e,f);
if(ans==-1)
printf("WTF\n");
else
printf("%d\n",ans);
}
return 0;
}