Description
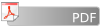
A robot has to patrol around a rectangular area which is in a form of mxn grid (m rows and n columns). The rows are labeled from 1 to m. The columns are labeled from 1 to n. A cell (i, j) denotes the cell in row i and column j in the grid. At each step, the robot can only move from one cell to an adjacent cell, i.e. from (x, y) to (x + 1, y), (x, y + 1), (x - 1, y) or (x, y - 1). Some of the cells in the grid contain obstacles. In order to move to a cell containing obstacle, the robot has to switch to turbo mode. Therefore, the robot cannot move continuously to more than k cells containing obstacles.
Your task is to write a program to find the shortest path (with the minimum number of cells) from cell (1, 1) to cell (m, n). It is assumed that both these cells do not contain obstacles.
Input
The input consists of several data sets. The first line of the input file contains the number of data sets which is a positive integer and is not bigger than 20. The following lines describe the data sets.
For each data set, the first line contains two positive integer numbers m and n separated by space (1m, n
20). The second line contains an integer number k(0
k
20). The ith line of the next m lines contains n integer aij separated by space (i = 1, 2,..., m;j = 1, 2,..., n). The value of aij is 1 if there is an obstacle on the cell (i, j), and is 0 otherwise.
Output
For each data set, if there exists a way for the robot to reach the cell (m, n), write in one line the integer number s, which is the number of moves the robot has to make; -1 otherwise.
Sample Input
3 2 5 0 0 1 0 0 0 0 0 0 1 0 4 6 1 0 1 1 0 0 0 0 0 1 0 1 1 0 1 1 1 1 0 0 1 1 1 0 0 2 2 0 0 1 1 0
Sample Output
7 10 -1
题意:给一个m行n列的矩阵每个小格里面有1和0两种状态,0代表路,1代表障碍,有一个机器人想从(1,1)-->(m,n),最少需要多少步,机器人也可以在障碍里面行走,但是最多只能连续走k个有障碍的格子。
算是迷宫问题,很容易想到要用bfs来做,对于每个没有障碍的小格子不可以重复走,但是对于每一个有障碍的小格子走到当前点的时候已经走过了1个有障碍的小格子和2个、3个....k个都是不一样的,所以vis数组不只要记录坐标,还要加上在当前坐标经过了多少个有障碍的格子。
#include<bits/stdc++.h>
using namespace std;
struct node{
int x, y, w, d;
node(int x, int y, int w, int d):x(x),y(y),w(w),d(d){}
};
int tx[5] = {1, -1, 0, 0};
int ty[5] = {0, 0, 1, -1};
int a[25][25], n, m, k, vis[25][25][25];
int bfs(){
memset(vis, 0, sizeof(vis));
queue<node> q;
q.push(node(1, 1, 0, 0));
vis[1][1][0] = 1;
while(!q.empty()){
node t = q.front();
q.pop();
if(t.x == n && t.y == m)
return t.w;
for(int i = 0; i < 4; i++){
int xx = t.x + tx[i];
int yy = t.y + ty[i];
if(xx > 0 && yy > 0 && xx <= n && yy <= m){
int temp;
if(a[xx][yy])
temp = t.d + 1;
else
temp = 0;
if(temp <= k && !vis[xx][yy][temp]){
q.push(node(xx, yy, t.w + 1, temp));
vis[xx][yy][temp] = 1;
}
}
}
}
return -1;
}
int main()
{
int T;
scanf("%d", &T);
while(T--){
scanf("%d%d%d", &n, &m, &k);
for(int i = 1; i <= n; i++)
for(int j = 1; j <= m; j++)
scanf("%d", &a[i][j]);
int t = bfs();
printf("%d\n", t);
}
return 0;
}