1059 Prime Factors (25)(25 分)
Given any positive integer N, you are supposed to find all of its prime factors, and write them in the format N = p~1~\^k~1~ * p~2~\^k~2~ *…*p~m~\^k~m~.
Input Specification:
Each input file contains one test case which gives a positive integer N in the range of long int.
Output Specification:
Factor N in the format N = p~1~\^k~1~ * p~2~\^k~2~ *…*p~m~\^k~m~, where p~i~'s are prime factors of N in increasing order, and the exponent k~i~ is the number of p~i~ -- hence when there is only one p~i~, k~i~ is 1 and must NOT be printed out.
Sample Input:
97532468
Sample Output:
97532468=2^2*11*17*101*1291
素数的应用,用的是厄拉多塞素数筛选法, 效率比较高。可见 https://blog.csdn.net/qq_34649947/article/details/79649247
#include <bits/stdc++.h>
using namespace std;
const int maxn = 100000 + 10;
int is_prime[maxn + 10];
int pNum = 0;
vector<int> prime;
void get_prime() {
for (int i = 2; i <= maxn; i++) {
is_prime[i] = 1;
}
for (int i = 2; i*i <= maxn; i++) {
if (is_prime[i]) {
for (int j = i*i; j <= maxn; j += i) {
is_prime[j] = 0;
}
}
}
for (int i = 2; i <= maxn; i++) {
if (is_prime[i]) {
pNum++;//在最大范围内所有的质数的数量
prime.push_back(i);//存储所有的质因子
}
}
}
//题目要求有底数和指数,所有用一个结构体来存
struct factor {
int x;//质因子
int cnt;//个数
}fac[100];
int n;
int main() {
get_prime();
int n, num = 0;//num为不同质因子的个数
cin >> n;
if (n == 1) cout << "1=1" << endl;
else {
cout << n << "=";
int sqr = (int)(sqrt(1.0*n));
//枚举
for (int i = 0; i < pNum && prime[i] <= sqr; i++) {
if (n % prime[i] == 0) {
fac[num].x = prime[i];
fac[num].cnt = 0;
while (n % prime[i] == 0) {
fac[num].cnt++;
n /= prime[i];
}
num++;
}
if (n == 1) break;//退出循环
}
//注意点,最后一个样例
if (n != 1) {//如果无法被根号n以内的质因子除尽,那就是质因子本身;
fac[num].x = n;
fac[num].cnt = 1;//
num++;
}
for (int i = 0; i < num; i++) {
if (i > 0) cout << "*";
cout << fac[i].x;
if (fac[i].cnt > 1) {
cout << "^" << fac[i].cnt;
}
}
}
return 0;
}
扫描二维码关注公众号,回复:
2432067 查看本文章
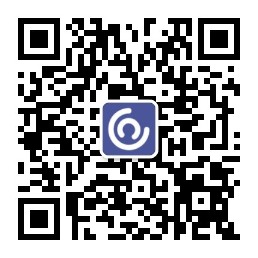