Treasure Hunt
Time Limit: 1000MS | Memory Limit: 10000K | |
Total Submissions: 7857 | Accepted: 3247 |
Description
Archeologists from the Antiquities and Curios Museum (ACM) have flown to Egypt to examine the great pyramid of Key-Ops. Using state-of-the-art technology they are able to determine that the lower floor of the pyramid is constructed from a series of straightline walls, which intersect to form numerous enclosed chambers. Currently, no doors exist to allow access to any chamber. This state-of-the-art technology has also pinpointed the location of the treasure room. What these dedicated (and greedy) archeologists want to do is blast doors through the walls to get to the treasure room. However, to minimize the damage to the artwork in the intervening chambers (and stay under their government grant for dynamite) they want to blast through the minimum number of doors. For structural integrity purposes, doors should only be blasted at the midpoint of the wall of the room being entered. You are to write a program which determines this minimum number of doors.
An example is shown below:
An example is shown below:
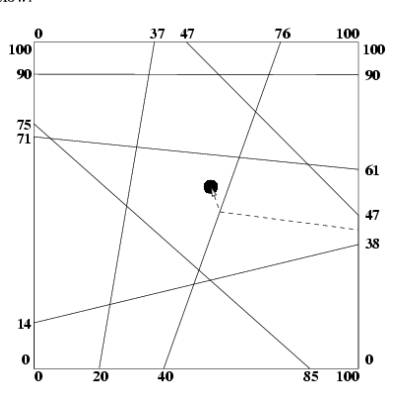
Input
The input will consist of one case. The first line will be an integer n (0 <= n <= 30) specifying number of interior walls, followed by n lines containing integer endpoints of each wall x1 y1 x2 y2 . The 4 enclosing walls of the pyramid have fixed endpoints at (0,0); (0,100); (100,100) and (100,0) and are not included in the list of walls. The interior walls always span from one exterior wall to another exterior wall and are arranged such that no more than two walls intersect at any point. You may assume that no two given walls coincide. After the listing of the interior walls there will be one final line containing the floating point coordinates of the treasure in the treasure room (guaranteed not to lie on a wall).
Output
Print a single line listing the minimum number of doors which need to be created, in the format shown below.
Sample Input
7 20 0 37 100 40 0 76 100 85 0 0 75 100 90 0 90 0 71 100 61 0 14 100 38 100 47 47 100 54.5 55.4
Sample Output
Number of doors = 2
Source
- 题意:在一个100*100的矩形中存在着条端点在边界上的墙,最多只能有2面墙交在同一点上,在其中有一个目标点,问从边界外进入内部到达目标点,需要开多少面墙,爆破墙面只能在墙的中点上。
- 我只想说,审题很重要,一开始把爆破墙面只能在墙的中点上理解成端点在边界上的墙的中点爆破而不是由各个墙面分割而成的相对更小的墙面,觉得很难处理Orz.
,看了discuss自己想岔了,实际是判断线段相交的问题,用连接端点和目标点的线段去判断相交,所得到的最小相交数+1就是答案了。而且枚举端点和枚举相邻端点的中点是一样,因为只要是从这两个相邻端点形成的墙面进去内部,内部还是一个封闭的,无法绕过墙来减少相交,所以相交数还是一样的。最外围的围墙爆破的墙面也要算进去,所以+1 - 代码如下
1 #include<iostream> 2 #include<cmath> 3 #include<algorithm> 4 using namespace std; 5 const int MAX = 1005; 6 const double eps = 1e-8; 7 typedef struct point { 8 double x; 9 double y; 10 point() { 11 12 } 13 point(double a, double b) { 14 x = a; 15 y = b; 16 } 17 }point; 18 typedef struct edge { 19 point start; 20 point end; 21 edge() { 22 23 } 24 edge(point a, point b) { 25 start = a; 26 end = b; 27 } 28 }edge; 29 30 int n; 31 double x, y, x11, y11, x2, y2; 32 point p1[MAX], p2[MAX],tg,tem;//两个端点 33 edge line[MAX], ed; 34 point know[4]{ 35 {0,0}, 36 {0,100}, 37 {100,0}, 38 {100,100} 39 }; 40 double multi(point p1, point p2, point p0) { //叉积 41 return (p2.y - p0.y)*(p1.x - p0.x) - (p2.x - p0.x)*(p1.y - p0.y); 42 } 43 44 inline double max(double a, double b) { return a > b ? a : b; } 45 inline double min(double a, double b) { return a < b ? a : b; } 46 bool Across(edge v1, edge v2) { //线段相交 47 if (max(v1.start.x, v1.end.x) >= min(v2.start.x, v2.end.x) && 48 max(v2.start.x, v2.end.x) >= min(v1.start.x, v1.end.x) && 49 max(v1.start.y, v1.end.y) >= min(v2.start.y, v2.end.y) && 50 max(v2.start.y, v2.end.y) >= min(v1.start.y, v1.end.y) && 51 multi(v2.start, v1.end, v1.start)*multi(v1.end, v2.end, v1.start) > 0 && 52 multi(v1.start, v2.end, v2.start)*multi(v2.end, v1.end, v2.start) > 0 53 ) 54 return true; 55 return false; 56 } 57 58 59 int main() { 60 cin >> n; 61 if (n == 0) { 62 cout << "Number of doors = 1" << endl; 63 return 0; 64 } 65 for (int i = 0; i < n; i++) { 66 cin >> x11 >> y11 >> x2 >> y2; 67 p1[i].x = x11, p1[i].y = y11; //一个端点 68 p2[i].x = x2, p2[i].y = y2; //另一个端点 69 line[i].start = p1[i]; 70 line[i].end = p2[i]; 71 } 72 cin >> x >> y; 73 tg.x = x, tg.y = y; 74 int ans = 0xffffff; 75 for (int i = 0; i < n; i++) { 76 ed.start = tg, ed.end = p1[i]; //目标点和其中一个端点连线 77 int cnt = 1; //最外围的围墙也要算 78 for (int j = 0; j < n; j++) { 79 if (Across(ed,line[j])) 80 cnt++; 81 } 82 ans = min(ans, cnt); 83 } 84 for (int i = 0; i < n; i++) { 85 ed.start = tg, ed.end = p2[i]; //目标点和其中一个端点连线 86 int cnt = 1; 87 for (int j = 0; j < n; j++) { 88 if (Across(ed, line[j])) 89 cnt++; 90 } 91 ans = min(ans, cnt); 92 } 93 for (int i = 0; i < 4; i++) { 94 ed.start = tg, ed.end = know[i]; //目标点和已知端点连线 95 int cnt = 1; 96 for (int j = 0; j < n; j++) { 97 if (Across(ed, line[j])) 98 cnt++; 99 } 100 ans = min(ans, cnt); 101 } 102 cout << "Number of doors = " <<ans<< endl; 103 return 0; 104 }