1. 最大堆结构定义如下:
// 最大堆结构定义
struct MaxHeap {
int* p; // 存储堆元素的数组
int size; // 堆当前元素个数
int capacity; // 堆的最大容量
};
2. 最大堆的基本操作函数如下:
- MaxHeap* createMaxHeap(int capacity); // 创建最大堆
- bool isFull(MaxHeap* maxHeap); // 判断最大堆是否已满)
- bool isEmpty(MaxHeap* maxHeap); // 判断最大堆是否为空
- void insertMaxHeap(MaxHeap* maxHeap, int val); // 插入元素
- void printMaxHeap(MaxHeap* maxHeap); // 遍历最大堆元素
- int deleteMax(MaxHeap* maxHeap); // 删除最大元素并返回
3. 具体代码实现如下:
#include <iostream>
#include <stdlib.h>
using namespace std;
#define MaxData 100000
// 最大堆结构定义
struct MaxHeap {
int* p; // 存储堆元素的数组
int size; // 堆当前元素个数
int capacity; // 堆的最大容量
};
// 创建最大堆
MaxHeap* createMaxHeap(int capacity) {
MaxHeap* maxHeap = (MaxHeap*)malloc(sizeof(MaxHeap));
maxHeap->p = (int*)malloc((capacity + 1) * sizeof(maxHeap->p));
maxHeap->size = 0;
maxHeap->capacity = capacity;
maxHeap->p[0] = MaxData; // 哨兵
return maxHeap;
}
// 判断最大堆是否已满
bool isFull(MaxHeap* maxHeap) {
if (maxHeap->size == maxHeap->capacity) {
return true;
}
return false;
}
// 判断最大堆是否为空
bool isEmpty(MaxHeap* maxHeap) {
if (maxHeap->size == 0) {
return true;
}
return false;
}
// 插入元素
void insertMaxHeap(MaxHeap* maxHeap, int val) {
if (maxHeap == NULL) {
cout << "The max heap is not created." << endl;
return;
}
if (isFull(maxHeap)) {
cout << "The max heap is full." << endl;
return;
}
int i = ++(maxHeap->size);
for (; maxHeap->p[i / 2] < val; i /= 2) {
maxHeap->p[i] = maxHeap->p[i / 2];
}
maxHeap->p[i] = val;
}
// 遍历最大堆元素
void printMaxHeap(MaxHeap* maxHeap) {
for (int i = 0; i < maxHeap->size; i++) {
cout << maxHeap->p[i + 1] << " ";
}
cout << endl;
}
// 删除最大元素并返回
int deleteMax(MaxHeap* maxHeap) {
if (maxHeap == NULL) {
cout << "The max heap is not created." << endl;
return 0;
}
if (isEmpty(maxHeap)) {
cout << "The max heap is empty." << endl;
return 0;
}
int max = maxHeap->p[1];
int last = maxHeap->p[maxHeap->size--];
int parent = 0;
int child = 0;
for (parent = 1; parent * 2 <= maxHeap->size; parent = child) {
child = parent * 2;
if (child != maxHeap->size && maxHeap->p[child] < maxHeap->p[child + 1]) {
child++;
}
if (last > maxHeap->p[child]) {
break;
}
else {
maxHeap->p[parent] = maxHeap->p[child];
}
}
maxHeap->p[parent] = last;
return max;
}
int main() {
MaxHeap* maxHeap = NULL;
maxHeap = createMaxHeap(10);
insertMaxHeap(maxHeap, 1);
insertMaxHeap(maxHeap, 2);
insertMaxHeap(maxHeap, 3);
insertMaxHeap(maxHeap, 4);
insertMaxHeap(maxHeap, 5);
printMaxHeap(maxHeap);
cout << deleteMax(maxHeap) << endl;
printMaxHeap(maxHeap);
cout << deleteMax(maxHeap) << endl;
printMaxHeap(maxHeap);
system("pause");
return 0;
}
4. 运行结果截图如下:
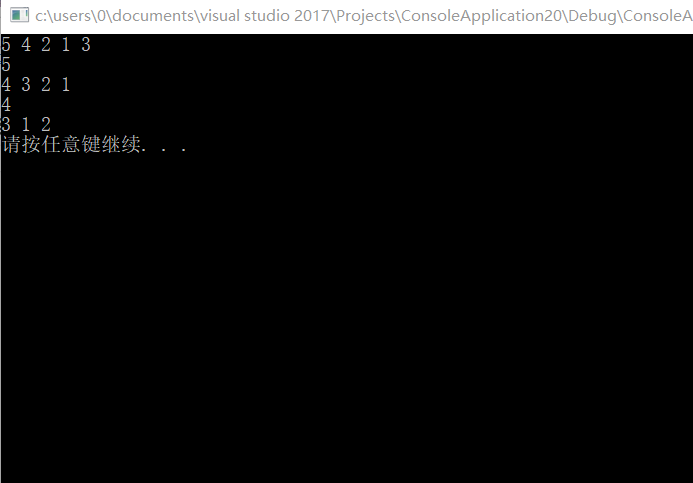