1. 顺序栈模型示意图如下:
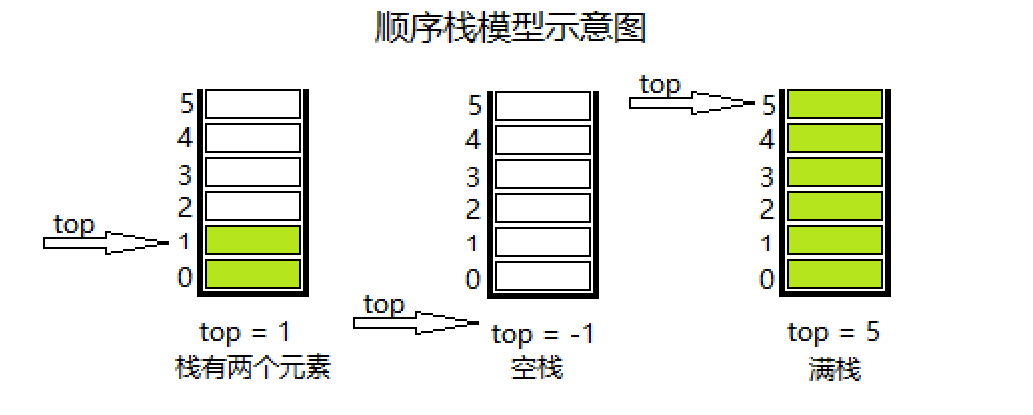
2. 顺序栈结构定义如下:
#define MAXSIZE 10
struct StackNode {
int data[MAXSIZE];
int top;
};
3. 顺序栈的基本操作函数如下:
- StackNode* createStack(); // 创建空栈
- void Push(StackNode* stack, int item); // 入栈
- int Pop(StackNode* stack); // 出栈,并返回出栈数据
- int getStackLength(StackNode* stack); // 获取栈元素个数
4. 具体代码实现如下:
#include <iostream>
using namespace std;
#define MAXSIZE 10
struct StackNode {
int data[MAXSIZE];
int top;
};
StackNode* createStack() {
StackNode* stack = (StackNode*)malloc(sizeof(StackNode));
if (stack == NULL) {
cout << "Memory allocate failed." << endl;
return NULL;
}
for (int i = 0; i < MAXSIZE; i++) {
stack->data[i] = 0;
}
stack->top = -1;
return stack;
}
void Push(StackNode* stack, int item) {
if (stack == NULL) {
cout << "The stack is not created." << endl;
return;
}
if (stack->top == MAXSIZE - 1) {
cout << "The stack is full." << endl;
return;
}
else {
stack->data[++(stack->top)] = item;
return;
}
}
int Pop(StackNode* stack) {
if (stack == NULL) {
cout << "The stack is not created." << endl;
return 0;
}
if (stack->top == -1) {
cout << "The stack is empty." << endl;
return 0;
}
else {
return (stack->data[(stack->top)--]);
}
}
int getStackLength(StackNode* stack) {
if (stack == NULL) {
cout << "The stack is not created." << endl;
return -1;
}
return (stack->top + 1);
}
int main() {
StackNode* stack = NULL;
stack = createStack();
Push(stack, 5);
Push(stack, 4);
Push(stack, 3);
cout << "The length of the stack is " << getStackLength(stack) << endl;
cout << Pop(stack) << endl;
cout << Pop(stack) << endl;
cout << Pop(stack) << endl;
cout << "The length of the stack is " << getStackLength(stack) << endl;
system("pause");
return 0;
}
5. 运行结果截图如下:
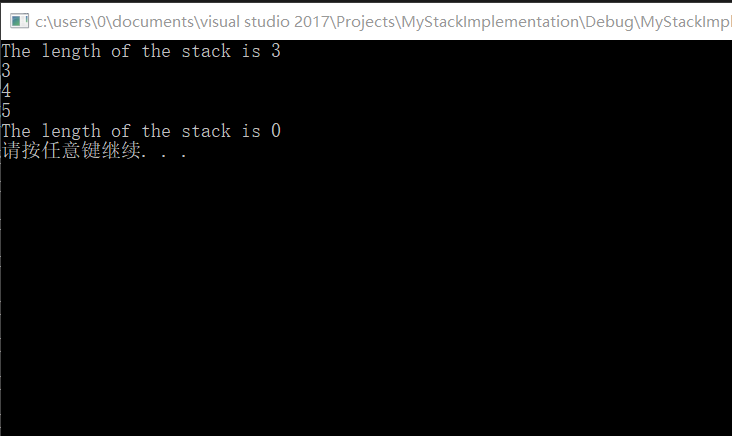