首先了解
二叉树顺序存储方式:
使用数组保存二叉树结构,使用层序遍历的方式放入数组中,遇到空结点就在该数组下标上放入‘#’(放啥随意只要可以表示为空就行),所以一般的树会造成造成空间的浪费,而完全二叉树就不会造成造成空间浪费。
所以堆(haep)的操作和实现我们就是利用数组的存储结构。
下标关系:
若是已知双亲结点parent的下标
其左孩子结点的下标就为:leftChild = 2parent + 1;
其有孩子结点的下标就为:rightChild = 2parent + 2;
若是知孩子child(无论左右)结点:
其parent结点下标就为:parent = (child - 1)/2;
堆(haep):
本质上就是一颗特殊的二叉树。
- 是完全二叉树。
- 要求树中任意结点:
当前结点的值必须大于或者等于其左孩子和右孩子结点的值—大堆、大根堆、大顶堆
当前结点的值必须小于或者等于其左孩子和右孩子结点的值—小堆、小根堆、小顶堆
大堆的操作和实现:
//堆的向下调整
public static void shiftDown(int[] array, int size, int index) {
int patent = index;
int child = 2 * patent + 1;
while (child < size) {
if(child + 1 < size && array[child + 1] > array[child]) {//取俩个结点的最大值
child += 1;
}
if(array[child] > array[patent]) {//孩子的值大于parent的值
int temp = array[child];
array[child] = array[patent];
array[patent] = temp;
}else {
break;
}
patent = child;
child = 2 * patent + 1;
}
}
//向上调整
public static void shiftUp(int[] array, int size, int index) {
int child = index;
int parent = (child - 1)/2;
while (child > 0) {
if(array[child] > array[parent]) {//孩子的值大于parent的值
int temp = array[child];
array[child] = array[parent];
array[parent] = temp;
}else {
break;
}
child = parent;
parent = (child - 1)/2;
}
}
利用向下调整{不推荐向下调整}(因为向下调整如果最大值在后几个数字会可能会建失败)或者向上调整都可以建大堆。
如果使用向下调整,我们在建堆的时候遍历数组要从前到后遍历。
int[] array = new int[] {9,5,2,7,3,6,8};
for (int i = 0; i < array.length; i++) {
shiftDown(array, array.length, i);
}
如果使用向上调整,我们在建堆的时候遍历数组要从后到前遍历。
int[] array1 = new int[] {9,5,2,7,3,6,8};
for (int i = array1.length - 1; i >= 0; i --) {
shiftUp(array1, array1.length ,i);
}
小堆的操作和实现:
//堆的向下调整
public static void shiftDown(int[] array, int size, int index) {
int patent = index;
int child = 2 * patent + 1;
while (child < size) {
if(child + 1 < size && array[child + 1] < array[child]) {//取俩个结点的最小值
child += 1;
}
if(array[child] < array[patent]) {//孩子的值小于parent的值
int temp = array[child];
array[child] = array[patent];
array[patent] = temp;
}else {
break;
}
patent = child;
child = 2 * patent + 1;
}
}
//向上调整
public static void shiftUp(int[] array, int size, int index) {
int child = index;
int parent = (child - 1)/2;
while (child > 0) {
if(array[child] < array[parent]) {//孩子的值小于parent的值
int temp = array[child];
array[child] = array[parent];
array[parent] = temp;
}else {
break;
}
child = parent;
parent = (child - 1)/2;
}
}
建大堆是每次循环找大的值,而建小堆就是每次循环找小的值。
同理建小堆的时候用向下调整还是会出现失败反例(最小值出现在后几个数字),而使用向上调整是不会出现这种情况的。
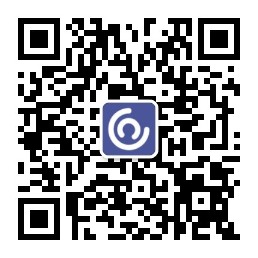
建小堆的时候用向下调整还是从前到后遍历数组。(因为index是根结点)
使用向上调整建立小堆的时候还是从后到前遍历数组。(因为index是最后一个叶子结点)
优先级队列的实现:
优先级队列本质还是队列只是我们在实现offer和poll操作的时候分别进行了向上调整和向下调整。(当然我们使用建大堆的向上和向下调整的话是值最高的优先级最高,最先出队列)
public class MyPriorQueue {
private int[] array = new int[100];
private int size = 0;
public void offer(int data) {
this.array[size] = data;
size ++;
shiftUp(array, size, size - 1);
}
private void shiftUp(int[] array, int size, int index) {
int child = index;
int parent = (child - 1)/2;
while (child > 0) {
if(array[child] > array[parent]) {
int temp = array[child];
array[child] = array[parent];
array[parent] = temp;
}else {
break;
}
child = parent;
parent = (child - 1)/2;
}
}
public Integer poll() {
if(size <= 0) {
return null;
}
int ret = this.array[0];
this.array[0] = this.array[size - 1];
size --;
shiftDown(array, size, 0);
return ret;
}
private void shiftDown(int[] array, int size, int index) {
int parent = index;
int child = 2 * parent + 1;
while (child < size) {
if(child + 1 < size && array[child] < array[child + 1]) {
child += 1;
}
if(array[child] > array[parent]) {
int temp = array[child];
array[child] = array[parent];
array[parent] = temp;
}else {
break;
}
parent = child;
child = 2 * parent + 1;
}
}
public Integer peek() {
if(size <= 0) {
return null;
}
return array[0];
}
public boolean empty() {
return size == 0;
}
/* public void display() {
for (int i:this.array
) {
System.out.print(i + " ");
}
System.out.println();
}*/
public int length() {
return size;
}
}
时间复杂度分析:
入队列-》向上调整O(logN)
出队列-》向下调整O(logN)
取队首元素O(1)
建堆操作的时间复杂度是O(N)
PriorityQueue 的使用:
(也就是库优先级队列)
public static void main(String[] args) {
PriorityQueue<Integer> pq = new PriorityQueue<>();
int[] nums = new int[] {3, 7, 4, 8, 3, 2, 9};
for (int i:nums
) {
pq.offer(i);
}
while (!pq.isEmpty()) {
System.out.print(pq.poll() + " ");
}
}
我们要注意库函数里的优先级队列使用的是建小堆的向上调整和向下调整。
库里的原始优先级队列遵循值越小,优先级越高思想。
当然我们也可以自己定义一个比较器对象,借助比较器我们自己定义谁优先谁不优先。
static class comp implements Comparator<Integer> {
@Override
public int compare(Integer o1, Integer o2) {
// return o1 - o2;
return o2 - o1;
}
}
public static void main(String[] args) {
PriorityQueue<Integer> pq = new PriorityQueue<>(new comp());
int[] nums = new int[] {3, 7, 4, 8, 3, 2, 9};
for (int i:nums
) {
pq.offer(i);
}
while (!pq.isEmpty()) {
System.out.print(pq.poll() + " ");
}
}
TopK 问题、堆排序:
只要掌握了优先级队列,这两问题是很简单的。
我们知道如果我们使用建大堆的调整思想,我们每次出队列的一定是当前队列里优先级最高的,也就是值最大的。
所以TopK我们只要出K次队列即可。
堆排序就是挨个出队列,就是这么简单。
升序:
public class Test {
static class comp implements Comparator<Integer> {
@Override
public int compare(Integer o1, Integer o2) {
// return o1 - o2;
return o2 - o1;
}
}
public static void main(String[] args) {
PriorityQueue<Integer> pq = new PriorityQueue<>(new comp());
int[] nums = new int[]{3, 7, 4, 8, 3, 2, 9};
for (int i : nums
) {
pq.offer(i);
}
while (!pq.isEmpty()) {
System.out.print(pq.poll() + " ");
}
}
}
执行结果:
9 8 7 4 3 3 2
降序:
public class Test {
static class comp implements Comparator<Integer> {
@Override
public int compare(Integer o1, Integer o2) {
return o1 - o2;
//return o2 - o1;
}
}
public static void main(String[] args) {
PriorityQueue<Integer> pq = new PriorityQueue<>(new comp());
int[] nums = new int[]{3, 7, 4, 8, 3, 2, 9};
for (int i : nums
) {
pq.offer(i);
}
while (!pq.isEmpty()) {
System.out.print(pq.poll() + " ");
}
}
}
运行结果:
2 3 3 4 7 8 9