一、素材处理
背景
(一)
在文件夹中找到back图片,并在检查器面板中将back图片的每单位像素数设置为16。
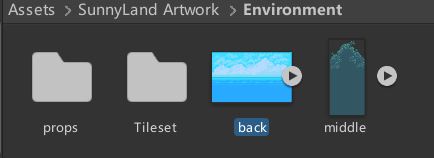
(文件所在地)
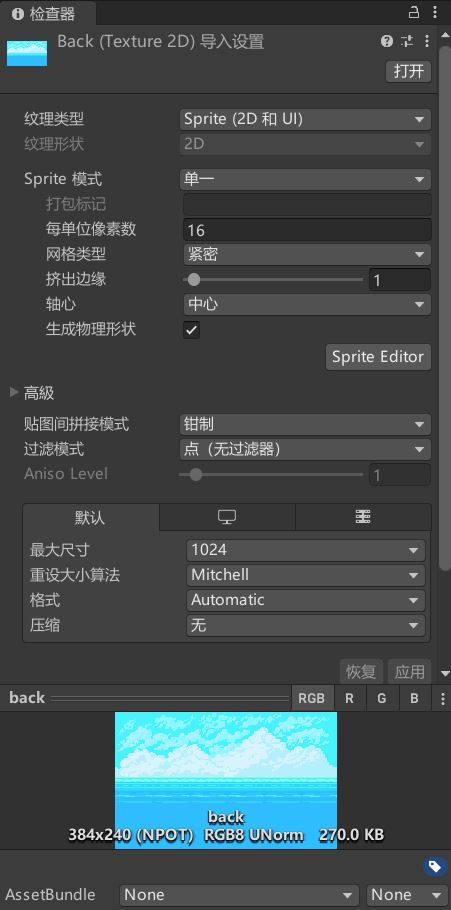
(面板设置)
(二)
将图片拖入到场景中
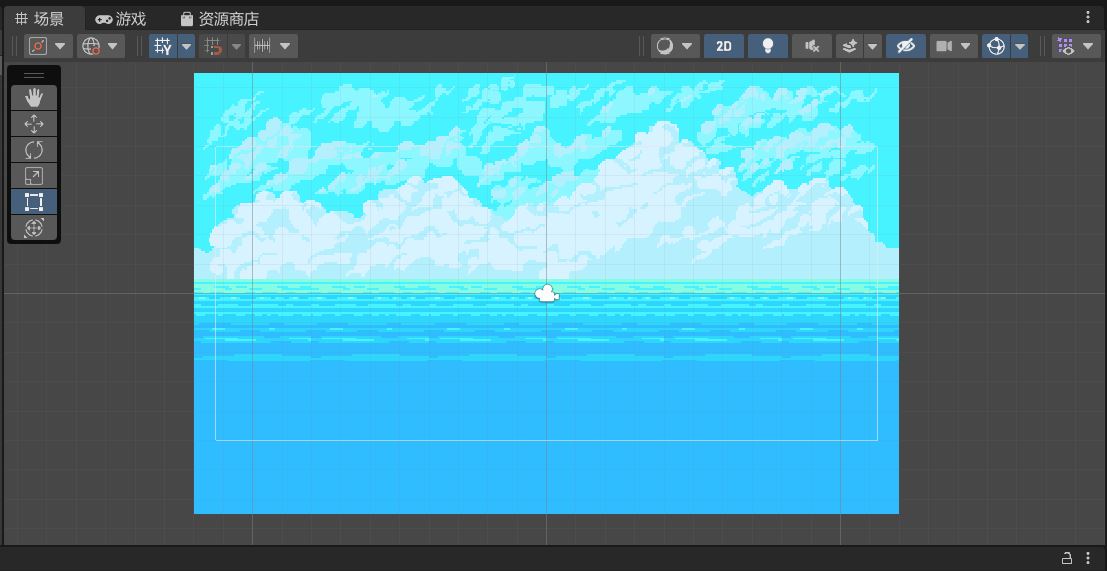
场景素材
(一)
生成矩形的瓦片地图
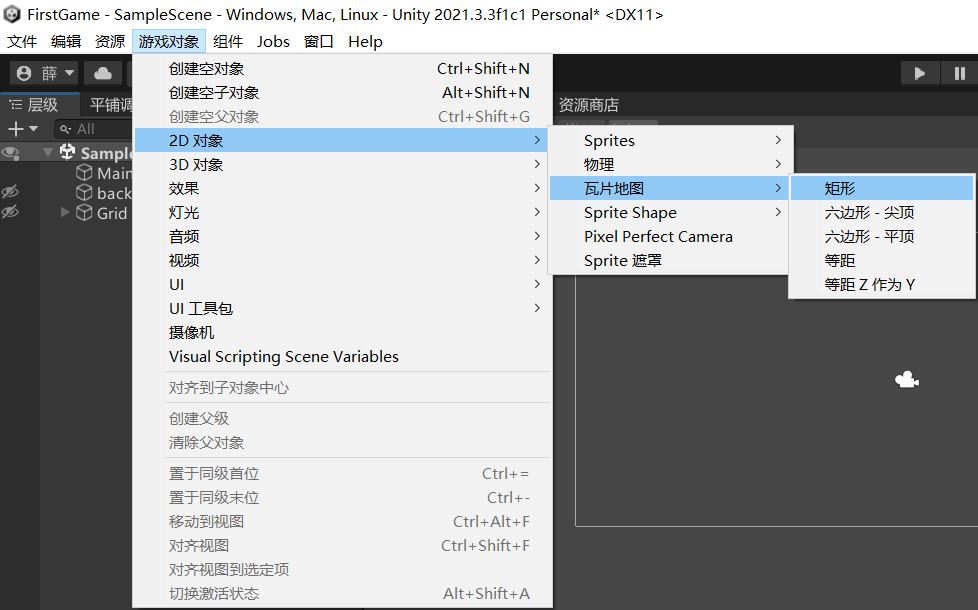
(二)
打开平铺调色板
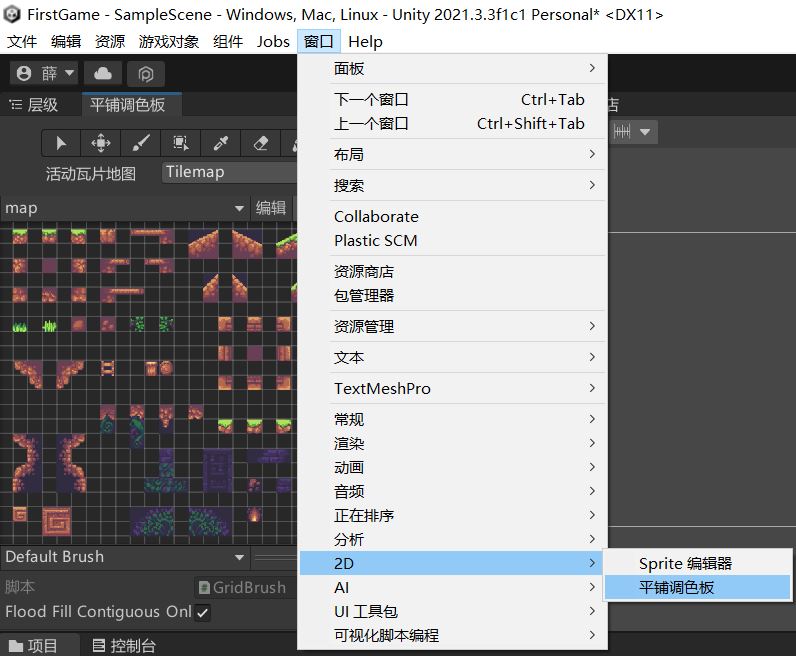
(三)
新建并命名为map,在原目录新建一个文件夹,用于存放你在这个瓦片地图里所使用的像素。
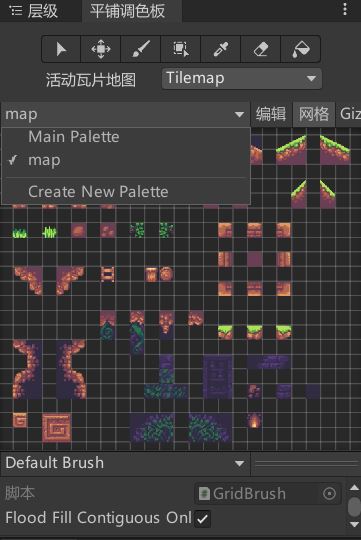
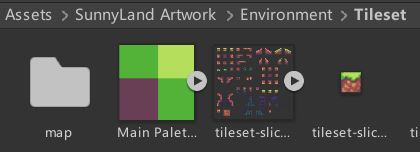
(四)
选中tileset-sliced,并在检查器面板中将tileset-sliced图片的每单位像素数设置为16。
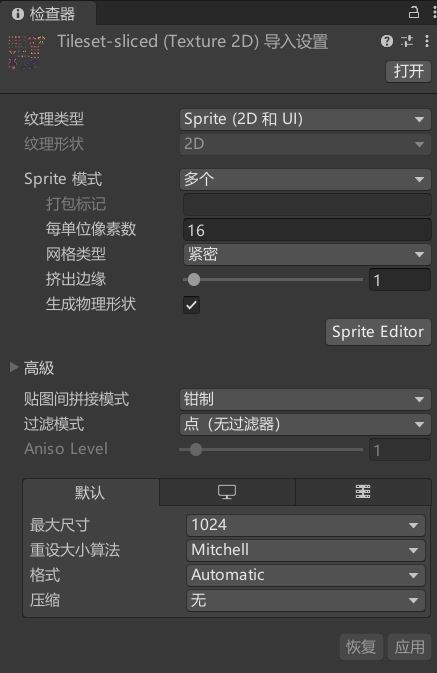
若没有切割好的tileset,选中tileset,并在检查器中将Sprite模式更改为多个并点击“Sprite Editor”,选择自定义切片,将数值更改为每单位像素数以方便你对每个像素的使用,最后点击“应用”确定。
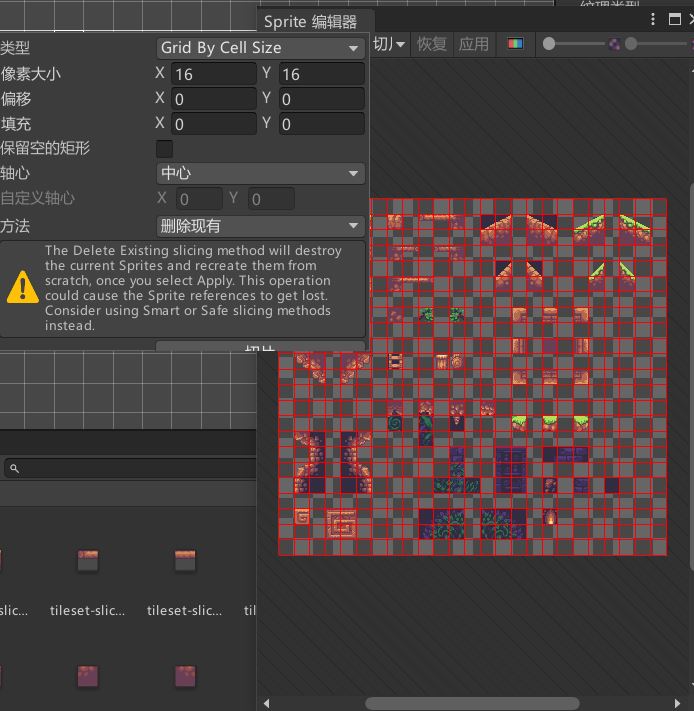
(五)
将修改完成的tileset-sliced放到平铺调色板中
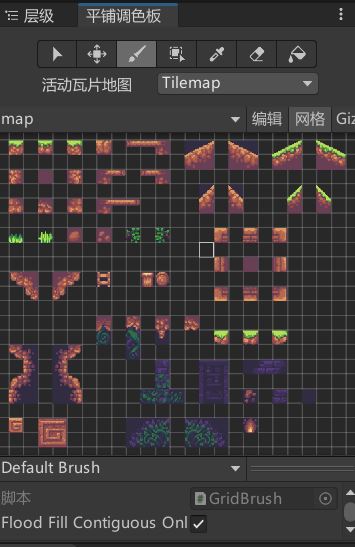
二、图层与角色建立
绘制场景
使用平铺调色板绘制来搭建场景
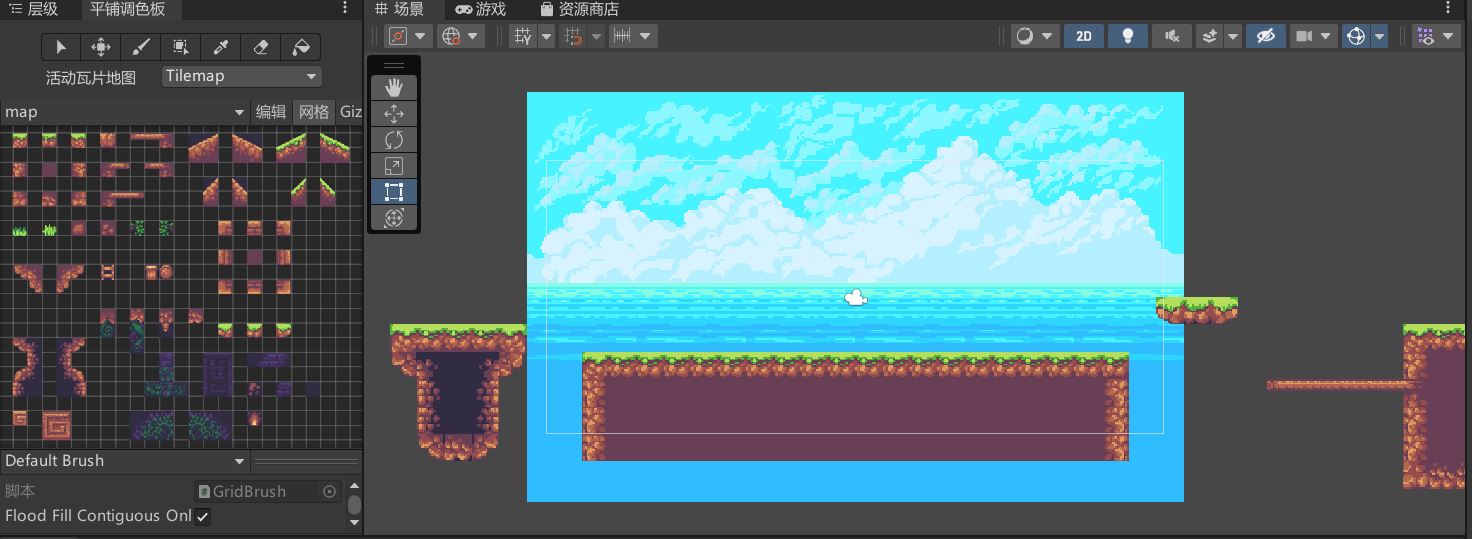
图层管理
在检查器中,选择排序图层添加标签“Background”“Fontground”.
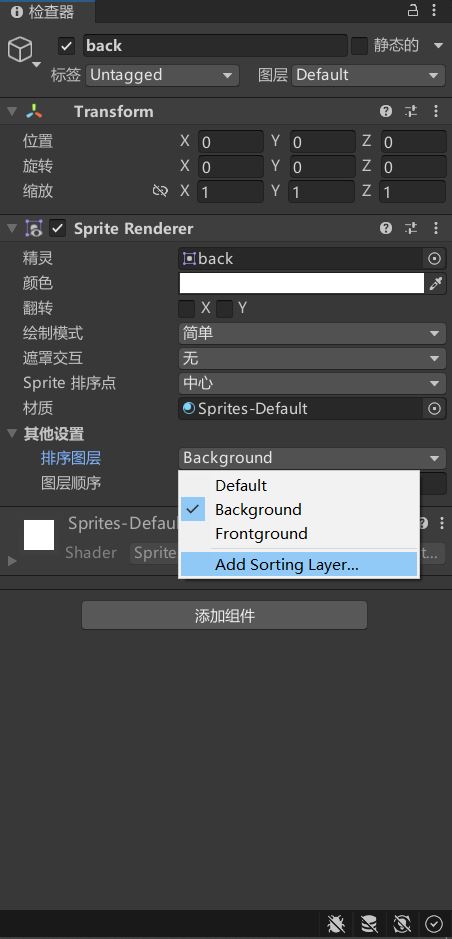
越是下面的图层,在场景中的显示就越上。
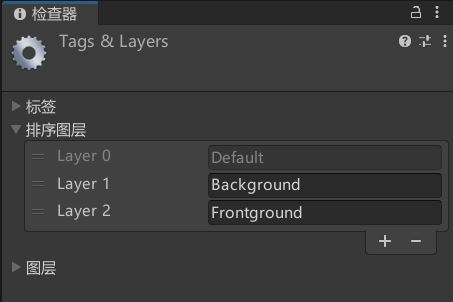
处于同一层的显示,则按照图层顺序的大小,越大则越优先显示。这里我们把背景图“back”的排序图层设置为“Background”,图层顺序设置为“0”;绘制的场景“Tilemap”的排序图层设置为“Background”,图层顺序设置为“1”,有需要可再做修改。

3.人物建立
(一)
在空白处右键新建一个精灵,选择正方形即可。
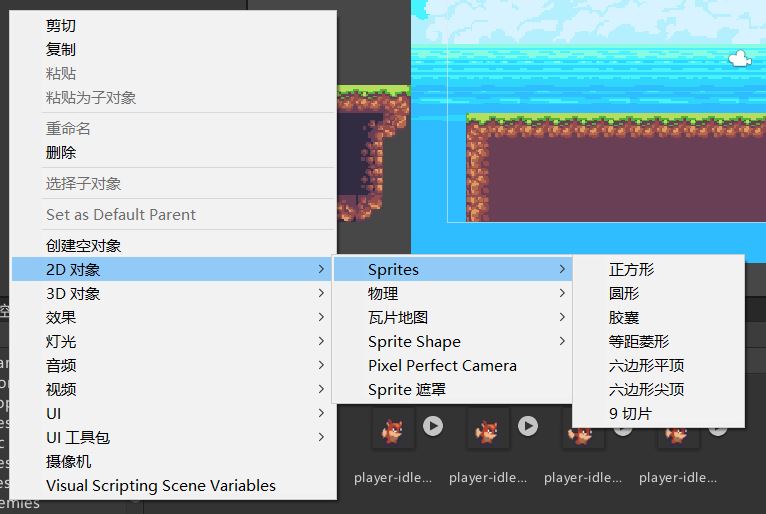
(二)
在下列途径中找到player-idle

记得更改每单位像素值
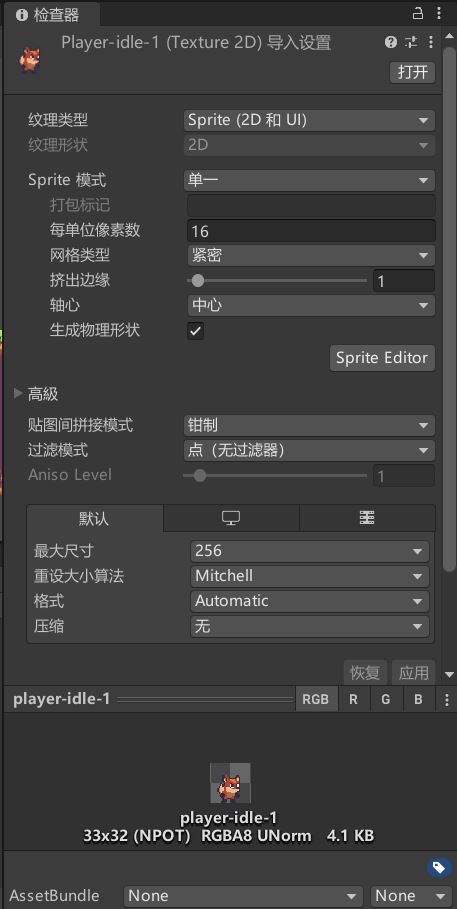
(三)
将player-idle-1拖入到sprite的组件Sprite Renderer中的精灵,并修改排序图层为Frontground。然后将sprite重命名为Player,将位置重置,接下来就能在场景中看见小狐狸了。
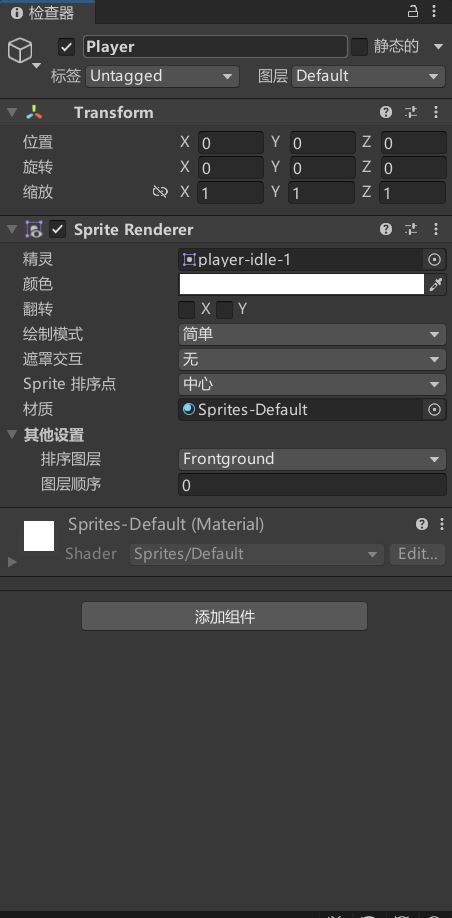
(四)
接下来要赋予Player重力以及碰撞,所以我们要给Player添加"Rigidbody 2D"组件和“Box Collider 2D”组件,再给我们绘制的地图“Tilemap”添加碰撞组件“Tilemap Collider 2D”。尝试运行,发现小狐狸收到重力影响掉落在绘制的场景中。
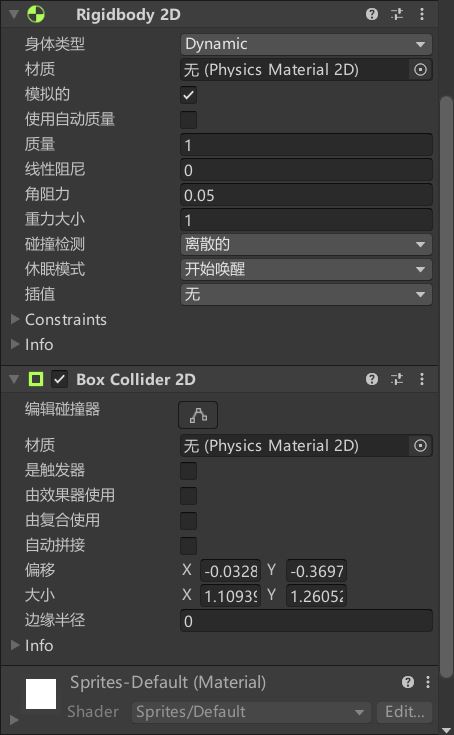
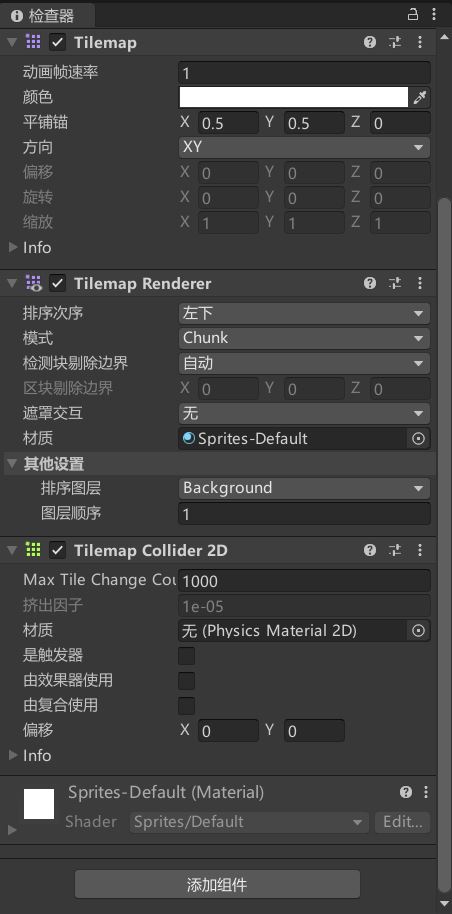
三、角色移动
1.Player移动脚本
新建脚本,命名为PlayerController,代码如下,实现对象根据按键来进行左右移动。请在控制面板锁定Player的rigbody2d组件的z轴。
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class PlayController : MonoBehaviour
{
public Rigidbody2D rb; //获取对象刚体
public float speed; //设置对象移动速度
// Start is called before the first frame update
void Start()
{
}
// Update is called once per frame
void Update()
{
Movement();
}
void Movement()//角色移动
{
float horizontalmove;
horizontalmove = Input.GetAxis("Horizontal");
if(horizontalmove != 0)
{
rb.velocity = new Vector2(horizontalmove * speed, rb.velocity.y);
}
}
}
(一)添加跳跃功能和对象转向功能
using UnityEngine;
using System.Collections;
using System.Collections.Generic;
public class PlayController : MonoBehaviour
{
public Rigidbody2D rb; //获取对象刚体
public float speed; //设置对象移动速度
public float jumpforce;//设置跳跃获得的纵向力
// Start is called before the first frame update
void Start()
{
}
// Update is called once per frame
void FixedUpdate()
{
Movement();
}
void Movement()//角色移动
{
float horizontalmove = Input.GetAxis("Horizontal");
float facedicetion = Input.GetAxisRaw("Horizontal");
//如果orizontalmove为1则向左走,-1则向右走
if (horizontalmove != 0)
{
rb.velocity = new Vector2(horizontalmove * speed * Time.deltaTime, rb.velocity.y);
}
//如果facedicetion为1则向左转,-1则向右转
if (facedicetion != 0)
{
transform.localScale = new Vector3(facedicetion, 1, 1);
}
//如果按下跳跃键,对象获得纵向力
if (Input.GetButtonDown("Jump"))
{
rb.velocity = new Vector2(rb.velocity.x,jumpforce * Time.deltaTime);
}
}
}
四、角色动画
前置工作
给Player添加组件Animator。
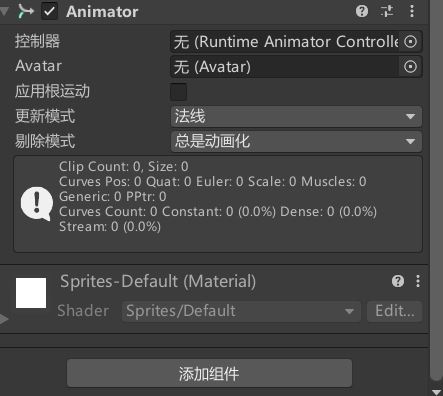
新建文件夹用于存放动画,并在对应文件夹新建动画控制器(Animator Contorller)。
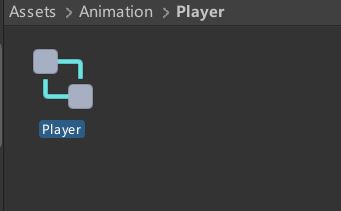
把动画控制器“Player”拖到对象“Player”的Animator组件。
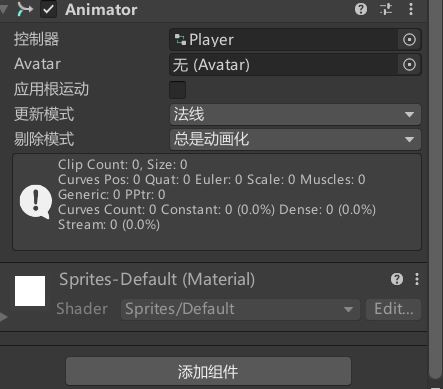
打开动画
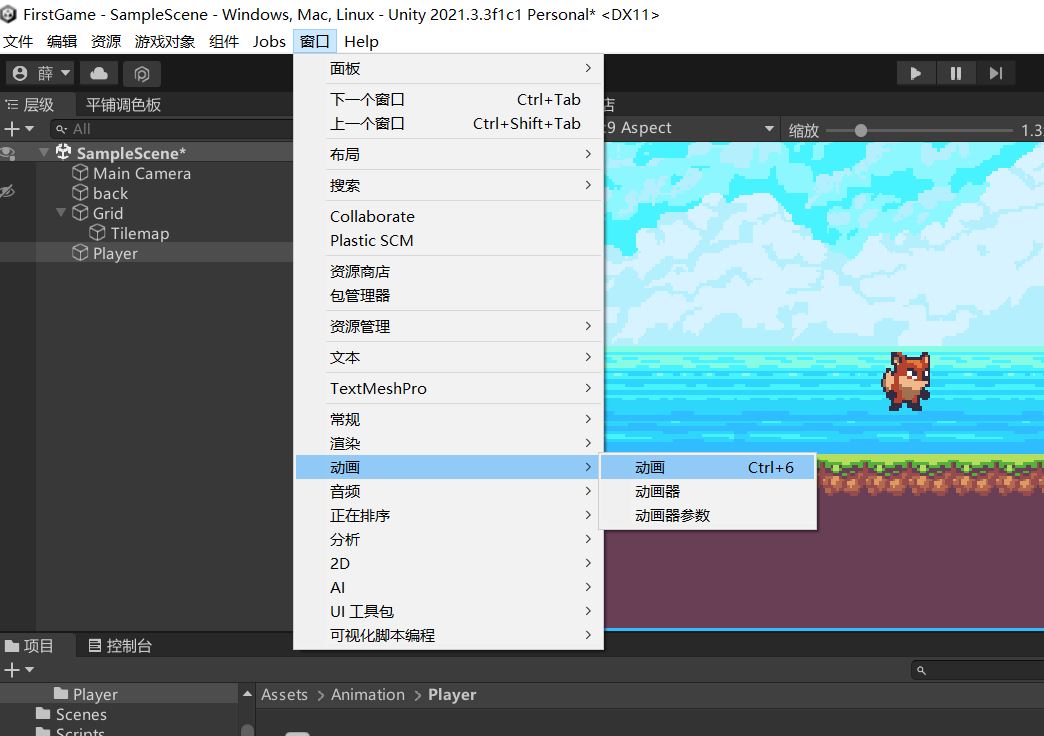
2.站立动画和跑动动画
单击对象“Player”,然后点击动画创建,在如下图所示找到对应的图片,放到Animation中,调整合适即可。
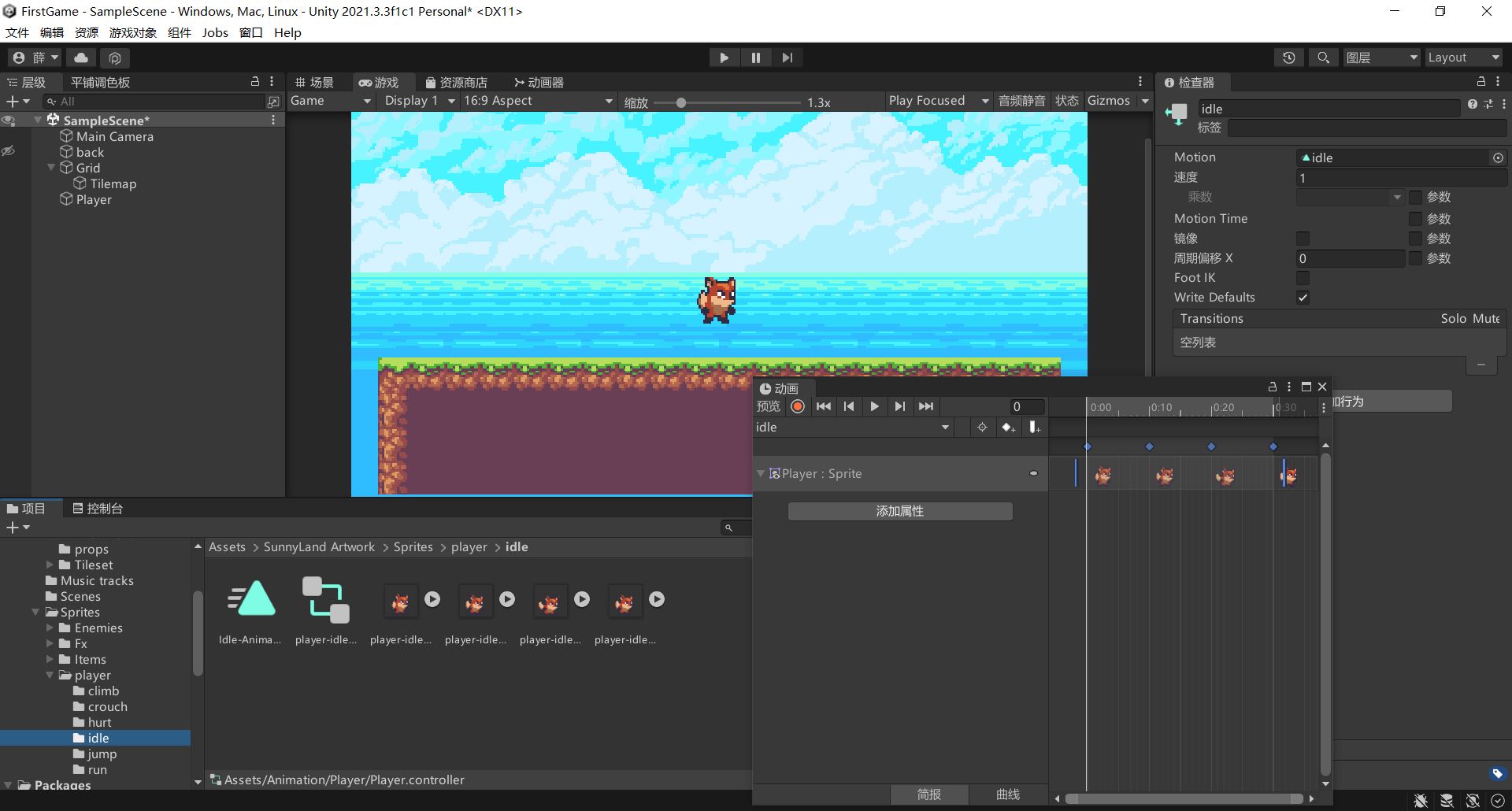
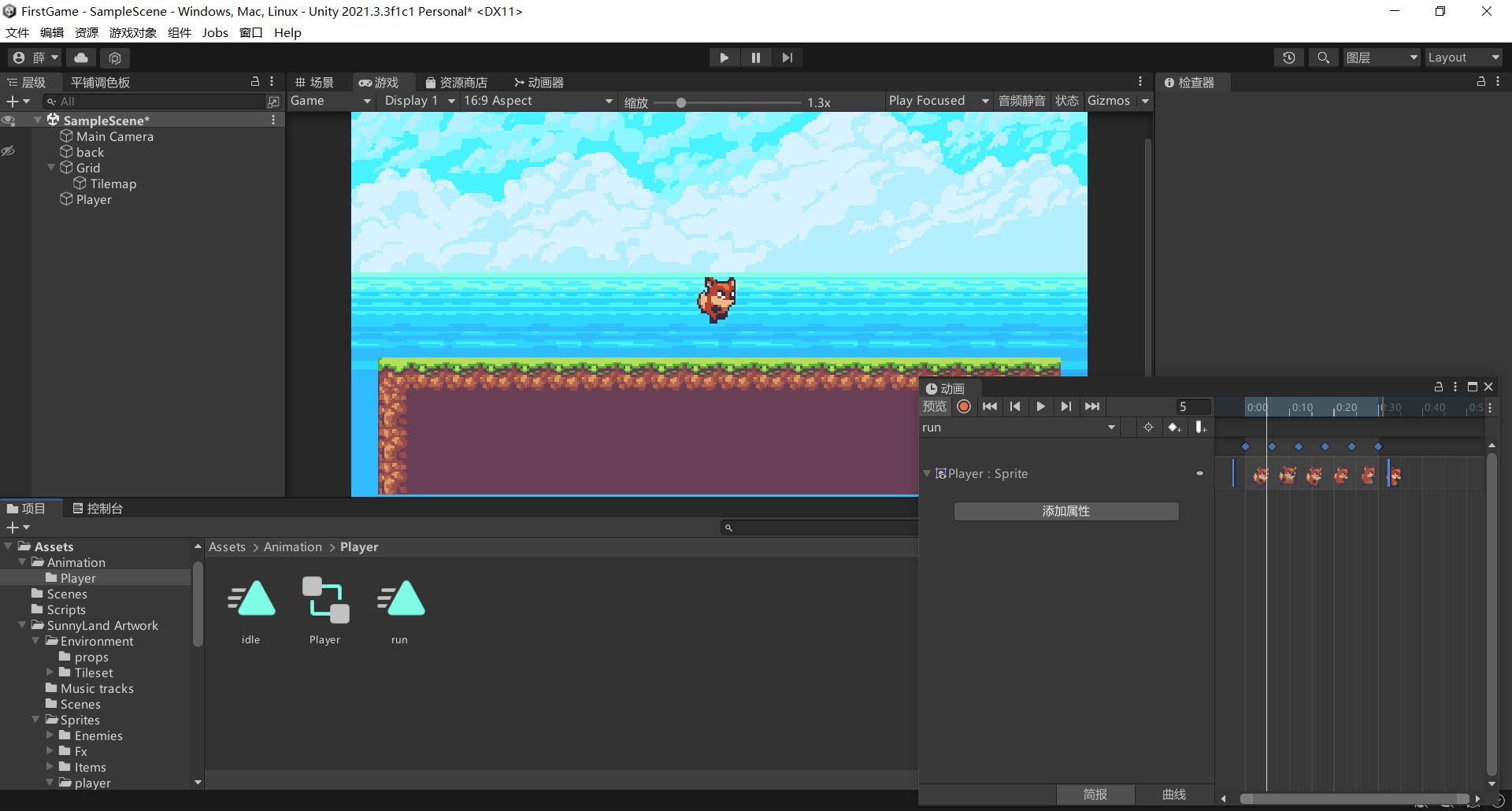
3.站立动画与跑动动画的转换
打开如下窗口
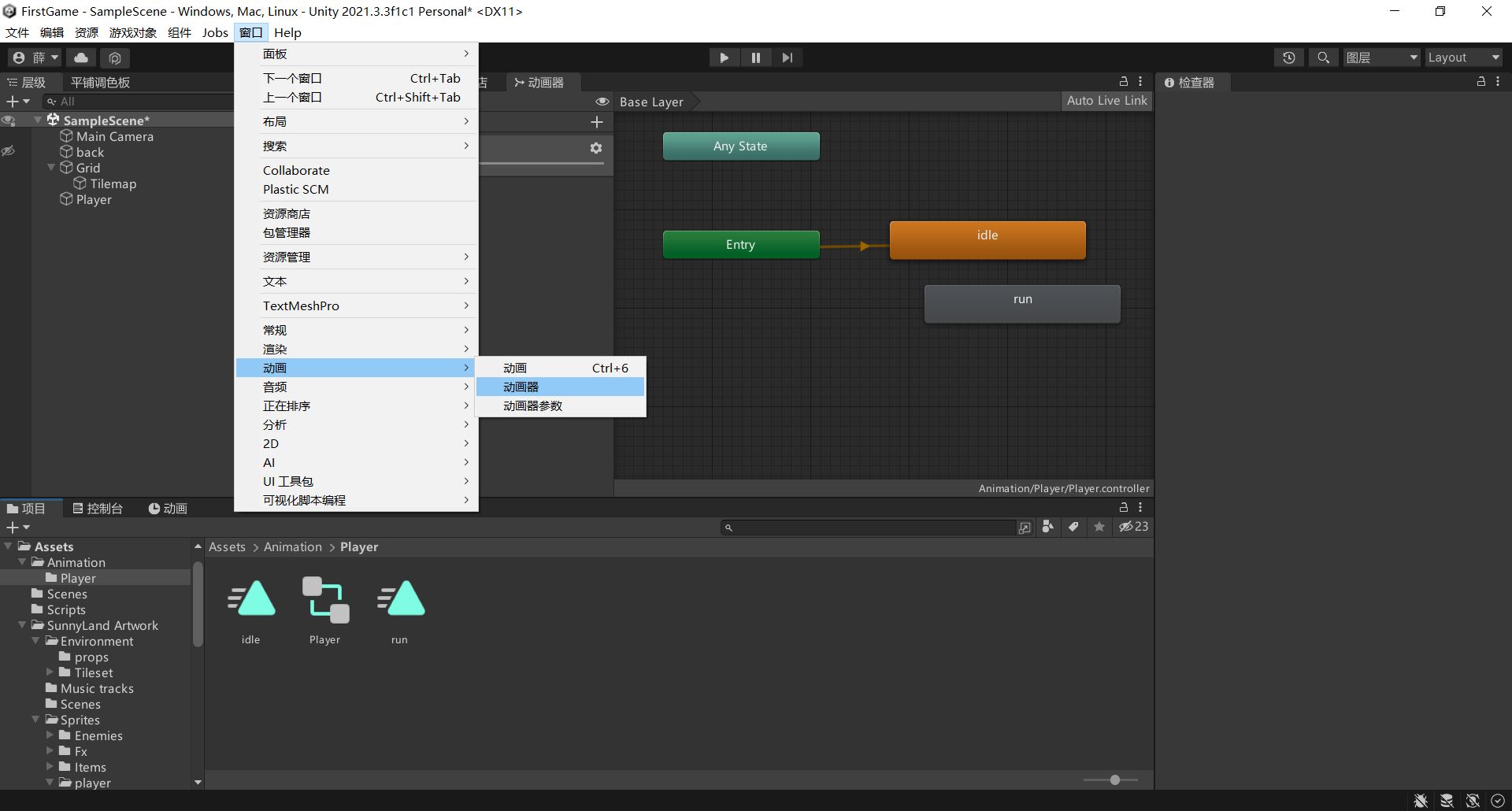
在“run”与“idle”两个动画之间创立过度
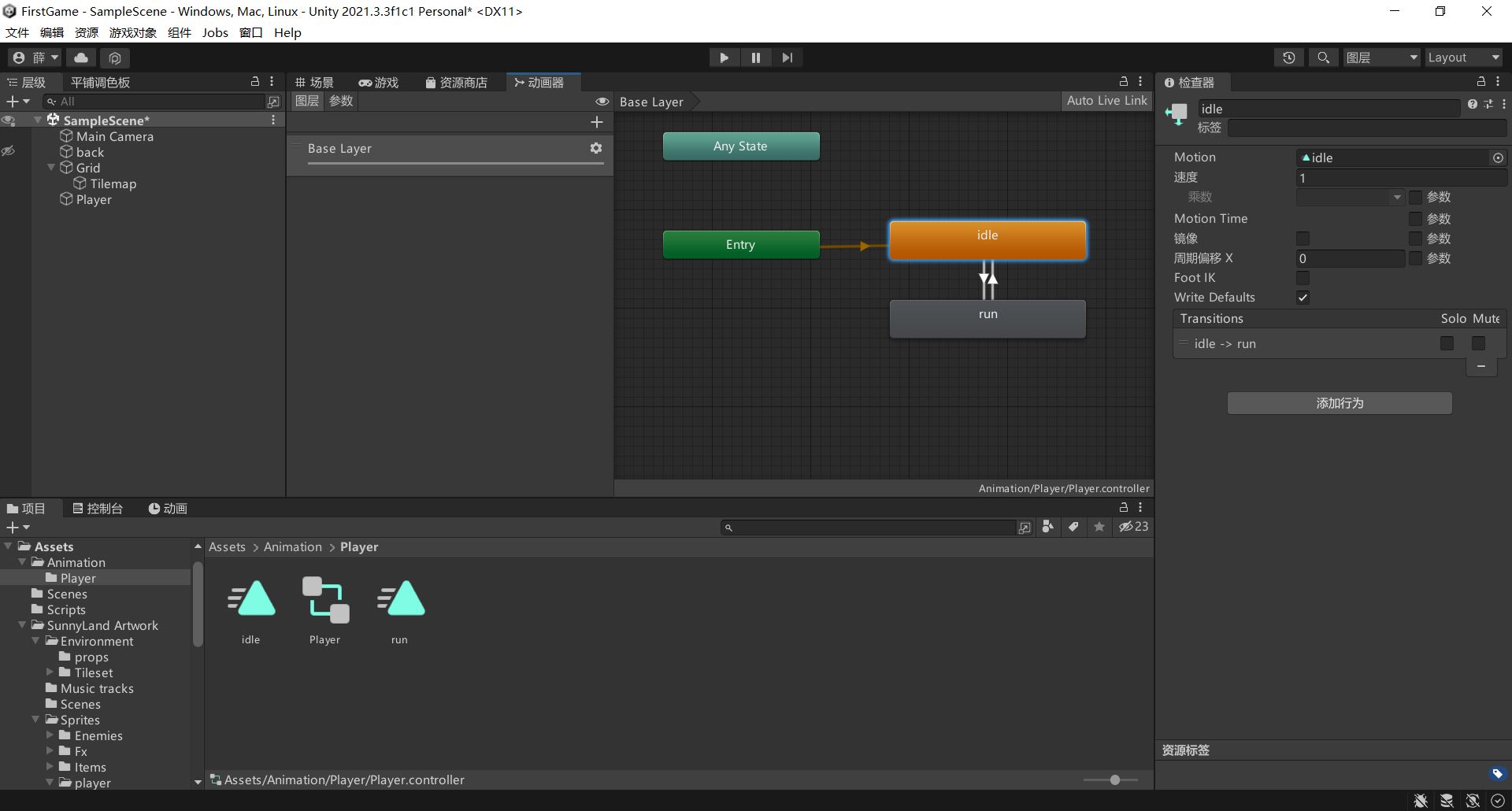
添加一个新参数“running”
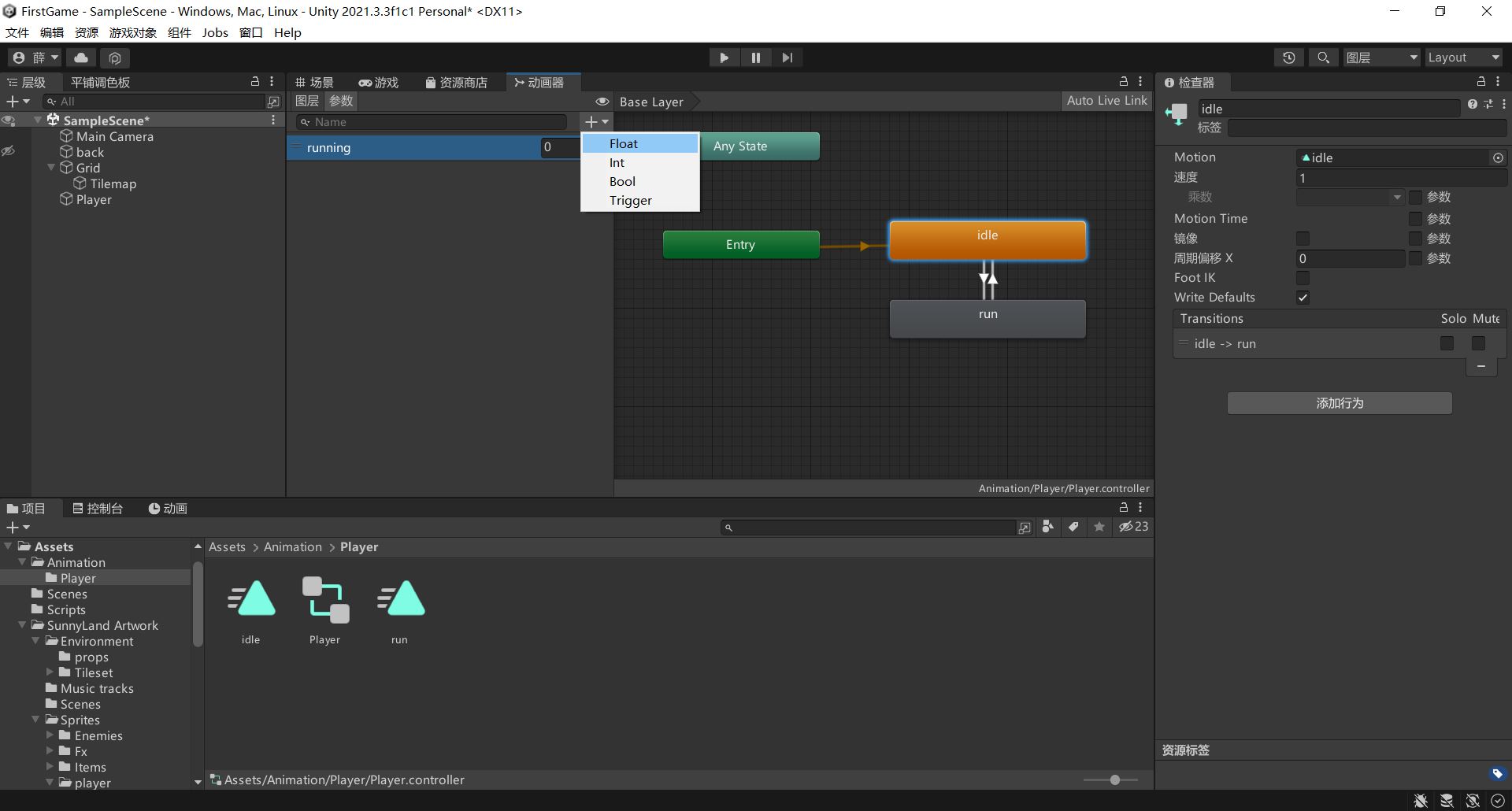
选中“idle”到“run”的过渡关系,进行如下调整:关闭退出时间,过渡持续时间为0,当移动速度大于0.1时,动画过度为“run”。同理设置“run”到“idle”的过渡关系。
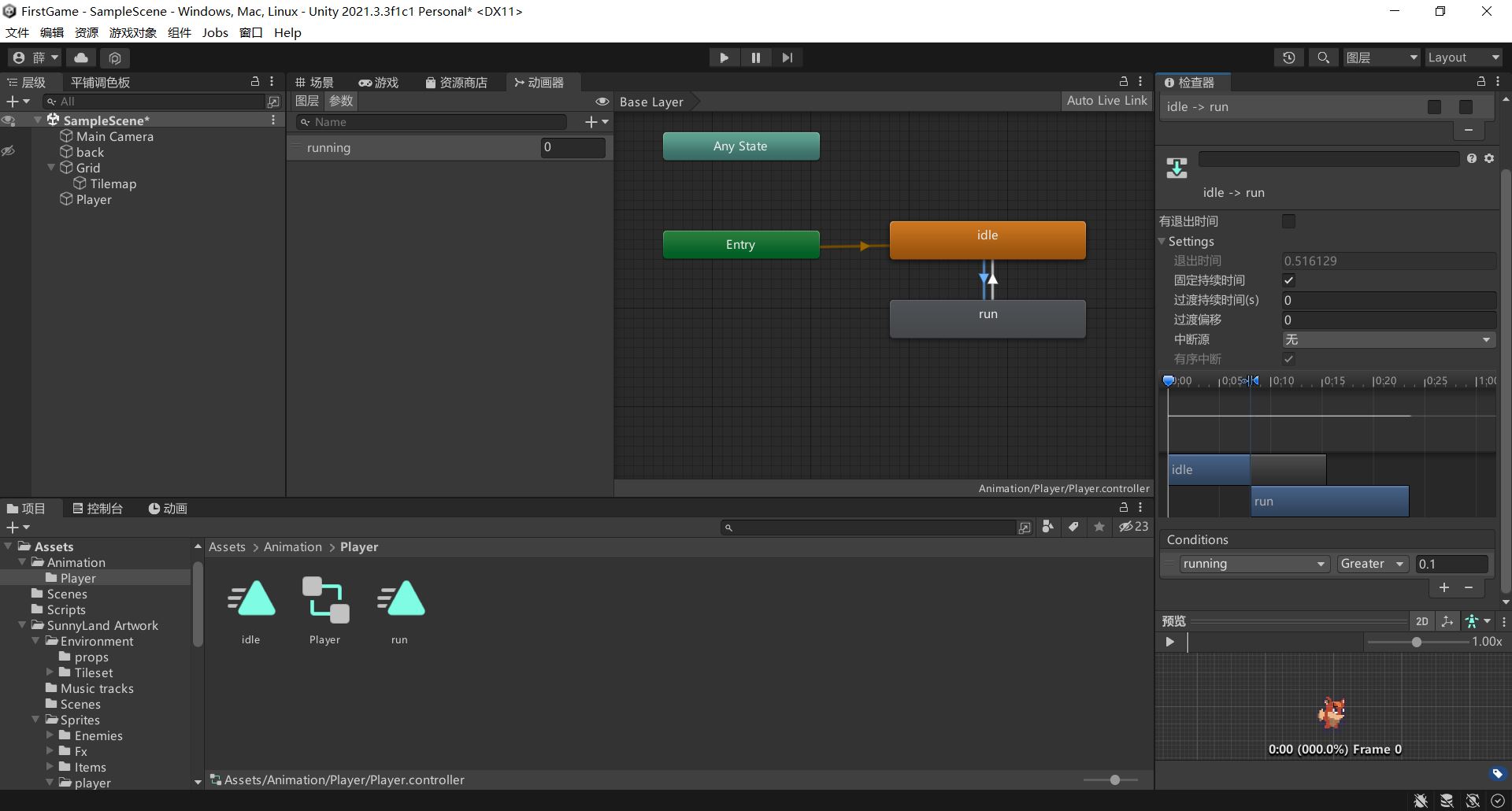
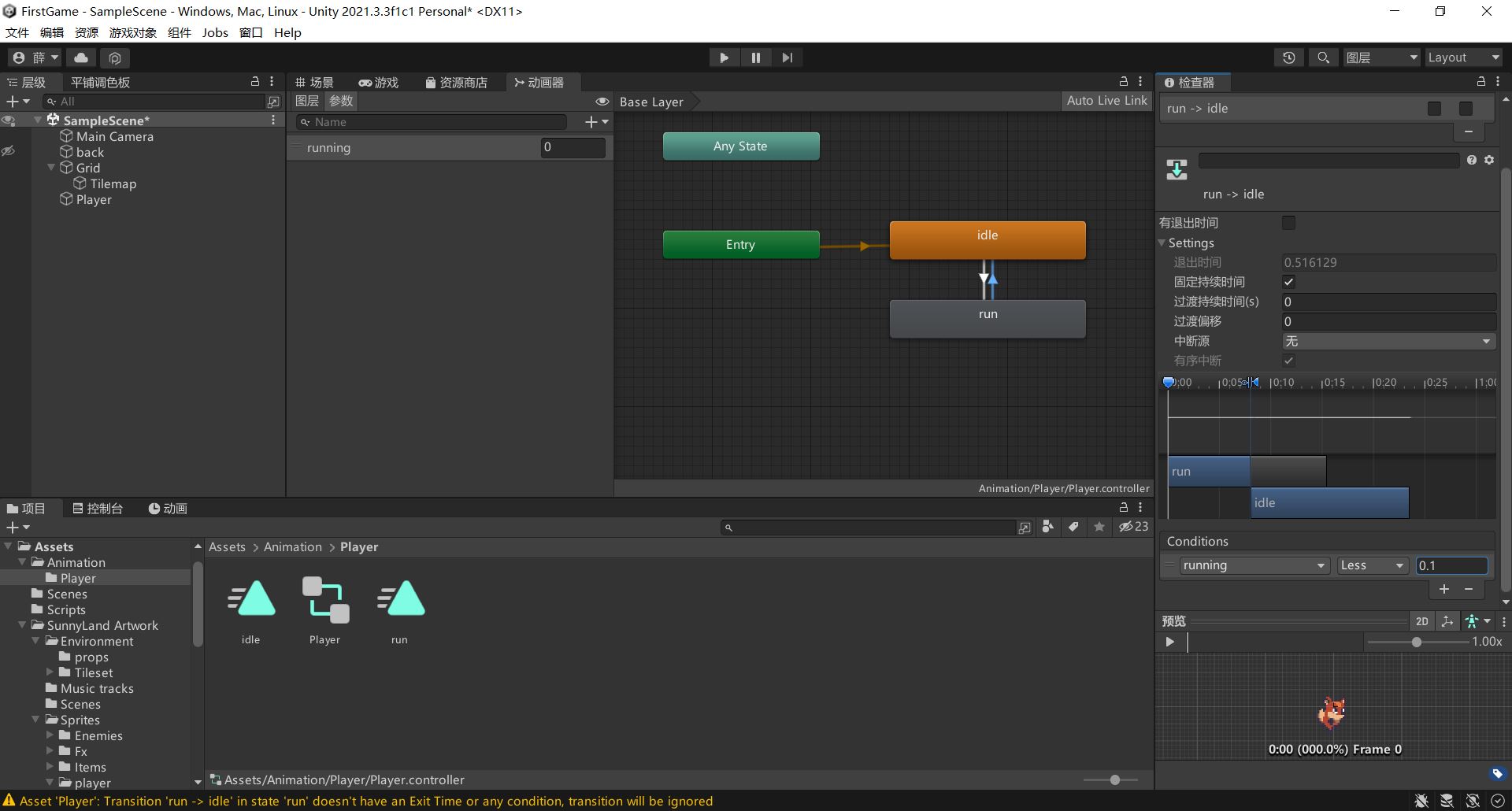
编辑脚本PlayerController.cs,添加由按键判断动画的功能。
using UnityEngine;
using System.Collections;
using System.Collections.Generic;
public class PlayController : MonoBehaviour
{
public Rigidbody2D rb; //获取对象刚体
public Animator anim; //获取对象动画组件
public float speed; //设置对象移动速度
public float jumpforce;//设置跳跃获得的纵向力
// Start is called before the first frame update
void Start()
{
}
// Update is called once per frame
void FixedUpdate()
{
Movement();
}
void Movement()//角色移动
{
float horizontalmove = Input.GetAxis("Horizontal");
float facedicetion = Input.GetAxisRaw("Horizontal");
//如果orizontalmove为1则向左走,-1则向右走
if (horizontalmove != 0)
{
rb.velocity = new Vector2(horizontalmove * speed * Time.deltaTime, rb.velocity.y);
anim.SetFloat("running", Mathf.Abs(horizontalmove)); //按左键时,horizontalmove为负数,动画由“run”转变为“idle”,故此处需要Mathf.Abs转变为绝对值
}
//如果facedicetion为1则向左转,-1则向右转
if (facedicetion != 0)
{
transform.localScale = new Vector3(facedicetion, 1, 1);
}
//如果按下跳跃键,对象获得纵向力
if (Input.GetButtonDown("Jump"))
{
rb.velocity = new Vector2(rb.velocity.x,jumpforce * Time.deltaTime);
}
}
}