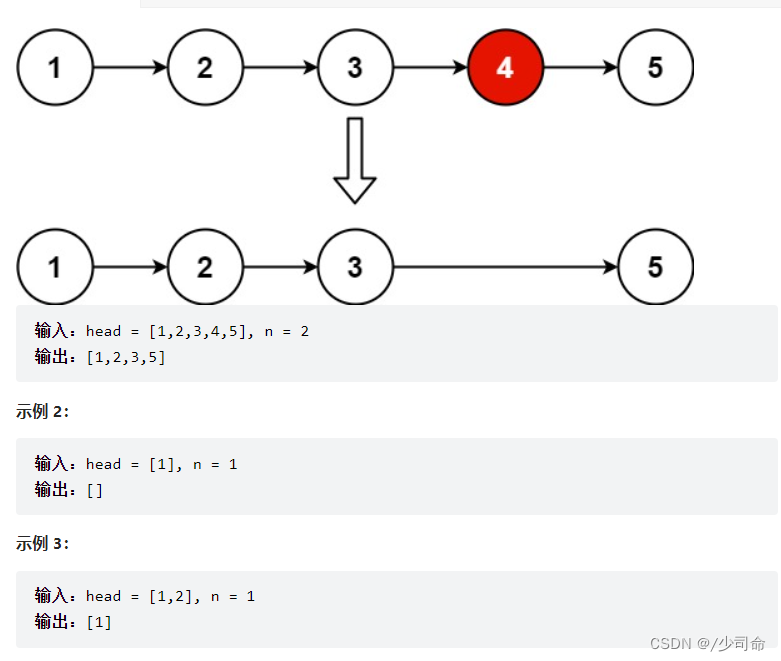
public ListNode removeNthFromEnd(ListNode head, int n) {
ListNode dummy = new ListNode(0,head);
Stack<ListNode> stack = new Stack<>();
ListNode cur = dummy;
while (cur != null){
stack.push(cur);
cur = cur.next;
}
for (int i = 0; i < n; i++){
stack.pop();
}
ListNode prev = stack.peek();
prev.next = prev.next.next;
return dummy.next;
}
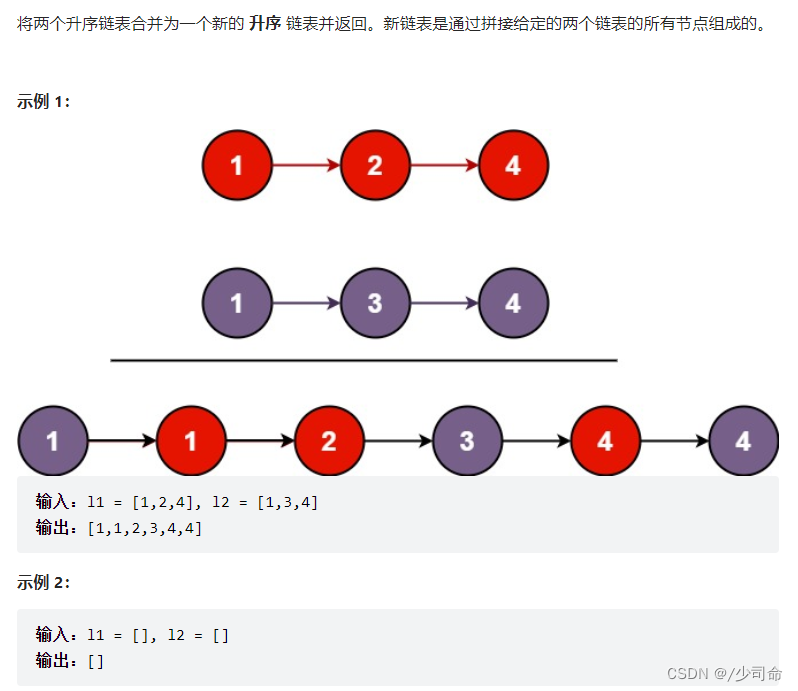
public ListNode mergeTwoLists(ListNode list1, ListNode list2) {
if (list1 == null){
return list2;
}
if (list2 == null){
return list1;
}
if (list1.val < list2.val){
list1.next = mergeTwoLists(list1.next,list2);
return list1;
}else {
list2.next = mergeTwoLists(list1,list2.next);
return list2;
}
}
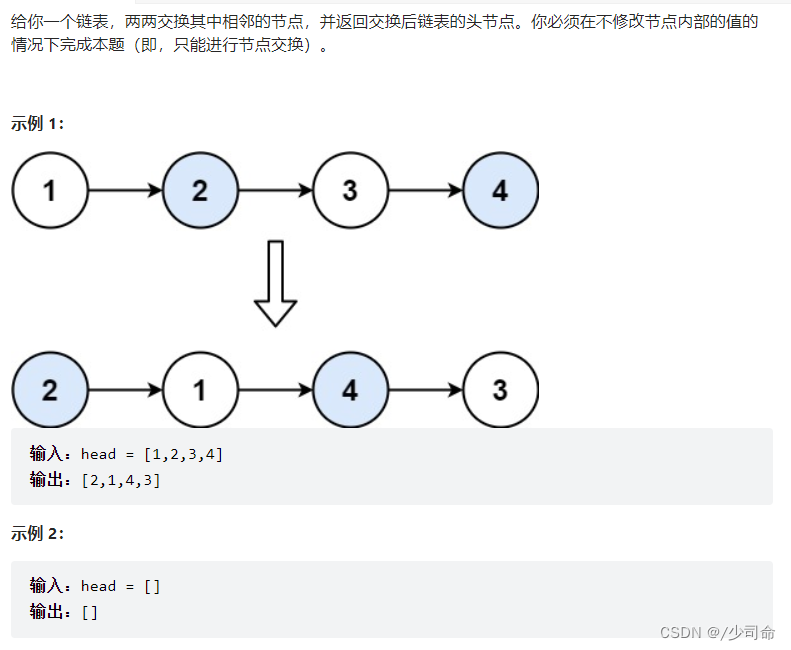
public ListNode swapPairs(ListNode head) {
if (head == null){
return null;
}
ListNode newHead = new ListNode(0);
newHead.next = head;
ListNode tmp = newHead;
while (tmp.next != null && tmp.next.next != null){
ListNode node1 = tmp.next;
ListNode node2 = tmp.next.next;
tmp.next = node2;
node1.next = node2.next;
node2.next = node1;
tmp = node1;
}
return newHead.next;
}
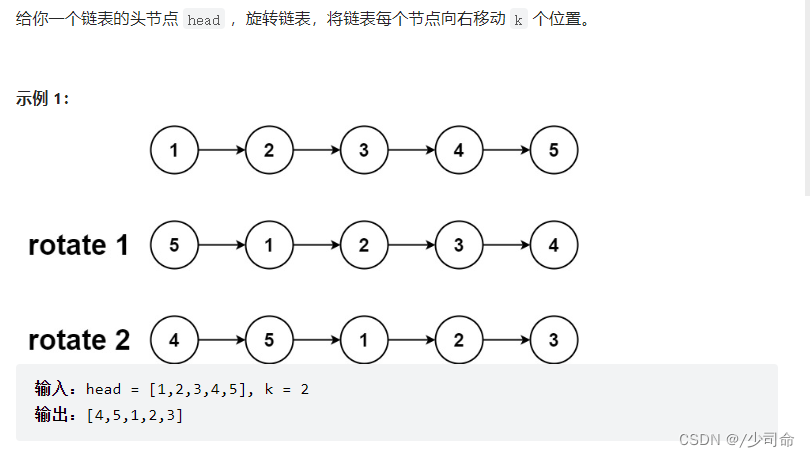
public ListNode rotateRight(ListNode head, int k) {
if (head == null || head.next == null || k == 0){
return head;
}
int count = 1;
ListNode tmp = head;
while (tmp.next != null){
count++;
tmp = tmp.next;
}
k %= count;
if (k == 0){
return head;
}
//首尾相连
tmp.next = head;
for (int i = 0; i < count - k; i++){
tmp = tmp.next;
}
ListNode newHead = tmp.next;
tmp.next = null;
return newHead;
}
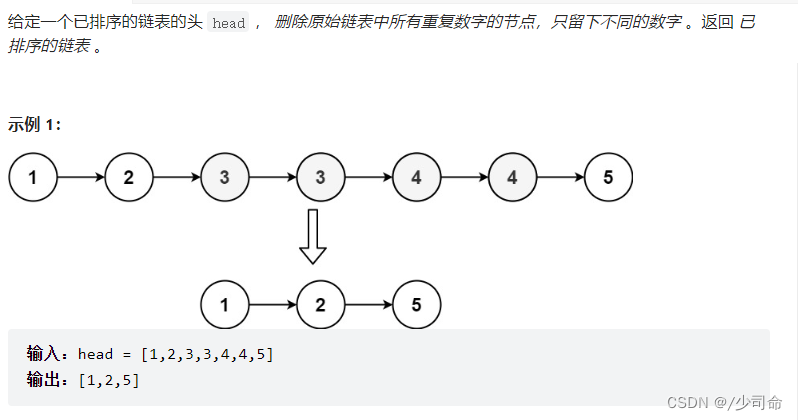
public ListNode deleteDuplicates(ListNode head) {
if (head == null){
return head;
}
ListNode newHead = new ListNode(0,head);
ListNode cur = newHead;
while (cur.next != null && cur.next.next != null){
if (cur.next.val == cur.next.next.val){
int x = cur.next.val;
while (cur.next != null && cur.next.val == x){
cur.next = cur.next.next;
}
}else {
cur = cur.next;
}
}
return newHead.next;
}
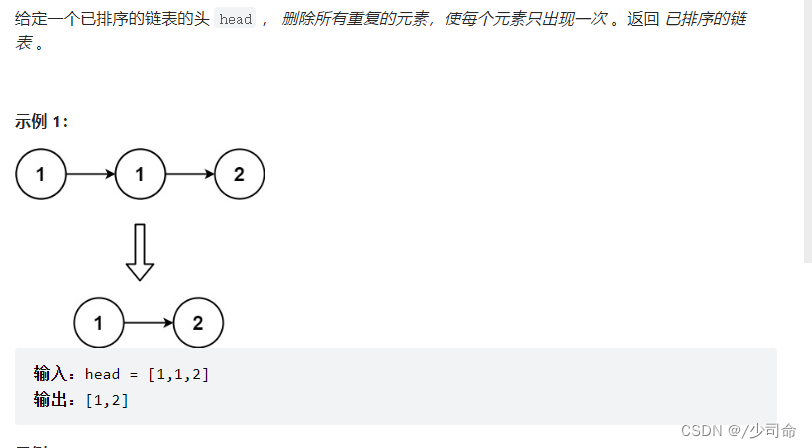
public ListNode deleteDuplicates(ListNode head) {
if (head == null){
return null;
}
ListNode cur = head;
while (cur != null && cur.next != null){
if (cur.val == cur.next.val){
cur.next = cur.next.next;
}else {
cur = cur.next;
}
}
return head;
}
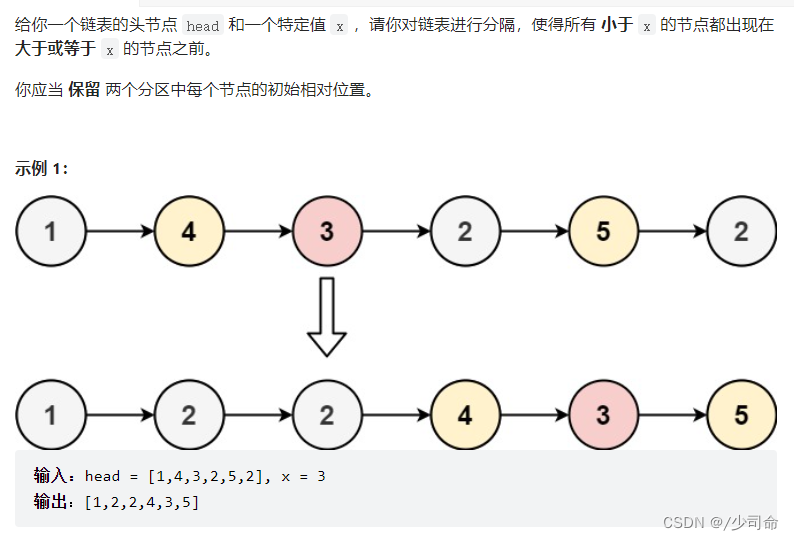
public ListNode partition(ListNode head, int x) {
ListNode small = new ListNode(0);
ListNode smallHead = small;
ListNode large = new ListNode(0);
ListNode largeHead = large;
while (head != null){
if (head.val < x){
small.next = head;
small = small.next;
}else {
large.next = head;
large = large.next;
}
head = head.next;
}
large.next = null;
small.next = largeHead.next;
return smallHead.next;
}
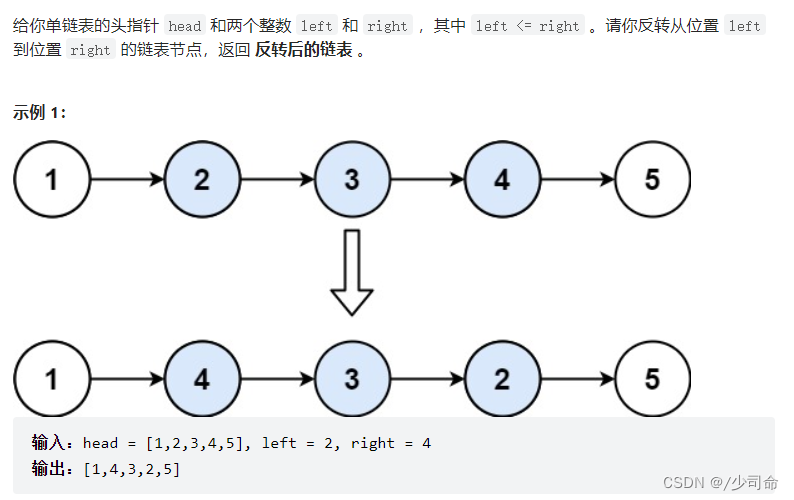
public ListNode reverseBetween(ListNode head, int left, int right) {
ListNode newHead = new ListNode(0);
ListNode pre = newHead;
newHead.next = head;
for (int i = 0; i < left-1; i++){
pre = pre.next;
}
ListNode cur = pre.next;
ListNode net;
for (int i = 0; i < right - left; i++){
net = cur.next;
cur.next = net.next;
net.next = pre.next;
pre.next = net;
}
return newHead.next;
}
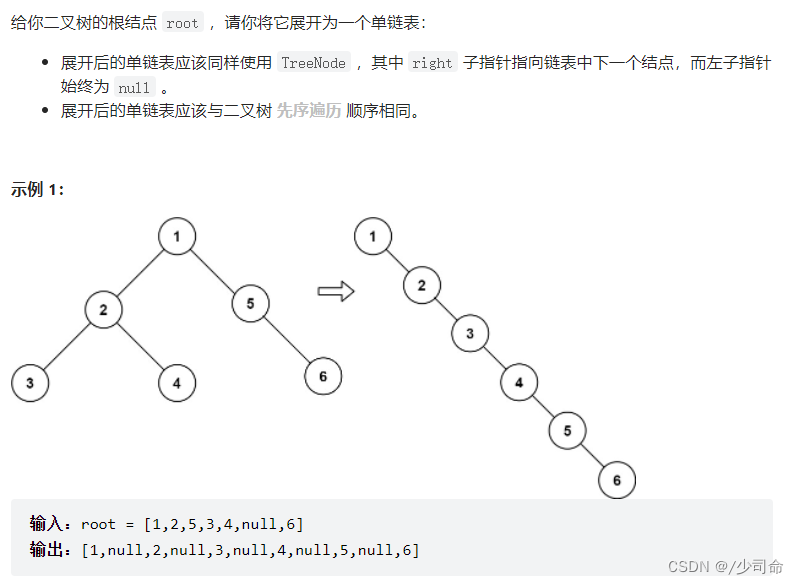
TreeNode pre = null;
public void flatten(TreeNode root) {
if (root == null){
return;
}
flatten(root.right);
flatten(root.left);
root.right = pre;
root.left = null;
pre = root;
}
}
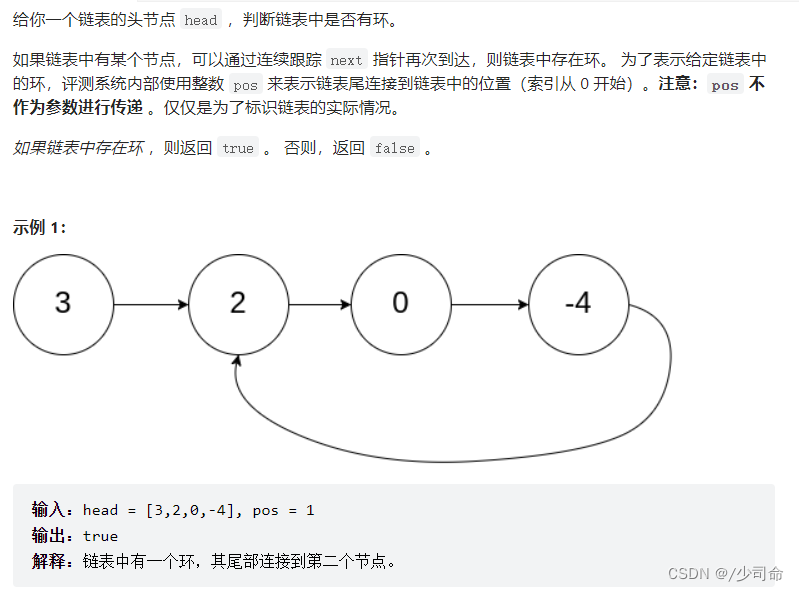
public boolean hasCycle(ListNode head) {
if (head == null || head.next == null){
return false;
}
ListNode slow = head;
ListNode fast = head.next;
while (slow != fast){
if (fast == null || fast.next == null){
return false;
}
slow = slow.next;
fast = fast.next.next;
}
return true;
}
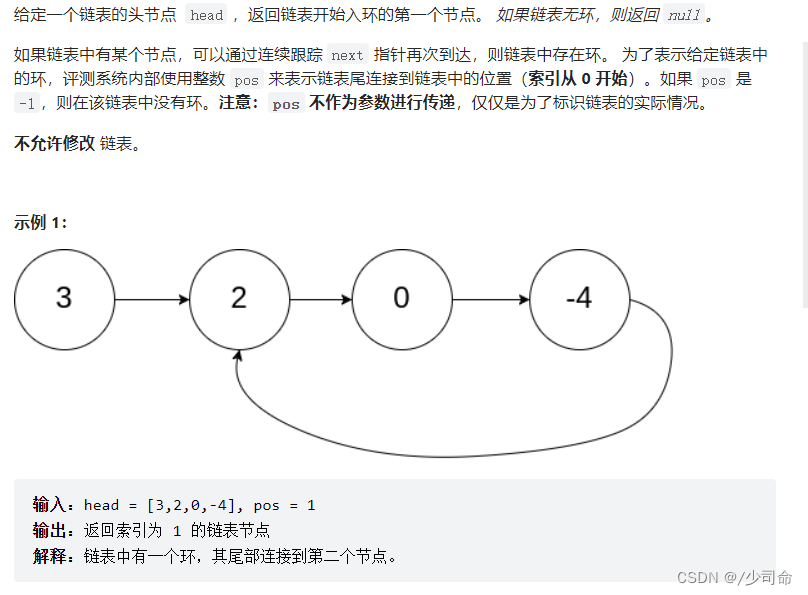
public ListNode detectCycle(ListNode head) {
ListNode pos = head;
HashSet<ListNode> set = new HashSet<>();
while (pos != null){
if (set.contains(pos)){
return pos;
}else {
set.add(pos);
}
pos = pos.next;
}
return null;
}
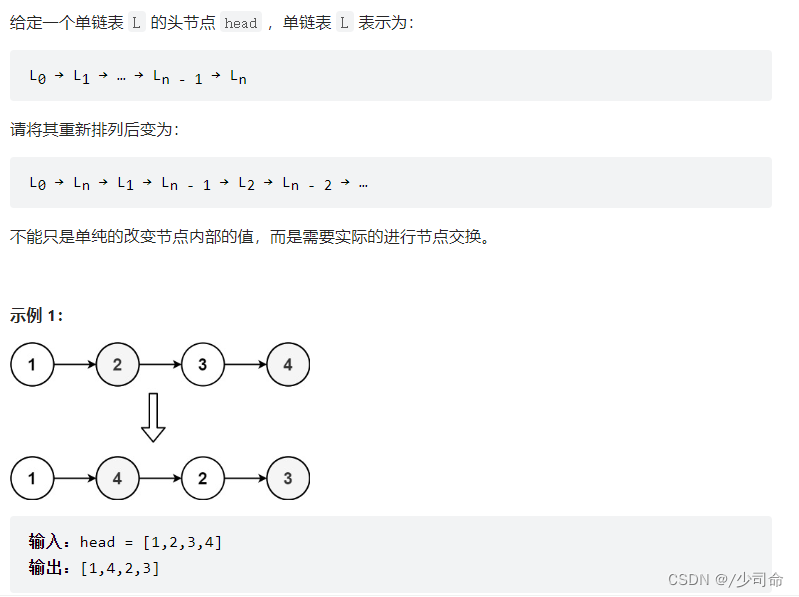
public void reorderList(ListNode head) {
if (head == null){
return;
}
List<ListNode> list = new ArrayList<>();
ListNode node = head;
while (node != null){
list.add(node);
node = node.next;
}
int i = 0, j = list.size() - 1;
while (i < j){
list.get(i).next = list.get(j);
i++;
if (i == j){
break;
}
list.get(j).next = list.get(i);
j--;
}
list.get(i).next = null;
}
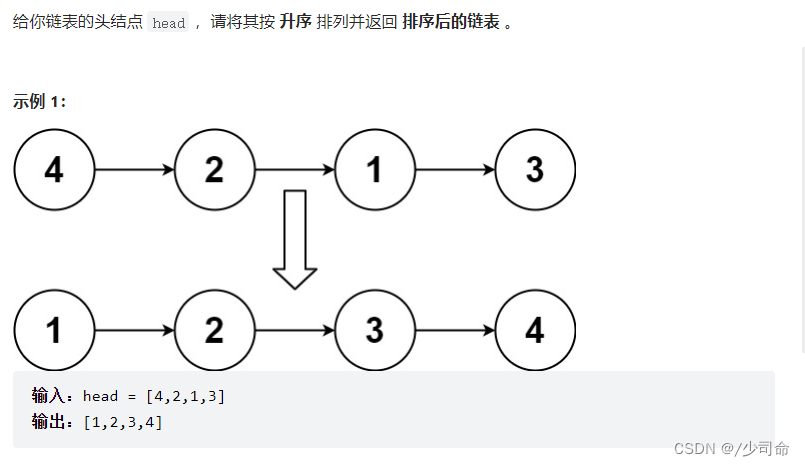
public ListNode sortList(ListNode head) {
if (head == null || head.next == null){
return head;
}
ListNode fast = head.next;
ListNode slow = head;
while (fast != null && fast.next != null){
fast = fast.next.next;
slow = slow.next;
}
ListNode tmp = slow.next;
slow.next = null;
ListNode left = sortList(head);
ListNode right = sortList(tmp);
ListNode h = new ListNode(0);
ListNode res = h;
while (left != null && right != null){
if (left.val < right.val){
h.next = left;
left = left.next;
}else {
h.next = right;
right = right.next;
}
h = h.next;
}
h.next = left != null ? left : right;
return res.next;
}
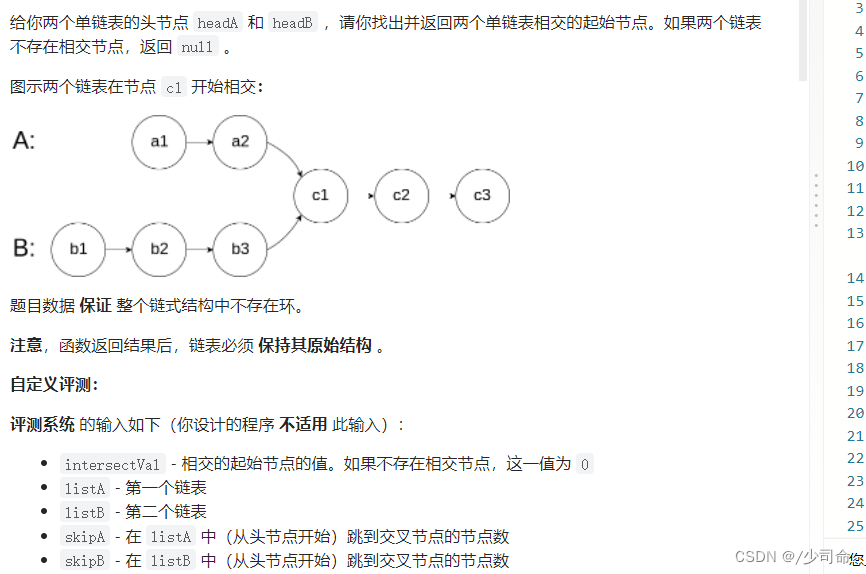
public ListNode getIntersectionNode(ListNode headA, ListNode headB) {
HashSet<ListNode> set = new HashSet<>();
ListNode tmp = headA;
while (tmp != null){
set.add(tmp);
tmp = tmp.next;
}
tmp = headB;
while (tmp != null){
if (set.contains(tmp)){
return tmp;
}
tmp = tmp.next;
}
return null;
}
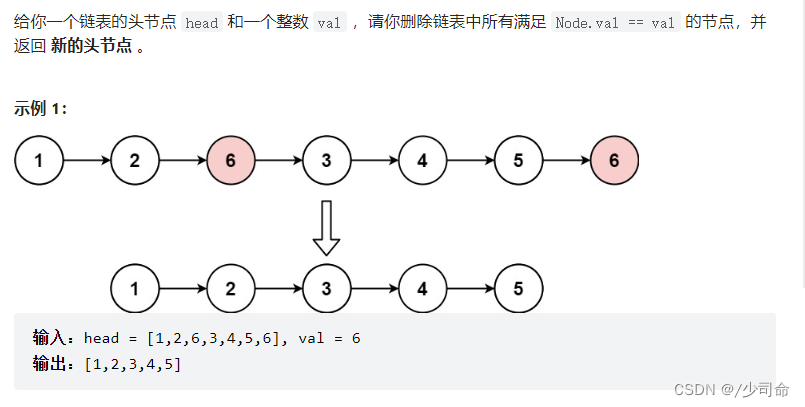
public ListNode removeElements(ListNode head, int val) {
if (head == null){
return head;
}
head.next = removeElements(head.next,val);
return head.val == val ? head.next : head;
}
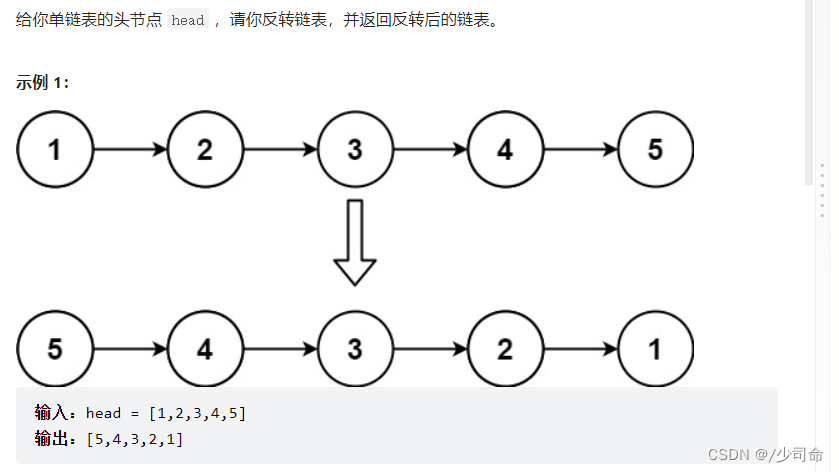
public ListNode reverseList(ListNode head) {
ListNode pre = null;
ListNode cur = head;
while (cur != null){
ListNode curNext = cur.next;
cur.next = pre;
pre = cur;
cur = curNext;
}
return pre;
}
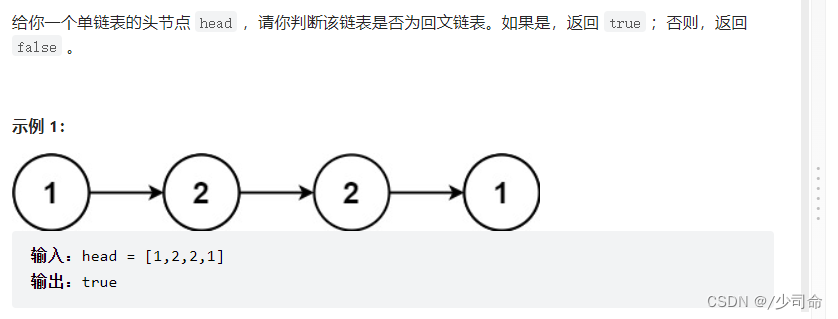
public boolean isPalindrome(ListNode head) {
ListNode slow,fast;
slow = fast = head;
while (fast != null && fast.next != null){
slow = slow.next;
fast = fast.next.next;
}
if (fast != null){
slow = slow.next;
}
ListNode left = head;
ListNode right = reverse(slow);
while (right != null){
if (left.val != right.val){
return false;
}
left = left.next;
right = right.next;
}
return true;
}
private ListNode reverse(ListNode head) {
ListNode pre = null;
ListNode cur = head;
while (cur != null){
ListNode curNext = cur.next;
cur.next = pre;
pre = cur;
cur = curNext;
}
return pre;
}
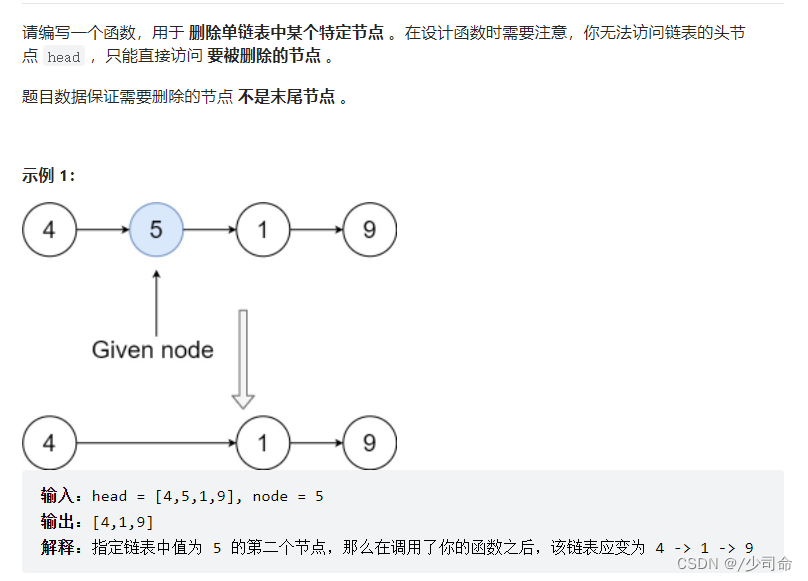
public void deleteNode(ListNode node) {
node.val = node.next.val;
node.next = node.next.next;
}
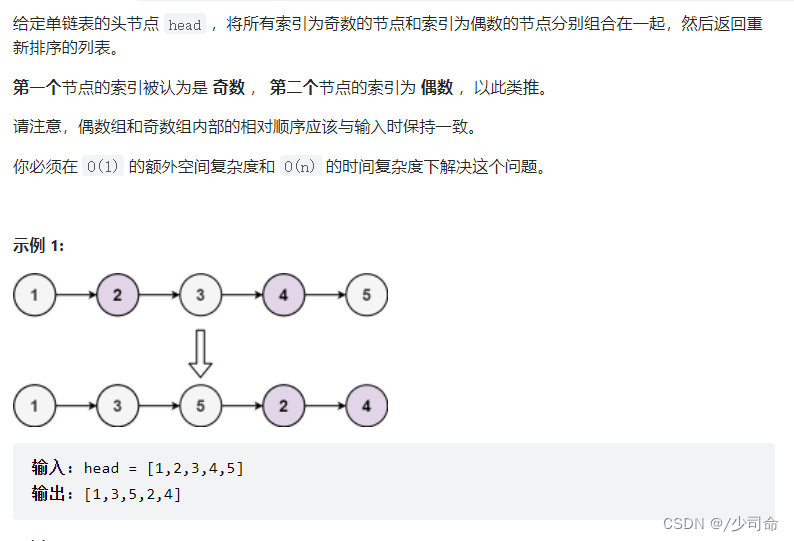
public ListNode oddEvenList(ListNode head) {
if (head == null) {
return null;
}
ListNode newHead = head.next;
ListNode odd = head;
ListNode even = head.next;
while (even != null && even.next != null){
odd.next = even.next;
odd = odd.next;
even.next = odd.next;
even = even.next;
}
odd.next = newHead;
return head;
}
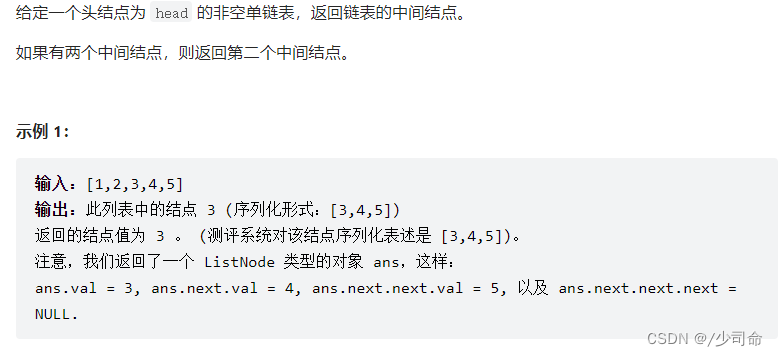
public ListNode middleNode(ListNode head) {
ListNode slow = head;
ListNode fast = head;
while (fast != null && fast.next != null){
slow = slow.next;
fast = fast.next.next;
}
return slow;
}