这段代码是一个用于处理JSON文件的脚本,主要包含以下几个函数和一个主程序:
cor_convert_simple(img_size, box): 这个函数用于将简单的坐标框格式转换为所需的格式。它接受图片尺寸img_size和一个坐标框box作为输入,并进行一系列的计算和转换,最后返回转换后的结果。具体的转换过程包括计算中心点坐标、宽度和高度,然后根据图片尺寸进行归一化处理。
cor_convert_seg(img_size, box_list): 这个函数用于将复杂的坐标框列表格式转换为所需的格式。它接受图片尺寸img_size和一个坐标框列表box_list作为输入,并进行一系列的计算和转换,最后返回转换后的结果。具体的转换过程和cor_convert_simple类似,但是需要处理多个坐标框。
rewrite_seg(from_path, to_path): 这个函数用于对JSON文件进行处理并将结果写入到一个新的文件中。它接受输入文件路径from_path和输出文件路径to_path作为参数。函数首先打开输入文件,并读取其中的JSON数据。然后根据指定的条件从JSON数据中提取特定的坐标框,并调用cor_convert_seg函数进行转换。最后,将转换后的结果写入到输出文件中。
主程序部分首先获取指定目录中的所有文件名,并遍历每个文件。对于每个文件,它提取文件名中的数字部分,构造输入文件路径和输出文件路径。然后调用rewrite_seg函数进行处理。
在代码中还使用了一些中文注释,主要是对代码的功能进行解释。例如:
# 计算归一化参数:用于解释计算归一化参数的过程。
# 将转换后的结果写入文件:用于解释将转换后的结果写入文件的操作。
import json
def cor_convert_simple(img_size, box):
# 计算归一化参数
dw = 1. / (img_size[0])
dh = 1. / (img_size[1])
# 计算中心点坐标、宽度和高度
x = (box[0] + box[2]) / 2.0
y = (box[1] + box[3]) / 2.0
w = box[2] - box[0]
h = box[3] - box[1]
# 归一化处理
x = x * dw
w = w * dw
y = y * dh
h = h * dh
temp_res = [x, y, w, h]
result = [str(x) for x in temp_res]
return result
def cor_convert_seg(img_size, box_list):
# 计算归一化参数
dw = 1. / (img_size[0])
dh = 1. / (img_size[1])
result = []
for box in box_list:
# 归一化处理并添加到结果列表
result.append(str(box[0] * dw))
result.append(str(box[1] * dh))
return result
def rewrite_seg(from_path, to_path):
label = ['class_1', 'class_2','class_3','class_4']
label_to_num = {lab: i for i, lab in enumerate(label)}
fw = open(to_path, "w", encoding='utf-8')
with open(from_path, "r", encoding='utf-8') as f:
origin_json = json.load(f)
img_size = [origin_json["imageWidth"], origin_json["imageHeight"]]
for reg_shape in origin_json["shapes"]:
reg_label = reg_shape["label"]
if reg_label == "screen_seg":
# 将转换后的结果写入文件
fw.write(
"{} {}\n".format(label_to_num["screen"], " ".join(cor_convert_seg(img_size, reg_shape["points"]))))
if __name__ == "__main__":
import os
filenames = os.listdir("./json/")
for fn in filenames:
num_str = fn.split('.')[0]
from_file = "./json/" + fn
to_file = "{}{}.txt".format("./gen_txt/", num_str)
rewrite_seg(from_file, to_file)
边界框的表达形式:
xyxy格式: 边界框由左上角坐标(x1,y1)和右下角坐标(x2,y2)表示
xywh格式: 边界框由中心坐标(x,y)和框的长宽(w,h)表示——YOLO中主要采用的是这种
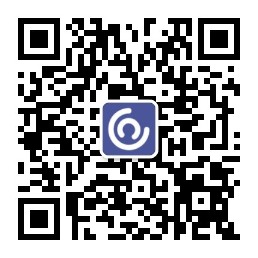
根据以上的标准,在进行转化之前,你需要知道你的json文件里面bbox存储的是哪种形式。
三、转换
1. bbox(x1,y1,x2,y2)的情况
size为图片的尺寸,一般json文件中可以获取,以list的形式储存,如[1920, 1080]
box为json里的边界框bbox,同样以list的形式表示
def cor_convert_simple(size,box):
dw = 1. / size[0]
dh = 1. / size[1]
x = (box[0] + box[2]) / 2.0
y = (box[1] + box[3]) / 2.0
w = box[2] - box[0]
h = box[3] - box[1]
x = x * dw
w = w * dw
y = y * dh
h = h * dh
return(x,y,w,h)
2. bbox(x,y,w,h)的情况
def cor_convert_simple(siez,box):
x, y, w, h = item['bbox']
dw = 1. / size[0]
dh = 1. / size[1]
x = x * dw
w = w * dw
y = y * dh
h = h * dh
return(x,y,w,h)