事务案例
1.AccountDao
2.AccountService
3.AccountTest
Account
package bull07.dao;
import java.sql.Connection;
import java.sql.SQLException;
import org.apache.commons.dbutils.QueryRunner;
public class AccountDao {
public void out(Connection conn ,String outUser, Double money) throws SQLException {
QueryRunner queryRunner = new QueryRunner();
String sql = "update account set money = money + ? where name = ?;";
Object[] param = {money,outUser};
queryRunner.update(conn,sql, param);
}
public void in(Connection conn ,String inUser, Double money) throws SQLException {
QueryRunner queryRunner = new QueryRunner();
String sql = "update account set money = money - ? where name = ?;";
Object[] param = {money,inUser};
queryRunner.update(conn,sql, param);
}
}
AccountService
package bull07.service;
import java.sql.Connection;
import java.sql.SQLException;
import org.apache.commons.dbutils.DbUtils;
import bull03.C3P0.C3P0Utils;
import bull07.dao.AccountDao;
public class AccountService {
public void transfer(String outUser,String inUser,Double money) {
Connection conn = null;
try {
conn = C3P0Utils.getConnection();
conn.setAutoCommit(false);
AccountDao accountDao = new AccountDao();
accountDao.out(conn,outUser,money);
accountDao.in(conn,inUser, money);
DbUtils.commitAndCloseQuietly(conn);
} catch (Exception e) {
DbUtils.rollbackAndCloseQuietly(conn);
throw new RuntimeException(e);
}
}
}
AccountTest
package bull07.test;
import java.sql.SQLException;
import bull07.service.AccountService;
public class AccountTest {
public static void main(String[] args) {
String outUser = "rose";
String inUser = "jack";
Double money = 200.0;
try {
AccountService accountService = new AccountService();
accountService.transfer(outUser, inUser, money);
System.out.println("转账成功!");
} catch (Exception e) {
System.out.println("转账失败!");
}
}
}
采用ThreadLocal实现
- 改写C3P0Utils工具类,将连接存到ThreadLocal中,同个线程通过工具类中获取相同连接,与此前相比不用传递Connection对象。
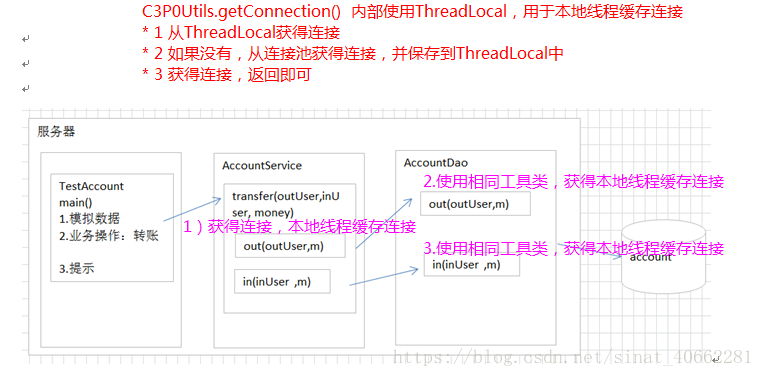
改写C3P0Utils
package bull08.ThreadLocal;
import java.sql.Connection;
import java.sql.SQLException;
import com.mchange.v2.c3p0.ComboPooledDataSource;
public class C3P0Utils {
private static ComboPooledDataSource dataSource = new ComboPooledDataSource("bull");
private static ThreadLocal<Connection> local = new ThreadLocal<Connection>();
public static Connection getConnection() {
Connection conn = local.get();
try {
if(conn == null) {
conn = dataSource.getConnection();
local.set(conn);
}
return conn;
} catch (Exception e) {
throw new RuntimeException(e);
}
}
}
AccountDao
package bull08.ThreadLocal;
import java.sql.SQLException;
import org.apache.commons.dbutils.QueryRunner;
public class AccountDao {
public void out(String outUser ,Double money) throws SQLException {
QueryRunner queryRunner = new QueryRunner();
String sql = "update account set money = money + ? where name = ?;";
Object[] params = {money,outUser};
queryRunner.update(C3P0Utils.getConnection(), sql, params);
}
public void in(String inUser ,Double money) throws SQLException {
QueryRunner queryRunner = new QueryRunner();
String sql = "update account set money = money - ? where name = ?;";
Object[] params = {money,inUser};
queryRunner.update(C3P0Utils.getConnection(), sql, params);
}
}
AccountService
package bull08.ThreadLocal;
import java.sql.Connection;
import org.apache.commons.dbutils.DbUtils;
public class AccountService {
public void transfer(String outUser ,String inUser ,Double money) {
Connection conn = null;
try {
conn = C3P0Utils.getConnection();
conn.setAutoCommit(false);
AccountDao accountDao = new AccountDao();
accountDao.out(outUser, money);
accountDao.in(inUser, money);
DbUtils.commitAndCloseQuietly(conn);
} catch (Exception e) {
DbUtils.rollbackAndCloseQuietly(conn);
throw new RuntimeException(e);
}
}
}
AccountTest
package bull08.ThreadLocal;
public class AccountTest {
public static void main(String[] args) {
String outUser = "jack";
String inUser = "rose";
Double money = 100.0;
try {
AccountService accountService = new AccountService();
accountService.transfer(outUser, inUser, money);
System.out.println("转账成功!");
} catch (Exception e) {
System.out.println("转账失败!");
}
}
}