GitHub - Endlessdaydream/Endless_Unity_Projects: Unity Projects of EndlessdaydramUnity Projects of Endlessdaydram. Contribute to Endlessdaydream/Endless_Unity_Projects development by creating an account on GitHub.
https://github.com/Endlessdaydream/Endless_Unity_Projects
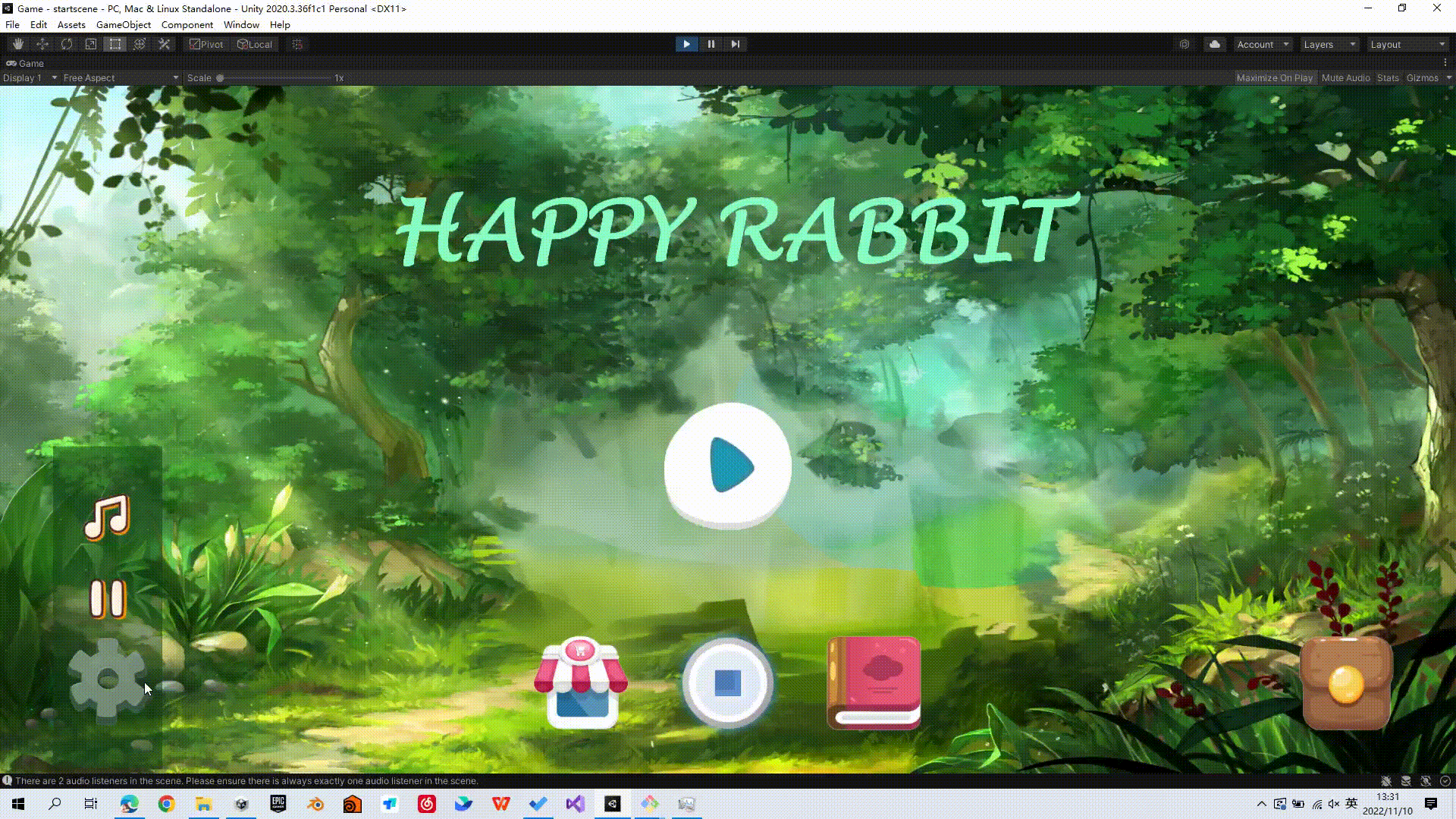
(37) How to make a 2D Bow and Arrow with UNITY & C#! - YouTube
https://www.youtube.com/watch?app=desktop&v=tNwLaGUJTK4
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class PlayerController : MonoBehaviour
{
PlayerCharacter player;
private void Start()
{
player = GetComponent<PlayerCharacter>();
}
private void Update()
{
if (Input.GetKeyDown(KeyCode.Tab))
player.TrySwitch();
if (Input.GetMouseButton(1))
player.TryBreak();
if (Input.GetMouseButton(0))
player.PrepareShoot();
if (Input.GetMouseButtonUp(0))
player.Shoot();
player.Move(Input.GetAxisRaw("Horizontal"));
if (Input.GetButtonDown("Jump"))
player.Jump();
player.updateAnim();
}
}
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class PlayerCharacter : MonoBehaviour
{
public int hp = 1;
public float speed = 4;
public float jumpSpeed = 14;
public float minShootSpeed = 5f, maxShootSpeed = 24f;
public Transform shootPoint;
public Transform flagPoint;
public List<Transform> arrowsPrefab;
public float maxStoreTime = 2f;
int arrowId = 0;
int curArrowId = -1;
Transform curArrow;
float shootSpeed;
float curStoreTime = 0;
Transform[] points;
Rigidbody2D r;
Animator anim;
bool isGround = false;
bool isAim = false;
bool isStopShoot = false;
Transform foot1;
Transform foot2;
Transform center;
LineRenderer line;
SpriteRenderer render;
bool cuphit = false;
Transform gameOverPanel;
Transform completePanel;
public static PlayerCharacter Instance { get; private set; }
private void Awake()
{
Instance = this;
}
private void Start()
{
render = GetComponent<SpriteRenderer>();
r = GetComponent<Rigidbody2D>();
anim = GetComponent<Animator>();
points = new Transform[30];
for(int i=0;i<points.Length;i++)
{
points[i] = Instantiate(flagPoint, transform);
var render = points[i].GetComponent<SpriteRenderer>();
var temp = render.color;
temp.a *= (float)(points.Length - i) / points.Length;
render.color = temp;
}
ShowPoints(false);
foot1 = transform.Find("foot1");
foot2 = transform.Find("foot2");
center = transform.Find("center");
line = GetComponent<LineRenderer>();
GameObject canvas = GameObject.Find("Canvas");
gameOverPanel = canvas.transform.Find("GameOverPanel");
gameOverPanel.gameObject.SetActive(false);
completePanel = canvas.transform.Find("CompletePanel");
completePanel.gameObject.SetActive(false);
}
private void FixedUpdate()
{
isAim = false;
isGround = false;
LayerMask layerMask = ~(1 << 7);
if (Physics2D.Raycast(foot1.position, Vector2.down, 0.25f, layerMask) || Physics2D.Raycast(foot2.position, Vector2.down, 0.25f, layerMask))
{
isGround = true;
}
line.enabled = false;
if (curArrowId == 1 && curArrow)
{
isStopShoot = true;
line.SetPosition(0, center.position);
line.SetPosition(1, curArrow.position);
line.enabled = true;
var dir = (curArrow.position - center.position).normalized;
Transform linkObj = curArrow.parent;
if (linkObj != null)
{
if(linkObj.GetComponent<Rigidbody2D>())
{
Rigidbody2D otherR = linkObj.GetComponent<Rigidbody2D>();
otherR.AddForce(-dir * 20f);
}
else
{
r.AddForce(dir * 65f);
}
}
}
}
public void PrepareShoot()
{
if (isStopShoot) return;
curArrowId = arrowId;
curArrow = null;
curStoreTime += Time.deltaTime;
shootSpeed = minShootSpeed + (maxShootSpeed - minShootSpeed) * (Mathf.Min(curStoreTime, maxStoreTime) / maxStoreTime);
isAim = true;
if (isGround) r.velocity = new Vector2(0, r.velocity.y);
DrawDir();
}
public void Shoot()
{
if (isStopShoot) return;
curStoreTime = 0;
ShowPoints(false);
curArrow = Instantiate(arrowsPrefab[curArrowId], shootPoint.position, shootPoint.rotation);
curArrow.GetComponent<Rigidbody2D>().velocity = shootSpeed * curArrow.right;
}
public void TryBreak()
{
if (isStopShoot)
{
isStopShoot = false;
Destroy(curArrow.gameObject);
curArrow = null;
}
}
public void TrySwitch()
{
if (isStopShoot) return;
arrowId ^= 1;
}
void DrawDir()
{
ShowPoints(true);
float time = 0.5f;
float deltaTime = time / points.Length;
float t = 0;
Vector2 v0 = shootPoint.right * shootSpeed;
Vector2 start = shootPoint.position;
for (int i = 0; i < points.Length; i++)
{
t += deltaTime;
points[i].position = start + v0 * t + 0.5f * Physics2D.gravity * arrowsPrefab[curArrowId].GetComponent<Rigidbody2D>().gravityScale * t * t;
}
}
void ShowPoints(bool isShow)
{
for (int i = 0; i < points.Length; i++)
points[i].gameObject.SetActive(isShow);
}
public void Move(float h)
{
if (Mathf.Abs(h) > 0.1f)
transform.right = h < 0 ? Vector2.left : Vector2.right;
if (isAim) return;
if(isGround)
{
r.velocity = new Vector2(h * speed, r.velocity.y);
}
else
{
r.AddForce(new Vector2(h * 30 * Time.deltaTime / Time.fixedDeltaTime, 0));
r.velocity = new Vector2(Mathf.Clamp(r.velocity.x, -speed, speed), r.velocity.y);
}
}
public void Jump()
{
if (!isGround||isAim) return;
r.velocity = new Vector2(r.velocity.x, jumpSpeed);
}
public void updateAnim()
{
anim.SetBool("isWalk", Mathf.Abs(r.velocity.x) > 0.01f);
anim.SetBool("isGround", isGround);
anim.SetBool("isFall", r.velocity.y < 0.01f);
anim.SetBool("isAim", isAim);
}
public void beHurt()
{
if (--hp <= 0)
{
Destroy(gameObject);
gameOverPanel.gameObject.SetActive(true);
}
else
StartCoroutine(TempTurnRed());
}
public void Win()
{
completePanel.gameObject.SetActive(true);
}
IEnumerator TempTurnRed()
{
render.color = Color.red;
yield return new WaitForSeconds(0.1f);
render.color = Color.white;
}
}
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class Bow : MonoBehaviour
{
private void LateUpdate()
{
Vector2 dir = Camera.main.ScreenToWorldPoint(Input.mousePosition) - transform.position;
transform.right = dir;
}
}
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class ArrowBase : MonoBehaviour
{
protected Rigidbody2D r;
public Transform hitObj = null;
private void Start()
{
r = GetComponent<Rigidbody2D>();
}
private void OnTriggerEnter2D(Collider2D collision)
{
if (transform.parent) return;
r.velocity = Vector2.zero;
r.isKinematic = true;
transform.parent = collision.transform;
}
private void Update()
{
if (transform.parent) return;
float angle = Mathf.Atan2(r.velocity.y, r.velocity.x) * Mathf.Rad2Deg;
transform.rotation = Quaternion.Euler(0, 0, angle);
}
}
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class Bee : MonsterBase
{
public Rigidbody2D bulletPrefab;
Transform shootPoint;
Rect range;
bool isOpenAttack = false;
private new void Start()
{
base.Start();
var bound = transform.parent.Find("Bound");
shootPoint = transform.Find("ShootPoint");
range.size = bound.localScale;
range.center = bound.position;
StartCoroutine(RandomMove(RandomTarget()));
}
private new void Update()
{
base.Update();
if(isVigilant&&!isOpenAttack&&!isDead)
{
isOpenAttack = true;
StartCoroutine(AutoAttack());
}
}
IEnumerator RandomMove(Vector2 target)
{
while(this)
{
while (Vector2.Distance(transform.position, target) > 0.1f && !isDead)
{
if (isVigilant && player)
{
transform.right = player.position.x < transform.position.x ? Vector2.right : Vector2.left;
}
else
{
transform.right = target.x < transform.position.x ? Vector2.right : Vector2.left;
}
transform.position = Vector2.MoveTowards(transform.position, target, 3f * Time.deltaTime);
yield return null;
}
yield return new WaitForSeconds(1f);
target = RandomTarget();
}
}
IEnumerator AutoAttack()
{
while(this&&player&&!isDead)
{
var r = Instantiate(bulletPrefab, shootPoint.position, Quaternion.identity);
r.velocity = (player.position - r.transform.position).normalized * 2f;
yield return new WaitForSeconds(3f);
}
}
Vector2 RandomTarget()
{
return new Vector2(Random.Range(range.xMin, range.xMax), Random.Range(range.yMin, range.yMax));
}
}
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class Flower : MonsterBase
{
bool stopMove = false;
Rigidbody2D r;
private void FixedUpdate()
{
LayerMask layerMask = 1 << 7;
if (Physics2D.Raycast(transform.position-new Vector3(0,0.3f), transform.right, 0.7f, layerMask))
{
anim.SetTrigger("attack");
stopMove = true;
r.velocity = Vector2.zero;
}
}
public void Attack()
{
LayerMask layerMask = 1 << 7;
var hitInfo = Physics2D.Raycast(transform.position - new Vector3(0, 0.3f), transform.right, 0.8f, layerMask);
if (hitInfo)
{
hitInfo.transform.GetComponent<PlayerCharacter>().beHurt();
}
stopMove = false;
}
private new void Start()
{
base.Start();
r = GetComponent<Rigidbody2D>();
}
private new void Update()
{
base.Update();
if (!isDead && !stopMove && isVigilant && player)
{
bool isLeft = player.position.x < transform.position.x;
transform.right = isLeft ? Vector2.left : Vector2.right;
r.velocity = new Vector2(isLeft ? -2 : 2, r.velocity.y);
}
}
}
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class MonsterBase : MonoBehaviour
{
public int hp = 1;
SpriteRenderer render;
protected Animator anim;
protected Transform player;
protected bool isDead = false, isVigilant = false;
public void Start()
{
render = GetComponent<SpriteRenderer>();
anim = GetComponent<Animator>();
player = GameObject.FindWithTag("Player").transform;
}
public void Update()
{
if (player&&Vector2.Distance(player.position, transform.position) < 5f)
isVigilant = true;
}
private void OnTriggerEnter2D(Collider2D collision)
{
if (isDead) return;
if (collision.gameObject.layer == 8)
beHurt();
}
public void beHurt()
{
isVigilant = true;
if(--hp<=0)
{
isDead = true;
var r = GetComponent<Rigidbody2D>();
r.constraints = RigidbodyConstraints2D.None;
r.isKinematic = false;
render.color = new Color(0.5f, 0.5f, 0.5f, 1);
bool isLeft = transform.position.x - player.position.x >= 0;
r.AddForce(new Vector2(isLeft ? 1 : -1, 1) * 200f);
//r.angularVelocity = (isLeft ? -1 : 1) * 100f;
anim.SetTrigger("die");
if(transform.parent)
{
Destroy(transform.root.gameObject, 2f);
}
else
{
Destroy(gameObject, 2f);
}
}
if(!isDead)
StartCoroutine(TempTurnRed());
}
IEnumerator TempTurnRed()
{
render.color = Color.red;
yield return new WaitForSeconds(0.1f);
render.color = Color.white;
}
}
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class Cup : MonoBehaviour
{
void Start()
{
}
private void OnTriggerEnter2D(Collider2D collision)
{
if (collision.gameObject.layer == 8)
{
//Destroy(collision.gameObject);
PlayerCharacter.Instance.Win();
}
}
// Update is called once per frame
void Update()
{
}
}
(37) How to make an AWESOME fully INTERACTIVE Game Menu in UNITY - Everything you need to know. - YouTube
https://www.youtube.com/watch?v=pGxI8_GYfUY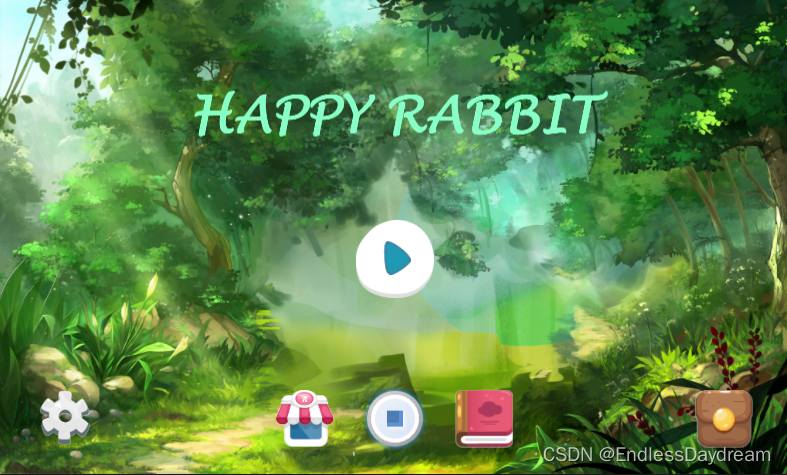
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class uisettingscript : MonoBehaviour
{
public GameObject Panel;
public void Setting()
{
Panel.GetComponent<Animator>().SetTrigger("Pop");
}
public void OpenSite()
{
Application.OpenURL("https://assetstore.unity.com/");
}
}
//======================================================================================
//==description:音频管理器
//==state:播放音频、暂停、暂停继续播放、停止播放、切换音频、音频回调播放器、延时音频播放器、生成2D音效、3D音效、指定音效播放
//======================================================================================
using System;
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public delegate void AudioCallBack();
[RequireComponent(typeof(AudioSource))]
public class Audio : MonoBehaviour
{
public class ClipData
{
public AudioClip audiodata(string name)
{
return Resources.Load<AudioClip>("Audios/" + name);
}
}
public static Audio Instance;
ClipData clipdata = new ClipData();
public AudioSource _audioSource;
void Awake()
{
Instance = this;
}
void Start()
{
_audioSource = GetComponent<AudioSource>();
}
/// <summary>
/// 播放音频 Resources/Audios/name
/// </summary>
/// <param name="name"></param>
public void AudioPlay(string name)
{
_audioSource.clip = clipdata.audiodata(name);
_audioSource.Play();
}
/// <summary>
/// 暂停播放
/// </summary>
public void AudioPause()
{
_audioSource.Pause();
}
/// <summary>
/// 暂停播放后继续播放
/// </summary>
public void AudioUnPause()
{
_audioSource.UnPause();
}
/// <summary>
/// 停止播放
/// </summary>
public void AudioStop()
{
_audioSource.Stop();
}
/// <summary>
/// 切换音频 Resources/Audios/name
/// </summary>
/// <param name="name"></param>
public void AudioSwitch(string name)
{
AudioClip _clip = clipdata.audiodata(name);
if (_audioSource.isPlaying)
{
_audioSource.Stop();
}
_audioSource.clip = _clip;
_audioSource.Play();
}
/// <summary>
/// 音频回调播放器 Resources/Audios/name callback=>音频播完后执行的方法。
/// </summary>
/// <param name="name"></param>
/// <param name="callback"></param>
public void AudioPlayer(string name, AudioCallBack callback)
{
_audioSource.clip = clipdata.audiodata(name);
_audioSource.Play();
StartCoroutine(AudioDelayedCallBack(_audioSource.clip.length, callback));
}
/// <summary>
/// 音频回调播放器 Resources/Audios/name callback=>音频播完后执行的方法。
/// </summary>
/// <param name="name"></param>
/// <param name="callback"></param>
public void AudioPlayer(string name, AudioCallBack callback, float time)
{
_audioSource.clip = clipdata.audiodata(name);
_audioSource.Play();
StartCoroutine(AudioDelayedCallBack(_audioSource.clip.length + time, callback));
}
//音频延迟回调
IEnumerator AudioDelayedCallBack(float time, AudioCallBack callback)
{
yield return new WaitForSeconds(time);
callback();
}
/// <summary>
/// 延时播放音频 Resources/Audios/name time=>延时时间
/// </summary>
/// <param name="name"></param>
/// <param name="time"></param>
public void AudioDelayPlay(string name, float time)
{
_audioSource.clip = clipdata.audiodata(name);
Invoke("AudioDelayTime", time);
}
public void AudioDelayTime() { _audioSource.Play(); }
/// <summary>
/// 生成2D音效 Resources/Audios/name 播放完毕消失
/// </summary>
/// <param name="name"></param>
public void AudioInstantiate(string name)
{
GameObject obj = new GameObject();
AudioSource _audio = obj.AddComponent<AudioSource>();
_audio.name = "AudioSource";
_audio.playOnAwake = true;
_audio.clip = clipdata.audiodata(name);
_audio.Play();
StartCoroutine(AudioFinish(_audio.clip.length, obj));
}
//音效结束销毁AudioGameObject
IEnumerator AudioFinish(float time, GameObject obj)
{
yield return new WaitForSeconds(time);
DestroyImmediate(obj);
}
/// <summary>
/// 3D音效 (只播放一次) Resources/Audios/name CameraPos:摄像机位置
/// </summary>
/// <param name="name"></param>
/// <param name="CameraPos"></param>
public void AudioAtPoint(string name, Vector3 CameraPos)
{
AudioSource.PlayClipAtPoint(clipdata.audiodata(name), CameraPos, 1.0f);
}
/// <summary>
/// 指定AudioSource播放音频
/// </summary>
/// <param name="obj"></param>
/// <param name="name"></param>
public void AudioSourceOther(GameObject obj, string name)
{
AudioSource audioSource = obj.GetComponent<AudioSource>();
audioSource.clip = clipdata.audiodata(name);
audioSource.Play();
}
public void CloseAudio()
{
StopAllCoroutines();
_audioSource.clip = null;
}
}
场景切换
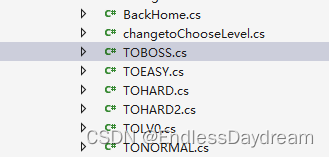
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.SceneManagement;
public class TOBOSS : MonoBehaviour
{
public void OnBtnStart()
{
SceneManager.LoadScene("BOSS");
}
}
DontDestroyOnLoad
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class BackgroundMusic : MonoBehaviour
{
static BackgroundMusic S;
private void Awake()
{
if (S == null)
{
S = this;
}
else if(S != this)
{
Destroy(gameObject);
}
DontDestroyOnLoad(gameObject);
}
}