一.
第一个想法是将数字转换为字符串,并检查字符串是否为回文。但是,这需要额外的非常量空间
具体实现:
(1)暴力解法:
class solution:
def isPalindrome(self,x:int)->bool:
return(str(x)==str(x)[::-1])#相当于反序
(1.1)C++解法
#include<iostream>
#include<string>
#include<algorithm>
using namespace std;
int main() {
string a;
cin >> a;
string b = a;
reverse(b.begin(), b.end());//反转字符串
//reverse()包含在algorithm函数中,.begin()和.end()用于取始末
if (a == b) cout << a << "是回文串" << endl;
else cout << a << "不是回文串" << endl;
return 0;
}
(2)二分法解法
class slution:
def isPalindrome(self,x:int)->bool:
s=str(x)
l=len(s)
h=l/2
return s[:h]==s[-1:-h-1:-1]#[起始:末尾:步长]
二.
第二种办法就是取模
(1)反转一半数字
public class solution
{
if(x<0||(x%10==0&&x!=0))
{
return false;
}
int revertNumber=0;
while(x>revertNumber)
{
revertNumber=revertNumber*10+x%10;//写的太妙了!!
x/=10;
}
return x==revertNumber||x==revertNumber/10;//相当于判断这俩条件,成立就true,不成立就false
三.
用指针
扫描二维码关注公众号,回复:
14747430 查看本文章
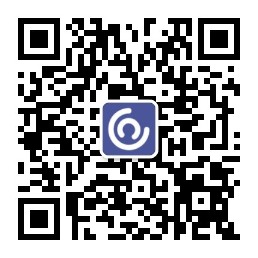
(1)(这是双指针)
#include<iostream>
#include<string>
#include<algorithm>
using namespace std;
int main(){
string a;
cin>>a;
string b=a;
int l=0,r=b.length()-1;
while(l<r){
swap(b[l],b[r]);
l++;r--;
}
if (a == b) cout << a << "是回文串" << endl;
else cout << a << "不是回文串" << endl;
return 0;
}
(2)剩下的进阶方法暂时不太懂,先挖个坑