A complete binary tree is a binary tree in which every level, except possibly the last, is completely filled, and all nodes are as far left as possible.
Design an algorithm to insert a new node to a complete binary tree keeping it complete after the insertion.
Implement the CBTInserter
class:
CBTInserter(TreeNode root)
Initializes the data structure with theroot
of the complete binary tree.int insert(int v)
Inserts aTreeNode
into the tree with valueNode.val == val
so that the tree remains complete, and returns the value of the parent of the insertedTreeNode
.TreeNode get_root()
Returns the root node of the tree.
Example 1:
Input ["CBTInserter", "insert", "insert", "get_root"] [[[1, 2]], [3], [4], []] Output [null, 1, 2, [1, 2, 3, 4]] Explanation CBTInserter cBTInserter = new CBTInserter([1, 2]); cBTInserter.insert(3); // return 1 cBTInserter.insert(4); // return 2 cBTInserter.get_root(); // return [1, 2, 3, 4]
Constraints:
- The number of nodes in the tree will be in the range
[1, 1000]
. 0 <= Node.val <= 5000
root
is a complete binary tree.0 <= val <= 5000
- At most
104
calls will be made toinsert
andget_root
.
题目:实现一个类,可以往二叉树中插入节点,使得二叉树保持完全二叉树
思路:容易错的点是初始化时不只是一个根节点,可能已经是一棵树。用queue来保存所有不完全的子节点。代码
扫描二维码关注公众号,回复:
14661728 查看本文章
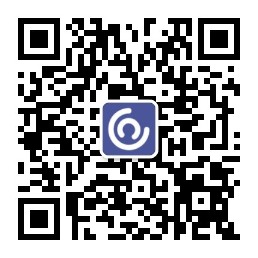
/**
* Definition for a binary tree node.
* struct TreeNode {
* int val;
* TreeNode *left;
* TreeNode *right;
* TreeNode() : val(0), left(nullptr), right(nullptr) {}
* TreeNode(int x) : val(x), left(nullptr), right(nullptr) {}
* TreeNode(int x, TreeNode *left, TreeNode *right) : val(x), left(left), right(right) {}
* };
*/
class CBTInserter {
private:
queue<TreeNode*> q;
TreeNode* rootNode;
public:
CBTInserter(TreeNode* root) {
rootNode = root;
q.push(root);
while(!q.empty()){
if(q.front()->left) q.push(q.front()->left);
if(q.front()->right) q.push(q.front()->right);
if(q.front()->left && q.front()->right)
q.pop();
else break;
}
}
int insert(int val) {
TreeNode* node = new TreeNode(val);
int res = q.front()->val;
if(!q.front()->left){
q.front()->left = node;
} else {
q.front()->right = node;
q.pop();
}
q.push(node);
return res;
}
TreeNode* get_root() {
return rootNode;
}
};
/**
* Your CBTInserter object will be instantiated and called as such:
* CBTInserter* obj = new CBTInserter(root);
* int param_1 = obj->insert(val);
* TreeNode* param_2 = obj->get_root();
*/