Mobilenetv2 中使用的的激活函数是ReLU6,采用InvertedResidual残差网络,其特征表达能力优于传统的残差网络,总共计算有10次残差计算。
其中,网络中把普通的卷积分为depth-wise的卷积核point-wise的卷积可以大大的减少计算量。
1.ReLU6
ReLU6(x) =0 x < 0
ReLU6(x) =x 6>x>0
ReLU6(x) =6 x>6
图像如下:
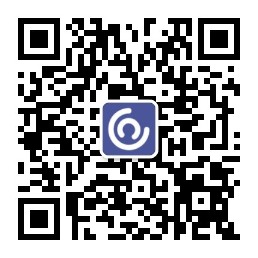
其核心如下图: 开头将深度变到32,结尾将深度变到1280 ,中间进行残差卷积(InvertedResidual)
图中的 t :代表 膨胀率,为 1 时不膨胀,不为1时进行膨胀
c : 代表卷积维度 ,n:代表 这一层有多少个 残差结构 ,s:代表步长
网络结构之特征提取(features)
(0)首先进行一个 3x32 的三维卷积 ,步长为(1,2,2),对时间维度不进行缩减
(features): Sequential(
(0): Sequential(
(0): Conv3d(3, 32, kernel_size=(3, 3, 3), stride=(1, 2, 2), padding=(1, 1, 1), bias=False)
(1): BatchNorm3d(32, eps=1e-05, momentum=0.1, affine=True, track_running_stats=True)
(2): ReLU6(inplace=True)
)
(1)其实是InvertedResidual残差:共有17个 这种残差机构。
(a)图为传统的残差结构 ,先用1x1卷积将输入的feature map的维度降低,然后进行3x3的卷积操作,最后再用1x1的卷积将维度变大。
(b)图为InvertedResidual结构,先用1x1卷积将输入的feature map维度变大,然后用3x3 depthwise convolution方式做卷积运算,最后使用1x1的卷积运算将其维度缩小。注意,此时的1x1卷积运算后,不再使用ReLU激活函数,而是使用线性激活函数,以保留更多特征信息,保证模型的表达能力。
MobilenetV2 也不是所有结构都使用这种残差网络,只有满足 ,步长为1,且卷积输入,输出的维度相同,使用 InvertedResidual 残差网络,共有10次
self.use_res_connect = self.stride == (1,1,1) and inp == oup
2.其整体网络结构
3.整体代码
'''MobilenetV2 in PyTorch.
See the paper "MobileNetV2: Inverted Residuals and Linear Bottlenecks" for more details.
'''
import torch
import math
import torch.nn as nn
import torch.nn.functional as F
from torch.autograd import Variable
def conv_bn(inp, oup, stride):
return nn.Sequential(
nn.Conv3d(inp, oup, kernel_size=3, stride=stride, padding=(1,1,1), bias=False),
nn.BatchNorm3d(oup),
nn.ReLU6(inplace=True)
)
def conv_1x1x1_bn(inp, oup):
return nn.Sequential(
nn.Conv3d(inp, oup, 1, 1, 0, bias=False),
nn.BatchNorm3d(oup),
nn.ReLU6(inplace=True)
)
class InvertedResidual(nn.Module):
def __init__(self, inp, oup, stride, expand_ratio):
super(InvertedResidual, self).__init__()
self.stride = stride
hidden_dim = round(inp * expand_ratio)
self.use_res_connect = self.stride == (1,1,1) and inp == oup
if expand_ratio == 1:
self.conv = nn.Sequential(
# dw
nn.Conv3d(hidden_dim, hidden_dim, 3, stride, 1, groups=hidden_dim, bias=False),
nn.BatchNorm3d(hidden_dim),
nn.ReLU6(inplace=True),
# pw-linear
nn.Conv3d(hidden_dim, oup, 1, 1, 0, bias=False),
nn.BatchNorm3d(oup),
)
else:
self.conv = nn.Sequential(
# pw
nn.Conv3d(inp, hidden_dim, 1, 1, 0, bias=False),
nn.BatchNorm3d(hidden_dim),
nn.ReLU6(inplace=True),
# dw
nn.Conv3d(hidden_dim, hidden_dim, 3, stride, 1, groups=hidden_dim, bias=False),
nn.BatchNorm3d(hidden_dim),
nn.ReLU6(inplace=True),
# pw-linear
nn.Conv3d(hidden_dim, oup, 1, 1, 0, bias=False),
nn.BatchNorm3d(oup),
)
def forward(self, x):
if self.use_res_connect: # 步长为1,且卷积维度相同
#print('c') #出现19次
return x + self.conv(x)
else:
return self.conv(x)
class MobileNetV2(nn.Module):
def __init__(self, num_classes=3, sample_size=224, width_mult=1.):
super(MobileNetV2, self).__init__()
block = InvertedResidual
input_channel = 32
last_channel = 1280
interverted_residual_setting = [
# t, c, n, s
[1, 16, 1, (1,1,1)],
[6, 24, 2, (2,2,2)],
[6, 32, 3, (2,2,2)],
[6, 64, 4, (2,2,2)],
[6, 96, 3, (1,1,1)],
[6, 160, 3, (2,2,2)],
[6, 320, 1, (1,1,1)],
]
# building first layer
assert sample_size % 16 == 0.
input_channel = int(input_channel * width_mult)
self.last_channel = int(last_channel * width_mult) if width_mult > 1.0 else last_channel
self.features = [conv_bn(3, input_channel, (1,2,2))]
# building inverted residual blocks
for t, c, n, s in interverted_residual_setting: #[1, 16, 1, (1,1,1)],
output_channel = int(c * width_mult) #[6, 24, 2, (2,2,2)],
for i in range(n):
stride = s if i == 0 else (1,1,1)
self.features.append(block(input_channel, output_channel, stride, expand_ratio=t))
input_channel = output_channel
# building last several layers
self.features.append(conv_1x1x1_bn(input_channel, self.last_channel))
# make it nn.Sequential
self.features = nn.Sequential(*self.features)
# building classifier
self.classifier = nn.Sequential(
nn.Dropout(0.2),
nn.Linear(self.last_channel, num_classes),
)
self._initialize_weights()
def forward(self, x):
#print(x.shape)
x = self.features(x)
#print(x.shape)
x = F.avg_pool3d(x, x.data.size()[-3:])
#print(x.shape)
x = x.view(x.size(0), -1)
#print(x.shape)
x = self.classifier(x)
return x
def _initialize_weights(self):
for m in self.modules():
if isinstance(m, nn.Conv3d):
n = m.kernel_size[0] * m.kernel_size[1] * m.kernel_size[2] * m.out_channels
m.weight.data.normal_(0, math.sqrt(2. / n))
if m.bias is not None:
m.bias.data.zero_()
elif isinstance(m, nn.BatchNorm3d):
m.weight.data.fill_(1)
m.bias.data.zero_()
elif isinstance(m, nn.Linear):
n = m.weight.size(1)
m.weight.data.normal_(0, 0.01)
m.bias.data.zero_()
def get_fine_tuning_parameters(model, ft_portion):
if ft_portion == "complete":
return model.parameters()
elif ft_portion == "last_layer":
ft_module_names = []
ft_module_names.append('classifier')
parameters = []
for k, v in model.named_parameters():
for ft_module in ft_module_names:
if ft_module in k:
parameters.append({'params': v})
break
else:
parameters.append({'params': v, 'lr': 0.0})
return parameters
else:
raise ValueError("Unsupported ft_portion: 'complete' or 'last_layer' expected")
def get_model(**kwargs):
"""
Returns the model.
"""
model = MobileNetV2(**kwargs)
return model
if __name__ == "__main__":
model = get_model(num_classes=3, sample_size=112, width_mult=1.)
model = model.cuda()
model = nn.DataParallel(model, device_ids=None)
print(model)
input_var = Variable(torch.randn(8, 3, 16, 112, 112))
output = model(input_var)
print(output.shape)