数组模拟环形队列:
使用数组模拟环形队列的思路分析:
思路:
1、front变量的含义做一个调整:front就指向队列的第一元素,也就是说arr[front]就是队列的第一个元素。
front的初始值=0;
2、rear变量的含义做一个调整:rear指向队列的最后一个元素的最后一个位置。(希望空出一个空间作为约定)
rear的初始值=0;
3、当队列满时,条件是
(rear+1) % maxSize == front【满】
4、对队列为空的条件,
rear==front【空】
5、队列中有效的数据的个数
(rear+maxSize-front)%maxSize //rear = 1 front=0
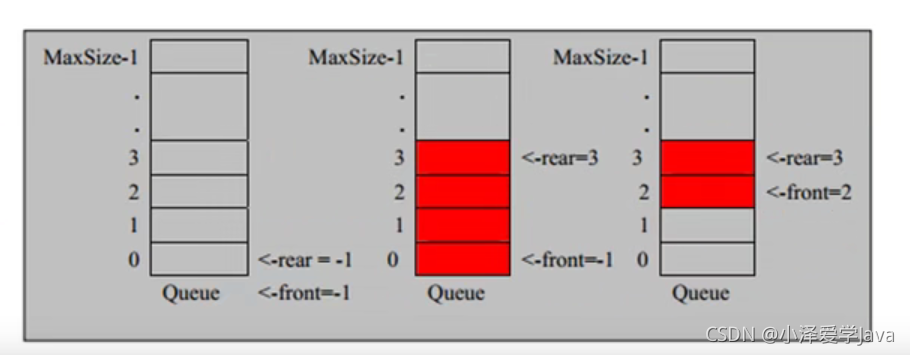
代码实现:
package com.huaze.sparsearray;
import java.util.Scanner;
public class CircleArrayQueue {
public static void main(String[] args) {
System.out.println("测试数组模拟环形队列的数据");
CircleArray queue = new CircleArray(4);//其队列的有效数据最大是3
char key = ' '; //接收用户输入
Scanner scanner = new Scanner(System.in);
boolean loop = true;
//输出一个菜单
while (loop){
System.out.println("s(show):显示队列");
System.out.println("e(exit):退出程序");
System.out.println("a(add):添加数据到队列");
System.out.println("g(get):从队列里取出数据");
System.out.println("h(head):查看队列头的数据");
key = scanner.next().charAt(0);//接收一个字符
switch (key){
case 's':
queue.showQueue();
break;
case 'a':
System.out.println("输入一个数字:");
int value = scanner.nextInt();
queue.addQueue(value);
break;
case 'g':
try {
int res = queue.getQueue();
System.out.printf("取出的数据是%d\n",res);
}catch (Exception e){
//TODO: handle exception
System.out.println(e.getMessage());
}
break;
case 'h': //查看队列头的数据
try {
int res = queue.headQueue();
System.out.printf("队列头的数据是%d\n",res);
}catch (Exception e){
//TODO: handle exception
System.out.println(e.getMessage());
}
break;
case 'e':
scanner.close();
loop = false;
break;
default:
break;
}
}
System.out.println("程序退出!");
}
}
class CircleArray {
private int maxSize; //表示数组的最大容量
//front变量的含义做一个调整:front就指向队列的第一元素,也就是说arr[front]就是队列的第一个元素。
// front的初始值=0;
private int front;
//rear变量的含义做一个调整:rear指向队列的最后一个元素的最后一个位置。(希望空出一个空间作为约定)
// rear的初始值=0;
private int rear;
private int[] arr; //该数组用于存放数据
//创建队列的构造器
public CircleArray(int arrMaxSize){
maxSize = arrMaxSize;
arr = new int[maxSize];
front = 0;
rear = 0;
}
//当队列满时,条件是(rear+1) % maxSize == front【满】
public boolean isFull(){
return (rear + 1) % maxSize == front;
}
//判断队列是否为空
public boolean isEmpty(){
return rear == front;
}
//添加数据到队列
public void addQueue(int n){
//判断队列是否满
if(isFull()){
System.out.println("队列满,不能加入数据。");
return;
}
//直接将数据加入
arr[rear] = n;
//将rear后移,这里必须考虑取模
rear = (rear + 1) % maxSize;
}
//获取队列数据,出队列
public int getQueue(){
//判断队列是否为空
if(isEmpty()){
//通过抛出异常来处理
throw new RuntimeException("队列空,不能取数据。");
}
// 这里需要分析出front 是指向队列的第一个元素
//先把front对应的值保存到一个临时变量
//将front后移,考虑取模
//将临时保存的变量返回
int value = arr[front];
front = (front + 1) % maxSize;
return value;
}
//显示队列的所有数据
public void showQueue(){
//遍历
if (isEmpty()){
System.out.println("队列是空,没有数据。");
return;
}
//从front开始遍历,遍历多少个元素
//
for (int i = front; i < front + size(); i++) {
System.out.printf("arr[%d]=%d\n",i % maxSize,arr[i % maxSize]);
}
}
//求出当前队列有效数据的个数
public int size () {
return (rear + maxSize - front) % maxSize;
}
//显示队列的头部数据,这里不是取出数据
public int headQueue(){
if (isEmpty()){
throw new RuntimeException("队列空,没有数据");
}
return arr[front];
}
}