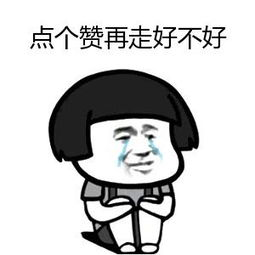
PyTorch | transforms常用方法
\qquad
torchvision.transforms
是
pytorch
中的图像预处理包。一般用
Compose
把多个步骤整合到一起。
Compose
会将
transforms
列表里面的
transform
操作进行遍历。
class torchvision.transforms.Compose(transforms):
# Composes several transforms together.
# Parameters: transforms (list of Transform objects) – list of transforms to compose.
\qquad 使用transform
操作之前需要导包:
import torchvision.transforms as transforms
\qquad 本文对transforms
中的各个预处理方法进行介绍和总结。官方文档只是将方法陈列,没有归纳总结,顺序很乱,这里总结一共有四大类,方便大家索引:
- 裁剪(Crop)
(1)随机裁剪:transforms.RandomCrop
(2)中心裁剪:transforms.CenterCrop
(3)随机长宽比裁剪:transforms.RandomResizedCrop
(4)上下左右中心裁剪:transforms.FiveCrop
(5)上下左右中心裁剪后翻转:transforms.TenCrop
- 翻转和旋转(Flip and Rotation)
(1)依概率 p p p水平翻转:transforms.RandomHorizontalFlip(p=0.5)
(2)依概率 p p p垂直翻转:transforms.RandomVerticalFlip(p=0.5)
(3)随机旋转:transforms.RandomRotation
- 图像变换(resize)
(1)调整PILImage
对象的尺寸:transforms.Resize
(2)标准化:transforms.Normalize
(3)转为tensor
,并归一化至[0-1]
:transforms.ToTensor
(4)填充:transforms.Pad
(5)修改亮度、对比度和饱和度:transforms.ColorJitter
(6)转灰度图:transforms.Grayscale
(7)线性变换:transforms.LinearTransformation
(8)仿射变换:transforms.RandomAffine
(9)依概率p转为灰度图:transforms.RandomGrayscale
(10)将数据转换为PILImage
:transforms.ToPILImage
(11)自定义自己的transform
策略:transforms.Lambda
- 对
transforms
操作,使数据增强更灵活
(1)从给定的一系列transforms
中选一个进行操作:transforms.RandomChoice(transforms)
(2)给一个transforms
加上概率,依概率进行操作:transforms.RandomApply(transforms, p=0.5)
(3)将transforms
中的操作随机打乱:transforms.RandomOrder
一、裁剪(Crop)
1.1 transforms.RandomCrop
# 功能:从图片中随机裁剪出尺寸为size的图片
torchvision.transforms.RandomCrop(size,padding=None,pad_if_need=False,fill=0,padding_mode='constant')
- s i z e size size:所需裁剪图片尺寸,数据类型为
sequence
或int
,若为sequence
,则为(h,w)
,若为int
,则(size,size)
。 - p a d d i n g padding padding:设置填充大小,默认值为None,即无填充。
- 当为
a
时上下左右均填充a
个像素。 - 当为
(a, b)
时,左右填充a
个像素,上下填充b
个像素。 - 当为
(a, b, c, d)
时,左、上、右、下分别填充a,b,c,d
。
- 当为
- p a d _ i f _ n e e d pad\_if\_need pad_if_need:当图像小于设定
size
时,是否填充,若为False
则可能引发异常。由于是在填充完成之后完成裁剪操作,因此会对填充后的图像进行随机裁剪。 - f i l l fill fill:当 p a d d i n g _ m o d e padding\_mode padding_mode 设置为 c o n s t a n t constant constant 时,设置填充的像素值。若传入数值,各通道均填充该值,默认值是 0 0 0;若传入长度为 3 3 3 的元组时,分别表示
RGB
三通道需要填充的值。 - p a d d i n g _ m o d e padding\_mode padding_mode:填充模式,有4种模式,默认为
constant
。- c o n s t a n t constant constant:填充像素值由 f i l l fill fill设定。
- e d g e edge edge:填充像素值由图像边缘像素决定。
- r e f l e c t reflect reflect:镜像填充,最后一个像素不镜像,eg:[1, 2, 3, 4] -->[ 3, 2, 1, 2, 3, 4, 3, 2](2个像素填充)
- s y m m e t r i c symmetric symmetric:镜像填充,最后一个像素镜像。eg:[1, 2, 3, 4] -->[ 2, 1, 1, 2, 3, 4, 4, 3](2个像素填充)
1.2 transforms.CenterCrop
# 功能:依据给定的size从中心裁剪
torchvision.transforms.CenterCrop(size)
- s i z e size size:所需裁剪图片尺寸,数据类型为
sequence
或int
,若为sequence
,则为(h,w)
,若为int
,则(size,size)
。
1.3 transforms.RandomResizedCrop
# 功能:按照随机大小、随机宽高比裁剪图片
torchvision.transforms.RandomResizedCrop(size, scale=(0.08, 1.0), ratio=(0.75, 1.3333333333333333), interpolation=2)
- s i z e size size:所需裁剪图片尺寸,数据类型为
sequence
或int
,若为sequence
,则为(h,w)
,若为int
,则(size,size)
。 - s c a l e scale scale:随机裁剪面积比例,默认为原始图像的
0.08
到1.0
倍。 - r a t i o ratio ratio:随机长宽比,默认为
3/4
到4/3
倍。 - i n t e r p o l a t i o n interpolation interpolation:插值方法,默认为双线性插值
PIL.Image.BILINEAR
。PIL.Image.NEAREST
\qquad 1PIL.Image.BILINEAR
\qquad 2PIL.Image.BICUBIC
\qquad 3PIL.Image.LANCZOS
\qquad 4
1.4 transforms.FiveCrop
# 功能:在图像的上下左右及中心裁剪出尺寸为size的图片,获得5张图片,返回一个4D-tensor
torchvision.transforms.FiveCrop(size)
- s i z e size size:所需裁剪图片尺寸,数据类型为
sequence
或int
,若为sequence
,则为(h,w)
,若为int
,则(size,size)
。
1.5 transforms.TenCrop
# 功能:对图片进行上下左右以及中心裁剪出尺寸为size的图片,然后全部翻转(水平或者垂直),获得10张图片,返回一个4D-tensor
torchvision.transforms.TenCrop(size, vertical_flip=False)
- s i z e size size:所需裁剪图片尺寸,数据类型为
sequence
或int
,若为sequence
,则为(h,w)
,若为int
,则(size,size)
。 - v e r t i c a l _ f l i p vertical\_flip vertical_flip:是否垂直翻转,默认为
False
,即默认为水平翻转。
二、翻转和旋转(Flip and Rotation)
2.1 依概率 p 水平翻转
# 功能:依据概率p对PIL图片进行水平翻转
torchvision.transforms.RandomHorizontalFlip(p=0.5)
- p p p:图像被翻转的概率,默认值为
0.5
。
2.2 依概率 p 垂直翻转
# 功能:依据概率p对PIL图片进行垂直翻转
torchvision.transforms.RandomVerticalFlip(p=0.5)
- p p p:图像被翻转的概率,默认值为
0.5
。
2.3 随机旋转:
# 功能:按照degrees随机旋转一定角度
torchvision.transforms.RandomRotation(degrees, resample=False, expand=False, center=None, fill=None)
- d e g r e e s degrees degrees:要旋转的角度范围。数据类型为
sequence
或int/float
,若为sequence
,则范围为(min,max)
,若为int/float
,则范围为(size,size)
。 - r e s a m p l e resample resample:可选的重采样过滤器。如果省略,或者图像具有模式“
1
”或“P
”,则将其设置为PIL.Image.NEAREST
。PIL.Image.NEAREST
\qquad 1PIL.Image.BILINEAR
\qquad 2PIL.Image.BICUBIC
\qquad 3PIL.Image.LANCZOS
\qquad 4
- e x p a n d expand expand:可选的扩展标志。如果为
true
,则展开输出以使其足够大以容纳整个旋转图像。如果为false或省略,则使输出图像与输入图像的大小相同。请注意,扩展标志假定围绕中心旋转而不进行平移。 - c e n t e r center center:可选的旋转中心坐标
(x,y)
,原点是图片左上角,默认值是图像的中心。 - f i l l fill fill:用于填充在旋转图片之外的像素点的像素值。若传入数值,各通道均填充该值,默认值是 0 0 0;若传入长度为 3 3 3 的元组时,分别表示
RGB
三通道需要填充的值。
三、图像变换(resize)
3.1 transforms.Resize
# 功能:调整PILImage对象的尺寸
torchvision.transforms.Resize(size, interpolation=2)
- s i z e size size:所需裁剪图片尺寸,数据类型为
sequence
或int
,若为sequence
,则为(h,w)
,若为int
,则(size,size)
。 - i n t e r p o l a t i o n interpolation interpolation:插值方法,默认为双线性插值
PIL.Image.BILINEAR
。PIL.Image.NEAREST
\qquad 1PIL.Image.BILINEAR
\qquad 2PIL.Image.BICUBIC
\qquad 3PIL.Image.LANCZOS
\qquad 4
3.2 transforms.Normalize
# 功能:将数据转换为标准高斯分布,即逐个channel的对图像进行标准化(均值变为0,标准差变为1),可以加快模型的收敛。
# 对每个通道而言,Normalize执行以下操作:input[channel]=(input[channel]-mean[channel])/std[channel]
torchvision.transforms.Normalize(mean, std)
- m e a n mean mean:每个通道的均值序列。
- s t d std std:每个通道的标准偏差序列。
3.3 transforms.ToTensor
# 功能:将PIL或numpy(即cv2读取)格式,shape为(H,W,C)的图像转为shape为(C,H,W)的tensor,
# 同时将每一个[0,255]范围的数值除以255归一化到[0,1]范围
torchvision.transforms.ToTensor()
3.4 transforms.Pad
# 功能:使用给定的“pad”值在所有面上填充给定的PIL图像
torchvision.transforms.Pad(padding, fill=0, padding_mode='constant')
- p a d d i n g padding padding:设置填充大小,默认值为None,即无填充。
- 当为
a
时上下左右均填充a
个像素。 - 当为
(a, b)
时,左右填充a
个像素,上下填充b
个像素。 - 当为
(a, b, c, d)
时,左、上、右、下分别填充a,b,c,d
。
- 当为
- f i l l fill fill:当 p a d d i n g _ m o d e padding\_mode padding_mode 设置为 c o n s t a n t constant constant 时,设置填充的像素值。若传入数值,各通道均填充该值,默认值是 0 0 0;若传入长度为 3 3 3 的元组时,分别表示
RGB
三通道需要填充的值。 - p a d d i n g _ m o d e padding\_mode padding_mode:填充模式,有4种模式,默认为
constant
。- c o n s t a n t constant constant:填充像素值由 f i l l fill fill设定。
- e d g e edge edge:填充像素值由图像边缘像素决定。
- r e f l e c t reflect reflect:镜像填充,最后一个像素不镜像,eg:[1, 2, 3, 4] -->[ 3, 2, 1, 2, 3, 4, 3, 2](2个像素填充)
- s y m m e t r i c symmetric symmetric:镜像填充,最后一个像素镜像。eg:[1, 2, 3, 4] -->[ 2, 1, 1, 2, 3, 4, 4, 3](2个像素填充)
3.5 transforms.ColorJitter
# 功能:随机改变图像的属性:亮度(brightness)、对比度(contrast)、饱和度(saturation)和色调(hue)
torchvision.transforms.ColorJitter(brightness=0, contrast=0, saturation=0, hue=0)
- b r i g h t n e s s brightness brightness:将图像的亮度随机变化为原图亮度的
[max(0, 1-brightness), 1+brightness]
。 - c o n t r a s t contrast contrast:将图像的对比度随机变化为原图对比度的
[max(0, 1-contrast), 1+contrast]
。 - s a t u r a t i o n saturation saturation:将图像的饱和度随机变化为原图饱和度的
[max(0, 1-saturation), 1+saturation]
。 - h u e hue hue:将图像的色调随机变化为原图色调的
[max(0, 1-hue), 1+hue]
。
3.6 transforms.Grayscale
# 功能:将图像转换为灰度图
torchvision.transforms.Grayscale(num_output_channels=1)
- n u m _ o u t p u t _ c h a n n e l s num\_output\_channels num_output_channels:输出通道数只能设置为 1 1 1 或 3 3 3,若
num_output_channels=1
则转换为单通道灰度图,若num_output_channels=3
则返回三通道图像,且三通道数值相等。
3.7 transforms.LinearTransformation
# 功能:用一个预先准备好的变换方阵(transformation_matrix)对图片张量做变换。
# 首先将原图像Flatten为一维向量,然后将一维向量逐元素与向量元素平均值mean_vector相减,
# 然后将计算结果与transformation_matrix计算点积(dot product),最后将shape转换成原始尺寸。
torchvision.transforms.LinearTransformation(transformation_matrix, mean_vector)
- t r a n s f o r m a t i o n _ m a t r i x transformation\_matrix transformation_matrix:变换方阵,是一个
shape
为[D x D]
的tensor
,D = C x H x W
。 - m e a n _ v e c t o r mean\_vector mean_vector:平均值向量,是一个
shape
为[D]
的tensor
,D = C x H x W
。
3.8 transforms.RandomAffine
# 功能:图像保持中心不变的随机仿射变换
torchvision.transforms.RandomAffine(degrees, translate=None, scale=None, shear=None, resample=False, fillcolor=0)
- d e g r e e s degrees degrees:要旋转的度数范围。如果
degrees
是一个数字而不是像(min,max)
这样的序列,则度数范围将是(-degrees,+degrees)
。设置为 0 0 0 可停用旋转。 - t r a n s l a t e translate translate(元组,可选) - 水平和垂直平移的最大绝对分数元组。例如translate =(a,b),然后在范围-img_width * a <dx <img_width * a中随机采样水平移位,并且在-img_height * b <dy <img_height * b范围内随机采样垂直移位。默认情况下不会翻译。
- s c a l e scale scale(元组,可选) - 缩放因子间隔,例如(a,b),然后从范围a <= scale <= b中随机采样缩放。默认情况下会保持原始比例。
- s h e a r shear shear(sequence 或float或int,optional) - 要选择的度数范围。如果degrees是一个数字而不是像(min,max)这样的序列,则度数范围将是(-degrees,+ degrees)。默认情况下不会应用剪切
- r e s a m p l e resample resample:可选的重采样过滤器。如果省略,或者图像具有模式“
1
”或“P
”,则将其设置为PIL.Image.NEAREST
。PIL.Image.NEAREST
PIL.Image.BILINEAR
PIL.Image.BICUBIC
PIL.Image.LANCZOS
- f i l l c o l o r fillcolor fillcolor:用于填充在旋转图片之外的像素点的像素值。若传入数值,各通道均填充该值,默认值是 0 0 0;若传入长度为 3 3 3 的元组时,分别表示
RGB
三通道需要填充的值。
3.9 transforms.RandomGrayscale
# 功能:以概率p(默认值0.1)随机转换输入图片为灰度图
torchvision.transforms.RandomGrayscale(p=0.1)
- p p p:图像被转换的概率,默认值为
0.1
。
3.10 transforms.ToPILImage
# 功能:将shape为(C,H,W)的Tensor或shape为(H,W,C)的numpy.ndarray转换成PIL.Image
torchvision.transforms.ToPILImage(mode=None)
- m o d e mode mode:若
mode
是None
(默认),则会对输入图片作一些假设:- 若输入图片有 4 4 4 个
channel
,则mode
被假定为RGBA
。 - 若输入图片有 3 3 3 个
channel
,则mode
被假定为RGB
。 - 若输入图片有 2 2 2 个
channel
,则mode
被假定为LA
。 - 若输入图片有 1 1 1 个
channel
,则mode
由数据类型决定(比如int,float,short
)。
- 若输入图片有 4 4 4 个
3.11 transforms.Lambda
# 功能:将用户自定义的lambda函数作为transform
torchvision.transforms.Lambda(lambd)
- l a m b d lambd lambd:用于转换的
Lambda
函数。
四、对 transforms 操作,使数据增强更灵活
4.1 transforms.RandomChoice
# 功能:从transforms(列表)中随机选择一个来使用
torchvision.transforms.RandomChoice(transforms)
- t r a n s f o r m s transforms transforms:待选的转换列表。
4.2 transforms.RandomApply
# 功能:以概率p来决定是否使用transforms(列表)中的转换
torchvision.transforms.RandomApply(transforms, p=0.5)
- t r a n s f o r m s transforms transforms:待选的转换列表。
- p p p:使用
transforms
(列表)的概率,默认值为0.5
。
4.3 transforms.RandomOrder
# 功能:随机打乱transforms中的顺序
torchvision.transforms.RandomOrder(transforms)
- t r a n s f o r m s transforms transforms:转换列表。