前言
在介绍赋值运算符重载之前,我们先看一段代码:
class Complex //定义一个复数类
{
public:
Complex (double r = 0.0,double i = 0.0) //构造函数
{
_real = r;
_imag = i;
}
void Show()const;
private:
double _real,_imag;
};
int main()
{
//声明2个对象
Complex a(10,20);
Complex b (20,30);
return 0;
}
此时,如果我们需要对a和b进行加法运算该咋整?一般情况下我们自然都能想到使用" + “运算符,写出一个表达式:” a + b",但是很遗憾,编译的时候必会报错,因为编译器不知道该怎么处理这个加法,这个时候就需要我们自己来编写作用在Complex类对象的"+"功能,这便引出了运算符重载
一、赋值运算符重载函数是什么?
赋值运算符重载是对已经有的运算符赋予多重含义,使同一个运算符作用于不同类型的数据时有着不同的含义。
函数名为:关键字operator后面加上需要重载的运算符符号
函数原型:
返回值类型 operator 运算符 (形参列表)
{
//函数体
}
Notes:运算符重载的实质就是函数的重载:在实现的过程中,首先把指定的运算表达式转化为对运算符的调用,将运算对象转化为运算符函数的参数,然后根据参数的类型来确定需要调用的函数。
二、细谈赋值运算符重载函数
2.1 参数列表
一般情况下,赋值运算符重载函数的参数是函数所在类的const类型的引用,加const的引用原因如下:
- 程序员不希望在函数中对已经用const修饰的值做任何修改。
- 加上const后,对于const和非const的参数,函数就能接受;如果不加,就只能接受非const的参数。
加引用的原因:
加上引用后可以有效的避免函数调用时对实参的拷贝,提高了程序的效率。
扫描二维码关注公众号,回复:
12761113 查看本文章
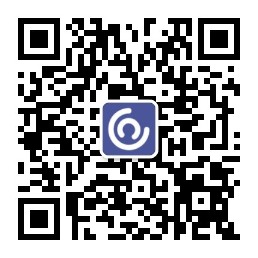
Notes:以上的规定不是强制性规定。可以不加const,也可以没有引用,甚至参数可以不是函数所在的对象。
2.2 返回值
一般情况下,返回值是被赋值者的引用,即*this,原因如下:
- 返回*this,避免了函数的一次拷贝,提高程序效率。
- 返回引用还可以达到连续赋值的作用,比如"a = b = c"。如果返回类型不是引用而是返回值类型,那么在执行b = c 时候,调用赋值运算符重载函数,在函数返回的时候,会形成一个无名的临时对象,接着拷贝临时对象,然后将这个临时对象返回,但是这临时对象是一个右值,再进行a = 时候,会出错。
2.3调用时机
当为一个类对象赋值时,会由该对象调用该类的赋值运算符重载函数。
MyStr str1;
str1 = str2;
MyStr str3 = str2;
比如上述例子,定义个MyStr的类。第二个语句和第三个语句在调用函数上面是有区别的。
MyStr str1;是str1的声明加定义,调用无参数的构造函数,所以str2 = str1时在str1已经存在的情况下用str2来为str1赋值,调用赋值运算符重载函数;而MyStr str3 = str2;调用的是拷贝构造函数。
二、赋值运算符重载函数练习
定义一个日期类,实现各种运算符重载:
#include<iostream>
#include <cassert>
using namespace std;
class Date
{
friend ostream& operator<<(ostream& out,const Date &d);
friend istream& operator>>(istream& is,Date &d);
public:
//构造函数
explicit Date (int year = 1900,int month = 1,int day = 1)
{
_year = year,_month = month,_day = day;
//参数检测
if ((year >= 1900) && (month >= 1 && month <= 12) && (day >= 1 && day <= Day_Of_Month(year,month)))
{
_year = year,_month = month,_day = day;
}else
{
cout<<"日期非法"<<endl;
_year = 1900,_month = 1,_day = 1;
}
}
//拷贝构造函数
Date (const Date& d) : _year(d._year),_month(d._month),_day(d._day)
{
}
//析构函数
~Date()
= default;
public:
//操作符重载
Date& operator=(const Date &d);
bool operator==(const Date &d);
bool operator!=(const Date &d);
bool operator>(const Date &d);
bool operator>=(const Date &d);
bool operator<(const Date &d);
bool operator<=(const Date &d);
Date operator+(int day);
Date& operator+=(int day);
Date operator-(int day);
int operator-(const Date &d); //两个日期相隔天数
Date& operator-=(int day);
Date& operator++();
Date operator++(int);
Date& operator--();
Date operator--(int);
public:
int Day_Of_Month(int year,int month)
{
//检查合法性
assert(month > 0 && month <= 12);
int arr[13] = {
0,31,29,31,30,31,30,31,31,30,31,30,31};
int day;
if (!(Is_Leap_Year()) && month == 2)
{
_day = arr[month] - 1;
}
day = arr[month];
return day;
}
bool Is_Leap_Year()
{
return (_year % 4 == 0 && _year % 100 != 0) || _year % 400 == 0 || false;
}
private:
int _year,_month,_day;
};
ostream& operator<<(ostream& out,const Date &d)
{
out<<d._year<<"-"<<d._month<<"-"<<d._day<<endl;
return out;
}
istream& operator>>(istream& is, Date &d)
{
cout<<"输入日期:";
is >>d._year;
is >>d._month;
is >>d._day;
return is;
}
//赋值
Date& Date::operator=(const Date &d)
{
if (*this != d)
{
_year = d._year;
_month = d._month;
_day = d._day;
}
return *this;
}
bool Date::operator==(const Date &d)
{
return (_year == d._year) && (_month == d._month) && (_day == d._day);
}
bool Date::operator!=(const Date &d)
{
return !(*this == d);
}
bool Date::operator>(const Date &d)
{
return (_year > d._year) || ((_year == d._year) && (_month > d._month)) || ((_year == d._year) && (_month == d._month) && (_day > d._day));
}
bool Date::operator>=(const Date &d)
{
return (*this == d) || (*this > d);
}
bool Date::operator<(const Date &d)
{
return (_year < d._year) || ((_year == d._year) && (_month < d._month)) || ((_year == d._year) && (_month == d._month) && (_day < d._day));
}
bool Date::operator<=(const Date &d)
{
return (*this == d) || (*this < d);
}
Date& Date::operator++()
{
if (_day > Day_Of_Month(_year,_month))
{
_day -= Day_Of_Month(_year,_month);
_month++;
if (_month > 12)
{
_year++;
_month = 1;
}
}
return *this;
}
Date Date::operator++(int)
{
Date ret = *this;
_day++;
if (_day > Day_Of_Month(_year,_month))
{
_day = Day_Of_Month(_year,_month);
_month++;
if (_month > 12)
{
_year++;
_month = 1;
}
}
return ret;
}
Date& Date::operator--()
{
_day--;
if (_day < 1)
{
_month--;
if (_month < 1)
{
_year--;
_month = 12;
}
_day += Day_Of_Month(_year,_month);
}
return *this;
}
Date Date::operator--(int)
{
Date ret = *this;
_day--;
if (_day < 1)
{
_month--;
if (_month < 1)
{
_year--;
_month = 12;
}
_day += Day_Of_Month(_year,_month);
}
return ret;
}
Date Date::operator+(int day)
{
Date ret = *this;
if (day < 0)
{
return ret - (-day);
}
ret._day += day;
while (ret._day > Day_Of_Month(ret._year,ret._month))
{
ret._day -= Day_Of_Month(ret._year,ret._month);
if (ret._month == 12)
{
ret._year++;
ret._month = 1;
} else{
ret._month++;
}
}
return ret;
}
Date& Date::operator+=(int day)
{
*this = *this + day;
return *this;
}
Date Date::operator-(int day)
{
Date ret = *this;
if (day < 0)
{
return ret + (-day);
}
ret._day -= day;
while (ret._day <= 0)
{
if (ret._month == 1)
{
ret._year--;
ret._month = 12;
}else{
--ret._month;
}
ret._day += Day_Of_Month(ret._year,ret._month);
}
return ret;
}
Date& Date::operator-=(int day)
{
*this -= day;
return *this;
}
int Date::operator-(const Date &d)
{
//比较两个日期的大小,找出最大的或者最小的
Date Max = *this;
Date Min = d;
int flag = 1;
if (*this < d)
{
Min = *this;
Max = d;
flag = -1;
}
int day = 0;
while (Max != Min)
{
--Max;
++day;
}
return flag * day;
}
int main()
{
Date d1(2021,12,31);
Date d2(2021,3,1);
cout<<d1;
int ret = d1 - d2;
cout<<ret<<endl;
Date d3 = d1 + 332;
cout<<d3<<endl;
Date d4(d2);
cout<<d4<<endl;
Date d5 = d1 - 1;
cout<<d5<<endl;
Date d6 = d1++;
cout<<d6<<endl;
Date d7;
cin>>d7;
cout<<d7;
d1 += 2;
cout<<d1<<endl;
return 0;
}