目录
前言:
ASP.NET Core 中的应用配置基于配置提供程序 建立的 键值对 。
配置提供程序将配置数据从各种配置源读取到键值对。
配置框架的核心实现包是Microsoft Extensions.Configuration
,
依赖于Microsoft Extensions.Configuration.Abstractions
抽象包。
使用了 接口实现分离 的设计模式,使用时引入实现包就可以了。
一、内存配置提供程序
若要激活内存中集合配置,请在 ConfigurationBuilder
的实例上调用 AddInMemoryCollection
扩展方法。
static void Main(string[] args)
{
IConfigurationBuilder builder = new ConfigurationBuilder();
// 通过 AddInMemoryCollection 的调用使用字典,以提供配置
builder.AddInMemoryCollection(
new Dictionary<string, string>()
{
{
"key1","value1" },
{
"section1:key2","value2" },
{
"section1:section2:key3","value3" }
});
IConfigurationRoot configurationRoot = builder.Build();
Console.WriteLine($"key1:{configurationRoot["key1"]}");
var section = configurationRoot.GetSection("section1");
Console.WriteLine($"key2:{section["key2"]}");
var section2 = section.GetSection("section2");
Console.WriteLine($"key3:{section2["key3"]}");
}
二、命令行配置提供程序
命令行配置需要额外引入Microsoft Extensions.Configuration.CommandLine
包
1) 激活命令行配置
要激活命令行配置,请在 ConfigurationBuilder
的实例上调用 AddCommandLine
扩展方法。
static void Main(string[] args)
{
var builder = new ConfigurationBuilder();
// 若要提供命令行参数可替代的应用配置,最后请调用 AddCommandLine
builder.AddCommandLine(args);
}
2) 自变量
命令行配置支持三种格式的命令:
- 无前缀
CommandLineKey1=value1
- 双划线 (–)
--CommandLineKey2=value2
,--CommandLineKey2 value2
- 正斜杠 (/)
/CommandLineKey3=value3
,/CommandLineKey3 value3
示例:
dotnet run CommandLineKey1=value1 --CommandLineKey2=value2 /CommandLineKey3=value3
dotnet run --CommandLineKey1 value1 /CommandLineKey2 value2
dotnet run CommandLineKey1= CommandLineKey2=value2
还可以在项目根目录的 ~/Properties/launchSettings.json
文件中设置调试模式启动时的命令参数
// ~/Properties/launchSettings.json
{
"profiles": {
"ConfigurationCommandLineDemo": {
"commandName": "Project",
"commandLineArgs": "Key1=val1 --Key2=val2 /Key3=val3"
}
}
}
3) 交换映射
交换映射支持键名替换逻辑。 使用 ConfigurationBuilder
手动构建配置时,需要为 AddCommandLine
方法提供交换替换字典。
交换映射字典键规则:
- 交换必须以单划线 (-) 或双划线 (–) 开头。
- 交换映射字典不得包含重复键。
示例:
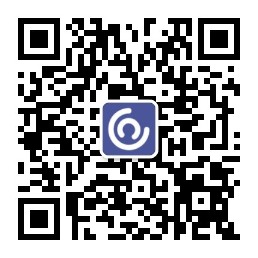
// dotnet run -k1=val1 -k2=val2 -k3=val3
static void Main(string[] args)
{
var mapper = new Dictionary<string, string> {
{
"-k1", "key1" },
{
"-k2", "key2" },
{
"-k3", "key3" }
};
var builder = new ConfigurationBuilder();
builder.AddCommandLine(args, mapper);
var configurationRoot = builder.Build();
Console.WriteLine($"helper:{configurationRoot["key1"]}");
Console.WriteLine($"helper:{configurationRoot["key2"]}");
Console.WriteLine($"helper:{configurationRoot["key3"]}");
}
三、环境变量配置提供程序
环境变量配置需要额外引入Microsoft Extensions.Configuration.EnvironmentVariables
包
在环境变量中使用分层键时,冒号分隔符 (:)
可能无法适用于所有平台(例如 Bash)。 所有平台均支持采用双下划线 (__)
,并可以用冒号自动替换。
还可以在项目根目录的 ~/Properties/launchSettings.json
文件中设置调试模式启动时的环境变量
// ~/Properties/launchSettings.json
{
"profiles": {
"ConfigurationEnvironmentVariablesDemo": {
"commandName": "Project",
"environmentVariables": {
"ASPNETCORE_ENVIRONMENT": "Development",
"SECTION__KEY": "val" // 双下划线
}
}
}
}
要激活环境变量配置,请在 ConfigurationBuilder
的实例上调用 AddEnvironmentVariables
扩展方法。
static void Main(string[] args)
{
var builder = new ConfigurationBuilder();
builder.AddEnvironmentVariables();
var configurationRoot = builder.Build();
// Development
var env = configurationRoot["ASPNETCORE_ENVIRONMENT"];
// val (双下划线作为分层键,也用冒号获取)
var key = configurationRoot["SECTION:KEY"];
var section = configurationRoot.GetSection("SECTION");
// val
var key1 = section["KEY"];
}
并且环境变量提供程序还支持根据环境变量的前缀来加载
static void Main(string[] args)
{
var builder = new ConfigurationBuilder();
builder.AddEnvironmentVariables("ASPNETCORE_");
var configurationRoot = builder.Build();
// Development
var env = configurationRoot["ENVIRONMENT"];
}
四、文件配置提供程序
FileConfigurationProvider
是从文件系统加载配置的基类。
文件配置提供程序支持指定文件可选和必选,还可以指定是否监视文件变更。
以下配置提供程序专用于特定文件类型:
- INI 配置提供程序 (需要引用
Microsoft Extensions.Configuration.Ini
包) - JSON 配置提供程序 (需要引用
Microsoft Extensions.Configuration.Json
包) - XML 配置提供程序 (需要引用
Microsoft Extensions.Configuration.Xml
包)
1) INI文件
冒号可用作 INI 文件配置中的节分隔符。
[section0]
key0=value
key1=value
[section1]
subsection:key=value
[section2:subsection0]
key=value3
[section2:subsection1]
key=value
若要激活 INI 文件配置,请在 ConfigurationBuilder 的实例上调用 AddIniFile 扩展方法。
static void Main(string[] args)
{
var builder = new ConfigurationBuilder();
// AddIniFile 的 optional 参数是设定文件是否可选
// AddIniFile 的 reloadOnChange 参数为是否重新加载配置
builder.AddIniFile("appsettings.ini");
var configurationRoot = builder.Build();
// value3
var key = configurationRoot["section2:subsection0:key"];
}
2) JSON文件
JsonConfigurationProvider 在运行时期间从 JSON 文件键值对加载配置。
{
"Logging": {
"LogLevel": {
"Default": "Debug",
"Microsoft": "Information"
}
}
}
若要激活 JSON 文件配置,请在 ConfigurationBuilder
的实例上调用 AddJsonFile
扩展方法。
static void Main(string[] args)
{
var builder = new ConfigurationBuilder();
// AddJsonFile 的 optional 参数是设定文件是否可选
// AddJsonFile 的 reloadOnChange 参数为是否重新加载配置
builder.AddJsonFile("appsettings.json");
var configurationRoot = builder.Build();
// Information
var micr = configurationRoot["Logging:LogLevel:Microsoft"];
}