AsyncTask简介
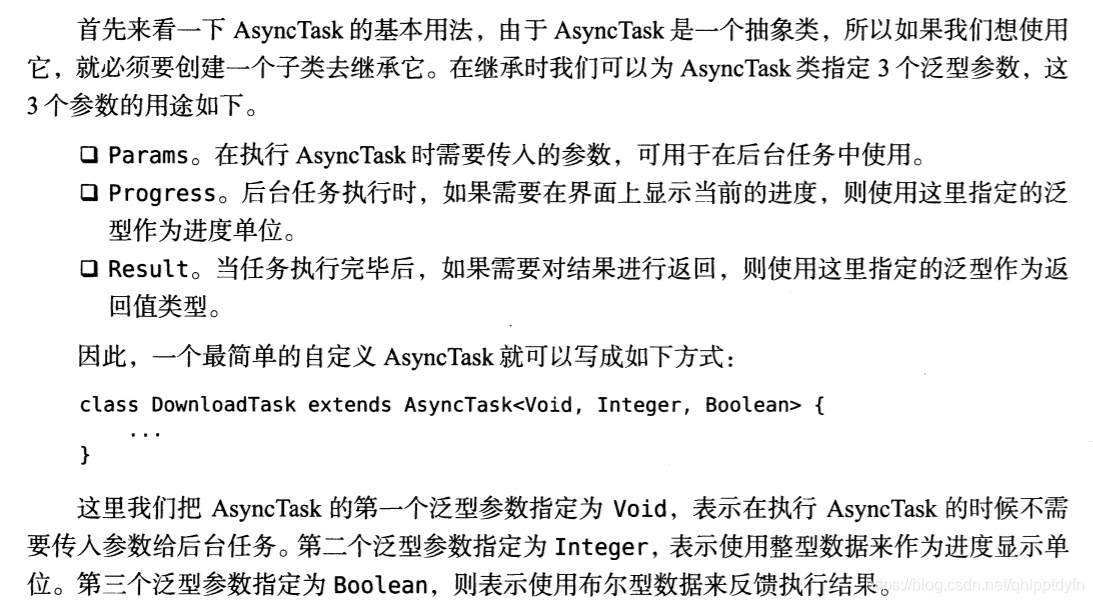
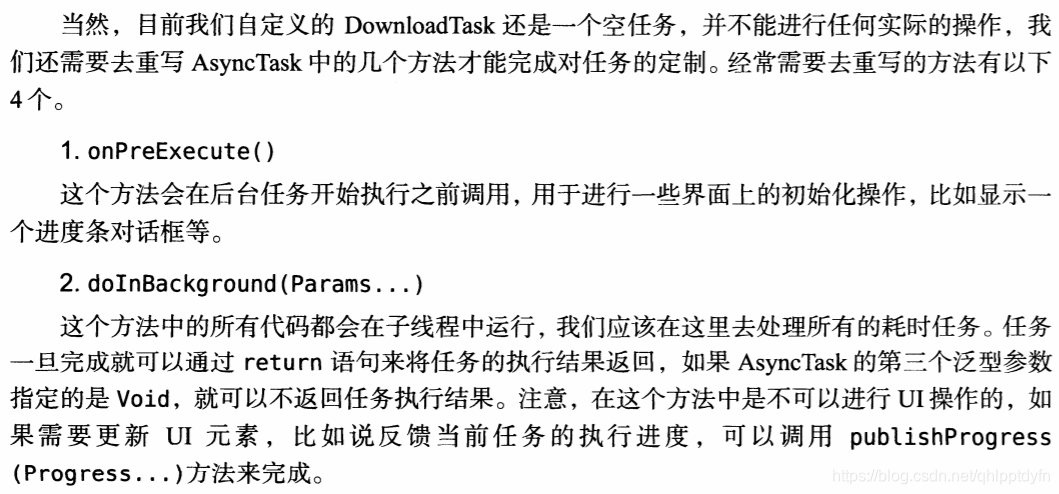
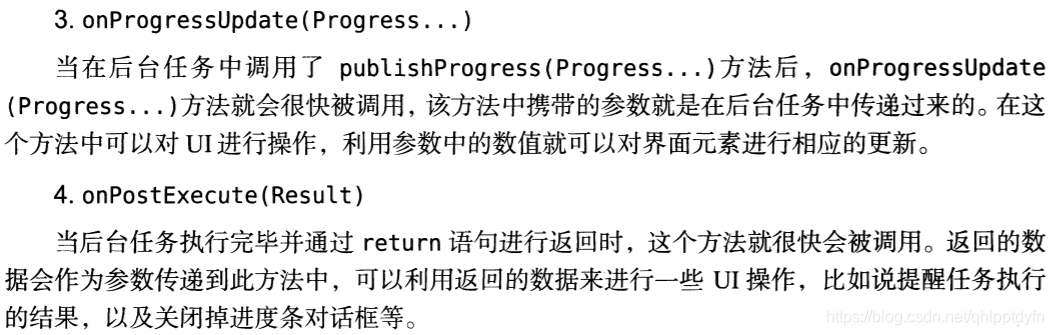
实例功能模块说明图
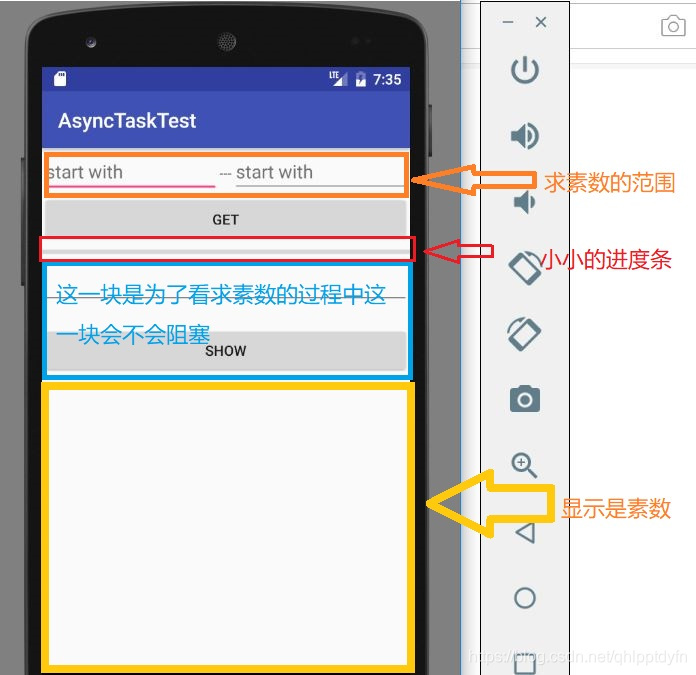
运行后效果图
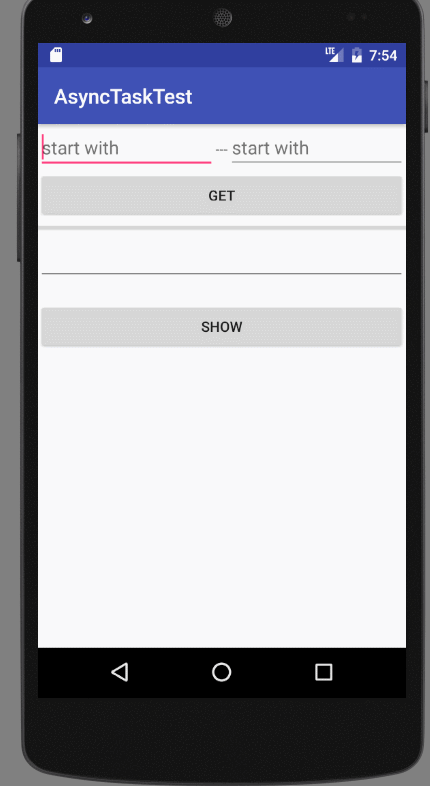
代码部分
界面代码
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:id="@+id/activity_main"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
tools:context="com.example.acer.asynctasktest.MainActivity">
<LinearLayout
android:orientation="horizontal"
android:layout_width="match_parent"
android:layout_height="wrap_content">
<EditText
android:id="@+id/startEdit"
android:layout_weight="1"
android:hint="start with"
android:layout_width="wrap_content"
android:layout_height="wrap_content" />
<TextView
android:text="---"
android:layout_width="wrap_content"
android:layout_height="wrap_content" />
<EditText
android:id="@+id/endtEdit"
android:layout_weight="1"
android:hint="start with"
android:layout_width="wrap_content"
android:layout_height="wrap_content" />
</LinearLayout>
<Button
android:text="GET"
android:id="@+id/button"
android:layout_width="match_parent"
android:layout_height="wrap_content" />
<ProgressBar
style="@style/Widget.AppCompat.ProgressBar.Horizontal"
android:max="100"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:id="@+id/progressBar" />
<EditText
android:id="@+id/bottomEdit"
android:layout_width="match_parent"
android:layout_height="wrap_content" />
<TextView
android:id="@+id/showBottom"
android:layout_width="match_parent"
android:layout_height="wrap_content" />
<Button
android:text="show"
android:id="@+id/showBottomBtn"
android:layout_width="match_parent"
android:layout_height="wrap_content" />
<ScrollView
android:id="@+id/scrollView"
android:layout_width="match_parent"
android:layout_height="match_parent">
<TextView
android:id="@+id/showTextView"
android:layout_width="match_parent"
android:layout_height="wrap_content" />
</ScrollView>
</LinearLayout>
主类代码
public class MainActivity extends AppCompatActivity {
public ProgressBar progressBar;//进度条控件
public TextView textView;//显示素数的文本显示框
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
progressBar = (ProgressBar)findViewById(R.id.progressBar);
progressBar.setProgress(0);
textView = (TextView)findViewById(R.id.showTextView);
Button button = (Button)findViewById(R.id.button);//get按钮,点击后开始进行求素数的活动
button.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
progressBar.setProgress(0);
textView.setText("");
//求素数范围的第一个输入框
EditText ed1 = (EditText)findViewById(R.id.startEdit);
//求素数范围的第二个输入框
EditText ed2 = (EditText)findViewById(R.id.endtEdit);
String st = ed1.getText().toString();
String ed = ed2.getText().toString();
new showPrimeByUI().execute(st+"@"+ed);//@作为分割符
}
});
Button showBtn = (Button)findViewById(R.id.showBottomBtn);//测试阻塞模块的show按钮
showBtn.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {//间输入框中的内容显示在下方的textview中
EditText ed = (EditText)findViewById(R.id.bottomEdit);
String s = ed.getText().toString();
TextView te = (TextView)findViewById(R.id.showBottom);
te.setText(s);
}
});
}
//异步处理主要功能类
class showPrimeByUI extends AsyncTask<String,Integer,Boolean>{
private int sum;//记录求素数的范围大小,主要是作为进度条的Max
@Override
//这个方法会在后台任务执行先调用,用于进行一些界面上的初始化操作,可以是显示一个进度条对话框,这里是显示一段提示文字
protected void onPreExecute() {
Toast.makeText(MainActivity.this,"start finding",Toast.LENGTH_SHORT).show();
}
@Override
//这个方法中所有的代码都会在子进程中运行,可以在这里执行耗时任务
//本例中是用来求素数
protected Boolean doInBackground(String... params) {
int start = Integer.parseInt(params[0].split("@")[0].trim());
int end = Integer.parseInt(params[0].split("@")[1].trim());
sum = end-start+1;
for (int i =start;i<=end;i++){
try {
//因为求素数的速度太快了,所以在这里停1s,有利于观察
new Thread().sleep(1000);
} catch (InterruptedException e) {
e.printStackTrace();
}
if(isPrime(i)){
publishProgress(i);
//调用publishProgress方法会执行下面的onProgressUpdate方法
}
else publishProgress(-1);
}
return true;
}
@Override
protected void onProgressUpdate(Integer... values) {
//有点类似进度条Max初始化的意味
if (progressBar.getMax()!=sum)progressBar.setMax(sum);
//因为不管是不是素数都执行了publishProgress方法所有在这里就+1相当于一个数判断完毕
progressBar.setProgress(progressBar.getProgress()+1);
if(values[0]!=-1){//是素数,就在显示区进行显示
textView.setText(textView.getText()+"\n"+values[0]);
}
}
@Override
//当后台任务执行完毕后通过return语句进行返回时,这个方法很快会被调用,可以利用返回的数据进行一些UI操作
protected void onPostExecute(Boolean aBoolean) {
if(aBoolean==true){
Toast.makeText(MainActivity.this,"finish finding",Toast.LENGTH_SHORT).show();
}
else{
Toast.makeText(MainActivity.this,"error stopped",Toast.LENGTH_SHORT).show();
}
}
//判断是不是素数
private boolean isPrime(int num){
if(num<=1)return false;
if(num==2||num==3)return true;
for(int i=2;i<=Math.sqrt(num);i++){
if(num%i==0)return false;
}
return true;
}
}
}