基础DOM和CSS操作
html、text、val
方法名 |
描述 |
html() |
获取元素中HTML内容 |
html(value) |
设置元素中HTML内容 |
text() |
获取元素中文本内容 |
text(value) |
设置元素中文本内容 |
val() |
获取表单中的文本内容 |
val(value) |
设置表单中的文本内容 |
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<span></span><br>
<span></span><br>
<button>按钮</button>
<script src="js/jquery-3.5.1.js"></script>
<script>
$('span').text('Hello text');
$('button').on('click', function(){
$('span:eq(0)').text('How are you? text');
})
console.log($('span').text());
$('span:first').html('<b>人生需要加粗。</b>');
$('span').last().html('<i>千万不要斜着走路</i>')
</script>
</body>
</html>
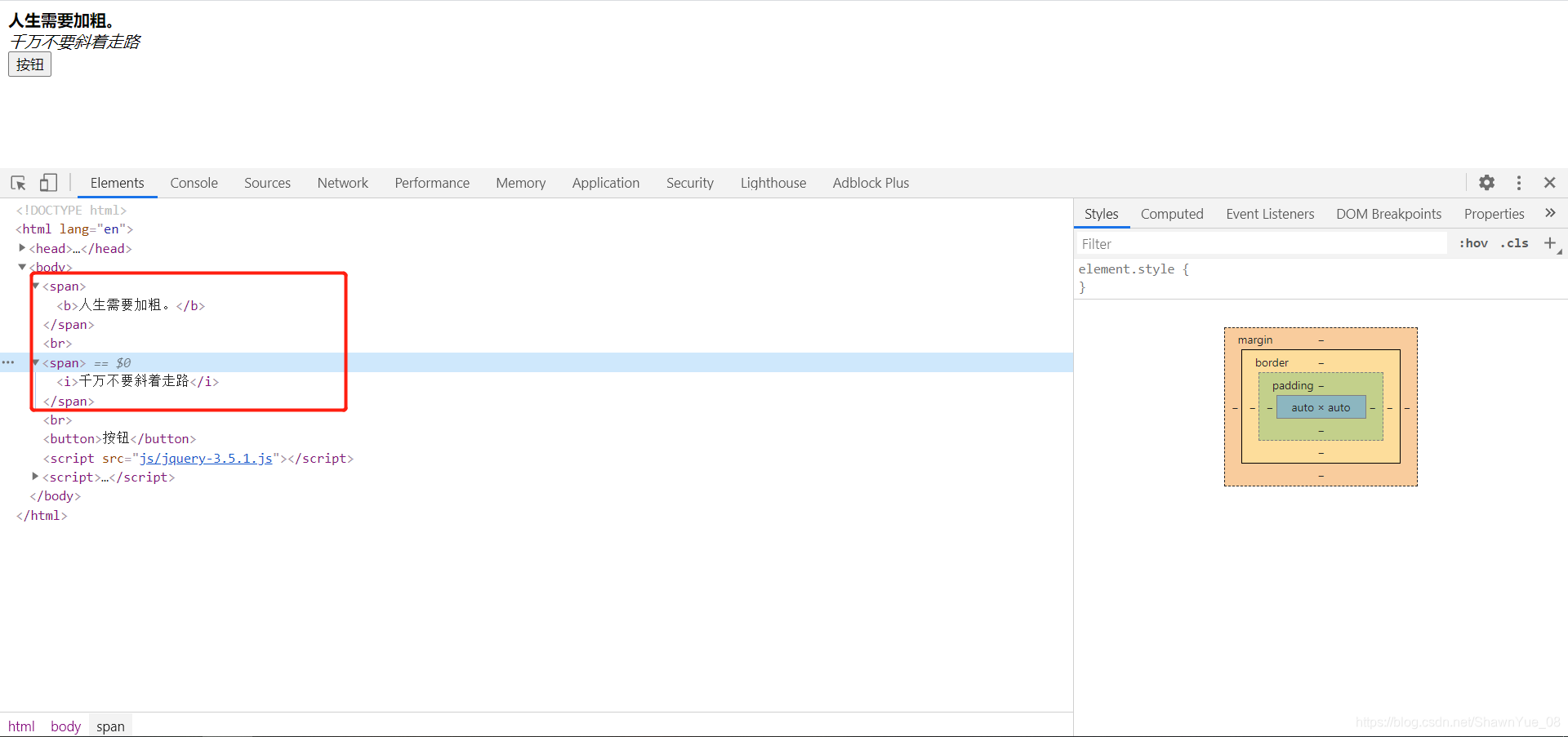
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<input type="text" value="耿耿">
<input type="text" value="余淮">
<script src="js/jquery-3.5.1.js"></script>
<script>
console.log($('input[type=text]').val());
$('input[type]').val('耿耿余淮');
</script>
</body>
</html>
attr、removeAttr
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<div>
div标签
</div>
<script src="js/jquery-3.5.1.js"></script>
<script>
$('div').attr('title','active');
console.log($('div').attr('title'));
$('div').attr({id:'div1',class:'demo'});
$('div').removeAttr('class');
</script>
</body>
</html>
css、addClass
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<div></div>
<div></div>
<script src="js/jquery-3.5.1.js"></script>
<script>
$('div').first().css('width','200px');
$('div:first').css('height','100px');
$('div').eq(0).css('backgroundColor','orange');
$('div').last().css({width:'200px',height:'100px',backgroundColor:'green',marginTop:'30px'});
console.log($('div:last').css('backgroundColor'));
console.log($('div:eq(1)').css('marginTop'));
$('div:eq(0)').addClass('active');
$('div:eq(1)').addClass('demo');
</script>
</body>
</html>
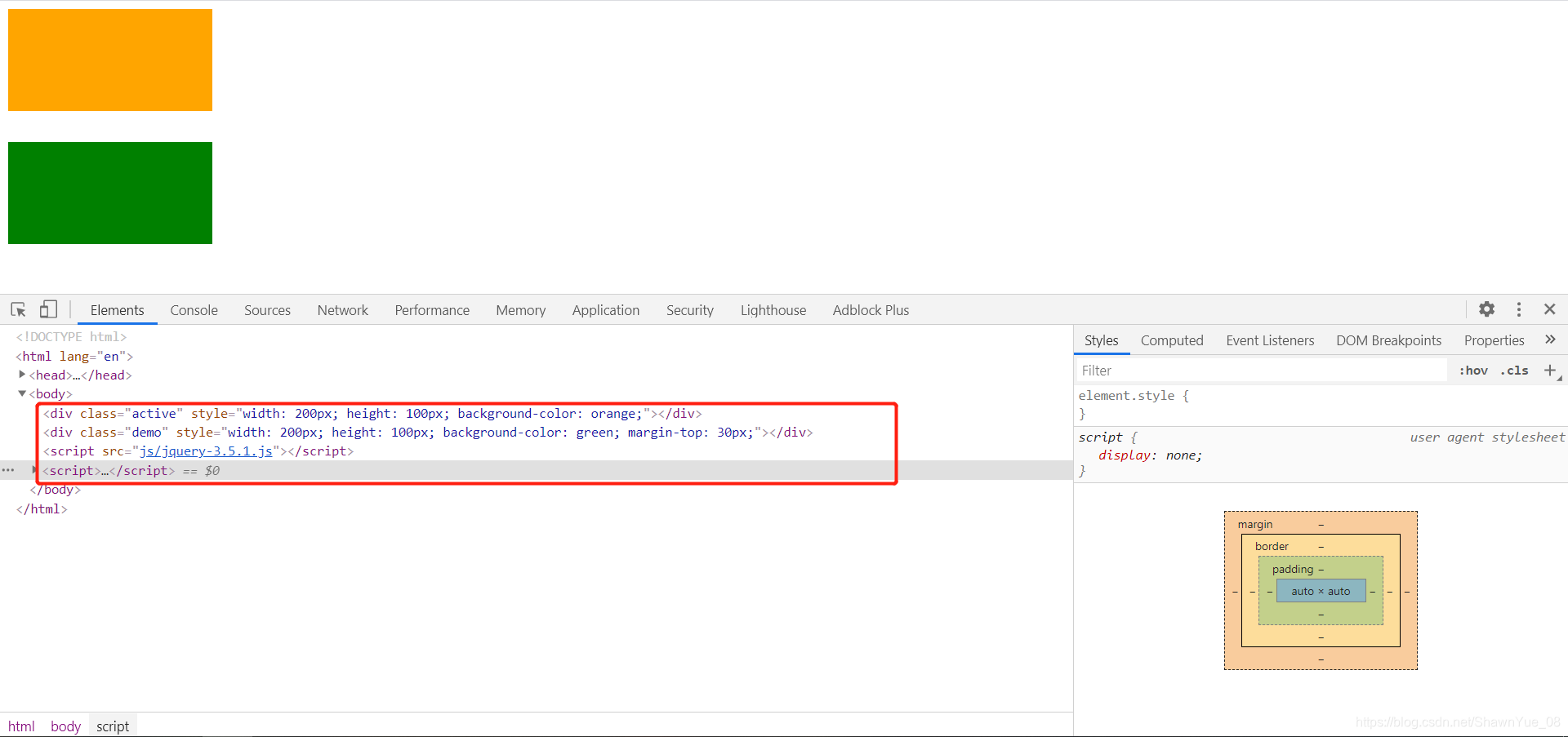
each
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<div id="d1"></div>
<div></div>
<div></div>
<script src="js/jquery-3.5.1.js"></script>
<script>
$('#d1').css({width:'200px',height:'100px',backgroundColor:'yellowgreen'});
var box = $('#d1').css(['width','height','backgroundColor']);
for(var i in box) {
console.log(i,'----',box[i]);
}
$.each(box, function(attr, value){
console.log(attr,':',value);
})
$('div').each(function(index, element){
console.log(index,element);
})
console.log($('div').length);
console.log($('div')[0]);
console.log($('div').get(1));
</script>
</body>
</html>
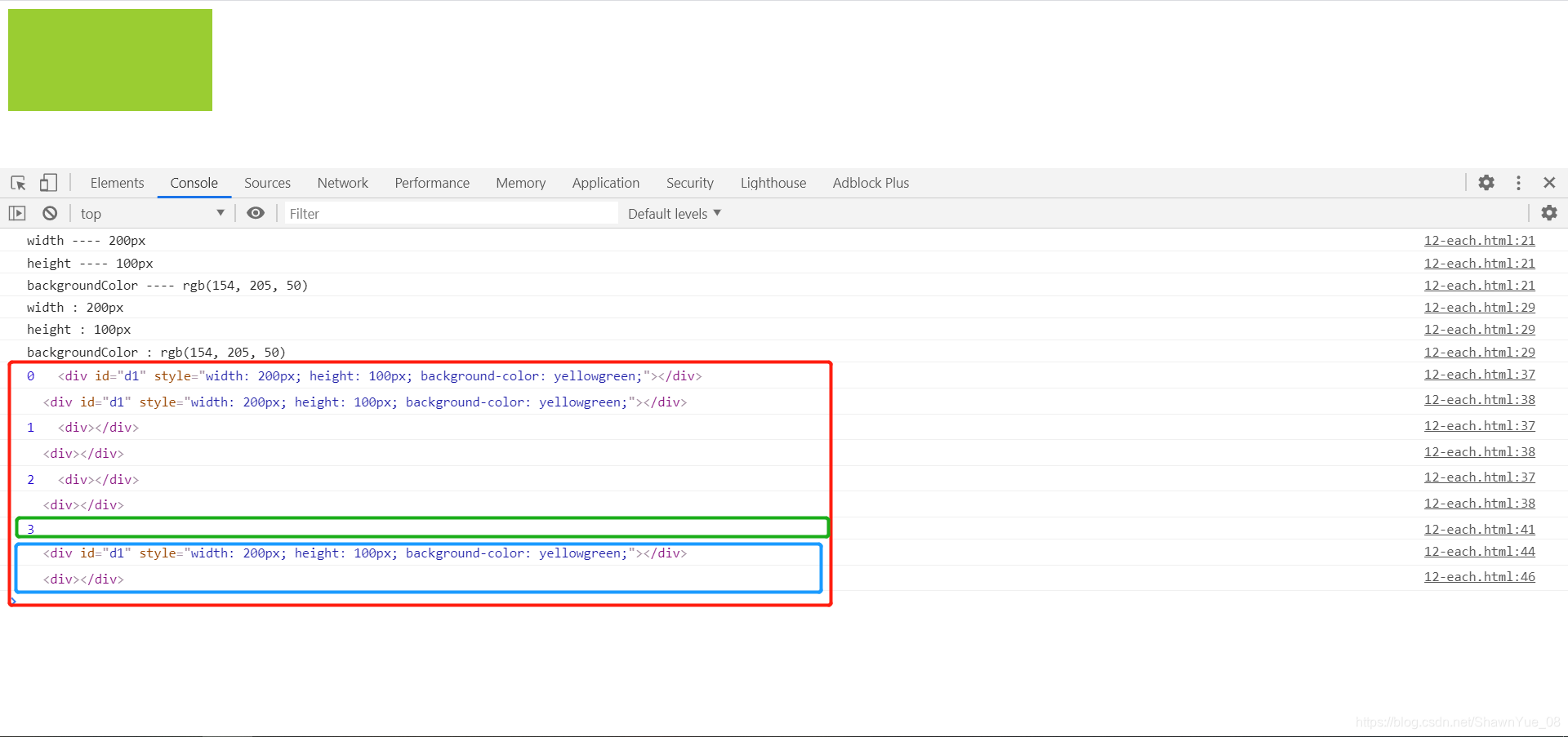
事件绑定
事件绑定简写方式
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<button>按钮</button>
<div></div>
<script src="js/jquery-3.5.1.js"></script>
<script>
$('button').click(function(){
$('div').first().css({width:'400px',height:'100px',backgroundColor:'orange'});
$('div:first').html(`<b>据确切消息称,邓紫棋在本次金曲奖入围名单中,她的专辑
《摩天动物园》入选了“年度专辑奖、年度歌曲奖、最佳作曲人奖、最佳国语女歌手奖
和最佳国语专辑奖”五项大奖,真是可喜可贺!</b>`);
})
</script>
</body>
</html>
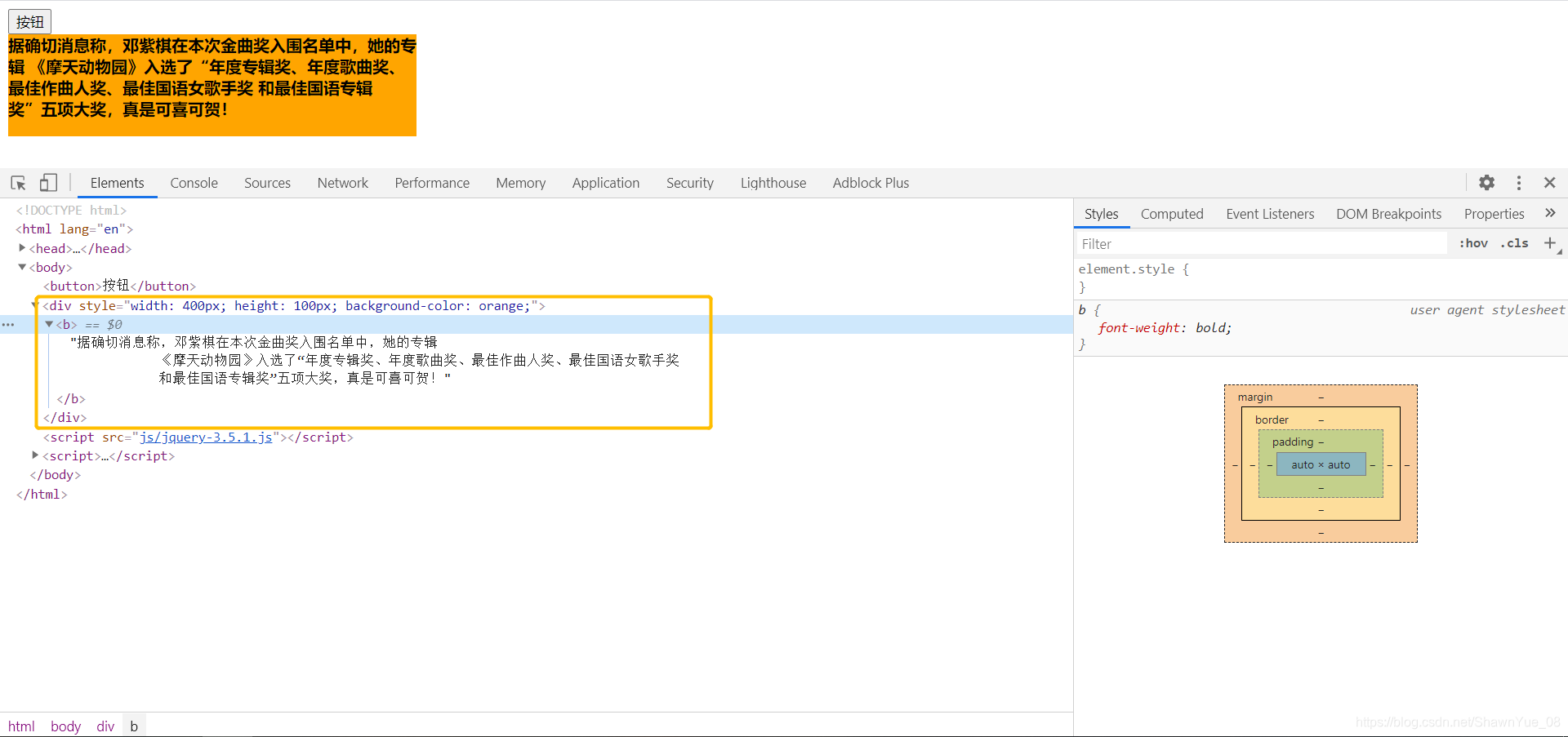
on、off、one
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<form action="#" method="GET">
用户名:<input type="text" name="username"><br>
密码:<input type="password" name="password"><br>
<input type="submit" value="提交">
</form>
<button>off</button>
<button>one</button>
<script src="js/jquery-3.5.1.js"></script>
<script>
$('form').on('submit', function(e) {
e.preventDefault();
})
$('button:first').on('click', function(){
$('form').off('submit');
})
$('button:last').one('dblclick',function(){
console.log('哈哈,我只打印一次哟!');
})
</script>
</body>
</html>