Unity 基础 之 IDragHanlder 多种方法简单实现 UGUI 元素随着鼠标移动,拖动的效果
目录
Unity 基础 之 IDragHanlder 多种方法简单实现 UGUI 元素随着鼠标移动,拖动的效果
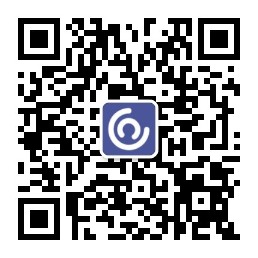
方法一:RectTransformUtility.ScreenPointToWorldPointInRectangle
方法二 :RectTransformUtility.ScreenPointToWorldPointInRectangle 并带位移 offset
方法三:RectTransformUtility.ScreenPointToLocalPointInRectangle 并带位移 Offset
方法四:Camera.WorldToScreenPoint 和 Camera.WorldToScreenPoint 并带位移 Offset
一、简单介绍
Unity中的一些基础知识点。
本节介绍,使用 IDraHandler ,简单的就实现 UGUI 元素,随着鼠标的移动而移动的效果。
二、实现原理
1、IBeginDragHandler, IDragHandler, IEndDragHandler 三个接口,进行实现拖拽的功能
2、RectTransformUtility.ScreenPointToWorldPointInRectangle 或者 RectTransformUtility.ScreenPointToLocalPointInRectangle
进行坐标转化,实现拖拽
3、必要的再结合 Camera.WorldToScreenPoint 和 Camera.ScreenToWorldPoint 一起实现拖拽移动效果
三、注意实现
1、根据 Canvas 的 Render Mode 不同的拖拽方法,移动的效果可能会略有不同,择需使用即可
四、效果预览
五、实现步骤
1、打开 Unity,新建一个空工程
2、在场景中搭建UI,布局如下
3、新建脚本,编辑方法移动 UI,把脚本对应赋值
六、多种方法实现拖拽 UI
方法一:RectTransformUtility.ScreenPointToWorldPointInRectangle
1、代码如下
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.EventSystems;
public class DragHandlerUGUI : MonoBehaviour, IBeginDragHandler, IDragHandler, IEndDragHandler
{
private RectTransform rectTransform;
// Start is called before the first frame update
void Start()
{
rectTransform = GetComponent<RectTransform>();
}
public void OnBeginDrag(PointerEventData eventData)
{
Debug.Log("开始拖拽");
}
public void OnDrag(PointerEventData eventData)
{
Vector3 pos;
RectTransformUtility.ScreenPointToWorldPointInRectangle(rectTransform, eventData.position, eventData.enterEventCamera, out pos);
rectTransform.position = pos;
}
public void OnEndDrag(PointerEventData eventData)
{
Debug.Log("结束拖拽");
}
}
2、效果如下
方法二 :RectTransformUtility.ScreenPointToWorldPointInRectangle 并带位移 offset
1、代码如下
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.EventSystems;
public class DragHandlerUGUI : MonoBehaviour, IBeginDragHandler, IDragHandler, IEndDragHandler
{
private RectTransform rectTransform;
private Vector3 pos; //控件初始位置
private Vector3 mousePos; //鼠标初始位置
private void Start()
{
rectTransform = GetComponent<RectTransform>();
}
public void OnBeginDrag(PointerEventData eventData)
{
Debug.Log("开始拖拽");
pos = this.GetComponent<RectTransform>().position;
RectTransformUtility.ScreenPointToWorldPointInRectangle(rectTransform, eventData.position, eventData.pressEventCamera, out mousePos);
}
public void OnDrag(PointerEventData eventData)
{
Vector3 newVec;
RectTransformUtility.ScreenPointToWorldPointInRectangle(rectTransform, eventData.position, eventData.pressEventCamera, out newVec);
Vector3 offset = new Vector3(newVec.x - mousePos.x, newVec.y - mousePos.y, 0);
rectTransform.position = pos + offset;
}
public void OnEndDrag(PointerEventData eventData)
{
Debug.Log("结束拖拽");
}
}
2、效果如下
方法三:RectTransformUtility.ScreenPointToLocalPointInRectangle 并带位移 Offset
1、代码如下
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.EventSystems;
public class DragHandlerUGUI : MonoBehaviour, IBeginDragHandler, IDragHandler, IEndDragHandler
{
private Vector3 pos; //控件初始位置
private Vector2 mousePos; //鼠标初始位置
private RectTransform canvasRec; //控件所在画布
private void Start()
{
canvasRec = this.GetComponentInParent<Canvas>().transform as RectTransform;
}
public void OnBeginDrag(PointerEventData eventData)
{
Debug.Log("开始拖拽");
//控件所在画布空间的初始位置
pos = this.GetComponent<RectTransform>().anchoredPosition;
//将屏幕空间鼠标位置eventData.position转换为鼠标在画布空间的鼠标位置
RectTransformUtility.ScreenPointToLocalPointInRectangle(canvasRec, eventData.position, eventData.pressEventCamera, out mousePos);
}
public void OnDrag(PointerEventData eventData)
{
Vector2 newVec;
RectTransformUtility.ScreenPointToLocalPointInRectangle(canvasRec, eventData.position, eventData.pressEventCamera, out newVec);
//鼠标移动在画布空间的位置增量
Vector3 offset = new Vector3(newVec.x - mousePos.x, newVec.y - mousePos.y, 0);
//原始位置增加位置增量即为现在位置
(this.transform as RectTransform).anchoredPosition = pos + offset;
}
public void OnEndDrag(PointerEventData eventData)
{
Debug.Log("结束拖拽");
}
}
2、效果如下
方法四:Camera.WorldToScreenPoint 和 Camera.WorldToScreenPoint 并带位移 Offset
1、代码如下
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.EventSystems;
public class DragHandlerUGUI : MonoBehaviour, IBeginDragHandler, IDragHandler, IEndDragHandler
{
Vector3 uiScreenPosition;
Vector3 mouseScreenPosition;
Vector3 mScreenPosition;
private Vector3 offset;
Camera mCamera;
// Start is called before the first frame update
void Start()
{
mCamera = Camera.main;
}
public void OnBeginDrag(PointerEventData eventData)
{
Debug.Log("开始拖拽");
//转换对象到当前屏幕位置
uiScreenPosition = mCamera.WorldToScreenPoint(transform.position);
mouseScreenPosition = mCamera.WorldToScreenPoint(eventData.position);
//鼠标屏幕坐标
mScreenPosition = new Vector3(mouseScreenPosition.x, mouseScreenPosition.y, uiScreenPosition.z);
//获得鼠标和对象之间的偏移量,拖拽时相机应该保持不动
offset = transform.position - mCamera.ScreenToWorldPoint(mScreenPosition);
}
public void OnDrag(PointerEventData eventData)
{
mouseScreenPosition = mCamera.WorldToScreenPoint(eventData.position);
//鼠标屏幕上新位置
mScreenPosition = new Vector3(mouseScreenPosition.x, mouseScreenPosition.y, uiScreenPosition.z);
// 对象新坐标
transform.position = offset + mCamera.ScreenToWorldPoint(mScreenPosition);
}
public void OnEndDrag(PointerEventData eventData)
{
Debug.Log("结束拖拽");
}
}
2、效果如下