一、类属性
类中的属性:对象属性、类属性(类的字段)
1.类属性
直接定义在类中的变量就是类属性
类属性的值不会因为对象不同而不一样
2.对象属性
通过 self.属性名 = 值 定义在init函数中的属性
对象属性的值会因为对象不同而不一样
class Person:
num = 61
x = 100
print(Person.num)
Person.num = 60
二、对象属性的增删改查
class Student:
def __init__(self, name, age=0, gender='男', score=0):
self.name = name
self.age = age
self.gender = gender
self.score = score
def __repr__(self):
return f'<{str(self.__dict__)[1:-1]}>'
stu1 = Student('小明')
stu2 = Student('小花', gender='女')
1.查(获取属性的值)
对象.属性 - 获取对象指定属性对应的值;属性不存在会报错
getattr(对象, 属性名) - 获取对象指定属性对应的值;属性不存在会报错
getattr(对象, 属性名, 默认值) - 获取对象指定属性对应的值; 属性不存在不会报错,并且返回默认值
print(stu1.name)
# print(stu1.name1) # AttributeError: 'Student' object has no attribute 'name1'
print(getattr(stu1, 'name')) # 小明
print(getattr(stu1, 'age')) # 0
# print(getattr(stu1, 'name1')) # AttributeError: 'Student' object has no attribute 'name1'
print(getattr(stu1, 'name', '无名氏')) # 小明
print(getattr(stu1, 'name1', '无名氏')) # 无名氏
# 根据输入的内容获取对象属性的值
# name -> 小明
# age -> 0
# value = input('请选择(name,age,gender,score):')
# print(getattr(stu1, value))
2.改、增
对象.属性 = 值 - 当属性不存在就给对象添加属性,属性存在的时候就修改指定属性的值
setattr(对象, 属性名, 值) - 当属性不存在就给对象添加属性,属性存在的时候就修改指定属性的值
# 属性存在是修改
stu1.name = 'xiaoming'
print(stu1)
stu1.height = 180
print(stu1) # <'name': 'xiaoming', 'age': 0, 'gender': '男', 'score': 0, 'height': 180>
print(stu1.height)
setattr(stu1, 'age', 18)
print(stu1) # <'name': 'xiaoming', 'age': 18, 'gender': '男', 'score': 0, 'height': 180>
setattr(stu1, 'weight', 80)
print(stu1) # <'name': 'xiaoming', 'age': 18, 'gender': '男', 'score': 0, 'height': 180, 'weight': 80>
print(stu2) # <'name': '小花', 'age': 0, 'gender': '女', 'score': 0>
3.删
del 对象.属性 - 删除对象中指定的属性
delattr(对象, 属性名) - 删除对象中指定的属性
del stu2.age # <'name': '小花', 'gender': '女', 'score': 0>
print(stu2)
delattr(stu2, 'gender')
print(stu2) # <'name': '小花', 'score': 0>
三、内置属性
# python在定义类的时候系统自动添加的属性(从基类中继承下来的属性)就是内置属性
class Student:
def __init__(self, name, age=18, score=0):
self.name = name
self.age = age
self.score = score
class Dog:
# 类属性
num = 100
# 对象属性
def __init__(self, name, age=1, color='白色'):
self.name = name
self.age = age
self.color = color
# 方法
def eat(self, food):
print(f'{self.name}在吃{food}')
@classmethod
def show_num(cls):
print(f'狗的数量:{cls.num}')
@staticmethod
def bark():
print('狗嗷嗷叫~')
def __repr__(self):
c = self.__class__
m = c.__module__
return f'<{m}模块.{c.__name__}类的对象: name={self.name},age={self.age},color={self.color}>'
dog1 = Dog('大黄')
1. module
类属性;
类.module - 获取定义的模块的模块名
print(Dog.__module__)
print(int.__module__)
2.class
对象.class - 获取对象对应的类
c = dog1.__class__
print(c)
print(type(dog1))
print(Dog)
3.name
类.name - 获取类名
print(Dog.__name__) # 'Dog'
# 重写 repr 方法, 要求以: <xxx模块.xxx类的对象: 属性1=值1,属性2=值2>
4.dict
类.dict - 将类转换成字典(类的类属性名作为key,类属性的值作为值)
对象.dict - 将对象转换成字典(对象属性名作为key,属性的值作为值)
print(Dog.__dict__)
print(dog1.__dict__)
5. doc
类.doc - 获取类的说明文档
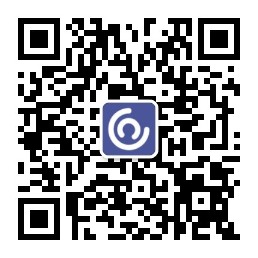
print(list.__doc__)
6.base、bases
类.base - 获取当前类的父类
类.bases - 获取当前类所有的父类
print(Dog.__base__) # <class 'object'>
print(Dog.__bases__) # (<class 'object'>,)
7. slots
class Person:
# __slots__可以约束当前类的对象能够拥有哪些对象属性
__slots__ = ('name', 'age', 'height')
def __init__(self, name, age=10):
self.name = name
self.age = age
p1 = Person('小明')
# p1.name1 = 'xiaoming'
p1.height = 180
# 注意: 如果给类属性__slots__赋值了,那么这个类的对象不能再使用__dict__属性
# print(p1.__dict__)
六、私有化
1.访问权限(针对属性和方法)
公开的:在类的内部和外部都可以使用,也能被继承
保护的:在类的内部可以使用,类的外部不能使用,可以被继承
私有的:只能在类的内部使用,不能被继承
严格来说,python中所有的属性和方法都是公开的,这儿说的私有化其实是假的私有化
class Person:
num = 100
__num2 = 61
def __init__(self):
self.name = '小明'
self.age = 10
self.__gender = '男'
def eat(self):
print(f'{self.name}在吃饭')
print(self.__gender)
p1 = Person()
print(Person.num)
print(p1.name, p1.age)
p1.eat()
# print(p1.__gender)
# print(Person.__num2)
私有化的原理:
print(p1.__dict__) # {'name': '小明', 'age': 10, '_Person__gender': '男'}
print(p1._Person__gender)
七、getter和setter
1.getter和setter的作用
getter作用:在获取某个属性值之前想要做别的事情,就给这个属性添加getter
setter作用:在给属性赋值之前想要做别的事情,就给这个属性添加setter
2.怎么添加getter和setter
1)getter
第一步:在需要添加getter的属性名前加_
第二步:定义getter对应的函数(1.需要@property装饰器 2.函数名就是不带_的属性名 3.函数需要一个返回值)
第三步:获取属性值的通过: 对象.不带_属性名 (本质就是在调用getter对应的函数,取到属性值就是函数的返回值)
2)setter
如果想要给属性添加setter必须先给属性添加getter
第一步:添加getter
第二步:定义setter对应的函数 (1.需要 @getter函数名.setter 装饰器 2.函数名就是不带_的属性名 3.需要一个参数不需要返回值,这个参数就是尝试给属性赋的值)
第三步:给属性赋值:对象.不带_属性名 = 值 (本质就是在调用setter对应的函数)
class Rect:
def __init__(self, length=0, width=0):
self.length = length
self.width = width
self._area = length*width
@property
def area(self):
print('area属性值被获取')
self._area = self.width * self.length
return self._area
@area.setter
def area(self, value):
# print(f'value:{value}')
# self._area = value
raise ValueError
r1 = Rect(4, 5)
print('==========')
print(r1.area)
print('++++++++')
# 不能让矩形直接修改面积属性值
# r1.area = 100
# print(r1.__dict__)
#
r1.width = 10
print(r1.area)
r1.length = 10
print(r1.area)
r1.area = 80