springboot是一个全新的框架,被设计用来简化spring的配置 。我们知道,以前我们使用spring来做开发时,需要做很多模板化的配置来完成基础功能的搭建,就算是一个很小的项目,也需要进行很多必需的配置才能使用spring的功能,显然这受到很多spring使用者的吐槽!!!那springboot的出现就是用来解决这个问题的,springboot框架使用自己独特的方式进行配置,使开发人员不再需要做模板化的配置,将所有的关注点全部集中在功能开发,所有的配置都交给springboot来完成。
下面我们来完成一个springboot版的hellowold
1.引入springboot相关依赖
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>2.0.0.RELEASE</version>
<relativePath/>
</parent>
开发Web程序需要用到spring-boot-starter-web包
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
</dependencies>
下面是Java代码:
package com.example.demo;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.EnableAutoConfiguration;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
@RestController
@EnableAutoConfiguration
public class SpringbootexampleApplication {
@RequestMapping("/")
String index(){
return "HelloWorld!";
}
public static void main(String[] args) {
SpringApplication.run(SpringbootexampleApplication.class, args);
}
}
@RestController 表示该类是一个Web Controller,可以用来接收Web请求
@EnableAutoConfiguration 表示开启springboot的自动配置功能,springboot会“猜测”你可能会用到哪些jar包,并且自动引入。比如,本例中加入spring-boot-starter-web依赖包,springboot就会自动引入tomcat、springMVC相关的jar包,并自动进行Web项目相关配置
本例中有main方法,可以像运行普通的Java main方法一样运行springboot,springboot提供SpringApplication.run()方法来启动应用程序
运行结果如下:
. ____ _ __ _ _
/\\ / ___'_ __ _ _(_)_ __ __ _ \ \ \ \
( ( )\___ | '_ | '_| | '_ \/ _` | \ \ \ \
\\/ ___)| |_)| | | | | || (_| | ) ) ) )
' |____| .__|_| |_|_| |_\__, | / / / /
=========|_|==============|___/=/_/_/_/
:: Spring Boot :: (v2.0.0.RELEASE)
2018-03-21 21:22:31.310 INFO 11596 --- [ main] c.e.demo.SpringbootexampleApplication : Starting SpringbootexampleApplication on PC-PZJ with PID 11596 (E:\workspace\springbootexample\target\classes started by arno.peng in E:\workspace\springbootexample)
2018-03-21 21:22:31.326 INFO 11596 --- [ main] c.e.demo.SpringbootexampleApplication : No active profile set, falling back to default profiles: default
2018-03-21 21:22:31.451 INFO 11596 --- [ main] ConfigServletWebServerApplicationContext : Refreshing org.springframework.boot.web.servlet.context.AnnotationConfigServletWebServerApplicationContext@b7f23d9: startup date [Wed Mar 21 21:22:31 CST 2018]; root of context hierarchy
2018-03-21 21:22:33.263 INFO 11596 --- [ main] o.s.b.w.embedded.tomcat.TomcatWebServer : Tomcat initialized with port(s): 8080 (http)
2018-03-21 21:22:33.295 INFO 11596 --- [ main] o.apache.catalina.core.StandardService : Starting service [Tomcat]
....
...中间部分省略
...
org.springframework.boot.autoconfigure.web.servlet.error.BasicErrorController.error(javax.servlet.http.HttpServletRequest)
2018-03-21 21:22:33.935 INFO 11596 --- [ main] s.w.s.m.m.a.RequestMappingHandlerMapping : Started SpringbootexampleApplication in 3.547 seconds (JVM running for 5.034)
2018-03-21 21:22:47.758 INFO 11596 --- [nio-8080-exec-1] o.a.c.c.C.[Tomcat].[localhost].[/] : Initializing Spring FrameworkServlet 'dispatcherServlet'
2018-03-21 21:22:47.758 INFO 11596 --- [nio-8080-exec-1] o.s.web.servlet.DispatcherServlet : FrameworkServlet 'dispatcherServlet': initialization started
2018-03-21 21:22:47.778 INFO 11596 --- [nio-8080-exec-1] o.s.web.servlet.DispatcherServlet : FrameworkServlet 'dispatcherServlet': initialization completed in 20 ms
从启动信息中,我们可以看到,springboot会默认帮我们启动内置的tomcat容器,端口号是8080
访问:http://localhost:8080/
可以看到,我们并没有进行任何的配置,就可以完整的运行起来,这就是springboot的零配置!
springboot还支持java -jar的方式来运行,一般生产环境会使用这种方式来运行
首先在pom.xml文件中加入maven插件的支持
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
</plugin>
</plugins>
</build>
然后在命令行执行打包命令
$ mvn package
打包成功后,会显示如下的信息:
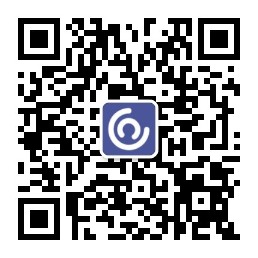
[INFO]
[INFO] ------------------------------------------------------------------------
[INFO] Building springbootexample 0.0.1-SNAPSHOT
[INFO] ------------------------------------------------------------------------
......中间部分省略
[INFO] ------------------------------------------------------------------------
[INFO] BUILD SUCCESS
[INFO] ------------------------------------------------------------------------
[INFO] Total time: 3:58.358s
[INFO] Finished at: Thu Mar 22 21:31:43 CST 2018
[INFO] Final Memory: 24M/88M
[INFO] ------------------------------------------------------------------------
此时,在你项目的target目录下会生成对应的jar文件,这就是可以直接运行的jar文件,里面包含项目所有的依赖以及内嵌的tomcat容器
执行命令
java -jar springbootexample-0.0.1-SNAPSHOT.jar
会出现跟我们之前直接运行一样的输出:
/\\ / ___'_ __ _ _(_)_ __ __ _ \ \ \ \
( ( )\___ | '_ | '_| | '_ \/ _` | \ \ \ \
\\/ ___)| |_)| | | | | || (_| | ) ) ) )
' |____| .__|_| |_|_| |_\__, | / / / /
=========|_|==============|___/=/_/_/_/
:: Spring Boot :: (v2.0.0.RELEASE)
2018-03-22 21:38:25.026 INFO 2268 --- [ main] c.e.demo.SpringbootexampleApplication : Starting SpringbootexampleApplication v0.0.1-SNAPSHOT on PC-PZJ with PID 2268 (E:\workspace\springbootexample\target\springbootexample-0.0.1-SNAPSHOT.jar started by arno.peng in E:\workspace\springbootexample\target)
2018-03-22 21:38:25.032 INFO 2268 --- [ main] c.e.demo.SpringbootexampleApplication : No active profile set, falling back to default profiles: default
2018-03-22 21:38:25.148 INFO 2268 --- [ main] ConfigServletWebServerApplicationContext : Refreshing org.springframework.boot.web.servlet.context.AnnotationConfigServletWebServerApplicationContext@75828a0f: startup date [Thu Mar 22 21:38:25 CST 2018]; root of context hierarchy
2018-03-22 21:38:27.725 INFO 2268 --- [ main] o.s.b.w.embedded.tomcat.TomcatWebServer : Tomcat initialized with port(s): 8080 (http)
2018-03-22 21:38:27.776 INFO 2268 --- [ main] o.apache.catalina.core.StandardService : Starting service [Tomcat]
2018-03-22 21:38:27.776 INFO 2268 --- [ main] org.apache.catalina.core.StandardEngine : Starting Servlet Engine: Apache Tomcat/8.5.28
.....
中间部分省略
.....
2018-03-22 21:38:29.188 INFO 2268 --- [ main] o.s.w.s.handler.SimpleUrlHandlerMapping : Mapped URL path [/webjars/**] onto handler of type [class org.springframework.web.servlet.resource.ResourceHttpRequestHandler]
2018-03-22 21:38:29.189 INFO 2268 --- [ main] o.s.w.s.handler.SimpleUrlHandlerMapping : Mapped URL path [/**] onto handler of type [class org.springframework.web.servlet.resource.ResourceHttpRequestHandler]
2018-03-22 21:38:29.262 INFO 2268 --- [ main] o.s.w.s.handler.SimpleUrlHandlerMapping : Mapped URL path [/**/favicon.ico] onto handler of type [class org.springframework.web.servlet.resource.ResourceHttpRequestHandler]
2018-03-22 21:38:29.530 INFO 2268 --- [ main] o.s.j.e.a.AnnotationMBeanExporter : Registering beans for JMX exposure on startup
2018-03-22 21:38:29.623 INFO 2268 --- [ main] o.s.b.w.embedded.tomcat.TomcatWebServer : Tomcat started on port(s): 8080 (http) with context path ''
2018-03-22 21:38:29.629 INFO 2268 --- [ main] c.e.demo.SpringbootexampleApplication : Started SpringbootexampleApplication in 5.29 seconds (JVM running for 6.002)
此时,在浏览器输入 http://localhost:8080 会得到同样的输出