G - Shuffle'm Up
A common pastime for poker players at a poker table is to shuffle stacks of chips. Shuffling chips is performed by starting with two stacks of poker chips, S1 and S2, each stack containing C chips. Each stack may contain chips of several different colors.
The actual shuffle operation is performed by interleaving a chip from S1 with a chip from S2 as shown below for C = 5:
The single resultant stack, S12, contains 2 * C chips. The bottommost chip of S12 is the bottommost chip from S2. On top of that chip, is the bottommost chip from S1. The interleaving process continues taking the 2nd chip from the bottom of S2 and placing that on S12, followed by the 2nd chip from the bottom of S1 and so on until the topmost chip from S1 is placed on top of S12.
After the shuffle operation, S12 is split into 2 new stacks by taking the bottommost C chips from S12 to form a new S1 and the topmost C chips from S12 to form a new S2. The shuffle operation may then be repeated to form a new S12.
For this problem, you will write a program to determine if a particular resultant stack S12 can be formed by shuffling two stacks some number of times.
The first line of input contains a single integer N, (1 ≤ N ≤ 1000) which is the number of datasets that follow.
Each dataset consists of four lines of input. The first line of a dataset specifies an integer C, (1 ≤ C ≤ 100) which is the number of chips in each initial stack (S1 and S2). The second line of each dataset specifies the colors of each of the C chips in stack S1, starting with the bottommost chip. The third line of each dataset specifies the colors of each of the C chips in stack S2 starting with the bottommost chip. Colors are expressed as a single uppercase letter (A through H). There are no blanks or separators between the chip colors. The fourth line of each dataset contains 2 * C uppercase letters (A through H), representing the colors of the desired result of the shuffling of S1 and S2 zero or more times. The bottommost chip’s color is specified first.
Output for each dataset consists of a single line that displays the dataset number (1 though N), a space, and an integer value which is the minimum number of shuffle operations required to get the desired resultant stack. If the desired result can not be reached using the input for the dataset, display the value negative 1 (−1) for the number of shuffle operations.
2 4 AHAH HAHA HHAAAAHH 3 CDE CDE EEDDCCSample Output
1 2 2 -1
思路:以s2的最底端为s12的最底端,从s1和s2中交叉取值,如果组成的排列与题目最初给的相符合,就输出洗牌的次数,如果不相符合就继续洗牌,让新的s1从s12的最底端开始取值,新的s2是新的s1取剩下的值,
如果洗牌顺序不会和题目给出一致,就输出 -1
#include<stdio.h> #include<stdlib.h> #include<iostream> #include<string.h> #include<map> #include<queue> using namespace std; int main() { int t=0,T,c,cnt,len; bool flag; char s1[110],s2[110],s12[220],s[220]; // 注意s数组的大小,要和s12数组的大小一致,不要开小了 scanf("%d",&T); for(int i=0;i<T;i++) { scanf("%d",&c); scanf("%s%s%s",s1,s2,s12); map<string,int>mp; cnt=0; t++; flag=false; while(1) { len=0; cnt++; for(int j=0;j<c;j++) { s[len]=s2[j]; len++; s[len]=s1[j]; len++; } s[len]='\0'; if(strcmp(s,s12)==0) { flag=true; break; } if(mp.find(s)!=mp.end()) // map中元素的查找 // find()函数返回一个迭代器指向键值为key的元素, // 如果没找到就返回指向map尾部的迭代器。 { break; } mp[s]=0; for(int j=0;j<c;j++) { s1[j]=s[j]; s2[j]=s[j+c]; } } if(flag) { printf("%d %d\n",t,cnt); } else { printf("%d -1\n",t); } } return 0; }
map知识
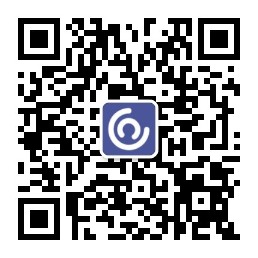
map最基本的构造函数
map<string ,int>mapstring;
map<int,string >mapint;
map<sring,char>mapstring;
map< char ,string>mapchar;
map<char,int>mapchar;
map<int ,char>mapint;
//这几个是最基本的,当然还有其它的构造函数的
- 1
- 2
- 3
- 4
- 5
- 6
- 7
向map中添加数据
使用insert方法来添加数据
map<int ,string>maplive;
1. maplive.insert(pair<int,string>(102,"aclive"));
2. maplive.insert(map<int,string>::value_type(321,"hai"));
3. maplive[112]="April";//map中最简单最常用的插入添加!
- 1
- 2
- 3
- 4
map中元素的查找
find()函数返回一个迭代器指向键值为key的元素,如果没找到就返回指向map尾部的迭代器。
map<int ,string >::iterator l_it;
l_it=maplive.find(112);//返回的是一个指针
if(l_it==maplive.end())
cout<<"we do not find112"<<endl;
else
cout<<"wo find112"<<endl;
map<string,string>m;
if(m[112]=="")
cout<<"we do not find112"<<endl;
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
- 11
- 12
- 13
map中元素的删除
map<int ,string>::iterator l_it;;
l_it =maplive.find(112);
if( l_it == maplive.end())
cout<<"we do not find112"<<endl;
else
maplive.erase(l_it);//delete 112;
- 1
- 2
- 3
- 4
- 5
- 6
* map中 swap的用法*
Map中的swap不是一个容器中的元素交换,而是两个容器交换
#include<map>
#include<iostream>
usingnamespace std;
int main( )
{
map<int, int> m1;
map <int,int>::iterator m1_Iter;
m1.insert (pair <int, int> (1, 20 ) );
m1.insert ( pair<int, int> ( 4, 40) );
m1.insert ( pair<int, int> ( 3, 60) );
m1.insert ( pair<int, int> ( 2, 50) );
m1.insert ( pair<int, int> ( 6, 40) );
m1.insert ( pair<int, int> ( 7, 30) );
cout<< "The original map m1is:"<<endl;
for ( m1_Iter = m1.begin( );m1_Iter != m1.end( ); m1_Iter++ )
cout << m1_Iter->first<<""<<m1_Iter->second<<endl;
}
The original map m1 is:
1 20
2 50
3 60
4 40
6 40
7 30
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
- 11
- 12
- 13
- 14
- 15
- 16
- 17
- 18
- 19
- 20
- 21
- 22
- 23
- 24
- 25
- 26
- 27
- 28
- 29
- 30
- 31
map的基本操作函数
Maps 是一种关联式容器,包含“关键字/值”对
begin() 返回指向map头部的迭代器
clear() 删除所有元素
count() 返回指定元素出现的次数
empty() 如果map为空则返回true
end() 返回指向map末尾的迭代器
equal_range() 返回特殊条目的迭代器对
erase() 删除一个元素
find() 查找一个元素
get_allocator() 返回map的配置器
insert() 插入元素
key_comp() 返回比较元素key的函数
lower_bound() 返回键值>=给定元素的第一个位置
max_size() 返回可以容纳的最大元素个数
rbegin() 返回一个指向map尾部的逆向迭代器
rend() 返回一个指向map头部的逆向迭代器
size() 返回map中元素的个数
swap() 交换两个map
upper_bound() 返回键值>给定元素的第一个位置
value_comp() 返回比较元素value的函数
multimap
//multimap允许重复的键值插入容器
// **********************************************************
// * pair只包含一对数值:pair<int,char> *
// * map是一个集合类型,永远保持排好序的, *
// pair * map每一个成员就是一个pair,例如:map<int,char> *
// * map的insert()可以把一个pair对象作为map的参数,例如map<p> *
// ***********************************************************
#pragma warning(disable:4786)
#include<map>
#include<iostream>
using namespace std;
int main(void)
{
multimap<int,char*> m;
//multimap的插入只能用insert()不能用数组
m.insert(pair<int,char*>(1,"apple"));
m.insert(pair<int,char*>(1,"pear"));//apple和pear的价钱完全有可能是一样的
m.insert(pair<int,char*>(2,"banana"));
//multimap的遍历只能用迭代器方式不能用数组
cout<<"***************************************"<<endl;
multimap<int,char*>::iterator i,iend;
iend=m.end();
for(i=m.begin();i!=iend;i++)
{
cout<<(*i).second<<"的价钱是"
<<(*i).first<<"元/斤\n";
}
cout<<"***************************************"<<endl;
//元素的反相遍历
multimap<int,char*>::reverse_iterator j,jend;
jend=m.rend();
for(j=m.rbegin();j!=jend;j++)
{
cout<<(*j).second<<"的价钱是"
<<(*j).first<<"元/斤\n";
}
cout<<"***************************************"<<endl;
//元素的搜索find(),pair<iterator,iterator>equal_range(const key_type &k)const
//和multiset的用法一样
multimap<int,char*>::iterator s;
s=m.find(1);//find()只要找到一个就行了,然后立即返回。
cout<<(*s).second<<" "
<<(*s).first<<endl;
cout<<"键值等于1的元素个数是:"<<m.count(1)<<endl;
cout<<"***************************************"<<endl;
//删除 erase(),clear()
m.erase(1);
for(i=m.begin();i!=iend;i++)
{
cout<<(*i).second<<"的价钱是"
<<(*i).first<<"元/斤\n";
}
return 0;
}