package com.Mysql02;
import java.util.Date;
public class user {
//user类封装用户数据
private int id;
private String user;
private String password;
private String email;
private Date birthday;
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
public String getUser() {
return user;
}
public void setUser(String user) {
this.user = user;
}
public String getPassword() {
return password;
}
public void setPassword(String password) {
this.password = password;
}
public String getEmail() {
return email;
}
public void setEmail(String email) {
this.email = email;
}
public Date getBirthday() {
return birthday;
}
public void setBirthday(Date birthday) {
this.birthday = birthday;
}
}
package com.Mysql02;
import java.sql.*;
public class JDBCUtils {
//封装成工具类
//获取连接对象的方法
public static Connection getcon() throws Exception {
//注册并加载驱动
Class.forName("com.mysql.jdbc.Driver");
//获取连接对象
Connection con = DriverManager.getConnection("jdbc:mysql://localhost:3306/jdbc", "root", "1111");
return con;
}
public static void realse(ResultSet rs, Statement st, Connection con) {
{
//6.关闭资源,释放资源
if (rs != null) {
try {
rs.close();
} catch (SQLException e) { // ignore }
rs = null;
}
}
if (st != null) {
try {
st.close();
} catch (SQLException e) { // ignore }
st = null;
}
}
if (con != null) {
try {
con.close();
} catch (SQLException e) { // ignore }
rs = null;
}
}
}
}
}
package com.Mysql02;
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.Statement;
import java.util.ArrayList;
import java.util.Date;
import java.util.List;
public class userText {
Connection con = null;
Statement st = null;
ResultSet rs = null;
//实现添加方法
public boolean insert(user user) {
try {
//获取加载驱动并且连接对象
con = JDBCUtils.getcon();
st = con.createStatement();
Date birthday = user.getBirthday();
String sqlbirthday = String.format("%tF", birthday);
String sql = "insert into message(id,user,password,email,birthday)" + "values ('"
+ user.getId() + "','"
+ user.getUser() + "','"
+ user.getPassword() + "','"
+ user.getEmail() + "','"
+ sqlbirthday + "'"
+ ")";
int row = st.executeUpdate(sql);
if (row > 0) {
return true;
}
} catch (Exception e) {
e.printStackTrace();
} finally {
JDBCUtils.realse(null, st, con);
}
return false;
}
//查找所有用户
public List<user> findAllUser() {
try {
con = JDBCUtils.getcon();
st = con.createStatement();
//执行sql语句
rs = st.executeQuery("select * from message");
//遍历结果集
List<user> list = new ArrayList<user>();
user user = new user();
while (rs.next()) {
java.sql.Date birthday = rs.getDate("birthday");
user.setId(rs.getInt(1));
user.setUser(rs.getString(2));
user.setPassword(rs.getString(3));
user.setEmail(rs.getString(4));
user.setBirthday(birthday);
list.add(user);
}
return list;
} catch (Exception e) {
e.printStackTrace();
} finally {
JDBCUtils.realse(rs, st, con);
}
return null;
}
//根据Id查找用户
public user findUserById(int id) {
{
try {
PreparedStatement st = null;
con = JDBCUtils.getcon();
String sql = "select * from message where id=?";
st = con.prepareStatement(sql);
//执行sql语句
st.setInt(1, id);
rs = st.executeQuery();
//遍历结果集
user user = new user();
if (rs.next()) {
java.sql.Date birthday = rs.getDate("birthday");
user.setId(rs.getInt(1));
user.setUser(rs.getString(2));
user.setPassword(rs.getString(3));
user.setEmail(rs.getString(4));
user.setBirthday(birthday);
return user;
}
} catch (Exception e) {
e.printStackTrace();
} finally {
JDBCUtils.realse(rs, st, con);
}
return null;
}
}
//根据id值修改记录
public boolean update(user user) {
{
try {
PreparedStatement st = null;
con = JDBCUtils.getcon();
String sql = "update message set user =?,password=? where id=?";
st = con.prepareStatement(sql);
//执行sql语句 占位符赋值
st.setString(1, user.getUser());
st.setString(2, user.getPassword());
st.setInt(3, user.getId());
int row = st.executeUpdate();
if (row > 0) {
return true;
}
} catch (Exception e) {
e.printStackTrace();
} finally {
JDBCUtils.realse(null, st, con);
}
return false;
}
}
//根据ID删除用户
public boolean delete(int id) {
{
try {
PreparedStatement st = null;
con = JDBCUtils.getcon();
String sql = "delete from message where id=?";
st = con.prepareStatement(sql);
//执行sql语句
st.setInt(1, id);
int row = st.executeUpdate();
if (row > 0) {
return true;
}
} catch (Exception e) {
e.printStackTrace();
} finally {
JDBCUtils.realse(rs, st, con);
}
return false;
}
}
}
package com.Mysql02;
import java.util.Date;
public class userInsertText {
public static void main(String[] args) {
//实例化对象
userText userText = new userText();
user user = new user();
user.setId(1);
user.setUser("xinfeng");
user.setPassword("111111");
user.setEmail("[email protected]");
user.setBirthday(new Date());
userText.insert(user);
}
}
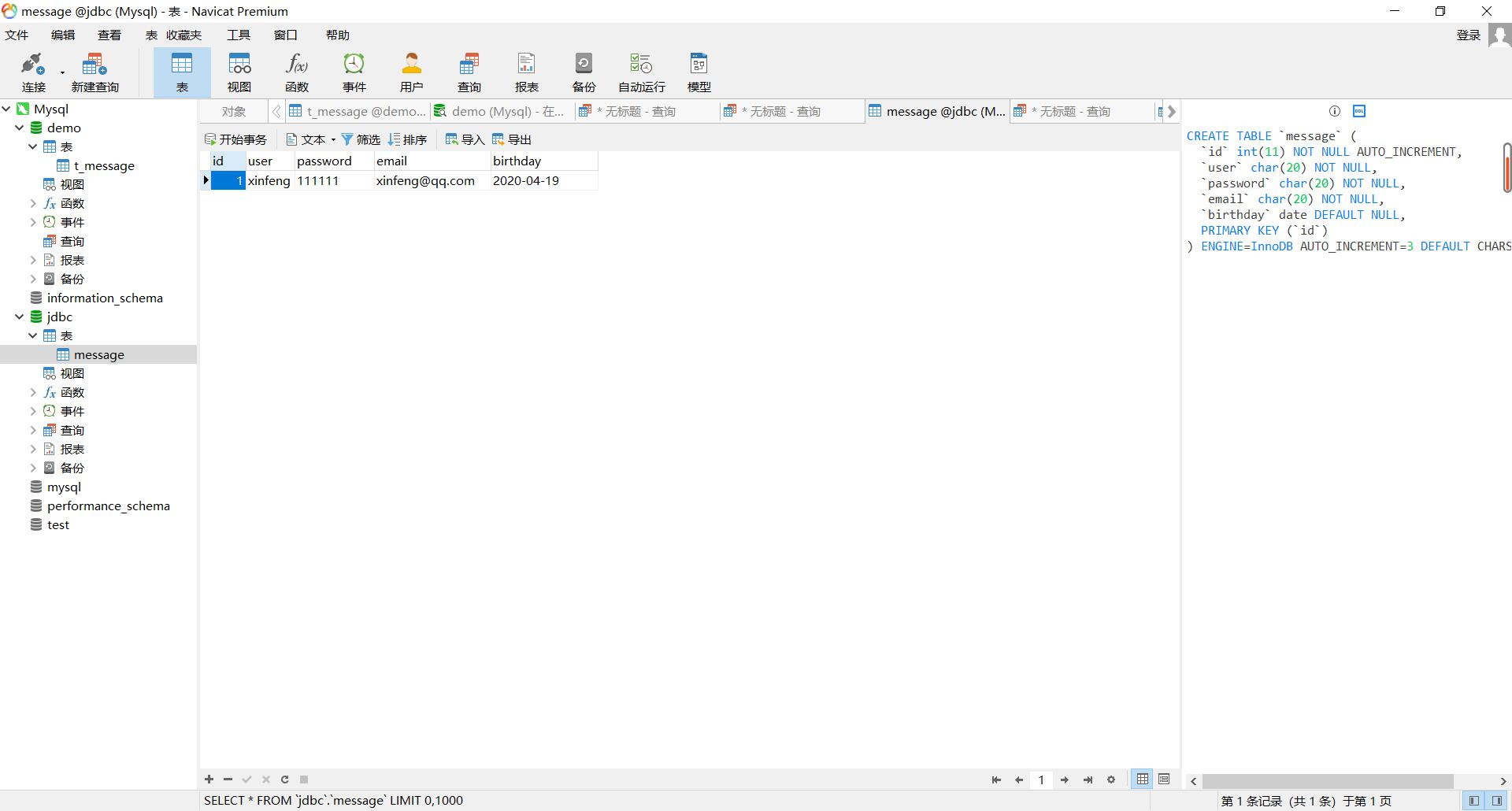
package com.Mysql02;
public class updateUser {
public static void main(String[] args) {
userText userText = new userText();
user user = new user();
user.setId(1);
user.setPassword("222222");
user.setUser("xinfeng");
userText.update(user);
}
}
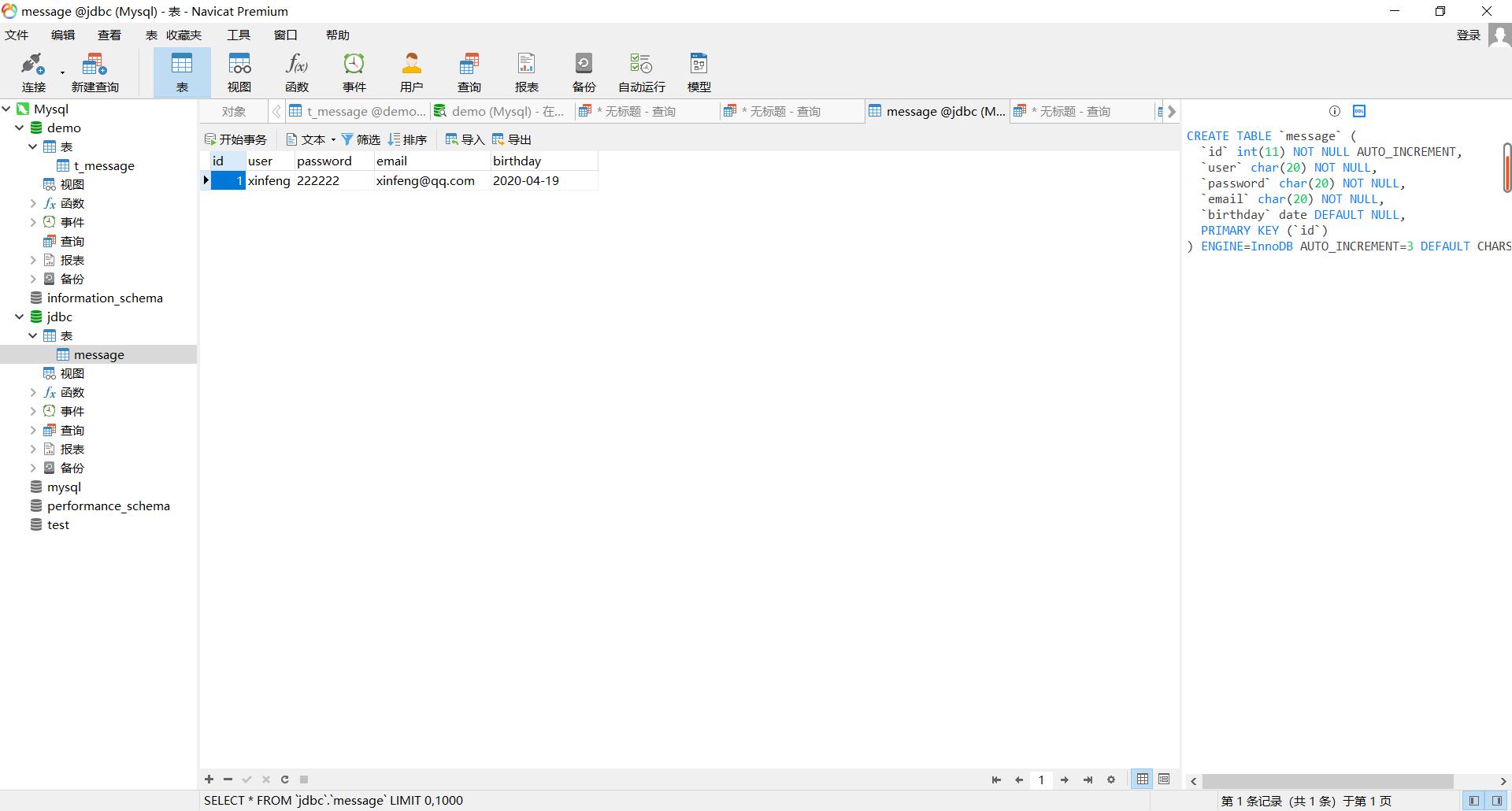
package com.Mysql02;
import java.util.List;
public class findAllUser {
public static void main(String[] args) {
userText userText = new userText();
List<user> list = userText.findAllUser();
System.out.println(list.size());
}
}
package com.Mysql02;
public class findUserById {
public static void main(String[] args) {
userText userText = new userText();
user u= userText.findUserById(1);
System.out.println(u.getUser());
}
}
package com.Mysql02;
public class deleteUser {
public static void main(String[] args) {
userText userText = new userText();
userText.delete(2);
}
}
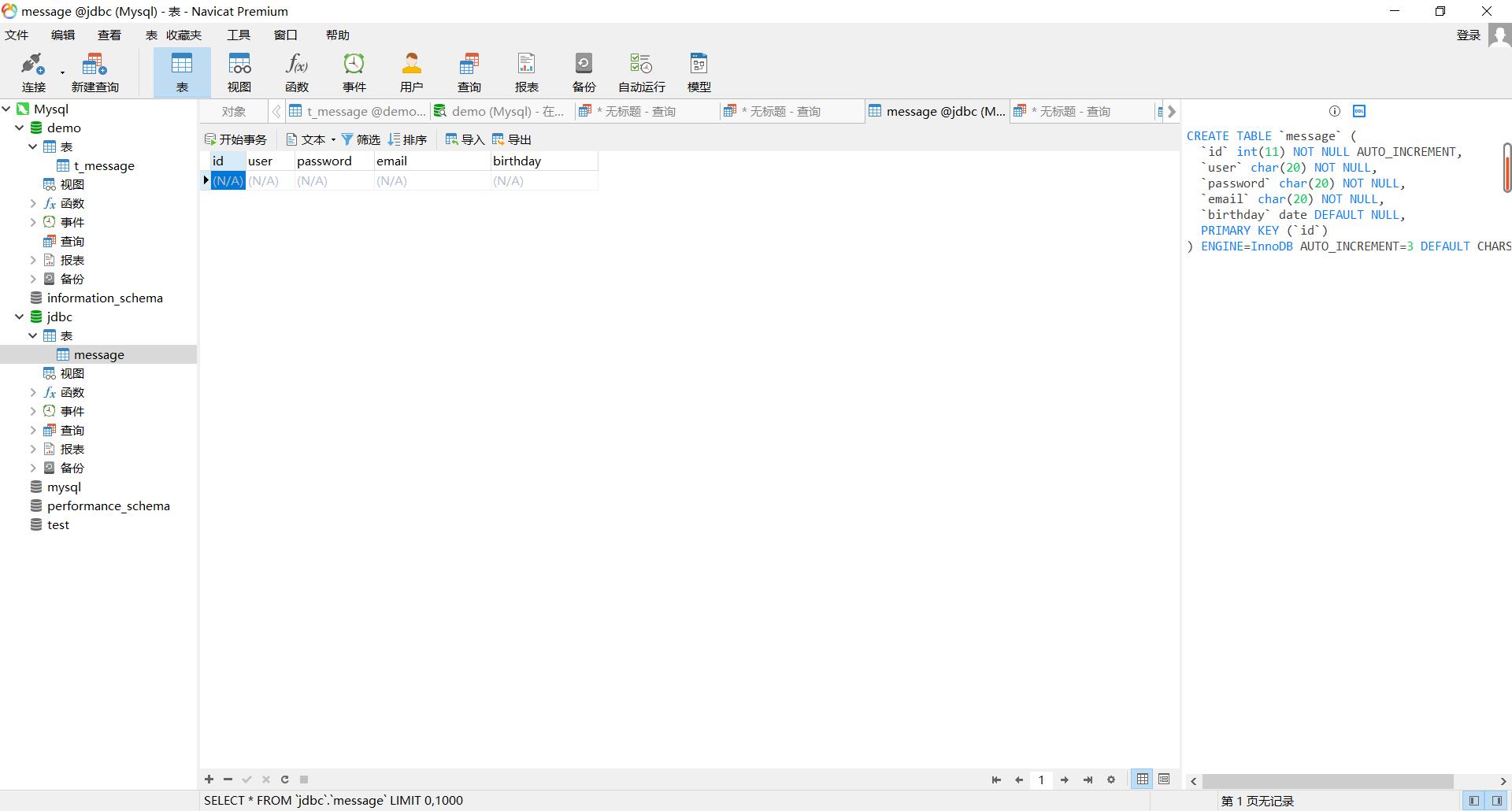
CREATE TABLE `message` (
`id` int(11) NOT NULL AUTO_INCREMENT,
`user` char(20) NOT NULL,
`password` char(20) NOT NULL,
`email` char(20) NOT NULL,
`birthday` date DEFAULT NULL,
PRIMARY KEY (`id`)