import tensorflow as tf
import numpy as np
import matplotlib.pyplot as plt
def loadDataSet(fileName,delim='\t'):
fr = open(fileName)
stringArr = [line.strip().split(delim) for line in fr.readlines()]
datArr = [list(map(float,line)) for line in stringArr]
return np.mat(datArr)
dataMat = loadDataSet("fileName")
trainX = dataMat[:,0]
trainY = dataMat[:,1]
m,n = np.shape(dataMat)
x = tf.placeholder(tf.float32,shape=[None,1])
y = tf.placeholder(tf.float32,shape=[None,1])
w1 = tf.Variable(tf.random_normal([1,20]))
w2 = tf.Variable(tf.random_normal([20,1]))
b1 = tf.Variable(tf.ones([m,20]))
b2 = tf.Variable(tf.ones([m,1]))
h = tf.nn.relu(tf.matmul(x,w1) + b1)
y_ = tf.nn.relu(tf.matmul(h,w2) + b2)
mse = tf.reduce_mean(tf.pow(y_-y,2))
train_step = tf.train.GradientDescentOptimizer(0.01).minimize(mse)
with tf.Session() as sess:
tf.global_variables_initializer().run()
for i in range(300):
sess.run(train_step,feed_dict={x:trainX,y:trainY})
y_pred = sess.run(y_,feed_dict={x:trainX})
mse = sess.run(mse,feed_dict={x:trainX,y:trainY})
print("均方误差为:",mse)
srtInd = trainX[:,0].argsort(0)
xSort = trainX[srtInd][:,0,:]
plt.plot(xSort[:,0],y_pred[srtInd][:,0],color='r',linewidth=3)
plt.scatter(trainX.tolist(),trainY.tolist(),edgecolors='k')
plt.grid()
plt.show()
均方误差为: 0.0018917992
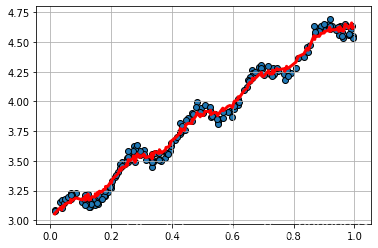