题目来自LeetCode,链接:括号生成。具体描述为:
数字 n 代表生成括号的对数,请你设计一个函数,用于能够生成所有可能的并且 有效的 括号组合。
示例:
输入:n = 3
输出:[
"((()))",
"(()())",
"(())()",
"()(())",
"()()()"
]
一看到这种括号的题目,应该能够条件反射般想到栈,栈可以用来判断/生成合法的括号对。这里就是用栈来生成合法括号对,当然,熟悉的同学也应该知道这种题目我们不用真的实现一个栈,因为栈里面放的永远都是左括号,所以可以直接用一个变量记录栈的长度来代替真正实例化一个栈。具体的做法就是根据括号对中已有的左括号数leftNum以及当前栈长度做判断:
- 栈为空:只能在括号对中加入左括号,且栈长度+1;
- 栈非空:
- leftNum == n:说明只能在括号对中加入右括号了(加的次数刚好就是当前栈长度);
- leftNum < n:说明可以加左括号(leftNum+1,栈长度+1)也可以加右括号(此时有一个匹配的左括号出栈,所以栈长度-1)。
JAVA版代码如下:
class Solution {
private List<String> result;
private void generate(char[] str, int idx, int n, int leftNum, int stackLen) {
if (stackLen == 0) {
// 栈空的情况下只能加左括号了
str[idx] = '(';
generate(str, idx + 1, n, leftNum + 1, 1);
}
else {
if (leftNum == n) {
// 左括号数满了,把右括号都给加上去
for (int i = 0; i < stackLen; ++i) {
str[idx + i] = ')';
}
result.add(new String(str));
}
else {
// 还可以加左括号或右括号
str[idx] = '(';
generate(str, idx + 1, n, leftNum + 1, stackLen + 1);
str[idx] = ')';
generate(str, idx + 1, n, leftNum, stackLen - 1);
}
}
}
public List<String> generateParenthesis(int n) {
result = new LinkedList<>();
generate(new char[n * 2], 0, n, 0, 0);
return result;
}
}
提交结果如下:
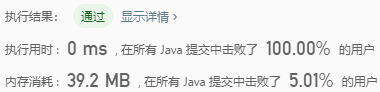
此外可以利用DFS,根据当前左括号数leftNum和右括号数rightNum做判断:
- leftNum < n:可以加左括号;
- rightNum < leftNum:可以加右括号
JAVA版代码如下:
class Solution {
private List<String> result;
private void dfs(char[] str, int idx, int leftNum, int rightNum, int n) {
if (idx == n * 2) {
result.add(new String(str));
return;
}
if (leftNum < n) {
str[idx] = '(';
dfs(str, idx + 1, leftNum + 1, rightNum, n);
}
// 右括号数小于左括号数的情况下才能加右括号
if (rightNum < leftNum) {
str[idx] = ')';
dfs(str, idx + 1, leftNum, rightNum + 1, n);
}
}
public List<String> generateParenthesis(int n) {
result = new LinkedList<>();
dfs(new char[n * 2], 0, 0, 0, n);
return result;
}
}
提交结果如下:
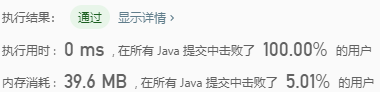
Python版代码如下:
class Solution:
def generateParenthesis(self, n: int) -> List[str]:
chars = [')' for _ in range(n * 2)]
result = []
def dfs(idx, leftNum, rightNum):
if idx == n * 2:
result.append(''.join(chars))
return
if leftNum < n:
chars[idx] = '('
dfs(idx + 1, leftNum + 1, rightNum)
if rightNum < leftNum:
chars[idx] = ')'
dfs(idx + 1, leftNum, rightNum + 1)
dfs(0, 0, 0)
return result
提交结果如下:
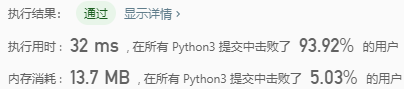