一、数据源
F12打开调试工具,选择 Network
→ XHR
→ list-total?t=317162642162
→ Headers
→ Request URL
获取到的链接如下:
https://c.m.163.com/ug/api/wuhan/app/data/list-total?t=317162642162
二、创建项目及环境
创建git仓库:g-disease,并使用勾选使用readme初始化仓库
克隆仓库到本地
git clone https://gitee.com/OliverDaDa/g-disease.git
在开发工具中打开工程目录,并初始化项目
npm init -y
cnpm i -D electron electron-builder
npx gitignore node
三、创建文件
新建index.html
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>今日疫情</title>
</head>
<body>
<div class="container">
<div class="row">
<h1 id="title">今日新增</h1>
<h1 id="number">获取中...</h1>
</div>
</div>
</body>
</html>
新建main.js
const { app, BrowserWindow } = require('electron')
function createWindow(){
let win = new BrowserWindow({
width:800,
height:600,
webPreferences:{
nodeIntegration: true
}
})
win.loadFile('./index.html')
}
app.whenReady().then(()=>createWindow())
安装插件nodemon
cnpm i -D nodemon
在package.json中配置nodemon命令,并将默认指定入口文件改为main.js
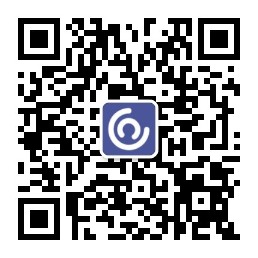
"main": "main.js",
"scripts": {
"start": "nodemon -e js,html --ignore ./package.json --exec electron ."
},
控制台输入:npm run start
运行程序
四、显示数据
在index.html的body结束标签前添加如下代码:
<script>
const title = document.querySelector('#title')
const number = document.querySelector('#number')
fetch('https://c.m.163.com/ug/api/wuhan/app/data/list-total?t=317162642162')
.then(res=>res.json()) //text()
.then(data=>{
const confirm = data.data.chinaTotal.today.confirm
number.innerHTML = confirm
})
</script>
通过绑定dom对象title和number,然后通过innerHtml属性显示获取到的今日新增人数。
控制台输入:npm run start
运行程序
五、调整样式
接下来在main,js调用BrowserWindow的构造方法中修改窗口大小、初始位置、无边框,并隐藏标题栏
let win = new BrowserWindow({
width:300,
height:220,
x:100,
y:200,
frame: false,
// transparent: true, // 窗体透明
resizable: false, // 窗口大小不可调
titleBarStyle:'hidden',
...
titleBarStyle:'hidden'
可能只在mac生效,windows系统可去掉
在index.html的div#title上新增div作为自定义的app头部
<div class="app-control">疫情实况</div>
然后新加style样式,使得.app-control可以拖拽,文字居中,并带下边框
.app-control{
height: 30px;
border-bottom: 1px solid whitesmoke;
-webkit-app-region: drag;
display: grid;
place-items: center;
}
引入线上的样式重置代码
<link href="https://cdn.bootcss.com/normalize/8.0.1/normalize.css" rel="stylesheet">
调整其他样式:
.container{
display: grid;
place-items: center;
flex: 1;
}
html,body{
height: 100%;
overflow: hidden;
}
body{
display: flex;
flex-direction: column;
}
h1{
text-align: center;
}
#title{
font-size: 20vmin;
}
#number{
font-size: 30vmin;
}
六、打包
制作图标并用工具制作为256*256的ico格式,存放于项目根目录
在package,json中设置electron-builder的打包配置
"build": {
"electronDownload":{
"mirror":"https://npm.taobao.org/mirrors/electron/"
},
"productName":"疫情实况",
"appId":"com.aimooc.top",
"mac":{
"category":"public.app-category.developer-tools"
},
"win":{
"target":{
"target":"nsis",
"arch":"ia32"
}
},
"nsis": {
"oneClick": false,
"allowElevation": true,
"allowToChangeInstallationDirectory": true,
"createDesktopShortcut": true,
"createStartMenuShortcut": true
}
},
配置学习可见:electron打包(electron-builder/electron-packager)及打包过程中的报错解决
配置打包命令
"build":"electron-builder"
控制台中执行命令npm run build
进行打包
没有问题,项目即完成
七、主要代码
工程目录
.
|-- LICENSE
|-- README.en.md
|-- README.md
|-- icon.ico
|-- index.html
|-- main.js
`-- package.json
package.json
{
"name": "g-disease",
"version": "1.0.0",
"description": "使用electron制作的一个疫情实况的小程序",
"main": "main.js",
"build": {
"electronDownload":{
"mirror":"https://npm.taobao.org/mirrors/electron/"
},
"productName":"疫情实况",
"appId":"com.aimooc.top",
"mac":{
"category":"public.app-category.developer-tools"
},
"win":{
"target":{
"target":"nsis",
"arch":"ia32"
}
},
"nsis": {
"oneClick": false,
"allowElevation": true,
"allowToChangeInstallationDirectory": true,
"createDesktopShortcut": true,
"createStartMenuShortcut": true
}
},
"scripts": {
"start": "nodemon -e js,html --ignore ./package.json --exec electron .",
"build":"electron-builder"
},
"repository": {
"type": "git",
"url": "https://gitee.com/OliverDaDa/g-disease.git"
},
"keywords": [],
"author": "AImooc-Oliver",
"license": "ISC",
"devDependencies": {
"electron": "^8.2.0",
"electron-builder": "^22.4.1",
"nodemon": "^2.0.2"
}
}
main.js
const { app, BrowserWindow } = require('electron')
function createWindow(){
let win = new BrowserWindow({
width:300,
height:220,
x:100,
y:200,
frame: false,
resizable: false, // 窗口大小不可调
webPreferences:{
nodeIntegration: true
}
})
win.loadFile('./index.html')
}
app.whenReady().then(()=>createWindow())
index.html
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>今日疫情</title>
<style>
.app-control{
height: 30px;
border-bottom: 1px solid whitesmoke;
-webkit-app-region: drag;
display: grid;
place-items: center;
}
.container{
display: grid;
place-items: center;
flex: 1;
}
html,body{
height: 100%;
overflow: hidden;
}
body{
display: flex;
flex-direction: column;
}
h1{
text-align: center;
margin: 0;
}
#title{
font-size: 20vmin;
color: mediumvioletred;
}
#number{
font-size: 30vmin;
color: mediumslateblue;
text-decoration: underline;
}
</style>
</head>
<body>
<div class="app-control">疫情实况</div>
<div class="container">
<div class="row">
<h1 id="title">今日新增</h1>
<h1 id="number">获取中...</h1>
</div>
</div>
<script>
const title = document.querySelector('#title')
const number = document.querySelector('#number')
fetch('https://c.m.163.com/ug/api/wuhan/app/data/list-total?t=317162642162')
.then(res=>res.json()) //text()
.then(data=>{
const confirm = data.data.chinaTotal.today.confirm
number.innerHTML = confirm
})
</script>
</body>
</html>
完整项目地址:g-disease
成品下载地址:疫情实况 Setup 1.0.0.exe
八、致谢
感谢视频作者分享的实战项目,侵权立删!
如有疑问欢迎留言交流!