复习一下
搜索与二叉树
1.查找无序列表
2.查找有序列表---二分法查找
3.二叉树
1.树与二叉树
2.遍历
树的非线性数据结构让我们可以实现更快的算法
重要:
A tree is a set of nodes storing elements in a parent-child relationship with the
following properties:
1.each non-empty tree has a root node.
2.each node different from the root node has a parent node
简略:
A tree is a set of nodes storing elements in a parent-child relationship.
重要:
Each node in a binary tree can have at most two children.
1.left child
It is the root node of the left subtree of the node.
2.right child
It is the root node of the right subtree of the node.
1.A is the root.
2.B is the parent of D and E.
3.D is a child of B ( left child)
4.E is a child of B (right child)
1.H,E,I,J,G are leaf nodes of the tree
2.A,B,C,D,F are non-leaf nodes of the tree
3.FIJ is a subtree (left subtree of node C)
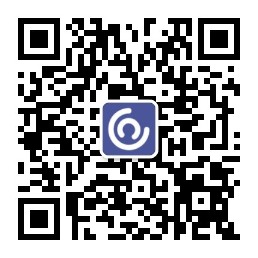
path, depth and height
1.A path of two nodes begins at the starting node and goes from node to node along the edges that join them until the ending node.
2.The length of a path is the number of the edges that compose it.
3.The depth of a node is the length of the path between the root and the node.
4.The height of a tree is the maxium depth of a leaf node.
The length of the path is 3.
The depth of I is 3.
The height of the tree is 3.
Binary Search Tree
A binary search tree is a binary tree whose nodes contain comparable object and are organized as follows.
1.for each node n in the tree
1.The data in the node n is greater than all data values stored in its left subtree T left.
简略:
A binary search tree is a binary tree whose nodes contain comparable object and are organized as values.
Why use binary search trees?
Support fast search.
The pre-order traversal is A-B-D-H-I-E-C-F-J-K-G.
The pre-order traversal is A-B-D-C-E-G-F-H-I.
The in-order traversal is B-D-A-G-E-C-H-F-I.
The post-order traversal is D-B-G-E-H-I-F-C-A.
Regular Expression
A regular expression is a special sequence of characters that are used to find and match a set of strings.
C.
def search_by_id(student_id):
for item in records:
if item[ID]==student_id:
return item[NAME]
return None
第01题。
用递归写一个二分法查找
# 用递归写二分法查找
def search(l, element):
return binary_search(l, 0, len(l) - 1, element)
def binary_search(list, start, end, element):
if start > end:
return False
mid = (end - start) // 2 + start
if list[mid] == element:
return True
elif list[mid] > element:
return binary_search(list, start, mid - 1, element)
else:
return binary_search(list, mid + 1, end, element)
l = [2, 4, 5, 43, 56, 65, 435, 654, 543, 654, 543, 54, 43, 5, 6, 5, 34, 54, 7, 6, 4, 5]
l.sort()
print(l)
print(search(l, 5))
print(search(l, 4))
print(search(l, 2))
print(search(l, 543))
print(search(l, 54))
print(search(l, 43))
print(search(l, 5434))
第02题。
Why use a tree?
Some data must be categorized into groups and subgroups
Tree's non-linear data structure allows us to implement faster algorithms.
第03题。
What is a tree?
A tree is a set of nodes storing elements in a parent-child relationship.
第04题。
What is a binary tree?
A binary tree is a tree that each node have at most two children.(left child and right child)
第05题。
What is path, length, depth and height?
A path of two nodes begin from starting node to the end node.
The length of a path is the number of the edges.
The depth of a node is the length of the path between the root and the node.
The height of a tree is the maximum depth of a leaf node.
第06题。
How is pre-order traversal, in-order traversal and post-order traversal
in-order
post-order
第07题。
What is a binary search tree?
a binary search tree is a binary tree whose nodes have comparable objects and organized as values.
第08题。
Why use a binary search tree?
Because binary search tree can support fast search.
第10周
第01题。
What is recursion?
Recursion is a programming technique that a method calls itself.
第02题。
Can you write a function to calculate the triangle loop?
For example: 1+2+3+4+5=15, 6!=21,7!=28
def triangle_loop(n):
sum = 0
while n > 0:
sum = sum + n
n = n - 1
return sum
print(triangle_loop(6))
print(triangle_loop(7))
print(triangle_loop(8))
第03题。
Can you use the recursion to calculate triangle loop?
def triangle_loop(n):
if n == 1:
return 1
else:
return n + triangle_loop(n - 1)
print(triangle_loop(6))
print(triangle_loop(7))
print(triangle_loop(8))
第04题。
What is characteristics of recursive methods?
1.It calls itself.
2.It breaks the problem into smaller problems.
3.At least one of the problems should have a solution.
第05题。
Is recursive efficient?
1.Recursive methods may involve more overhead.
2.Recursive is usually used because it simplifies a problem conceptually, not more efficient.
第06题。
Write a bubble sort
# bubble sorting
def bubble_sort(l):
for i in range(0, len(l) - 1):
for j in range(i + 1, len(l)):
if l[i] > l[j]:
l[i], l[j] = l[j], l[i]
l = [3, 43, 5, 4, 6, 65, 65, 43, 4, 5, 5, 54, 54, 34, 3, 43, 43, 43]
bubble_sort(l)
print(l)
第07题。
Write a selection sort.
# selection sort
def selection_sort(l):
for i in range(0, len(l)):
min = i
for j in range(i, len(l)):
if l[min] > l[j]:
min=j
l[i],l[min]=l[min],l[i]
l = [3, 43, 5, 4, 6, 65, 65, 43, 4, 5, 5, 54, 54, 34, 3, 43, 43, 43]
selection_sort(l)
print(l)
第08题。
Write a inserting sort.
# insertion sort
def insertion_sort(l):
for j in range(0, len(l)):
num = l.pop(j)
for i in range(0, len(l)):
if num < l[i]:
l.insert(i, num)
break
elif i == len(l) - 1:
l.insert(len(l) - 1, num)
l = [3, 43, 5, 4, 6, 65, 65, 43, 4, 5, 5, 54, 54, 34, 3, 43, 43, 43]
insertion_sort(l)
print(l)