文章目录
matplotlib图形绘制
通过哔哩哔哩视频教程“莫烦python”学习,大部分是自己敲写代码整理
1. 基础绘图
import matplotlib.pyplot as plt
import numpy as np
#显示静态图像
%matplotlib inline
x=np.linspace(-1,1,50)#-1到1中画50个点
y=x**2
plt.figure(num=1,figsize=(15,10))#num=1图片编号,图片大小figsize
plt.plot(x,y)
#改变纵横坐标刻度颜色
plt.tick_params(axis='x',colors='white')
plt.tick_params(axis='y',colors='white')
plt.show()
2. 画多张图
import matplotlib.pyplot as plt
import numpy as np
#显示静态图像
%matplotlib inline
x=np.linspace(-3,3,50)#-1到1中画50个点
y1=2*x+1
y2=x**2
####第一张图
plt.figure(num=1,figsize=(15,10))
plt.plot(x,y1)
plt.tick_params(axis='x',colors='red')#默认纵横坐标都设置,改变纵横坐标刻度颜色,labelsize=15,可以用来更改坐标字体大小
plt.tick_params(axis='y',colors='red')
####第二张图
plt.figure(num=2,figsize=(15,10))
plt.plot(x,y2)
plt.plot(x,y1,color='red',linewidth=1.0,linestyle='--')
plt.tick_params(axis='x',colors='red')
plt.tick_params(axis='y',colors='red')
plt.show()
3. 修改坐标轴参数\坐标刻度用文字代替
import matplotlib.pyplot as plt
import numpy as np
#显示静态图像
%matplotlib inline
x=np.linspace(-3,3,50)#-1到1中画50个点
y1=2*x+1
y2=x**2
plt.figure(figsize=(15,10))
plt.plot(x,y2)
plt.plot(x,y1,color='red',linewidth=1.0,linestyle='--')
plt.tick_params(colors='red',labelsize=15)#默认纵横坐标都设置,改变纵横坐标刻度颜色
# plt.tick_params(axis='y',colors='white')
plt.xlim((-1,2))
plt.ylim((-2,3))
plt.xlabel(r'$I\ am\ x$',color='red',fontdict={'size':20})
plt.ylabel(r'$I\ am\ y$',color='red',fontdict={'size':20})
# new_ticks=np.linspace(-1,2,5)
# print(new_ticks)
plt.xticks(new_ticks)#设置取值的点。
#####r'$really\ bad$':r表示正则表达式,$是以数学的形式,显示字体,比较美观;反斜杠”\“转义字符,输出空格。
plt.yticks([-2,-1.8,-1,1.22,3],[r'$really\ bad$',r'$bad\ \alpha$',r'normal',r'$good$',r'$really good$'])
plt.show()
4. 设置坐标轴位置gca
import matplotlib.pyplot as plt
import numpy as np
#显示静态图像
%matplotlib inline
x=np.linspace(-3,3,50)#-1到1中画50个点
y1=2*x+1
y2=x**2
plt.figure(figsize=(15,10))
plt.plot(x,y2)
plt.plot(x,y1,color='red',linewidth=1.0,linestyle='--')
plt.tick_params(colors='red',labelsize=15)#默认纵横坐标都设置,改变纵横坐标刻度颜色
# plt.tick_params(axis='y',colors='red')
plt.xlim((-1,2))
plt.ylim((-2,3))
plt.xlabel(r'$I\ am\ x$',color='red',fontdict={'size':20})
plt.ylabel(r'$I\ am\ y$',color='red',fontdict={'size':20})
new_ticks=np.linspace(-1,2,5)
# print(new_ticks)
plt.xticks(new_ticks)#设置取值的点。
#####r'$really\ bad$':r表示正则表达式,$是以数学的形式,显示字体,比较美观;反斜杠”\“转义字符,输出空格。
plt.yticks([-2,-1.8,-1,1.22,3],[r'$really\ bad$',r'$bad\ \alpha$',r'normal',r'$good$',r'$really good$'])
#gca=plt.gca
ax=plt.gca()
ax.spines['right'].set_color('green')#设置右坐标轴属性
ax.spines['top'].set_color('green')#设置顶坐标轴属性
ax.spines['bottom'].set_color('green')#设置右坐标轴属性
ax.spines['left'].set_color('green')#设置顶坐标轴属性
ax.spines['right'].set_color('none')#设置右坐标轴属性
ax.spines['top'].set_color('none')#设置顶坐标轴属性
ax.xaxis.set_ticks_position('bottom')
ax.yaxis.set_ticks_position('left')
ax.spines['bottom'].set_position(('data',0))#设置横坐标位置为纵坐标轴的-1位置
ax.spines['left'].set_position(('data',0))
plt.show()
5. 图例(legend)设置
import matplotlib.pyplot as plt
import numpy as np
#显示静态图像
%matplotlib inline
x=np.linspace(-3,3,50)#-1到1中画50个点
y1=2*x+1
y2=x**2
plt.figure(figsize=(8,6))
plt.tick_params(colors='red',labelsize=15)
plt.xlim((-1,2))
plt.ylim((-2,3))
plt.xlabel(r'$I\ am\ x$',color='red',fontdict={'size':20})
plt.ylabel(r'$I\ am\ y$',color='red',fontdict={'size':20})
new_ticks=np.linspace(-1,2,5)
plt.xticks(new_ticks)
plt.yticks([-2,-1.8,-1,1.22,3],[r'$really\ bad$',r'$bad\ \alpha$',r'normal',r'$good$',r'$really good$'])
l1,=plt.plot(x,y2,label='up')###把返回值传进handle中,所以获取其返回值,并且加一个”,“
l2,=plt.plot(x,y1,color='red',linewidth=1.0,linestyle='--',label='best')###把返回值传进handle中,所以获取其返回值,并且加一个”,“
###############################################################
# plt.legend()这样也可以
########################也可以设置参数
plt.legend(handles=[l1,l2],labels=['aaa','bbb'],loc='best')#label设置名字
plt.show()
6. 图片中设置注解、标注
import matplotlib.pyplot as plt
import numpy as np
x=np.linspace(-3,3,50)
y=2*x+1
plt.figure(num=1,figsize=(15,10))
plt.plot(x,y)
# plt.scatter(x,y)
ax=plt.gca()
ax.spines['right'].set_color('none')#设置右坐标轴属性
ax.spines['top'].set_color('none')#设置顶坐标轴属性
ax.xaxis.set_ticks_position('bottom')
ax.yaxis.set_ticks_position('left')
ax.spines['bottom'].set_position(('data',0))#设置横坐标位置为纵坐标轴的-1位置
ax.spines['left'].set_position(('data',0))
x0=1
y0=2*x0+1
plt.scatter(x0,y0,color='b')
plt.plot([x0,x0],[y0,0],'k--',lw=2.5)
# method1
####################################
# plt.annotate(r'$2x+1=%s$' % y0,xy=(x0,y0),xycoords='data',xytext=(+20,-10),)
##xycoords='data'表示根据数据点设置坐标,xytext=(+10,-10):表示文字距离坐标点的位置依据textcoords='offset points'
#connectionstyle='arc3,rad=.5’设置连接线的性质,arc3:弧线,rad:弧度
plt.annotate(r"$2x+1=%s$" % y0,xy=(x0,y0),xycoords='data',
xytext=(+20,-10),textcoords='offset points',fontsize=16,
arrowprops=dict(arrowstyle="->",connectionstyle='arc3,rad=.5'))
# method2
plt.text(-3.2,5,r'$This\ is\ a\ beautiful\ axis:\ \gamma\ \sigma_i\ \alpha_t $',fontdict={'size':16,'color':'red'})
plt.show()
7. 图像遮挡坐标处理
import matplotlib.pyplot as plt
import numpy as np
x=np.linspace(-3,3,50)
y=0.1*x
plt.figure(num=1,figsize=(15,10))
plt.plot(x,y,linewidth=10)#zorder=1为了突显被遮挡的坐标,要比后面的zorder数值小才可以
plt.ylim(-2,2)
ax1=plt.gca()
ax1.spines['right'].set_color('none')#设置右坐标轴属性
ax1.spines['top'].set_color('none')#设置顶坐标轴属性
ax1.xaxis.set_ticks_position('bottom')
ax1.spines['bottom'].set_position(('data',0))#设置横坐标位置为纵坐标轴的-1位置
ax1.yaxis.set_ticks_position('left')
ax1.spines['left'].set_position(('data',0))
plt.text(-3.2,1,'处理之前的图像',fontdict={'size':28,'color':'red'})
plt.show()
########################################################################
plt.figure(num=2,figsize=(15,10))#放在图像属性,开始设置位置前
plt.plot(x,y,linewidth=10,zorder=1)#zorder=1为了突显被遮挡的坐标,要比后面的zorder数值小才可以
plt.ylim(-2,2)
ax=plt.gca()
ax.spines['right'].set_color('none')#设置右坐标轴属性
ax.spines['top'].set_color('none')#设置顶坐标轴属性
ax.xaxis.set_ticks_position('bottom')
ax.spines['bottom'].set_position(('data',0))#设置横坐标位置为纵坐标轴的-1位置
ax.yaxis.set_ticks_position('left')
ax.spines['left'].set_position(('data',0))
for label in ax.get_xticklabels()+ax.get_yticklabels():
label.set_zorder(2)#zorder=2为了突显被遮挡的坐标,比前面zorder数值大
label.set_fontsize(12)#更改刻度字体大小
label.set_bbox(dict(facecolor='white',edgecolor='none',alpha=0.7))#调整刻度背景,边缘,alpha为透明度设置
plt.text(-3.2,1,'处理之后的图像',fontdict={'size':28,'color':'red'})
plt.show()
8. Scatter散点图的绘制
import matplotlib.pyplot as plt
import numpy as np
n=1024
x=np.random.normal(0,1,n)#均值为0,方差为1的1024个随机点
y=np.random.normal(0,1,n)
T=np.arctan2(y,x)#设置颜色值
plt.figure(num=1,figsize=(15,10))
plt.tick_params(colors='red',labelsize=15)
plt.scatter(x,y,s=75,c=T,alpha=0.5)#s:size,c:color,
plt.xlim((-1.5,1.5))
plt.ylim((-1.5,1.5))
################隐藏刻度#########################
plt.xticks(())
plt.yticks(())
plt.show()
9. 生成柱状图
import matplotlib.pyplot as plt
import numpy as np
n=12
X=np.arange(n)
Y1=(1 - X /float(n))*np.random.uniform(0.5, 1.0, n)
Y2=(1 - X /float(n))*np.random.uniform(0.5, 1.0, n)
plt.figure(num=1,figsize=(15,10))
plt.bar(X, +Y1,facecolor='#9999ff',edgecolor='white')
plt.bar(X, -Y2,facecolor='#ff9999',edgecolor='white')
for x, y in zip(X,Y1):
# ha: horizontal alignment:横向对齐
# va: vertical alignment:纵向对齐
#x , y + 0.05设置文本坐标点,不是坐标值,坐标值由%.2f输出
plt.text(x , y + 0.05, '%.2f' % y, ha='center', va='bottom')
for x, y in zip(X, Y2):
# ha: horizontal alignment
# va: vertical alignment
plt.text(x, -y - 0.05, '%.2f' % y, ha='center', va='top')
plt.xlim(-.5, n)
plt.xticks(())#隐藏坐标刻度值
plt.ylim(-1.25, 1.25)
plt.yticks(())#隐藏坐标刻度值
plt.show()
10. 等高线(Contours)图
import matplotlib.pyplot as plt
import numpy as np
def f(x,y):
return (1-x/2+x**5+y**3)*np.exp(-x**2-y**2)
n=256
x=np.linspace(-3,3,n)
y=np.linspace(-3,3,n)
plt.figure(num=1,figsize=(15,10))
X,Y=np.meshgrid(x,y)#meshgrid函数用两个坐标轴上的点在平面上画网格。
plt.contourf(X,Y,f(X,Y),8,alpha=0.9,cmap=plt.cm.cool)#filling表示fill颜色,8表示8中颜色,alpha表示透明度,cmap用颜色表示f(X,Y)的值
#画等高线
C=plt.contour(X,Y,f(X,Y),8,colors='black',linewidths=0.5)#8表示8+2条等高线
plt.xticks(())#隐藏坐标刻度值
plt.yticks(())#隐藏坐标刻度值
###等高线表值
plt.clabel(C,inline=True,fontsize=10)
plt.show()
11. 数值图片(image)
import matplotlib.pyplot as plt
import numpy as np
# image data
a = np.array([0.313660827978, 0.365348418405, 0.423733120134,
0.365348418405, 0.439599930621, 0.525083754405,
0.423733120134, 0.525083754405, 0.651536351379]).reshape(3,3)
"""
for the value of "interpolation", check this:
http://matplotlib.org/examples/images_contours_and_fields/interpolation_methods.html
for the value of "origin"= ['upper', 'lower'], check this:
http://matplotlib.org/examples/pylab_examples/image_origin.html
"""
plt.figure(num=1,figsize=(15,10))
plt.imshow(a, interpolation='nearest', cmap='bone', origin='lower')#展示图片,interpolation:表示空白处插值的方式,origin=upper改变图片排列方式
plt.colorbar(shrink=.92)#shrink=.92压缩colorbar
plt.xticks(())
plt.yticks(())
plt.show()
12. 3D图像
import numpy as np
import matplotlib.pyplot as plt
from mpl_toolkits.mplot3d import Axes3D
fig=plt.figure(num=1,figsize=(15,10))
ax = Axes3D(fig)
# X, Y value
X = np.arange(-4, 4, 0.25)
Y = np.arange(-4, 4, 0.25)
X, Y = np.meshgrid(X, Y)
R = np.sqrt(X ** 2 + Y ** 2)
# height value
Z = np.cos(R)
ax.plot_surface(X, Y, Z, rstride=1, cstride=1, cmap=plt.get_cmap('rainbow'),edgecolors='black')
"""
============= ================================================
Argument Description
============= ================================================
*X*, *Y*, *Z* Data values as 2D arrays
*rstride* Array row stride (step size), defaults to 10
*cstride* Array column stride (step size), defaults to 10
*color* Color of the surface patches
*cmap* A colormap for the surface patches.
*facecolors* Face colors for the individual patches
*norm* An instance of Normalize to map values to colors
*vmin* Minimum value to map
*vmax* Maximum value to map
*shade* Whether to shade the facecolors
============= ================================================
"""
# I think this is different from plt12_contours
ax.contourf(X, Y, Z, zdir='z', offset=-2, cmap=plt.get_cmap('rainbow'))#投影等高线,改变zdir='x', offset=-4实现投影到不同坐标轴
"""
========== ================================================
Argument Description
========== ================================================
*X*, *Y*, Data values as numpy.arrays
*Z*
*zdir* The direction to use: x, y or z (default)
*offset* If specified plot a projection of the filled contour
on this position in plane normal to zdir
========== ================================================
"""
ax.set_zlim(-2, 2)
plt.show()
13. 多子图(subplot)
import numpy as np
import matplotlib.pyplot as plt
plt.figure(num=1,figsize=(15,10))
plt.subplot(2,2,1)
plt.plot([0,1],[0,1])
plt.tick_params(colors='white',labelsize=15)
plt.subplot(2,2,2)
plt.plot([0,1],[0,2])
plt.tick_params(colors='white',labelsize=15)
plt.subplot(2,2,3)
plt.plot([0,1],[0,1])
plt.tick_params(colors='white',labelsize=15)
plt.subplot(2,2,4)
plt.plot([0,1],[0,2])
plt.tick_params(colors='white',labelsize=15)
plt.show()
import numpy as np
import matplotlib.pyplot as plt
plt.figure(num=1,figsize=(15,10))
plt.subplot(2,1,1)#第一行一列
plt.plot([0,1],[0,1])
plt.tick_params(colors='white',labelsize=15)
plt.subplot(2,3,4)#第二行三列
plt.plot([0,1],[0,2])
plt.tick_params(colors='white',labelsize=15)
plt.subplot(2,3,5)
plt.plot([0,1],[0,1])
plt.tick_params(colors='white',labelsize=15)
plt.subplot(2,3,6)
plt.plot([0,1],[0,2])
plt.tick_params(colors='white',labelsize=15)
plt.show()
14. 图中图
import matplotlib.pyplot as plt
fig = plt.figure()
x = [1, 2, 3, 4, 5, 6, 7]
y = [1, 3, 4, 2, 5, 8, 6]
# below are all percentage
left, bottom, width, height = 0.1, 0.1, 0.8, 0.8
ax1 = fig.add_axes([left, bottom, width, height]) # main axes
ax1.plot(x, y, 'r')
ax1.set_xlabel('x')
ax1.set_ylabel('y')
ax1.set_title('title')
plt.tick_params(colors='white',labelsize=15)
ax2 = fig.add_axes([0.2, 0.6, 0.25, 0.25]) # inside axes
ax2.plot(y, x, 'b')
ax2.set_xlabel('x')
ax2.set_ylabel('y')
ax2.set_title('title inside 1')
# different method to add axes
####################################
plt.axes([0.6, 0.2, 0.25, 0.25])
plt.plot(y[::-1], x, 'g')
plt.xlabel('x')
plt.ylabel('y')
plt.title('title inside 2')
plt.show()
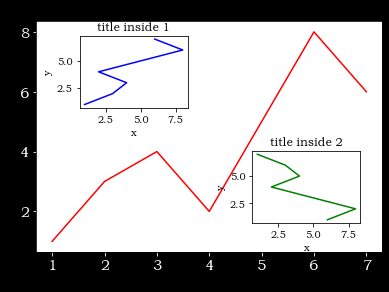
15. 主次坐标轴
import matplotlib.pyplot as plt
import numpy as np
%matplotlib inline
x = np.arange(0, 10, 0.1)
y1 = 0.05 * x**2
y2 = -1 *y1
fig, ax1 = plt.subplots(figsize=(15,10))##subplot设置画布大小
ax2 = ax1.twinx() # mirror the ax1
ax1.plot(x, y1, 'g-')
ax2.plot(x, y2, 'b-')
ax1.set_xlabel('X data',color='white',fontdict={'size':20})
ax1.set_ylabel('Y1 data',color='g',fontdict={'size':20})
ax1.tick_params(colors='white',labelsize=15)
ax2.set_ylabel('Y2 data', color='b',fontdict={'size':20})
ax2.tick_params(colors='white',labelsize=15)
plt.show()
16. 动画(Animation)
import numpy as np
from matplotlib import pyplot as plt
from matplotlib import animation
fig, ax = plt.subplots()
x = np.arange(0, 2*np.pi, 0.01)
line, = ax.plot(x, np.sin(x))
def animate(i):
line.set_ydata(np.sin(x + i/10.0)) # update the data
return line,
# Init only required for blitting to give a clean slate.
def init():
line.set_ydata(np.sin(x))
return line,
# call the animator. blit=True means only re-draw the parts that have changed.
# blit=True dose not work on Mac, set blit=False
# interval= update frequency
ani = animation.FuncAnimation(fig=fig, func=animate, frames=100, init_func=init,
interval=20, blit=False)
# save the animation as an mp4. This requires ffmpeg or mencoder to be
# installed. The extra_args ensure that the x264 codec is used, so that
# the video can be embedded in html5. You may need to adjust this for
# your system: for more information, see
# http://matplotlib.sourceforge.net/api/animation_api.html
# anim.save('basic_animation.mp4', fps=30, extra_args=['-vcodec', 'libx264'])
plt.show()