springsecurity配置
第一步创建SecurityConfig
/**
* SpringSecurity的配置
*/
@Configuration
@EnableWebSecurity
@EnableGlobalMethodSecurity(prePostEnabled=true)
public class SecurityConfig extends WebSecurityConfigurerAdapter {
//认证成功逻辑
@Autowired
MyAuthenticationSucessHandler authenticationSucessHandler;
//认证失败逻辑
@Autowired
private MyAuthenticationFailureHandler authenticationFailureHandler;
@Override
protected void configure(HttpSecurity http) throws Exception {
http.formLogin() // 表单登录
// http.httpBasic() // HTTP Basic
.loginPage("/authentication/require")
.loginProcessingUrl("/login")
.successHandler(authenticationSucessHandler)//处理登陆成功
.failureHandler(authenticationFailureHandler) // 处理登录失败
.and()
.authorizeRequests() // 授权配置
.antMatchers("/authentication/require","/login.html").permitAll()
.anyRequest() // 所有请求
.authenticated() // 都需要认证
.and()
.csrf()
.disable();
}
//密码加密方式
@Bean
public PasswordEncoder passwordEncoder() {
return new BCryptPasswordEncoder();
}
}
写一个登陆页面
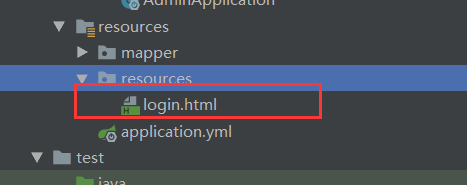
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>登录</title>
</head>
<body>
<form action="/login" method="post">
<div class="form">
<h3>账户登录</h3>
<input type="text" placeholder="用户名" name="username" required="required" />
<input type="password" placeholder="密码" name="password" required="required" />
<button type="submit">登录</button>
</div>
</form>
</body>
</html>
写登陆成功逻辑
@Component
public class MyAuthenticationSucessHandler implements AuthenticationSuccessHandler {
private RequestCache requestCache = new HttpSessionRequestCache();
private RedirectStrategy redirectStrategy = new DefaultRedirectStrategy();
@Override
public void onAuthenticationSuccess(HttpServletRequest httpServletRequest, HttpServletResponse httpServletResponse, Authentication authentication) throws IOException, ServletException {
SavedRequest savedRequest = requestCache.getRequest(httpServletRequest, httpServletResponse);
//登录成功后页面将跳转回引发跳转的页面
// redirectStrategy.sendRedirect(httpServletRequest, httpServletResponse, savedRequest.getRedirectUrl());
redirectStrategy.sendRedirect(httpServletRequest, httpServletResponse, "/index");
}
}
写登陆失败逻辑
@Component
public class MyAuthenticationFailureHandler implements AuthenticationFailureHandler {
@Override
public void onAuthenticationFailure(HttpServletRequest request, HttpServletResponse response, AuthenticationException exception) throws IOException, ServletException {
response.setStatus(HttpStatus.INTERNAL_SERVER_ERROR.value());
response.setContentType("application/json;charset=utf-8");
response.getWriter().write(exception.getMessage());
}
}
控制器那边
//Spring Security提供的用于缓存请求的对象,通过调用它的getRequest方法可以获取到本次请求的HTTP信息
private RequestCache requestCache = new HttpSessionRequestCache();
//DefaultRedirectStrategy的sendRedirect为Spring Security提供的用于处理重定向的方法
private RedirectStrategy redirectStrategy = new DefaultRedirectStrategy();
@GetMapping("/authentication/require")
@ResponseStatus(HttpStatus.UNAUTHORIZED)//返回错误号码
public String requireAuthentication(HttpServletRequest request, HttpServletResponse response) throws IOException {
SavedRequest savedRequest = requestCache.getRequest(request, response);
if (savedRequest != null) {
String targetUrl = savedRequest.getRedirectUrl();
//如果后缀为.html都给他重定向到login.html
if (StringUtils.endsWithIgnoreCase(targetUrl, ".html"))
redirectStrategy.sendRedirect(request, response, "/login.html");
}
return "访问的资源需要身份认证!";
}
@GetMapping("index")
public Object index(){
//利用此方法可以获取权限信息
return SecurityContextHolder.getContext().getAuthentication();
}