SpringBoot开发web项目
1、新建应该SpringBoot项目
我们先启动一下,访问localhost:8080,可以发现,这个网站没有首页,所以我们先定制一个首页!
2、首页定制
在我们的静态资源目录下,新建一个index.html文件
资源存放目录说明
我们可以看到,只有两个目录
static:静态资源
templates:页面,下面的目录只能通过controller来访问
但是我们点进源码
扫描二维码关注公众号,回复:
9602150 查看本文章
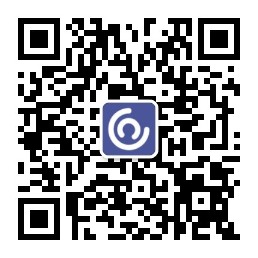
可以看到,源码中还有两个目录可以存放资源:
resources:也可以存放资源文件
public:静态资源公共的可以放在这里
一般用上面两个就够!
在public、static、resources下创建index.html,都可以当做首页访问到!
但是在templates下只能通过controller来跳转,而且需要导入thymeleaf依赖!
使用thymeleaf
导入静态资源模板即使用html编写的页面
页面跳转
- 导入对应maven依赖
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-thymeleaf</artifactId>
</dependency>
- 编写html页面放到templtes目录下
- 使用controller进行跳转
package com.star.controller;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.RequestMapping;
@Controller
public class IndexController {
//只要访问了 / 就会跳转到 templates目录下的 index 页面,而首页即就是访问 /
@RequestMapping("/")
public String index(){
return "index";
}
@RequestMapping("/user/index")
public String userIndex(){
return "user/index";
}
@RequestMapping("/user/user")
public String userUser(){
return "user/user";
}
}
测试结果:
页面传递值
我们查看thymelef帮助文档
在后端方法中使用Model传递值
在前端使用th:xxx去接收后端传递值,注意:一定要导入头文件约束
index页面
<!DOCTYPE html>
<html lang="en" xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>首页</title>
</head>
<body>
<h1>首页</h1>
<!--普通取值-->
<p th:text="${msg}"></p>
<!--遍历循环 接收后端传递的users,遍历的结果都是user,可以在标签内使用!-->
<h1 th:each="user:${users}" th:text="${user}"></h1>
<!--行内写法-->
<h1 th:each="user:${users}">[[${user}]]</h1>
</body>
</html>
测试类
@RequestMapping("/")
public String index(Model model){
model.addAttribute("msg","Hello,SpringBoot!");
model.addAttribute("users", Arrays.asList("a","b","c"));
return "index";
}
测试结果: