collections模块
-
collections基于python自带的数据类型之上额外增加的几个数据类型
命名元组
from collections import nametuple
l = nametuple('limit', ['x', 'y'])
print(l(1,2)) # limit(1,2)
limit = l(1,2)
print(limit.x) # 1
print(limit[0]) # 1
双端队列
from collections import deque
l = deque([1,2])
l.append(3)
l.appendleft(0)
l.pop()
l.popleft()
print(l)
队列:先进先出
栈:先进后出
有序字典 -- python3.6 默认是显示有序
from collections import OrderedDict
python2中有用
dic = OrderedDict(k=1,v=11,k1=12)
print(dic)
print(dic.get("k"))
dic.move_to_end("k")
print(dic)
默认字典
from collections import defaultdict
lst = [11,22,33,44,55,66,77,88,99]
dic = defaultdict(list) # 默认字典的值为列表
for i in lst:
if i > 66:
dic['key'].append(i)
else:
dic['key1'].append(i)
print(dict(dic))
计数
from collections import Counter
lst = [1,2,54,1,55,4,74,2,4,16,1,1,2,1,4,5,1]
d = Counter(lst) # d是一个字典
print(d) # Counter({1: 6, 2: 3, 4: 3, 54: 1, 55: 1, 74: 1, 16: 1, 5: 1})
print(dict(d)) # {1: 6, 2: 3, 54: 1, 55: 1, 4: 3, 74: 1, 16: 1, 5: 1}
print(dict(d.most_common())) # 按出现次数的高低排序
from collections import Iterable,Iterator
lst = [1,2,4,5,6]
print(isinstance(lst,list)) # True
print(isinstance(lst,Iterable)) # True
print(isinstance(lst,Iterator)) # False
hashlib
-
hashlib 摘要算法、加密算法
-
加密、校验
md5 加密速度快,安全系数低
sha512 加密速度慢,安全系数高
注意:
扫描二维码关注公众号,回复:
9584289 查看本文章
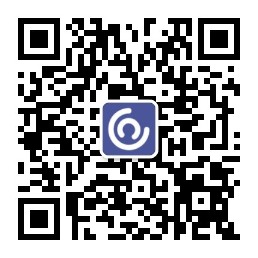
-
当要加密的内容相同时,密文一定是相同的
-
不可逆
加密
import haslib
md5 = haslib.md5() # 初始化
md5.update('beauty'.encode('utf-8')) # 将明文转换成字节添加到新初始化的md5中
print(md5.hexdigest()) # 进行加密
加盐
# 加固定盐
import haslib
md5 = haslib.md5('love'.encode('utf-8')) # 初始化
md5.update('beauty'.encode('utf-8'))
print(md5.hexdigest()) # 进行加密
# 加动态盐
import haslib
user= input("username:")
pwd = input("passweord:")
md5 = hashlib.md5(user.encode("utf-8")) # 初始化
md5.update(pwd.encode("utf-8"))
print(md5.hexdigest()) # 进行加密
pwd = md5.hexdigest()
中文内容相同编码不同时密文不一致,英文的密文都是一致的
import hashlib
sha1 = hashlib.sha1()
sha1.update("我不做大哥好多年".encode("utf-8"))
print(sha1.hexdigest())
cd029abac535b039dc23cbc5c185aa58e928e75f
import hashlib
sha1 = hashlib.sha1()
sha1.update("我不做大哥好多年".encode("gbk"))
print(sha1.hexdigest())
190c52fc9d5b9bd2a72c0c73c6a865e97fb1dfe6
校验
# 校验python解释器的md5值是否相同
import haslib
def file_check(file_path):
with open(file_path,mode='rb') as f1:
sha256 = haslib.md5()
while 1:
content = f1.read(1024)
if content:
sha256.update(content)
else:
return sha256.hexdigest()
print(file_check('python-3.6.6-amd64.exe'))